Programming Bitcoin. Learn How to Program Bitcoin from Scratch - Helion
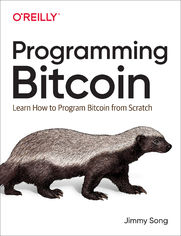
ISBN: 978-14-920-3143-7
stron: 322, Format: ebook
Data wydania: 2019-02-08
Księgarnia: Helion
Cena książki: 203,15 zł (poprzednio: 236,22 zł)
Oszczędzasz: 14% (-33,07 zł)
Dive into Bitcoin technology with this hands-on guide from one of the leading teachers on Bitcoin and Bitcoin programming. Author Jimmy Song shows Python programmers and developers how to program a Bitcoin library from scratch. You’ll learn how to work with the basics, including the math, blocks, network, and transactions behind this popular cryptocurrency and its blockchain payment system.
By the end of the book, you'll understand how this cryptocurrency works under the hood by coding all the components necessary for a Bitcoin library. Learn how to create transactions, get the data you need from peers, and send transactions over the network. Whether you’re exploring Bitcoin applications for your company or considering a new career path, this practical book will get you started.
- Parse, validate, and create bitcoin transactions
- Learn Script, the smart contract language behind Bitcoin
- Do exercises in each chapter to build a Bitcoin library from scratch
- Understand how proof-of-work secures the blockchain
- Program Bitcoin using Python 3
- Understand how simplified payment verification and light wallets work
- Work with public-key cryptography and cryptographic primitives
Osoby które kupowały "Programming Bitcoin. Learn How to Program Bitcoin from Scratch", wybierały także:
- Scratch. Komiksowa przygoda z programowaniem 36,06 zł, (11,90 zł -67%)
- Mały programista. Kurs video. Programowanie gier w Scratch 2.0 59,00 zł, (23,60 zł -60%)
- M 37,00 zł, (18,50 zł -50%)
- Scratch 3 dla najm 39,90 zł, (19,95 zł -50%)
- Młodzi giganci programowania. Scratch. Wydanie II 39,90 zł, (19,95 zł -50%)
Spis treści
Programming Bitcoin. Learn How to Program Bitcoin from Scratch eBook -- spis treści
- Foreword
- Preface
- Who Is This Book For?
- What Do I Need to Know?
- How Is the Book Arranged?
- Setting Up
- Answers
- Conventions Used in This Book
- Using Code Examples
- OReilly Online Learning
- How to Contact Us
- Acknowledgments
- 1. Finite Fields
- Learning Higher-Level Math
- Finite Field Definition
- Defining Finite Sets
- Constructing a Finite Field in Python
- Exercise 1
- Modulo Arithmetic
- Modulo Arithmetic in Python
- Finite Field Addition and Subtraction
- Exercise 2
- Coding Addition and Subtraction in Python
- Exercise 3
- Finite Field Multiplication and Exponentiation
- Exercise 4
- Exercise 5
- Coding Multiplication in Python
- Exercise 6
- Coding Exponentiation in Python
- Exercise 7
- Finite Field Division
- Exercise 8
- Exercise 9
- Redefining Exponentiation
- Conclusion
- 2. Elliptic Curves
- Definition
- Coding Elliptic Curves in Python
- Exercise 1
- Exercise 2
- Point Addition
- Math of Point Addition
- Coding Point Addition
- Exercise 3
- Point Addition for When x1x2
- Exercise 4
- Coding Point Addition for When x1x2
- Exercise 5
- Point Addition for When P1 = P2
- Exercise 6
- Coding Point Addition for When P1 = P2
- Exercise 7
- Coding One More Exception
- Conclusion
- 3. Elliptic Curve Cryptography
- Elliptic Curves over Reals
- Elliptic Curves over Finite Fields
- Exercise 1
- Coding Elliptic Curves over Finite Fields
- Point Addition over Finite Fields
- Coding Point Addition over Finite Fields
- Exercise 2
- Exercise 3
- Scalar Multiplication for Elliptic Curves
- Exercise 4
- Scalar Multiplication Redux
- Mathematical Groups
- Identity
- Closure
- Invertibility
- Commutativity
- Associativity
- Exercise 5
- Coding Scalar Multiplication
