Swift Pocket Reference. Programming for iOS and OS X. 2nd Edition - Helion
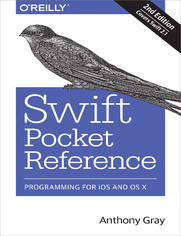
ISBN: 978-14-919-4002-0
stron: 236, Format: ebook
Data wydania: 2015-11-12
Księgarnia: Helion
Cena książki: 59,90 zł
Get quick answers for developing and debugging applications with Swift, Apple’s multi-paradigm programming language. Updated to cover the latest features in Swift 2.0, this pocket reference is the perfect on-the-job tool for learning Swift’s modern language features, including type safety, generics, type inference, closures, tuples, automatic memory management, and support for Unicode.
Designed to work with Cocoa and Cocoa Touch, Swift can be used in tandem with Objective-C, and either language can call APIs implemented in the other. Swift is still evolving, but Apple clearly sees it as the future language of choice for iOS and OS X software development.
Topics include:
- Supported data types, such as strings, arrays, array slices, sets, and dictionaries
- Program flow: loops, conditional execution, and error handling
- Classes, structures, enumerations, and functions
- Protocols, extensions, and generics
- Memory management
- Closures: similar to blocks in Objective-C and lambdas in C#
- Optionals: values that can explicitly have no value
- Operators, operator overloading, and custom operators
- Access control: restricting access to types, methods, and properties
- Ranges, intervals, and strides
- A full list of built-in global functions and their parameter requirements
Osoby które kupowały "Swift Pocket Reference. Programming for iOS and OS X. 2nd Edition", wybierały także:
- Zen Steve'a Jobsa 29,67 zł, (8,90 zł -70%)
- Programowanie aplikacji mobilnych dla iOS z wykorzystaniem Xcode, Swift 3.0 i iOS 10 SDK. Kurs video. Poziom pierwszy 99,00 zł, (44,55 zł -55%)
- Flutter i Dart 2 dla początkujących. Przewodnik dla twórców aplikacji mobilnych 89,00 zł, (44,50 zł -50%)
- Dostosuj się lub giń. Jak odnieść sukces w branży aplikacji mobilnych 44,90 zł, (22,45 zł -50%)
- Podstawy języka Swift. Programowanie aplikacji dla platformy iOS 49,00 zł, (24,50 zł -50%)
Spis treści
Swift Pocket Reference. Programming for iOS and OS X. 2nd Edition eBook -- spis treści
- Introduction
- Conventions Used in This Book
- Using Code Examples
- Safari Books Online
- How to Contact Us
- Acknowledgments
- Getting Started with Swift 2
- The Swift REPL
- Multiple Xcode installations
- Starting the REPL
- Swift as a Scripting Language
- Swift Playgrounds
- The Swift REPL
- A Taste of Swift
- Basic Language Features
- Comments
- Semicolons
- Whitespace
- Naming Conventions
- Importing Code from Other Modules
- Types
- Specific Integer Types
- Numeric Literals
- Character and String Literals
- Type Aliases
- Nested Types
- Other Types
- Variables and Constants
- Computed Variables
- Variable Observers
- Tuples
- Tuple Variables and Constants
- Extracting Tuple Components
- Naming Tuple Components
- Using Type Aliases with Tuples
- Tuples as Return Types
- Operators
- No Implicit Type Conversion
- Arithmetic Operators
- Bitwise Operators
- Assignment Operators
- Comparison Operators
- Logical Operators
- Overflow Operators
- Type Casting Operators
- Range Operators
- Ternary Conditional Operator
- Operator Precedence
- Strings and Characters
- String Properties
- Comparing Strings
- Escaped Characters in Strings
- String Interpolation
- Converting Strings to Numeric Types
- Character-Level Access in Strings
- String Inherited Functionality
- Arrays
- Accessing Array Elements
- Array Properties
- Modifying a Mutable Array
- Iterating Over Arrays
- Array Inherited Functionality
- Slices
- Dictionaries
- Accessing Dictionary Elements
- Dictionary Properties
- Modifying a Mutable Dictionary
- Iterating Over Dictionaries
- Dictionary Inherited Functionality
- Sets
- Accessing Set Items and Properties
- Modifying a Mutable Set
- Iterating Over SetsÂ
- Set Operations
- Set Inherited Functionality
- Option Sets
- Functions
- Parameter Types
- Returning Optional Values
- Returning Multiple Values by using Tuples
- The optional tuple return type
- Local and External Parameter Names
- Default Parameter Values
- Variadic Parameters
- Function Types
- Closures
- Automatic Argument Names
- Trailing Closures
- Capturing Values
- Capturing Values by Reference
- Optionals
- Unwrapping Optionals
- Implicitly Unwrapped Optionals
- Optional Binding
- Optional Chaining
- Program Flow
- Loops
- for-condition-increment loops
- for-in loops
- for-in variations
- while loops
- repeat-while loops
- Early termination of loops
- Conditional Execution
- if-else
- if-case
- guard-else
- switch
- Matching ranges in a case clause
- Using tuples in a case clause
- Value binding with tuples and ranges
- The where qualifier
- Using switch with enumerations
- Statement labels
- Do scopes
- Deferred execution
- if-else
- Error Handling
- Rethrowing functions and methods
- Forced Try
- Optional Try
- Loops
- Classes
- Defining a Base Class
- Instances
- Properties
- Stored properties
- Lazy initialization of stored properties
- Computed properties
- Property observers
- Instance versus type properties
- Static properties
- Computed type properties
- Constant properties
- Stored properties
- Methods
- Local and external parameter names
- Self
- Type methods
- Subscripts
- Member Protection
- Inheritance: Deriving One Class from Another
- Overriding Superclass Entities
- Accessing overridden superclass entities
- Overriding properties
- Overriding methods and subscripts
- Preventing Overrides and Subclassing
- Initialization
- Designated initializers
- Convenience initializers
- Failable initializers
- Initialization and Inheritance
- Overriding initializers
- Required initializers
- Deinitialization
- Structures
- Properties in Structures
- Methods in Structures
- Mutating methods
- Type Methods for Structures
- Initializers in Structures
- Initializer delegation in structures
- Enumerations
- Raw Member Values
- Associated Values
- Methods in Enumerations
- Type Methods for Enumerations
- Recursive Enumerations
- Failable Initializers in Enumerations
- Access Control
- Specifying Access Control Levels
- Default Access Control Levels
- Extensions
- Computed Property Extensions
- Initializer Extensions
- Method Extensions
- Subscript Extensions
- Checking and Casting Types
- Any and AnyObject
- Checking Types
- Downcasting Types
- Protocols
- Required Properties
- Required Methods
- Optional Methods and Properties
- Initializers in Protocols
- Adopting Protocols with Extensions
- Inheritance and Protocols
- Using a Protocol as a Type
- Checking Protocol Conformance
- Protocol Extensions
- Built-In Protocols
- The GeneratorType Protocol
- The SequenceType Protocol
- The CollectionType Protocol
- Memory Management
- How Reference Counting Works
- Retain Cycles and Strong References
- Weak References
- Unowned References
- Retain Cycles and Closures
- Generics
- Generic Functions
- Generic Types
- Constraining Types
- The where type constraint clause
- Generic Protocols
- Operator Overloading
- Overloading Unary Operators
- Custom Operators
- Custom Operator Precedence
- Ranges, Intervals, and Strides
- Ranges
- Intervals
- Strides
- Global Functions
- Changes From Swift 1.0
- Index