React Cookbook - Helion
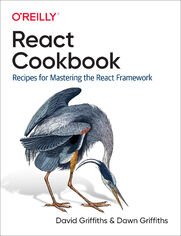
ISBN: 9781492085799
stron: 512, Format: ebook
Data wydania: 2021-08-11
Księgarnia: Helion
Cena książki: 186,15 zł (poprzednio: 216,45 zł)
Oszczędzasz: 14% (-30,30 zł)
React helps you create and work on an app in just a few minutes. But learning how to put all the pieces together is hard. How do you validate a form? Or implement a complex multistep user action without writing messy code? How do you test your code? Make it reusable? Wire it to a backend? Keep it easy to understand? The React Cookbook delivers answers fast.
Many books teach you how to get started, understand the framework, or use a component library with React, but very few provide examples to help you solve particular problems. This easy-to-use cookbook includes the example code developers need to unravel the most common problems when using React, categorized by topic area and problem.
You'll learn how to:
- Build a single-page application in React using a rich UI
- Create progressive web applications that users can install and work with offline
- Integrate with backend services such as REST and GraphQL
- Automatically test for accessibility problems in your application
- Secure applications with fingerprints and security tokens using WebAuthn
- Deal with bugs and avoid common functional and performance problems
Osoby które kupowały "React Cookbook", wybierały także:
- Windows Media Center. Domowe centrum rozrywki 66,67 zł, (8,00 zł -88%)
- Ruby on Rails. Ćwiczenia 18,75 zł, (3,00 zł -84%)
- Przywództwo w świecie VUCA. Jak być skutecznym liderem w niepewnym środowisku 58,64 zł, (12,90 zł -78%)
- Scrum. O zwinnym zarządzaniu projektami. Wydanie II rozszerzone 58,64 zł, (12,90 zł -78%)
- Od hierarchii do turkusu, czyli jak zarządzać w XXI wieku 58,64 zł, (12,90 zł -78%)
Spis treści
React Cookbook eBook -- spis treści
- Preface
- Conventions Used in This Book
- Using Code Examples
- OReilly Online Learning
- How to Contact Us
- Acknowledgments
- 1. Creating Applications
- Generate a Simple Application
- Problem
- Solution
- Discussion
- Build Content-Rich Apps with Gatsby
- Problem
- Solution
- Discussion
- Build Universal Apps with Razzle
- Problem
- Solution
- Discussion
- Manage Server and Client Code with Next.js
- Problem
- Solution
- Discussion
- Create a Tiny App with Preact
- Problem
- Solution
- Discussion
- Build Libraries with nwb
- Problem
- Solution
- Discussion
- Add React to Rails with Webpacker
- Problem
- Solution
- Discussion
- Create Custom Elements with Preact
- Problem
- Solution
- Discussion
- Use Storybook for Component Development
- Problem
- Solution
- Discussion
- Test Your Code in a Browser with Cypress
- Problem
- Solution
- Discussion
- Generate a Simple Application
- 2. Routing
- Create Interfaces with Responsive Routes
- Problem
- Solution
- Discussion
- Move State into Routes
- Problem
- Solution
- Discussion
- Use MemoryRouter for Unit Testing
- Problem
- Solution
- Discussion
- Use Prompt for Page Exit Confirmations
- Problem
- Solution
- Discussion
- Create Transitions with React Transition Group
- Problem
- Solution
- Discussion
- Create Secured Routes
- Problem
- Solution
- Discussion
- Create Interfaces with Responsive Routes
- 3. Managing State
- Use Reducers to Manage Complex State
- Problem
- Solution
- Discussion
- Create an Undo Feature
- Problem
- Solution
- Discussion
- Create and Validate Forms
- Problem
- Solution
- Discussion
- Measure Time with a Clock
- Problem
- Solution
- Discussion
- Monitor Online Status
- Problem
- Solution
- Discussion
- Manage Global State with Redux
- Problem
- Solution
- Discussion
- Survive Page Reloads with Redux Persist
- Problem
- Solution
- Discussion
- Calculate Derived State with Reselect
- Problem
- Solution
- Discussion
- Use Reducers to Manage Complex State
- 4. Interaction Design
- Build a Centralized Error Handler
- Problem
- Solution
- Discussion
- Create an Interactive Help Guide
- Problem
- Solution
- Discussion
- Use Reducers for Complex Interactions
- Problem
- Solution
