Learning React Native. Building Native Mobile Apps with JavaScript. 2nd Edition - Helion
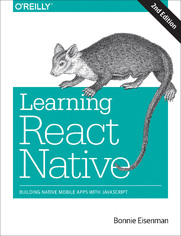
ISBN: 978-14-919-8909-8
stron: 242, Format: ebook
Data wydania: 2017-10-23
Księgarnia: Helion
Cena książki: 186,15 zł (poprzednio: 216,45 zł)
Oszczędzasz: 14% (-30,30 zł)
Get a practical introduction to React Native, the JavaScript framework for writing and deploying fully featured mobile apps that render natively. The second edition of this hands-on guide shows you how to build applications that target iOS, Android, and other mobile platforms instead of browsers—apps that can access platform features such as the camera, user location, and local storage.
Through code examples and step-by-step instructions, web developers and frontend engineers familiar with React will learn how to build and style interfaces, use mobile components, and debug and deploy apps. You’ll learn how to extend React Native using third-party libraries or your own Java and Objective-C libraries.
- Understand how React Native works under the hood with native UI components
- Examine how React Native’s mobile-based components compare to basic HTML elements
- Create and style your own React Native components and applications
- Take advantage of platform-specific APIs, as well as modules from the framework’s community
- Incorporate platform-specific components into cross-platform apps
- Learn common pitfalls of React Native development, and tools for dealing with them
- Combine a large application’s many screens into a cohesive UX
- Handle state management in a large app with the Redux library
Osoby które kupowały "Learning React Native. Building Native Mobile Apps with JavaScript. 2nd Edition", wybierały także:
- Windows Media Center. Domowe centrum rozrywki 66,67 zł, (8,00 zł -88%)
- Ruby on Rails. Ćwiczenia 18,75 zł, (3,00 zł -84%)
- Przywództwo w świecie VUCA. Jak być skutecznym liderem w niepewnym środowisku 58,64 zł, (12,90 zł -78%)
- Scrum. O zwinnym zarządzaniu projektami. Wydanie II rozszerzone 58,64 zł, (12,90 zł -78%)
- Od hierarchii do turkusu, czyli jak zarządzać w XXI wieku 58,64 zł, (12,90 zł -78%)
Spis treści
Learning React Native. Building Native Mobile Apps with JavaScript. 2nd Edition eBook -- spis treści
- Preface
- Prerequisites
- Conventions Used in This Book
- Using Code Examples
- OReilly Safari
- How to Contact Us
- Resources
- Acknowledgments
- 1. What Is React Native?
- Advantages of React Native
- Developer Experience
- Code Reuse and Knowledge Sharing
- Risks and Drawbacks
- Summary
- Advantages of React Native
- 2. Working with React Native
- How Does React Native Work?
- Rendering Lifecycle
- Creating Components in React Native
- Working with Views
- Using JSX
- Styling Native Components
- Host Platform APIs
- Summary
- 3. Building Your First Application
- Setting Up Your Environment
- Developer Setup: Create React Native App
- Creating Your First Application with create-react-native-app
- Previewing Your App on iOS or Android
- Developer Setup: The Traditional Approach
- Creating Your First Application with react-native
- Running Your App on iOS
- Running Your App on Android
- Exploring the Sample Code
- Building a Weather App
- Handling User Input
- Displaying Data
- Fetching Data from the Web
- Adding a Background Image
- Putting It All Together
- Summary
- 4. Components for Mobile
- Analogies Between HTML Elements and Native Components
- The <Text> Component
- The <Image> Component
- Working with Touch and Gestures
- Creating Basic Interactions with <Button>
- Using the <TouchableHighlight> Component
- Using the PanResponder Class
- Choosing how to handle touch
- Working with Lists
- Using the Basic <FlatList> Component
- Updating the <FlatList> Contents
- Integrating Real Data
- Working with <SectionList>
- Navigation
- Other Organizational Components
- Summary
- Analogies Between HTML Elements and Native Components
- 5. Styles
- Declaring and Manipulating Styles
- Using Inline Styles
- Styling with Objects
- Using StyleSheet.create
- Concatenating Styles
- Organization and Inheritance
- Exporting Style Objects
- Passing Styles as Props
- Reusing and Sharing Styles
- Positioning and Designing Layouts
- Using Layouts with Flexbox
- Using Absolute Positioning
- Putting It Together
- Summary
- Declaring and Manipulating Styles
- 6. Platform APIs
- Using Geolocation
- Reading the Users Location
- Handling Permissions
- Testing Geolocation in Emulated Devices
- Watching the Users Location
- Working Around Limitations
- Updating the Weather Application
- Accessing the Users Images and Camera
- Interacting with the CameraRoll Module
- Requesting Images with GetPhotoParams
- Rendering an Image from the Camera Roll
- Uploading an Image to a Server
- Storing Persistent Data with AsyncStorage
- The SmarterWeather Application
- The <WeatherProject> Component
- The <Forecast> Component
- The <Button> Component
- The <LocationButton> Component
- The <PhotoBackdrop> Component
- Summary
- Using Geolocation
- 7. Modules and Native Code
- Installing JavaScript Libraries with npm
- Installing Third-Party Components with Native Code
- Using the Video Component
- Objective-C Native Modules
- Writing an Objective-C Native Module for iOS
- Exploring react-native-video for iOS
- Java Native Modules
- Writing a Java Native Module for Android
- Exploring react-native-video for Java
- Cross-Platform Native Modules
- Summary
- 8. Platform-Specific Code
- iOS- or Android-Only Components
- Components with Platform-Specific Implementations
- Using Platform-Specific File Extensions
- Using the Platform Module
- When to Use Platform-Specific Components
- 9. Debugging and Developer Tools
- JavaScript Debugging Practices, Translated
- Activating the Developer Options
- Debugging with console.log
- Using the JavaScript Debugger
- Working with the React Developer Tools
- React Native Debugging Tools
- Using Inspect Element
- Interpreting the Red Screen of Death
- Debugging Beyond JavaScript
- Common Development Environment Issues
- Common Xcode Problems
- Common Android Problems
- The React Native Packager
- Issues Deploying to an iOS Device
- Simulator Behavior
- Testing Your Code
- Type Checking with Flow
- Unit Testing with Jest
- Snapshot Testing with Jest
- When Youre Stuck
- Summary
- JavaScript Debugging Practices, Translated
- 10. Navigation and Structure in Larger Applications
- The Flashcard Application
- Project Structure
- Application Screens
- Reusable Components
- Styles
- Data Models
- Using React-Navigation
- Creating a StackNavigator
- Using navigation.navigate to Transition Between Screens
- Configuring the Header with navigationOptions
- Implementing the Rest
- Summary
- 11. State Management in Larger Applications
- Using Redux to Manage State
- Actions
- Reducers
- Connecting Redux
- Persisting Data with AsyncStorage
- Summary and Homework
- Conclusion
- A. Modern JavaScript Syntax
- let and const
- Importing Modules
- Destructuring
- Function Shorthand
- Fat-Arrow Functions
- Default Parameters
- String Interpolation
- Working with Promises
- B. Deploying Your Application
- Check Your Application Assets and Specify Target OS Versions and Devices
- Create a Release Build
- Complete Your Paperwork
- Beta Test Your Application
- Create a Listing
- Wait for Review
- Release
- C. Working with Expo Applications
- Ejecting from Expo
- Index