Programming C# 8.0. Build Cloud, Web, and Desktop Applications - Helion
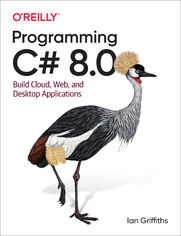
ISBN: 9781492056768
stron: 800, Format: ebook
Data wydania: 2019-11-26
Księgarnia: Helion
Cena książki: 203,15 zł (poprzednio: 236,22 zł)
Oszczędzasz: 14% (-33,07 zł)
C# is undeniably one of the most versatile programming languages available to engineers today. With this comprehensive guide, you’ll learn just how powerful the combination of C# and .NET can be. Author Ian Griffiths guides you through C# 8.0 fundamentals and techniques for building cloud, web, and desktop applications.
Designed for experienced programmers, this book provides many code examples to help you work with the nuts and bolts of C#, such as generics, LINQ, and asynchronous programming features. You’ll get up to speed on .NET Core and the latest C# 8.0 additions, including asynchronous streams, nullable references, pattern matching, default interface implementation, ranges and new indexing syntax, and changes in the .NET tool chain.
- Discover how C# supports fundamental coding features, such as classes, other custom types, collections, and error handling
- Learn how to write high-performance memory-efficient code with .NET Core’s Span and Memory types
- Query and process diverse data sources, such as in-memory object models, databases, data streams, and XML documents with LINQ
- Use .NET’s multithreading features to exploit your computer’s parallel processing capabilities
- Learn how asynchronous language features can help improve application responsiveness and scalability
Osoby które kupowały "Programming C# 8.0. Build Cloud, Web, and Desktop Applications", wybierały także:
- WPF. Kurs video. Om 210,00 zł, (39,90 zł -81%)
- ASP.NET 4 z wykorzystaniem C# i VB. Zaawansowane programowanie 190,00 zł, (39,90 zł -79%)
- ASP.NET Core 6. Kurs video. Rozwijaj aplikacje webowe z Entity Framework Core 173,48 zł, (39,90 zł -77%)
- C# 9.0 w pigułce 173,48 zł, (39,90 zł -77%)
- Debugowanie i refaktoryzacja kodu. Kurs video. Clean code w C# i Visual Studio 166,25 zł, (39,90 zł -76%)
Spis treści
Programming C# 8.0. Build Cloud, Web, and Desktop Applications eBook -- spis treści
- Preface
- Who This Book Is For
- Conventions Used in This Book
- Using Code Examples
- OReilly Online Learning
- How to Contact Us
- Acknowledgments
- 1. Introducing C#
- Why C#?
- C#s Defining Features
- Managed Code and the CLR
- Prefer Generality to Specialization
- C# Standards and Implementations
- Many Microsoft .NETs (Temporarily)
- Targeting Multiple .NET Versions with .NET Standard
- Visual Studio and Visual Studio Code
- Anatomy of a Simple Program
- Adding a Project to an Existing Solution
- Referencing One Project from Another
- Referencing External Libraries
- Writing a Unit Test
- Namespaces
- Nested namespaces
- Classes
- Program Entry Point
- Unit Tests
- Summary
- 2. Basic Coding in C#
- Local Variables
- Scope
- Variable name ambiguity
- Local variable instances
- Scope
- Statements and Expressions
- Statements
- Expressions
- Comments and Whitespace
- Preprocessing Directives
- Compilation Symbols
- #error and #warning
- #line
- #pragma
- #nullable
- #region and #endregion
- Fundamental Data Types
- Numeric Types
- Numeric conversions
- Checked contexts
- BigInteger
- Booleans
- Strings and Characters
- Immutability of strings
- Formatting data in strings
- Verbatim string literals
- Tuples
- Deconstruction
- Dynamic
- Object
- Numeric Types
- Operators
- Flow Control
- Boolean Decisions with if Statements
- Multiple Choice with switch Statements
- Loops: while and do
- C-Style for Loops
- Collection Iteration with foreach Loops
- Patterns
- Getting More Specific with when
- Patterns in Expressions
- Summary
- Local Variables
- 3. Types
- Classes
- Static Members
- Static Classes
- Reference Types
- Banishing null with non-nullable references
- Structs
- When to Write a Value Type
- Guaranteeing Immutability
- Members
- Fields
- Constructors
- Default constructors and zero-argument constructors
- Chaining constructors
- Static constructors
- Deconstructors
- Methods
- Passing arguments by reference
- Reference variables and return values
- Optional arguments