- Defining the Curve for Bitcoin
- Working with secp256k1
- Public Key Cryptography
- Signing and Verification
- Inscribing the Target
- Verification in Depth
- Verifying a Signature
- Exercise 6
- Programming Signature Verification
- Signing in Depth
- Creating a Signature
- Exercise 7
- Programming Message Signing
- Conclusion
- 4. Serialization
- Uncompressed SEC Format
- Exercise 1
- Compressed SEC Format
- Exercise 2
- DER Signatures
- Exercise 3
- Base58
- Transmitting Your Public Key
- Exercise 4
- Address Format
- Exercise 5
- WIF Format
- Exercise 6
- Big- and Little-Endian Redux
- Exercise 7
- Exercise 8
- Exercise 9
- Conclusion
- Uncompressed SEC Format
- 5. Transactions
- Transaction Components
- Version
- Exercise 1
- Inputs
- Parsing Script
- Exercise 2
- Outputs
- Exercise 3
- Locktime
- Exercise 4
- Exercise 5
- Coding Transactions
- Transaction Fee
- Calculating the Fee
- Exercise 6
- Conclusion
- 6. Script
- Mechanics of Script
- How Script Works
- Example Operations
- Coding Opcodes
- Exercise 1
- Parsing the Script Fields
- Coding a Script Parser and Serializer
- Combining the Script Fields
- Coding the Combined Instruction Set
- Standard Scripts
- p2pk
- Coding Script Evaluation
- Stack Elements Under the Hood
- Exercise 2
- Problems with p2pk
- Solving the Problems with p2pkh
- p2pkh
- Scripts Can Be Arbitrarily Constructed
- Exercise 3
- Utility of Scripts
- Exercise 4
- SHA-1 Piata
- Conclusion
- 7. Transaction Creation and Validation
- Validating Transactions
- Checking the Spentness of Inputs
- Checking the Sum of the Inputs Versus the Sum of the Outputs
- Checking the Signature
- Step 1: Empty all the ScriptSigs
- Step 2: Replace the ScriptSig of the input being signed with the previous ScriptPubKey
- Step 3: Append the hash type
- Exercise 1
- Exercise 2
- Verifying the Entire Transaction
- Creating Transactions
- Constructing the Transaction
- Making the Transaction
- Signing the Transaction
- Exercise 3
- Creating Your Own Transactions on testnet
- Exercise 4
- Exercise 5
- Conclusion
- Validating Transactions
- 8. Pay-to-Script Hash
- Bare Multisig
- Coding OP_CHECKMULTISIG
- Exercise 1
- Problems with Bare Multisig
- Pay-to-Script-Hash (p2sh)
- Coding p2sh
- More Complicated Scripts
- Addresses
- Exercise 2
- Exercise 3
- p2sh Signature Verification
- Step 1: Empty all the ScriptSigs
- Step 2: Replace the ScriptSig of the p2sh input being signed with the RedeemScript
- Step 3: Append the hash type
- Exercise 4
- Exercise 5
- Conclusion
- 9. Blocks
- Coinbase Transactions
- Exercise 1
- ScriptSig
- BIP0034
- Exercise 2
- Block Headers
- Exercise 3
- Exercise 4
- Exercise 5
- Version
- Exercise 6
- Exercise 7
- Exercise 8
- Previous Block
- Merkle Root
- Timestamp
- Bits
- Nonce
- Proof-of-Work
- How a Miner Generates New Hashes
- The Target
- Exercise 9
- Difficulty
- Exercise 10
- Checking That the Proof-of-Work Is Sufficient
- Exercise 11
- Difficulty Adjustment
- Exercise 12
- Exercise 13
- Conclusion
- Coinbase Transactions
- 10. Networking
- Network Messages
- Exercise 1
- Exercise 2
- Exercise 3
- Parsing the Payload
- Exercise 4
- Network Handshake
- Connecting to the Network
- Exercise 5
- Getting Block Headers
- Exercise 6
- Headers Response
- Conclusion
- Network Messages
- 11. Simplified Payment Verification
- Motivation
- Merkle Tree
- Merkle Parent
- Exercise 1
- Merkle Parent Level
- Exercise 2
- Merkle Root
- Exercise 3
- Merkle Root in Blocks
- Exercise 4
- Using a Merkle Tree
- Merkle Block
- Merkle Tree Structure
- Exercise 5
- Coding a Merkle Tree
- The merkleblock Command
- Exercise 6
- Using Flag Bits and Hashes
- Exercise 7
- Conclusion
- 12. Bloom Filters
- What Is a Bloom Filter?
