iOS Swift Game Development Cookbook. Simple Solutions for Game Development Problems. 3rd Edition - Helion
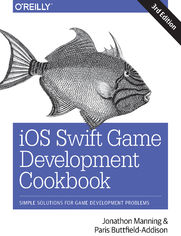
ISBN: 978-14-919-9903-5
stron: 350, Format: ebook
Data wydania: 2018-10-02
Księgarnia: Helion
Cena książki: 177,65 zł (poprzednio: 206,57 zł)
Oszczędzasz: 14% (-28,92 zł)
Ready to make amazing games for the iPhone and iPad? With Apple’s Swift programming language, it’s never been easier. This updated cookbook provides detailed recipes for managing a wide range of common iOS game-development issues, ranging from 2D and 3D math, SpriteKit, and OpenGL to augmented reality with ARKit.
You get simple, direct solutions to common problems found in iOS game programming. Need to figure out how to give objects physical motion, or want a refresher on gaming-related math problems? This book provides sample projects and straightforward answers. All you need to get started is some familiarity with iOS development in Swift.
Osoby które kupowały "iOS Swift Game Development Cookbook. Simple Solutions for Game Development Problems. 3rd Edition", wybierały także:
- Zen Steve'a Jobsa 29,67 zł, (8,90 zł -70%)
- Programowanie aplikacji mobilnych dla iOS z wykorzystaniem Xcode, Swift 3.0 i iOS 10 SDK. Kurs video. Poziom pierwszy 99,00 zł, (44,55 zł -55%)
- Flutter i Dart 2 dla początkujących. Przewodnik dla twórców aplikacji mobilnych 89,00 zł, (53,40 zł -40%)
- Dostosuj się lub giń. Jak odnieść sukces w branży aplikacji mobilnych 44,90 zł, (26,94 zł -40%)
- Podstawy języka Swift. Programowanie aplikacji dla platformy iOS 49,00 zł, (29,40 zł -40%)
Spis treści
iOS Swift Game Development Cookbook. Simple Solutions for Game Development Problems. 3rd Edition eBook -- spis treści
- Preface
- Audience
- Organization of This Book
- Additional Resources
- Conventions Used in This Book
- Using Code Examples
- OReilly Safari
- How to Contact Us
- Acknowledgments
- 1. Laying Out a Game
- 1.1. Laying Out Your Engine
- 1.2. Creating an Inheritance-Based Game Layout
- 1.3. Creating a Component-Based Game Layout
- 1.4. Creating a Component-Based Game Layout Using GameplayKit
- 1.5. Calculating Delta Times
- 1.6. Detecting When the User Enters and Exits Your Game
- 1.7. Updating Based on a Timer
- 1.8. Updating Based on When the Screen Updates
- 1.9. Pausing a Game
- 1.10. Calculating Time Elapsed Since the Game Start
- 1.11. Working with Closures
- 1.12. Writing a Method That Calls a Closure
- 1.13. Working with Operation Queues
- 1.14. Performing a Task in the Future
- 1.15. Making Operations Depend on Each Other
- 1.16. Filtering an Array with Closures
- 1.17. Loading New Assets During Gameplay
- 1.18. Adding Unit Tests to Your Game
- 1.19. 2D Grids
- 1.20. Using Randomization
- 1.21. Building a State Machine
- 2. Views and Menus
- 2.1. Working with Storyboards
- 2.2. Creating View Controllers
- 2.3. Using Segues to Move Between Screens
- 2.4. Using Constraints to Lay Out Views
- 2.5. Adding Images to Your Project
- 2.6. Slicing Images for Use in Buttons
- 2.7. Using UI Dynamics to Make Animated Views
- 2.8. Moving an Image with Core Animation
- 2.9. Rotating an Image View
- 2.10. Animating a Popping Effect on a View
- 2.11. Theming UI Elements with UIAppearance
- 2.12. Rotating a UIView in 3D
- 2.13. Overlaying Menus on Top of Game Content
- 2.14. Designing Effective Game Menus
- 3. Input
- 3.1. Detecting When a View Is Touched
- 3.2. Responding to Tap Gestures
- 3.3. Dragging an Image Around the Screen
- 3.4. Detecting Rotation Gestures
- 3.5. Detecting Pinching Gestures
- 3.6. Creating Custom Gestures
- 3.7. Receiving Touches in Custom Areas of a View
- 3.8. Detecting Shakes
- 3.9. Detecting Device Tilt
- 3.10. Getting the Compass Heading
- 3.11. Accessing the Users Location
- 3.12. Calculating the Users Speed
- 3.13. Pinpointing the Users Proximity to Landmarks
- 3.14. Receiving Notifications When the User Changes Location
- 3.15. Looking Up GPS Coordinates for a Street Address
- 3.16. Looking Up Street Addresses from the Users Location
- 3.17. Using the Device as a Steering Wheel
- 3.18. Detecting Magnets
- 3.19. Utilizing Inputs to Improve Game Design
- 4. Sound
- 4.1. Playing Sound with AVAudioPlayer
- 4.2. Recording Sound with AVAudioRecorder
- 4.3. Working with Multiple Audio Players
- 4.4. Cross-Fading Between Tracks
- 4.5. Synthesizing Speech
- 4.6. Getting Information About What the Music App Is Playing
