The Hitchhiker's Guide to Python. Best Practices for Development - Helion
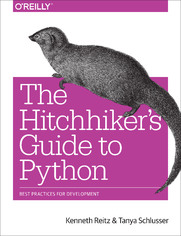
ISBN: 978-14-919-3322-0
stron: 338, Format: ebook
Data wydania: 2016-08-30
Księgarnia: Helion
Cena książki: 80,74 zł (poprzednio: 94,99 zł)
Oszczędzasz: 15% (-14,25 zł)
The Hitchhiker's Guide to Python takes the journeyman Pythonista to true expertise. More than any other language, Python was created with the philosophy of simplicity and parsimony. Now 25 years old, Python has become the primary or secondary language (after SQL) for many business users. With popularity comes diversity—and possibly dilution.
This guide, collaboratively written by over a hundred members of the Python community, describes best practices currently used by package and application developers. Unlike other books for this audience, The Hitchhiker’s Guide is light on reusable code and heavier on design philosophy, directing the reader to excellent sources that already exist.
Osoby które kupowały "The Hitchhiker's Guide to Python. Best Practices for Development", wybierały także:
- Cisco CCNA 200-301. Kurs video. Administrowanie bezpieczeństwem sieci. Część 3 665,00 zł, (39,90 zł -94%)
- Cisco CCNA 200-301. Kurs video. Administrowanie urządzeniami Cisco. Część 2 665,00 zł, (39,90 zł -94%)
- Cisco CCNA 200-301. Kurs video. Podstawy sieci komputerowych i konfiguracji. Część 1 665,00 zł, (39,90 zł -94%)
- Cisco CCNP Enterprise 350-401 ENCOR. Kurs video. Programowanie i automatyzacja sieci 443,33 zł, (39,90 zł -91%)
- CCNP Enterprise 350-401 ENCOR. Kurs video. Mechanizmy kierowania ruchem pakiet 443,33 zł, (39,90 zł -91%)
Spis treści
The Hitchhiker's Guide to Python. Best Practices for Development eBook -- spis treści
- Preface
- Conventions Used in This Book
- Safari Books Online
- How to Contact Us
- Acknowledgments
- I. Getting Started
- 1. Picking an Interpreter
- The State of Python 2 Versus Python 3
- Recommendations
- So3?
- Implementations
- CPython
- Stackless
- PyPy
- Jython
- IronPython
- PythonNet
- Skulpt
- MicroPython
- 2. Properly Installing Python
- Installing Python on Mac OSÂ X
- Setuptools and pip
- virtualenv
- Installing Python on Linux
- Setuptools and pip
- Development Tools
- virtualenv
- Installing Python on Windows
- Setuptools and pip
- virtualenv
- Commercial Python Redistributions
- Installing Python on Mac OSÂ X
- 3. Your Development Environment
- Text Editors
- Sublime Text
- Vim
- Python-mode
- Emacs
- TextMate
- Atom
- Code
- IDEs
- PyCharm/IntelliJ IDEA
- Aptana Studio 3/Eclipse + LiClipse + PyDev
- WingIDE
- Spyder
- NINJA-IDE
- Komodo IDE
- Eric (the Eric Python IDE)
- Visual Studio
- Enhanced Interactive Tools
- IDLE
- IPython
- bpython
- Isolation Tools
- Virtual Environments
- Create and activate the virtual environment
- On Mac OS X and Linux
- On Windows
- Add libraries to the virtual environment
- Deactivate the virtual environment
- Create and activate the virtual environment
- pyenv
- Autoenv
- virtualenvwrapper
- Buildout
- Conda
- Docker
- Virtual Environments
- Text Editors
- II. Getting Down to Business
- 4. Writing Great Code
- Code Style
- PEP 8
- PEPÂ 20 (a.k.a. The Zen of Python)
- General Advice
- Explicit is better than implicit
- Sparse is better than dense
- Errors should never pass silently / Unless explicitly silenced
- Function arguments should be intuitive to use
- If the implementation is hard to explain, its a bad idea
- We are all responsible users
- Return values from one place
- Conventions
- Alternatives to checking for equality
- Accessing dictionary elements
- Manipulating lists
- Continuing a long line of code
- Idioms
- Unpacking
- Ignoring a value
- Creating a length-N list of the same thing
- Exception-safe contexts
- Common Gotchas
- Mutable default arguments
- Late binding closures
- Structuring Your Project
- Modules
- Importing modules
- Packages
- Object-Oriented Programming
- Decorators
- Dynamic Typing
- Mutable and Immutable Types
- Vendorizing Dependencies
- Modules
- Testing Your Code
- Tips for testing
- Just one thing per test
- Independence is imperative
- Precision is better than parsimony
- Speed counts
- RTMF (Read the manual, friend!)