- Discussion
- Add Keyboard Interaction
- Problem
- Solution
- Discussion
- Use Markdown for Rich Content
- Problem
- Solution
- Discussion
- Animate with CSS Classes
- Problem
- Solution
- Discussion
- Animate with React Animation
- Problem
- Solution
- Discussion
- Animate Infographics with TweenOne
- Problem
- Solution
- Discussion
- Build a Centralized Error Handler
- 5. Connecting to Services
- Convert Network Calls to Hooks
- Problem
- Solution
- Discussion
- Refresh Automatically with State Counters
- Problem
- Solution
- Discussion
- Cancel Network Requests with Tokens
- Problem
- Solution
- Discussion
- Make Network Calls with Redux Middleware
- Problem
- Solution
- Discussion
- Connect to GraphQL
- Problem
- Solution
- Discussion
- Reduce Network Load with Debounced Requests
- Problem
- Solution
- Discussion
- Convert Network Calls to Hooks
- 6. Component Libraries
- Use Material Design with Material-UI
- Problem
- Solution
- Discussion
- Create a Simple UI with React Bootstrap
- Problem
- Solution
- Discussion
- View Data Sets with React Window
- Problem
- Solution
- Discussion
- Create Responsive Dialogs with Material-UI
- Problem
- Solution
- Discussion
- Build an Admin Console with React Admin
- Problem
- Solution
- Discussion
- No Designer? Use Semantic UI
- Problem
- Solution
- Discussion
- Use Material Design with Material-UI
- 7. Security
- Secure Requests, Not Routes
- Problem
- Solution
- Discussion
- Authenticate with Physical Tokens
- Problem
- Solution
- Discussion
- Enable HTTPS
- Problem
- Solution
- Discussion
- Authenticate with Fingerprints
- Problem
- Solution
- Discussion
- Use Confirmation Logins
- Problem
- Solution
- Discussion
- Use Single-Factor Authentication
- Problem
- Solution
- Discussion
- Test on an Android Device
- Problem
- Solution
- Discussion
- Check Security with ESlint
- Problem
- Solution
- Discussion
- Make Login Forms Browser Friendly
- Problem
- Solution
- Discussion
- Secure Requests, Not Routes
- 8. Testing
- Use the React Testing Library
- Problem
- Solution
- Discussion
- Use Storybook for Render Tests
- Problem
- Solution
- Discussion
- Test Without a Server Using Cypress
- Problem
- Solution
- Discussion
- Use Cypress for Offline Testing
- Problem
- Solution
- Discussion
- Test in a Browser with Selenium
- Problem
- Solution
- Discussion
- Test Cross-Browser Visuals with ImageMagick
- Problem
- Solution
- Discussion
- Add a Console to Mobile Browsers
- Problem
- Solution
- Discussion
- Remove Randomness from Tests
- Problem
- Solution
- Discussion
- Time Travel
- Problem
- Solution
- Discussion
- Use the React Testing Library
- 9. Accessibility
- Use Landmarks
- Problem
- Solution
- Discussion
- Apply Roles, Alts, and Titles
- Problem
- Solution
- Discussion
- Check Accessibility with ESlint
- Problem
- Solution
- Discussion
- Use Axe DevTools at Runtime
- Problem
- Solution
- Discussion
- Automate Browser Testing with Cypress Axe
- Problem
- Solution
- Discussion
- Add Skip Buttons
- Problem
- Solution
- Discussion
- Add Skip Regions
- Problem
- Solution
- Discussion
- Capture Scope in Modals
- Problem
- Solution
- Discussion
- Create a Page Reader with the Speech API
- Problem
- Solution
- Discussion
- Use Landmarks
- 10. Performance
- Use Browser Performance Tools
- Problem
- Solution
- Discussion
- Track Rendering with Profiler
- Problem
- Solution
- Discussion
- Create Profiler Unit Tests
- Problem
- Solution
- Discussion
- Measure Time Precisely
- Problem
- Solution
- Discussion
- Shrink Your App with Code Splitting
- Problem
- Solution
- Discussion
- Combine Network Promises
- Problem
- Solution
- Discussion
- Use Server-Side Rendering
- Problem
- Solution
- Discussion
- Use Web Vitals
- Problem
- Solution
- Discussion
- Use Browser Performance Tools
- 11. Progressive Web Applications
- Create Service Workers with Workbox
- Problem
- Solution
- Discussion
- Build a PWA with Create React App
- Problem
- Solution
- Discussion
- Cache Third-Party Resources
- Problem
- Solution
- Discussion
- Automatically Reload Workers
- