Think Julia. How to Think Like a Computer Scientist - Helion
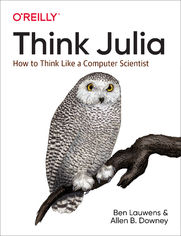
ISBN: 978-14-920-4498-7
stron: 296, Format: ebook
Data wydania: 2019-04-05
Księgarnia: Helion
Cena książki: 152,15 zł (poprzednio: 176,92 zł)
Oszczędzasz: 14% (-24,77 zł)
If you’re just learning how to program, Julia is an excellent JIT-compiled, dynamically-typed language with a clean syntax. This hands-on guide uses Julia (version 1.0) to walk you through programming one step at a time, beginning with basic programming concepts before moving on to more advanced capabilities, such as creating new types and multiple dispatch.
Designed from the beginning for high performance, Julia is a general-purpose language not only ideal for numerical analysis and computational science, but also for web programming or scripting. Through exercises in each chapter, you’ll try out programming concepts as you learn them.
Think Julia is ideal for students at the high school or college level, as well as self-learners, home-schooled students, and professionals who need to learn programming basics.
- Start with the basics, including language syntax and semantics
- Get a clear definition of each programming concept
- Learn about values, variables, statements, functions, and data structures in a logical progression
- Discover how to work with files and databases
- Understand types, methods, and multiple dispatch
- Use debugging techniques to fix syntax, runtime, and semantic errors
- Explore interface design and data structures through case studies
Osoby które kupowały "Think Julia. How to Think Like a Computer Scientist", wybierały także:
- Windows Media Center. Domowe centrum rozrywki 66,67 zł, (8,00 zł -88%)
- Ruby on Rails. Ćwiczenia 18,75 zł, (3,00 zł -84%)
- Przywództwo w świecie VUCA. Jak być skutecznym liderem w niepewnym środowisku 58,64 zł, (12,90 zł -78%)
- Scrum. O zwinnym zarządzaniu projektami. Wydanie II rozszerzone 58,64 zł, (12,90 zł -78%)
- Od hierarchii do turkusu, czyli jak zarządzać w XXI wieku 58,64 zł, (12,90 zł -78%)
Spis treści
Think Julia. How to Think Like a Computer Scientist eBook -- spis treści
- Preface
- Why Julia?
- Who Is This Book For?
- Conventions Used in This Book
- Using Code Examples
- OReilly Online Learning
- How to Contact Us
- Acknowledgments
- Contributor List
- 1. The Way of the Program
- What Is a Program?
- Running Julia
- The First Program
- Arithmetic Operators
- Values and Types
- Formal and Natural Languages
- Debugging
- Glossary
- Exercises
- Exercise 1-1
- Exercise 1-2
- 2. Variables, Expressions, and Statements
- Assignment Statements
- Variable Names
- Expressions and Statements
- Script Mode
- Exercise 2-1
- Operator Precedence
- String Operations
- Comments
- Debugging
- Glossary
- Exercises
- Exercise 2-2
- Exercise 2-3
- 3. Functions
- Function Calls
- Math Functions
- Composition
- Adding New Functions
- Definitions and Uses
- Exercise 3-1
- Flow of Execution
- Parameters and Arguments
- Variables and Parameters Are Local
- Stack Diagrams
- Fruitful Functions and Void Functions
- Why Functions?
- Debugging
- Glossary
- Exercises
- Exercise 3-2
- Exercise 3-3
- Exercise 3-4
- 4. Case Study: Interface Design
- Turtles
- Exercise 4-1
- Simple Repetition
- Exercises
- Exercise 4-2
- Exercise 4-3
- Exercise 4-4
- Exercise 4-5
- Exercise 4-6
- Exercise 4-7
- Encapsulation
- Generalization
- Interface Design
- Refactoring
- A Development Plan
- Docstring
- Debugging
- Glossary
- Exercises
- Exercise 4-8
- Exercise 4-9
- Exercise 4-10
- Exercise 4-11
- Exercise 4-12
- Turtles
- 5. Conditionals and Recursion
- Floor Division and Modulus
- Boolean Expressions
- Logical Operators
- Conditional Execution
- Alternative Execution
- Chained Conditionals
- Nested Conditionals
- Recursion
- Stack Diagrams for Recursive Functions
- Exercise 5-1
- Infinite Recursion
- Keyboard Input
- Debugging
- Glossary
- Exercises
- Exercise 5-2
- Exercise 5-3
- Exercise 5-4
- Exercise 5-5
- Exercise 5-6
- Exercise 5-7
- 6. Fruitful Functions
- Return Values
- Exercise 6-1
- Incremental Development
- Exercise 6-2
- Composition
- Boolean Functions
- Exercise 6-3
- More Recursion
- Leap of Faith
- One More Example
- Checking Types
- Debugging
- Glossary
- Exercises
- Exercise 6-4
- Exercise 6-5
- Exercise 6-6
- Exercise 6-7
- Exercise 6-8
- Return Values
- 7. Iteration
- Reassignment
- Updating Variables
- The while Statement
- Exercise 7-1
- break
- continue
- Square Roots
- Algorithms
- Debugging
- Glossary
- Exercises
- Exercise 7-2
- Exercise 7-3
- Exercise 7-4
- 8. Strings
- Characters
- A String Is a Sequence
- length
- Traversal
- Exercise 8-1
- Exercise 8-2
- String Slices
- Exercise 8-3
- Strings Are Immutable
- String Interpolation
- Searching
- Exercise 8-4
- Looping and Counting
- Exercise 8-5
- String Library
- The Operator
- String Comparison
- Debugging
- Exercise 8-6
- Glossary
- Exercises
- Exercise 8-7
- Exercise 8-8
- Exercise 8-9
- Exercise 8-10
- Exercise 8-11
- 9. Case Study: Word Play
- Reading Word Lists
- Exercises
- Exercise 9-1
- Exercise 9-2
- Exercise 9-3
- Exercise 9-4
- Exercise 9-5
- Exercise 9-6
- Search
- Looping with Indices
- Debugging
- Glossary
- Exercises
- Exercise 9-7
- Exercise 9-8
- Exercise 9-9
- 10. Arrays
- An Array Is a Sequence
- Arrays Are Mutable
- Traversing an Array
- Array Slices
- Array Library
- Map, Filter, and Reduce
- Dot Syntax
- Deleting (Inserting) Elements
- Arrays and Strings
- Objects and Values
- Aliasing
- Array Arguments
- Debugging
- Glossary
- Exercises
- Exercise 10-1
- Exercise 10-2
- Exercise 10-3
- Exercise 10-4
- Exercise 10-5
- Exercise 10-6
- Exercise 10-7
- Exercise 10-8
- Exercise 10-9
- Exercise 10-10
- Exercise 10-11
- Exercise 10-12
- 11. Dictionaries
- A Dictionary Is a Mapping
- Dictionaries as Collections of Counters
- Exercise 11-1
- Looping and Dictionaries
- Reverse Lookup
- Dictionaries and Arrays
- Memos
- Global Variables
- Debugging
- Glossary
- Exercises
- Exercise 11-2
- Exercise 11-3
- Exercise 11-4
- Exercise 11-5
- Exercise 11-6
- Exercise 11-7
- 12. Tuples
- Tuples Are Immutable
- Tuple Assignment
- Tuples as Return Values
- Variable-Length Argument Tuples
- Exercise 12-1
- Arrays and Tuples
- Dictionaries and Tuples
- Sequences of Sequences
- Debugging
- Glossary
- Exercises
- Exercise 12-2
- Exercise 12-3
- Exercise 12-4
- Exercise 12-5
- 13. Case Study: Data Structure Selection
- Word Frequency Analysis
- Exercise 13-1
- Exercise 13-2
- Exercise 13-3
- Exercise 13-4
- Random Numbers
- Exercise 13-5
- Word Histogram
- Most Common Words
- Optional Parameters
- Dictionary Subtraction
- Exercise 13-6
- Random Words
- Exercise 13-7
- Markov Analysis
- Exercise 13-8
- Data Structures
- Debugging
- Glossary
- Exercises
- Exercise 13-9
- Word Frequency Analysis
- 14. Files
- Persistence
- Reading and Writing
- Formatting
- Filenames and Paths
- Catching Exceptions
- Databases
- Serialization
- Command Objects
- Modules
- Exercise 14-1
- Debugging
- Glossary
- Exercises
- Exercise 14-2
- Exercise 14-3
- Exercise 14-4
- 15. Structs and Objects
- Composite Types
- Structs Are Immutable
- Mutable Structs
- Rectangles
- Instances as Arguments
- Exercise 15-1
- Instances as Return Values
- Copying
- Exercise 15-2
- Debugging
- Glossary
- Exercises
- Exercise 15-3
- Exercise 15-4
- 16. Structs and Functions
- Time
- Exercise 16-1
- Exercise 16-2
- Pure Functions
- Modifiers
- Exercise 16-3
- Exercise 16-4
- Prototyping Versus Planning
- Exercise 16-5
- Debugging
- Glossary
- Exercises
- Exercise 16-6
- Exercise 16-7
- Time
- 17. Multiple Dispatch
- Type Declarations
- Methods
- Exercise 17-1
- Additional Examples
- Constructors
- show
- Exercise 17-2
- Operator Overloading
- Multiple Dispatch
- Exercise 17-3
- Generic Programming
- Interface and Implementation
- Debugging
- Glossary
- Exercises
- Exercise 17-4
- Exercise 17-5
- 18. Subtyping
- Cards
- Global Variables
- Comparing Cards
- Exercise 18-1
- Unit Testing
- Decks
- Add, Remove, Shuffle, and Sort
- Exercise 18-2
- Abstract Types and Subtyping
- Abstract Types and Functions
- Type Diagrams
- Debugging
- Data Encapsulation
- Exercise 18-3
- Glossary
- Exercises
- Exercise 18-4
- Exercise 18-5
- Exercise 18-6
- 19. The Goodies: Syntax
- Named Tuples
- Functions
- Blocks
- Control Flow
- Types
- Methods
- Constructors
- Conversion and Promotion
- Metaprogramming
- Missing Values
- Calling C and Fortran Code
- Glossary
- 20. The Goodies: Base and Standard Library
- Measuring Performance
- Collections and Data Structures
- Exercise 20-1
- Mathematics
- Strings
- Arrays
- Interfaces
- Interactive Utilities
- Debugging
- Glossary
- 21. Debugging
- Syntax Errors
- Runtime Errors
- Semantic Errors
- A. Unicode Input
- B. JuliaBox
- Index