- Test everything when you startand again when you finish
- Version control automation hooks are fantastic
- Write a breaking test if you want to take a break
- In the face of ambiguity, debug using a test
- If the test is hard to explain, good luck finding collaborators
- If the test is easy to explain, it is almost always a good idea
- Above all, dont panic
- Testing Basics
- unittest
- Mock (in unittest)
- doctest
- Examples
- Example: Testing in Tablib
- Example: Testing in Requests
- Other Popular Tools
- pytest
- Nose
- tox
- Options for older versions of Python
- unittest2
- Mock
- fixture
- Lettuce and Behave
- Tips for testing
- Documentation
- Project Documentation
- Project Publication
- Sphinx
- reStructured Text
- Docstring Versus Block Comments
- Logging
- Logging in a Library
- Logging in an Application
- Example configuration via an INI file
- Example configuration via a dictionary
- Example configuration directly in code
- Choosing a License
- Upstream Licenses
- Options
- Licensing Resources
- Code Style
- 5. Reading Great Code
- Common Features
- HowDoI
- Reading a Single-File Script
- Read HowDoIs documentation
- Use HowDoI
- Read HowDoIs code
- Structure Examples from HowDoI
- Let each function do just one thing
- Leverage data available from the system
- Style Examples from HowDoI
- Underscore-prefixed function names (we are all responsible users)
- Handle compatibility in just one place (readability counts)
- Pythonic choices (beautiful is better than ugly)
- Reading a Single-File Script
- Diamond
- Reading a Larger Application
- Read Diamonds documentation
- Use Diamond
- Reading Diamonds code
- Structure Examples from Diamond
- Separate different functionality into namespaces (they are one honking great idea)
- User-extensible custom classes (complex is better than complicated)
- Complex versus complicated
- The simple user interface
- The more complex internal code
- Style Examples from Diamond
- Example use of a closure (when the gotcha isnt a gotcha)
- Reading a Larger Application
- Tablib
- Reading a Small Library
- Read Tablibs documentation
- Use Tablib
- Read Tablibs code
- Structure Examples from Tablib
- No needless object-oriented code in formats (use namespaces for grouping functions)
- Descriptors and the property decorator (engineer immutability when the API would benefit)
- Programmatically registered file formats (dont repeat yourself)
- Vendorized dependencies in packages (an example of how to vendorize)
- Saving memory with __slots__ (optimize judiciously)
- Style Examples from Tablib
- Operator overloading (beautiful is better than ugly)
- Reading a Small Library
- Requests
- Reading a Larger Library
- Read Requestss documentation
- Use Requests
- Read Requestss code
- Structure Examples from Requests
- Top-level API (preferably only one obvious way to do it)
- The Request and PreparedRequest objects (were all responsible users)
- Style Examples from Requests
- Sets and set arithmetic (a nice, Pythonic idiom)
- Status codes (readability counts)
- Reading a Larger Library
- Werkzeug
- Reading Code in a Toolkit
- Read Werkzeugs documentation
- Use Werkzeug
- Read Werkzeugs code
- Style Examples from Werkzeug
- Elegant way to guess type (if the implementation is easy to explain, it may be a good idea)