- 4.7. Detecting When the Currently Playing Track Changes
- 4.8. Controlling Music Playback
- 4.9. Allowing the User to Select Music
- 4.10. Cooperating with Other Applications Audio
- 4.11. Determining How to Best Use Sound in Your Game Design
- 5. Data Storage
- 5.1. Storing Structured Information
- 5.2. Storing Data Locally
- 5.3. Using iCloud to Save Games
- 5.4. Using the iCloud Key-Value Store
- 5.5. Deciding When to Use Files or a Database
- 5.6. Managing a Collection of Assets
- 5.7. Storing Information in UserDefaults
- 5.8. Implementing the Best Data Storage Strategy
- 5.9. In-Game Currency
- 5.10. Setting Up CloudKit
- 5.11. Adding Records to a CloudKit Database
- 5.12. Querying Records to a CloudKit Database
- 5.13. Deleting Records from a CloudKit Database
- 6. 2D Graphics and SpriteKit
- 6.1. Getting Familiar with 2D Math
- 6.2. Creating a SpriteKit View
- 6.3. Creating a Scene
- 6.4. Adding a Sprite
- 6.5. Adding a Text Sprite
- 6.6. Determining Available Fonts
- 6.7. Including Custom Fonts
- 6.8. Transitioning Between Scenes
- 6.9. Moving Sprites and Labels Around
- 6.10. Adding a Texture Sprite
- 6.11. Creating Texture Atlases
- 6.12. Using Shape Nodes
- 6.13. Using Blending Modes
- 6.14. Using Image Effects to Change the Way That Sprites Are Drawn
- 6.15. Using Bézier Paths
- 6.16. Creating Smoke, Fire, and Other Particle Effects
- 6.17. Shaking the Screen
- 6.18. Animating a Sprite
- 6.19. Parallax Scrolling
- 6.20. Creating Images Using Noise
- 7. Physics
- 7.1. Reviewing Physics Terms and Definitions
- 7.2. Adding Physics to Sprites
- 7.3. Creating Static and Dynamic Objects
- 7.4. Defining Collider Shapes
- 7.5. Setting Velocities
- 7.6. Working with Mass, Size, and Density
- 7.7. Creating Walls in Your Scene
- 7.8. Controlling Gravity
- 7.9. Keeping Objects from Falling Over
- 7.10. Controlling Time in Your Physics Simulation
- 7.11. Detecting Collisions
- 7.12. Finding Objects
- 7.13. Working with Joints
- 7.14. Working with Forces
- 7.15. Adding Thrusters to Objects
- 7.16. Creating Explosions
- 7.17. Using Device Orientation to Control Gravity
- 7.18. Dragging Objects Around
- 7.19. Creating a Car
- 8. SceneKit
- 8.1. Setting Up for SceneKit
- 8.2. Creating a SceneKit Scene
- 8.3. Showing a 3D Object
- 8.4. Working with SceneKit Cameras
- 8.5. Creating Lights
- 8.6. Animating Objects
- 8.7. Working with Text Nodes
- 8.8. Customizing Materials
- 8.9. Texturing Objects
- 8.10. Normal Mapping
- 8.11. Constraining Objects
- 8.12. Loading COLLADA Files
- 8.13. Using 3D Physics
- 8.14. Adding Reflections
- 8.15. Hit-Testing the Scene
- 8.16. Loading a Scene File
- 8.17. Particle Systems
- 8.18. Using Metal
- 9. Artificial Intelligence and Behavior
- 9.1. Making Vector Math Nicer in Swift
- 9.2. Making an Object Move Toward a Position
- 9.3. Making Things Follow a Path
- 9.4. Making an Object Intercept a Moving Target
- 9.5. Making an Object Flee When Its in Trouble
- 9.6. Making an Object Decide on a Target
- 9.7. Making an Object Steer Toward a Point
- 9.8. Making an Object Know Where to Take Cover
- 9.9. Calculating a Path for an Object to Take
- 9.10. Pathfinding on a Grid
- 9.11. Finding the Next Best Move for a Puzzle Game
- 9.12. Determining If an Object Can See Another Object
- 9.13. Tagging Parts of Speech with NSLinguisticTagger
- 9.14. Using AVFoundation to Access the Camera
- 9.15. Importing a Core ML Model
- 9.16. Identifying Objects in Images
- 9.17. Using AI to Enhance Your Game Design
- 10. Working with the Outside World
- 10.1. Detecting Controllers
- 10.2. Getting Input from a Game Controller
- 10.3. Showing Content via AirPlay
- 10.4. Using External Screens
- 10.5. Designing Effective Graphics for Different Screens
- 10.6. Dragging and Dropping
- 10.7. Providing Haptic Feedback with UIFeedbackGenerator
- 10.8. Recording the Screen with ReplayKit
- 10.9. Displaying Augmented Reality with ARKit
- 10.10. Hit-Testing the AR Scene
- 10.11. Using TestFlight to Test Your App
- 10.12. Using Fastlane to Build and Release Your App
- 11. Performance and Debugging
- 11.1. Improving Your Frame Rate
- 11.2. Making Levels Load Quickly
- 11.3. Dealing with Low-Memory Issues
- 11.4. Tracking Down a Crash
- 11.5. Working with Compressed Textures
- 11.6. Working with Watchpoints
- 11.7. Logging Effectively
- 11.8. Creating Breakpoints That Use Speech
- Index