- Variable argument count with the params keyword
- Local functions
- Expression-bodied methods
- Extension methods
- Properties
- Properties and mutable value types
- Indexers
- Initializer Syntax
- Operators
- Events
- Nested Types
- Interfaces
- Default Interface Implementation
- Enums
- Other Types
- Anonymous Types
- Partial Types and Methods
- Summary
- Classes
- 4. Generics
- Generic Types
- Constraints
- Type Constraints
- Reference Type Constraints
- Value Type Constraints
- Value Types All the Way Down with Unmanaged Constraints
- Not Null Constraints
- Other Special Type Constraints
- Multiple Constraints
- Zero-Like Values
- Generic Methods
- Type Inference
- Generics and Tuples
- Inside Generics
- Summary
- 5. Collections
- Arrays
- Array Initialization
- Searching and Sorting
- Multidimensional Arrays
- Jagged arrays
- Rectangular arrays
- Copying and Resizing
- List<T>
- List and Sequence Interfaces
- Implementing Lists and Sequences
- Implementing IEnumerable<T> with Iterators
- Collection<T>
- ReadOnlyCollection<T>
- Addressing Elements with Index and Range Syntax
- System.Index
- System.Range
- Supporting Index and Range in Your Own Types
- Dictionaries
- Sorted Dictionaries
- Sets
- Queues and Stacks
- Linked Lists
- Concurrent Collections
- Immutable Collections
- ImmutableArray<T>
- Summary
- Arrays
- 6. Inheritance
- Inheritance and Conversions
- Interface Inheritance
- Generics
- Covariance and Contravariance
- System.Object
- The Ubiquitous Methods of System.Object
- Accessibility and Inheritance
- Virtual Methods
- Abstract Methods
- Inheritance and Library Versioning
- Sealed Methods and Classes
- Accessing Base Members
- Inheritance and Construction
- Special Base Types
- Summary
- 7. Object Lifetime
- Garbage Collection
- Determining Reachability
- Accidentally Defeating the Garbage Collector
- Weak References
- Reclaiming Memory
- Garbage Collector Modes
- Temporarily Suspending Garbage Collections
- Accidentally Defeating Compaction
- Forcing Garbage Collections
- Destructors and Finalization
- IDisposable
- Optional Disposal
- Boxing
- Boxing Nullable<T>
- Summary
- Garbage Collection
- 8. Exceptions
- Exception Sources
- Exceptions from APIs
- Failures Detected by the Runtime
- Handling Exceptions
- Exception Objects
- Multiple catch Blocks
- Exception Filters
- Nested try Blocks
- finally Blocks
- Throwing Exceptions
- Rethrowing Exceptions
- Failing Fast
- Exception Types
- Custom Exceptions
- Unhandled Exceptions
- Summary
- Exception Sources
- 9. Delegates, Lambdas, and Events
- Delegate Types
- Creating a Delegate
- Multicast Delegates
- Invoking a Delegate
- Common Delegate Types
- Type Compatibility
- Behind the Syntax
- Anonymous Functions
- Captured Variables
- Lambdas and Expression Trees
- Events
- Standard Event Delegate Pattern
- Custom Add and Remove Methods
- Events and the Garbage Collector
- Events Versus Delegates
- Delegates Versus Interfaces
- Summary
- Delegate Types
- 10. LINQ
- Query Expressions
- How Query Expressions Expand
- Supporting Query Expressions
- Deferred Evaluation
- LINQ, Generics, and IQueryable<T>
- Standard LINQ Operators
- Filtering
- Select
- Data shaping and anonymous types
- Projection and mapping
- SelectMany
- Ordering
- Containment Tests
- Specific Items and Subranges
- Aggregation
- Set Operations
- Whole-Sequence, Order-Preserving Operations
- Grouping
- Joins
- Conversion
- Sequence Generation
- Other LINQ Implementations
- Entity Framework
- Parallel LINQ (PLINQ)
- LINQ to XML
- Reactive Extensions
- Tx (LINQ to Logs and Traces)
- Summary
- Query Expressions
- 11. Reactive Extensions
- Fundamental Interfaces
- IObserver<T>
- IObservable<T>
- Implementing cold sources
- Implementing hot sources
- Publishing and Subscribing with Delegates
- Creating an Observable Source with Delegates
- Subscribing to an Observable Source with Delegates
- Sequence Builders
- Empty
- Never
- Return
- Throw
- Range
- Repeat
- Generate
- LINQ Queries
- Grouping Operators
- Join Operators
- SelectMany Operator
- Aggregation and Other Single-Value Operators
- Concat Operator
- Rx Query Operators
- Merge
- Windowing Operators
- Demarcating windows with observables
- The Scan Operator
- The Amb Operator