- Regular expressions (readability counts)
- Structure Examples from Werkzeug
- Class-based decorators (a Pythonic use of dynamic typing)
- Response.__call__
- Mixins (also one honking great idea)
- Reading Code in a Toolkit
- Flask
- Reading Code in a Framework
- Read Flasks documentation
- Use Flask
- Read Flasks code
- Style Examples from Flask
- Flasks routing decorators (beautiful is better than ugly)
- Structure Examples from Flask
- Application specific defaults (simple is better than complex)
- Modularity (also one honking great idea)
- Reading Code in a Framework
- 6. Shipping Great Code
- Useful Vocabulary and Concepts
- Packaging Your Code
- Conda
- PyPI
- Sample project
- Use pip, not easy_install
- Personal PyPI
- Pypiserver
- S3-hosted PyPI
- VCS support for pip
- Freezing Your Code
- PyInstaller
- cx_Freeze
- py2app
- py2exe
- bbFreeze
- Packaging for Linux-Built Distributions
- Executable ZIP Files
- III. Scenario Guide
- 7. User Interaction
- Jupyter Notebooks
- Command-Line Applications
- argparse
- docopt
- Plac
- Click
- Clint
- cliff
- GUI Applications
- Widget Libraries
- Tk
- Kivy
- Qt
- GTK+
- wxWidgets
- Objective-C
- Game Development
- Widget Libraries
- Web Applications
- Web Frameworks/Microframeworks
- Django
- Flask
- Tornado
- Pyramid
- Web Template Engines
- Jinja2
- Chameleon
- Mako
- Web Deployment
- Hosting
- Web servers
- WSGI servers
- Web Frameworks/Microframeworks
- 8. Code Management and Improvement
- Continuous Integration
- Tox
- System Administration
- Travis-CI
- Jenkins
- Buildbot
- Server Automation
- Salt
- Ansible
- Puppet
- Chef
- CFEngine
- System and Task Monitoring
- Psutil
- Fabric
- Luigi
- Speed
- Threading
- Multiprocessing
- Subprocess
- PyPy
- Cython
- Numba
- GPU libraries
- Interfacing with C/C++/FORTRAN Libraries
- C Foreign Function Interface
- ctypes
- F2PY
- SWIG
- Boost.Python
- Continuous Integration
- 9. Software Interfaces
- Web Clients
- Web APIs
- JSON parsing
- XML parsing
- Web scraping
- lxml
- Web APIs
- Data Serialization
- Pickle
- Cross-language serialization
- Compression
- The buffer protocol
- Distributed Systems
- Networking
- Performance networking tools in Pythons Standard Library
- gevent
- Twisted
- PyZMQ
- RabbitMQ
- Networking
- Cryptography
- ssl, hashlib, and secrets
- pyOpenSSL
- PyNaCl and libnacl
- Cryptography
- PyCrypto
- bcrypt
- Web Clients
- 10. Data Manipulation
- Scientific Applications
- IPython
- NumPy
- SciPy
- Matplotlib
- Pandas
- Scikit-Learn
- Rpy2
- decimal, fractions, and numbers
- SymPy
- Text Manipulation and Text Mining
- String Tools in Pythons Standard Library
- nltk
- SyntaxNet
- Image Manipulation
- Pillow
- cv2
- Scikit-Image
- String Tools in Pythons Standard Library
- Scientific Applications
- 11. Data Persistence
- Structured Files
- Database Libraries
- sqlite3
- SQLAlchemy
- Django ORM
- peewee
- PonyORM
- SQLObject
- Records
- NoSQL database libraries
- A. Additional Notes
- Pythons Community
- BDFL
- Python Software Foundation
- PEPs
- Notable PEPs
- Submitting a PEP
- Python conferences
- Python user groups
- Learning Python
- Beginners
- Intermediate
- Advanced
- For Engineers and Scientists
- Miscellaneous Topics
- References
- Documentation
- News
- Pythons Community
- Index