Think Data Structures. Algorithms and Information Retrieval in Java - Helion
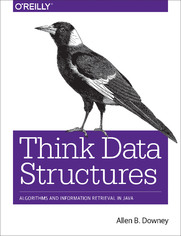
ISBN: 978-14-919-7231-1
stron: 158, Format: ebook
Data wydania: 2017-07-07
Księgarnia: Helion
Cena książki: 126,65 zł (poprzednio: 147,27 zł)
Oszczędzasz: 14% (-20,62 zł)
If you’re a student studying computer science or a software developer preparing for technical interviews, this practical book will help you learn and review some of the most important ideas in software engineering—data structures and algorithms—in a way that’s clearer, more concise, and more engaging than other materials.
By emphasizing practical knowledge and skills over theory, author Allen Downey shows you how to use data structures to implement efficient algorithms, and then analyze and measure their performance. You’ll explore the important classes in the Java collections framework (JCF), how they’re implemented, and how they’re expected to perform. Each chapter presents hands-on exercises supported by test code online.
- Use data structures such as lists and maps, and understand how they work
- Build an application that reads Wikipedia pages, parses the contents, and navigates the resulting data tree
- Analyze code to predict how fast it will run and how much memory it will require
- Write classes that implement the Map interface, using a hash table and binary search tree
- Build a simple web search engine with a crawler, an indexer that stores web page contents, and a retriever that returns user query results
Other books by Allen Downey include Think Java, Think Python, Think Stats, and Think Bayes.
Osoby które kupowały "Think Data Structures. Algorithms and Information Retrieval in Java", wybierały także:
- Jak zhakowa 125,00 zł, (10,00 zł -92%)
- Biologika Sukcesji Pokoleniowej. Sezon 3. Konflikty na terytorium 126,36 zł, (13,90 zł -89%)
- Windows Media Center. Domowe centrum rozrywki 66,67 zł, (8,00 zł -88%)
- Podręcznik startupu. Budowa wielkiej firmy krok po kroku 92,67 zł, (13,90 zł -85%)
- Ruby on Rails. Ćwiczenia 18,75 zł, (3,00 zł -84%)
Spis treści
Think Data Structures. Algorithms and Information Retrieval in Java eBook -- spis treści
- Preface
- The Philosophy Behind the Book
- Prerequisites
- Working with the Code
- Conventions Used in This Book
- Safari Books Online
- How to Contact Us
- Contributors
- 1. Interfaces
- Why Are There Two Kinds of List?
- Interfaces in Java
- List Interface
- Exercise 1
- 2. Analysis of Algorithms
- Selection Sort
- Big O Notation
- Exercise 2
- 3. ArrayList
- Classifying MyArrayList Methods
- Classifying add
- Problem Size
- Linked Data Structures
- Exercise 3
- A Note on Garbage Collection
- 4. LinkedList
- Classifying MyLinkedList Methods
- Comparing MyArrayList and MyLinkedList
- Profiling
- Interpreting Results
- Exercise 4
- 5. Doubly Linked List
- Performance Profiling Results
- Profiling LinkedList Methods
- Adding to the End of a LinkedList
- Doubly Linked List
- Choosing a Structure
- 6. Tree Traversal
- Search Engines
- Parsing HTML
- Using jsoup
- Iterating Through the DOM
- Depth-First Search
- Stacks in Java
- Iterative DFS
- 7. Getting to Philosophy
- Getting Started
- Iterables and Iterators
- WikiFetcher
- Exercise 5
- 8. Indexer
- Data Structure Selection
- TermCounter
- Exercise 6
- 9. The Map Interface
- Implementing MyLinearMap
- Exercise 7
- Analyzing MyLinearMap
- 10. Hashing
- Hashing
- How Does Hashing Work?
- Hashing and Mutation
- Exercise 8
- 11. HashMap
- Exercise 9
- Analyzing MyHashMap
- The Tradeoffs
- Profiling MyHashMap
- Fixing MyHashMap
- UML Class Diagrams
- 12. TreeMap
- Whats Wrong with Hashing?
- Binary Search Tree
- Exercise 10
- Implementing a TreeMap
- 13. Binary Search Tree
- A Simple MyTreeMap
- Searching for Values
- Implementing put
- In-Order Traversal
- The Logarithmic Methods
- Self-Balancing Trees
- One More Exercise
- 14. Persistence
- Redis
- Redis Clients and Servers
- Making a Redis-Backed Index
- Redis Data Types
- Exercise 11
- More Suggestions If You Want Them
- A Few Design Hints
- 15. Crawling Wikipedia
- The Redis-Backed Indexer
- Analysis of Lookup
- Analysis of Indexing
- Graph Traversal
- Exercise 12
- 16. Boolean Search
- Crawler Solution
- Information Retrieval
- Boolean Search
- Exercise 13
- Comparable and Comparator
- Extensions
- 17. Sorting
- Insertion Sort
- Exercise 14
- Analysis of Merge Sort
- Radix Sort
- Heap Sort
- Bounded Heap
- Space Complexity
- Index