- Exercise 1
- Going a Step Further
- BIP0037 Bloom Filters
- Exercise 2
- Exercise 3
- Loading a Bloom Filter
- Exercise 4
- Getting Merkle Blocks
- Exercise 5
- Getting Transactions of Interest
- Exercise 6
- Conclusion
- What Is a Bloom Filter?
- 13. Segwit
- Pay-to-Witness-Pubkey-Hash (p2wpkh)
- Transaction Malleability
- Fixing Malleability
- p2wpkh Transactions
- p2sh-p2wpkh
- Coding p2wpkh and p2sh-p2wpkh
- Pay-to-Witness-Script-Hash (p2wsh)
- p2sh-p2wsh
- Coding p2wsh and p2sh-p2wsh
- Other Improvements
- Conclusion
- Pay-to-Witness-Pubkey-Hash (p2wpkh)
- 14. Advanced Topics and Next Steps
- Suggested Topics to Study Next
- Wallets
- Hierarchical Deterministic Wallets
- Mnemonic Seeds
- Payment Channels and Lightning Network
- Wallets
- Contributing
- Suggested Next Projects
- Testnet Wallet
- Block Explorer
- Web Shop
- Utility Library
- Finding a Job
- Conclusion
- Suggested Topics to Study Next
- A. Solutions
- Chapter 1: Finite Fields
- Exercise 1
- Exercise 2
- Exercise 3
- Exercise 4
- Exercise 5
- Exercise 6
- Exercise 7
- Exercise 8
- Exercise 9
- Chapter 2: Elliptic Curves
- Exercise 1
- Exercise 2
- Exercise 3
- Exercise 4
- Exercise 5
- Exercise 6
- Exercise 7
- Chapter 3: Elliptic Curve Cryptography
- Exercise 1
- Exercise 2
- Exercise 3
- Exercise 4
- Exercise 5
- Exercise 6
- Exercise 7
- Chapter 4: Serialization
- Exercise 1
- Exercise 2
- Exercise 3
- Exercise 4
- Exercise 5
- Exercise 6
- Exercise 7
- Exercise 8
- Exercise 9
- Chapter 5: Transactions
- Exercise 1
- Exercise 2
- Exercise 3
- Exercise 4
- Exercise 5
- Exercise 6
- Chapter 6: Script
- Exercise 1
- Exercise 2
- Exercise 3
- Exercise 4
- Chapter 7: Transaction Creation and Validation
- Exercise 1
- Exercise 2
- Exercise 3
- Exercise 4
- Exercise 5
- Chapter 8: Pay to Script Hash
- Exercise 1
- Exercise 2
- Exercise 3
- Exercise 4
- Exercise 5
- Chapter 9: Blocks
- Exercise 1
- Exercise 2
- Exercise 3
- Exercise 4
- Exercise 5
- Exercise 6
- Exercise 7
- Exercise 8
- Exercise 9
- Exercise 10
- Exercise 11
- Exercise 12
- Exercise 13
- Chapter 10: Networking
- Exercise 1
- Exercise 2
- Exercise 3
- Exercise 4
- Exercise 5
- Exercise 6
- Chapter 11: Simplified Payment Verification
- Exercise 1
- Exercise 2
- Exercise 3
- Exercise 4
- Exercise 5
- Exercise 6
- Exercise 7
- Chapter 12: Bloom Filters
- Exercise 1
- Exercise 2
- Exercise 3
- Exercise 4
- Exercise 5
- Exercise 6
- Chapter 1: Finite Fields
- Index