WebSocket. Lightweight Client-Server Communications - Helion
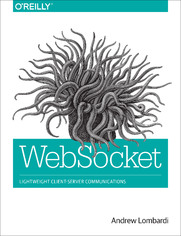
ISBN: 978-14-493-6923-1
stron: 144, Format: ebook
Data wydania: 2015-09-09
Księgarnia: Helion
Cena książki: 80,73 zł (poprzednio: 94,98 zł)
Oszczędzasz: 15% (-14,25 zł)
Until recently, creating desktop-like applications in the browser meant using inefficient Ajax or Comet technologies to communicate with the server. With this practical guide, you’ll learn how to use WebSocket, a protocol that enables the client and server to communicate with each other on a single connection simultaneously. No more asynchronous communication or long polling!
For developers with a good grasp of JavaScript (and perhaps Node.js), author Andrew Lombardi provides useful hands-on examples throughout the book to help you get up to speed with the WebSocket API. You’ll also learn how to use WebSocket with Transport Layer Security (TLS).
- Learn how to use WebSocket API events, messages, attributes, and methods within your client application
- Build bi-directional chat applications on the client and server with WebSocket as the communication layer
- Create a subprotocol over WebSocket for STOMP 1.0, the Simple Text Oriented Messaging Protocol
- Use options for older browsers that don’t natively support WebSocket
- Protect your WebSocket application against various attack vectors with TLS and other tools
- Debug applications by learning aspects of the WebSocket lifecycle
Osoby które kupowały "WebSocket. Lightweight Client-Server Communications", wybierały także:
- D3.js w akcji 67,42 zł, (20,90 zł -69%)
- Tablice informatyczne. Node.js 16,86 zł, (5,90 zł -65%)
- React i GatsbyJS. Kurs video. Nowoczesne strony internetowe w oparciu o headless CMS i GraphQL 148,98 zł, (59,59 zł -60%)
- TypeScript i React. Kurs video. Tworzenie nowoczesnych komponent 129,00 zł, (51,60 zł -60%)
- Vue.js w praktyce. Kurs video. Poznaj narz 148,98 zł, (59,59 zł -60%)
Spis treści
WebSocket. Lightweight Client-Server Communications eBook -- spis treści
- Preface
- Who Should Read This Book
- Goals of This Book
- Navigating This Book
- Conventions Used in This Book
- Using Code Examples
- Safari Books Online
- How to Contact Us
- Acknowledgments
- 1. Quick Start
- Getting Node and npm
- Installing on Windows
- Installing on OS X
- Installing on Linux
- Hello, World! Example
- Why WebSocket?
- Summary
- Getting Node and npm
- 2. WebSocket API
- Initializing
- Stock Example UI
- WebSocket Events
- Event: Open
- Event: Message
- Event: Error
- Event: PING/PONG
- Event: Close
- WebSocket Methods
- Method: Send
- Method: Close
- WebSocket Attributes
- Attribute: readyState
- Attribute: bufferedAmount
- Attribute: protocol
- Stock Example Server
- Testing for WebSocket Support
- Summary
- 3. Bidirectional Chat
- Long Polling
- Writing a Basic Chat Application
- WebSocket Client
- Client Identity
- Events and Notifications
- The Server
- The Client
- Summary
- 4. STOMP over WebSocket
- Implementing STOMP
- Getting Connected
- Connecting via the Server
- Setting Up RabbitMQ
- Connecting the Server to RabbitMQ
- The Stock Price Daemon
- Processing STOMP Requests
- Client
- Using RabbitMQ with Web-Stomp
- STOMP Client for Web and Node.js
- Installing the Web-Stomp Plug-in
- Echo Client for Web-Stomp
- Summary
- Implementing STOMP
- 5. WebSocket Compatibility
- SockJS
- SockJS Chat Server
- SockJS Chat Client
- Socket.IO
- Adobe Flash Socket
- Connecting
- Socket.IO Chat Server
- Socket.IO Chat Client
- Pusher.com
- Channels
- Events
- Pusher Chat Server
- Pusher Chat Client
- Dont Forget: Pusher Is a Commercial Solution
- Reverse Proxy
- Summary
- SockJS
- 6. WebSocket Security
- TLS and WebSocket
- Generating a Self-Signed Certificate
- Installing on Windows
- Installing on OS X
- Installing on Linux
- Setting up WebSocket over TLS
- WebSocket Server over TLS Example
- Origin-Based Security Model
- Clickjacking
- X-Frame-Options for Framebusting
- Denial of Service
- Frame Masking
- Validating Clients
- Setting Up Dependencies and Inits
- Listening for Web Requests
- WebSocket Server
- Summary
- TLS and WebSocket
- 7. Debugging and Tools
- The Handshake
- The Server
- The Client
- Download and Configure ZAP
- WebSocket Secure to the Rescue
- Validating the Handshake
- Inspecting Frames
- Masked Payloads
- Closing Connection
- Summary
- The Handshake
- 8. WebSocket Protocol
- HTTP 0.9The Web Is Born
- HTTP 1.0 and 1.1
- WebSocket Open Handshake
- Sec-WebSocket-Key and Sec-WebSocket-Accept
- Generating the Sec-WebSocket-Key
- Responding with the Sec-WebSocket-Accept
- WebSocket HTTP Headers
- Sec-WebSocket-Key and Sec-WebSocket-Accept
- WebSocket Frame
- Fin Bit
- Frame Opcodes
- Masking
- Length
- Fragmentation
- WebSocket Close Handshake
- WebSocket Subprotocols
- WebSocket Extensions
- Alternate Server Implementations
- Summary
- Index