- DistinctUntilChanged
- Schedulers
- Specifying Schedulers
- ObserveOn
- SubscribeOn
- Passing schedulers explicitly
- Built-in Schedulers
- Specifying Schedulers
- Subjects
- Subject<T>
- BehaviorSubject<T>
- ReplaySubject<T>
- AsyncSubject<T>
- Adaptation
- IEnumerable<T> and IAsyncEnumerable<T>
- .NET Events
- Asynchronous APIs
- Timed Operations
- Interval
- Timer
- Timestamp
- TimeInterval
- Throttle
- Sample
- Timeout
- Windowing Operators
- Delay
- DelaySubscription
- Summary
- Fundamental Interfaces
- 12. Assemblies
- Anatomy of an Assembly
- .NET Metadata
- Resources
- Multifile Assemblies
- Other PE Features
- Win32-style resources
- Console versus GUI
- Type Identity
- Loading Assemblies
- Assembly Resolution
- Explicit Loading
- Isolation and Plugins with AssemblyLoadContext
- Assembly Names
- Strong Names
- Version
- Version numbers and assembly loading
- Culture
- Protection
- Summary
- Anatomy of an Assembly
- 13. Reflection
- Reflection Types
- Assembly
- Module
- MemberInfo
- Type and TypeInfo
- Generic types
- MethodBase, ConstructorInfo, and MethodInfo
- ParameterInfo
- FieldInfo
- PropertyInfo
- EventInfo
- Reflection Contexts
- Summary
- Reflection Types
- 14. Attributes
- Applying Attributes
- Attribute Targets
- Compiler-Handled Attributes
- Names and versions
- Description and related resources
- Caller information attributes
- CLR-Handled Attributes
- InternalsVisibleToAttribute
- Serialization attributes
- JIT compilation
- STAThread and MTAThread
- Interop
- Defining and Consuming Attributes
- Attribute Types
- Retrieving Attributes
- Reflection-only load
- Summary
- Applying Attributes
- 15. Files and Streams
- The Stream Class
- Position and Seeking
- Flushing
- Copying
- Length
- Disposal
- Asynchronous Operation
- Concrete Stream Types
- One Type, Many Behaviors
- Text-Oriented Types
- TextReader and TextWriter
- Concrete Reader and Writer Types
- StreamReader and StreamWriter
- StringReader and StringWriter
- Encoding
- Code page encodings
- Using encodings directly
- Files and Directories
- FileStream Class
- File Class
- Directory Class
- Path Class
- FileInfo, DirectoryInfo, and FileSystemInfo
- Known Folders
- Serialization
- BinaryReader, BinaryWriter, and BinaryPrimitives
- CLR Serialization
- JSON.NET
- JsonSerializer and JsonConvert
- LINQ to JSON
- Summary
- The Stream Class
- 16. Multithreading
- Threads
- Threads, Variables, and Shared State
- Thread-local storage
- The Thread Class
- The Thread Pool
- Launching thread pool work with Task
- Thread creation heuristics
- I/O completion threads
- Thread Affinity and SynchronizationContext
- ExecutionContext
- Threads, Variables, and Shared State
- Synchronization
- Monitors and the lock Keyword
- How the lock keyword expands
- Waiting and notification
- Timeouts
- SpinLock
- Reader/Writer Locks
- Event Objects
- Barrier
- CountdownEvent
- Semaphores
- Mutex
- Interlocked
- Lazy Initialization
- Lazy<T>
- LazyInitializer
- Other Class Library Concurrency Support
- Monitors and the lock Keyword
- Tasks
- The Task and Task<T> Classes
- ValueTask and ValueTask<T>
- Task creation options
- Task status
- Retrieving the result
- Continuations
- Continuation options
- Schedulers
- Error Handling
- Custom Threadless Tasks
- Parent/Child Relationships
- Composite Tasks
- The Task and Task<T> Classes
- Other Asynchronous Patterns
- Cancellation
- Parallelism
- The Parallel Class
- Parallel LINQ
- TPL Dataflow
- Summary
- Threads
- 17. Asynchronous Language Features
- Asynchronous Keywords: async and await
- Execution and Synchronization Contexts
- Multiple Operations and Loops
- Consuming and producing asynchronous sequences
- Asynchronous disposal
- Returning a Task
- Applying async to Nested Methods
- The await Pattern
- Error Handling
- Validating Arguments
- Singular and Multiple Exceptions
- Concurrent Operations and Missed Exceptions
- Summary
- Asynchronous Keywords: async and await
- 18. Memory Efficiency
- (Dont) Copy That
- Representing Sequential Elements with Span<T>
- Utility Methods
- Stack Only
- Representing Sequential Elements with Memory<T>
- ReadOnlySequence<T>
- Processing Data Streams with Pipelines
- Processing JSON in ASP.NET Core
- Summary
- Index