iPhone 3D Programming. Developing Graphical Applications with OpenGL ES - Helion
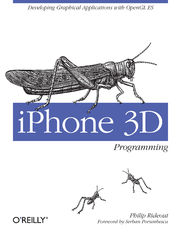
ISBN: 978-14-493-9062-4
stron: 440, Format: ebook
Data wydania: 2010-05-03
Księgarnia: Helion
Cena książki: 118,15 zł (poprzednio: 137,38 zł)
Oszczędzasz: 14% (-19,23 zł)
What does it take to build an iPhone app with stunning 3D graphics? This book will show you how to apply OpenGL graphics programming techniques to any device running the iPhone OS -- including the iPad and iPod Touch -- with no iPhone development or 3D graphics experience required. iPhone 3D Programming provides clear step-by-step instructions, as well as lots of practical advice, for using the iPhone SDK and OpenGL.
You'll build several graphics programs -- progressing from simple to more complex examples -- that focus on lighting, textures, blending, augmented reality, optimization for performance and speed, and much more. All you need to get started is a solid understanding of C++ and a great idea for an app.
- Learn fundamental graphics concepts, including transformation matrices, quaternions, and more
- Get set up for iPhone development with the Xcode environment
- Become familiar with versions 1.1 and 2.0 of the OpenGL ES API, and learn to use vertex buffer objects, lighting, texturing, and shaders
- Use the iPhone's touch screen, compass, and accelerometer to build interactivity into graphics applications
- Build iPhone graphics applications such as a 3D wireframe viewer, a simple augmented reality application, a spring system simulation, and more
Osoby które kupowały "iPhone 3D Programming. Developing Graphical Applications with OpenGL ES", wybierały także:
- Zen Steve'a Jobsa 29,67 zł, (8,90 zł -70%)
- Programowanie aplikacji mobilnych dla iOS z wykorzystaniem Xcode, Swift 3.0 i iOS 10 SDK. Kurs video. Poziom pierwszy 99,00 zł, (44,55 zł -55%)
- Podstawy języka Swift. Programowanie aplikacji dla platformy iOS 49,00 zł, (26,95 zł -45%)
- Dotknij, przesuń, potrząśnij. Od pomysłu do gry na iPhone'a i iPada 39,00 zł, (21,45 zł -45%)
- Flutter i Dart 2 dla początkujących. Przewodnik dla twórców aplikacji mobilnych 87,41 zł, (48,95 zł -44%)
Spis treści
iPhone 3D Programming. Developing Graphical Applications with OpenGL ES eBook -- spis treści
- iPhone 3D Programming
- SPECIAL OFFER: Upgrade this ebook with OReilly
- A Note Regarding Supplemental Files
- Foreword
- Preface
- How to Read This Book
- Conventions Used in This Book
- Using Code Examples
- Safari Books Online
- How to Contact Us
- Acknowledgments
- 1. Quick-Start Guide
- Transitioning to Apple Technology
- Objective-C
- A Brief History of OpenGL ES
- Choosing the Appropriate Version of OpenGL ES
- Getting Started
- Installing the iPhone SDK
- Building the OpenGL Template Application with Xcode
- Deploying to Your Real iPhone
- HelloArrow with Fixed Function
- Layering Your 3D Application
- Starting from Scratch
- Linking in the OpenGL and Quartz Libraries
- Subclassing UIView
- Hooking Up the Application Delegate
- Setting Up the Icons and Launch Image
- Dealing with the Status Bar
- Defining and Consuming the Rendering Engine Interface
- Implementing the Rendering Engine
- Handling Device Orientation
- Animating the Rotation
- HelloArrow with Shaders
- Shaders
- Frameworks
- GLView
- RenderingEngine Implementation
- Wrapping Up
- Transitioning to Apple Technology
- 2. Math and Metaphors
- The Assembly Line Metaphor
- Assembling Primitives from Vertices
- Associating Properties with Vertices
- The Life of a Vertex
- The Photography Metaphor
- Setting the Model Matrix
- Scale
- Translation
- Rotation
- Setting the View Transform
- Setting the Projection Transform
- Setting the Model Matrix
- Saving and Restoring Transforms with Matrix Stacks
- Animation
- Interpolation Techniques
- Animating Rotation with Quaternions
- Vector Beautification with C++
- HelloCone with Fixed Function
- RenderingEngine Declaration
- OpenGL Initialization and Cone Tessellation
- Smooth Rotation in Three Dimensions
- Render Method
- HelloCone with Shaders
- Wrapping Up
- 3. Vertices and Touch Points
- Reading the Touchscreen
- Saving Memory with Vertex Indexing
- Boosting Performance with Vertex Buffer Objects
- Creating a Wireframe Viewer
- Parametric Surfaces for Fun
- Designing the Interfaces
- Handling Trackball Rotation
- Implementing the Rendering Engine
- Poor Mans Tab Bar
- Animating the Transition
- Wrapping Up
- 4. Adding Depth and Realism
- Examining the Depth Buffer
- Beware the Scourge of Depth Artifacts
- Creating and Using the Depth Buffer
- Filling the Wireframe with Triangles
- Surface Normals
- Feeding OpenGL with Normals
- The Math Behind Normals
- Normal Transforms Arent Normal
- Generating Normals from Parametric Surfaces
- Lighting Up
- Ho-Hum Ambiance
- Matte Paint with Diffuse Lighting
- Give It a Shine with Specular
- Adding Light to ModelViewer
- Using Light Properties
- Shaders Demystified
- Adding Shaders to ModelViewer
- New Rendering Engine
- Per-Pixel Lighting
- Toon Shading
- Better Wireframes Using Polygon Offset
- Loading Geometry from OBJ Files
- Managing Resource Files
- Implementing ISurface
- Wrapping Up
- Examining the Depth Buffer
- 5. Textures and Image Capture
- Adding Textures to ModelViewer
- Enhancing IResourceManager
- Generating Texture Coordinates
- Enabling Textures with ES1::RenderingEngine
- Enabling Textures with ES2::RenderingEngine
- Texture Coordinates Revisited
- Fight Aliasing with Filtering
- Boosting Quality and Performance with Mipmaps
- Modifying ModelViewer to Support Mipmaps
- Texture Formats and Types
- Hands-On: Loading Various Formats
- Texture Compression with PVRTC
- The PowerVR SDK and Low-Precision Textures
- Generating and Transforming OpenGL Textures with Quartz
- Dealing with Size Constraints
- Scaling to POT
- Creating Textures with the Camera
- CameraTexture: Rendering Engine Implementation
- Wrapping Up
- Adding Textures to ModelViewer
- 6. Blending and Augmented Reality
- Blending Recipe
- Wrangle Premultiplied Alpha
- Blending Caveats
- Blending Extensions and Their Uses
- Why Is Blending Configuration Useful?
- Shifting Texture Color with Per-Vertex Color
- Poor Mans Reflection with the Stencil Buffer
- Rendering the Disk to Stencil Only
- Rendering the Reflected Object with Stencil Testing
- Rendering the Real Object
- Rendering the Disk with Front-to-Back Blending
- Stencil Alternatives for Older iPhones
- Anti-Aliasing Tricks with Offscreen FBOs
- A Super Simple Sample App for Supersampling
- Jittering
- Other FBO Effects
- Rendering Anti-Aliased Lines with Textures
- Holodeck Sample
- Application Skeleton
- Rendering the Dome, Clouds, and Text
- Handling the Heads-Up Display
- Replacing Buttons with Orientation Sensors
- Adding accelerometer support
- Adding compass support
- Overlaying with a Live Camera Image
- Wrapping Up
- 7. Sprites and Text
- Text Rendering 101: Drawing an FPS Counter
- Generating a Glyphs Texture with Python
- Rendering the FPS Text
- Stabilizing the counter with a low-pass filter
- Fleshing out the FpsRenderer class
- Simplify with glDrawTexOES
- Crisper Text with Distance Fields
- Generating Distance Fields with Python
- Use Distance Fields Under ES 1.1 with Alpha Testing
- Adding Text Effects with Fragment Shaders
- Smoothing and Derivatives
- Implementing Outline, Glow, and Shadow Effects
- Animation with Sprite Sheets
- Image Composition and a Taste of Multitexturing
- Mixing OpenGL ES and UIKit
- Rendering Confetti, Fireworks, and More: Point Sprites
- Chapter Finale: SpringyStars
- Physics Diversion: Mass-Spring System
- C++ Interfaces and GLView
- ApplicationEngine Implementation
- OpenGL ES 1.1 Rendering Engine and Additive Blending
- Point Sprites with OpenGL ES 2.0
- Wrapping Up
- Text Rendering 101: Drawing an FPS Counter
- 8. Advanced Lighting and Texturing
- Texture Environments under OpenGL ES 1.1
- Texture Combiners
- Bump Mapping and DOT3 Lighting
- Another Foray into Linear Algebra
- Generating Basis Vectors
- Normal Mapping with OpenGL ES 2.0
- Normal Mapping with OpenGL ES 1.1
- Generating Object-Space Normal Maps
- Reflections with Cube Maps
- Render to Cube Map
- Anisotropic Filtering: Textures on Steroids
- Image-Processing Example: Bloom
- Better Performance with a Hybrid Approach
- Sample Code for Gaussian Bloom
- Wrapping Up
- Texture Environments under OpenGL ES 1.1
- 9. Optimizing
- Instruments
- Understand the CPU/GPU Split
- Vertex Submission: Above and Beyond VBOs
- Batch, Batch, Batch
- Interleaved Vertex Attributes
- Optimize Your Vertex Format
- Use the Best Topology and Indexing
- Lighting Optimizations
- Object-Space Lighting
- DOT3 Lighting Revisited
- Baked Lighting
- Texturing Optimizations
- Culling and Clipping
- Polygon Winding
- User Clip Planes
- CPU-Based Clipping
- Shader Performance
- Conditionals
- Fragment Killing
- Texture Lookups Can Hurt!
- Optimizing Animation with Vertex Skinning
- Skinning: Common Code
- Skinning with OpenGL ES 2.0
- Skinning with OpenGL ES 1.1
- Generating Weights and Indices
- Watch Out for Pinching
- Further Reading
- A. C++ Vector Library
- Disclaimer Regarding Performance
- Vector.hpp
- Matrix.hpp
- Quaternion.hpp
- Index
- About the Author
- Colophon
- SPECIAL OFFER: Upgrade this ebook with OReilly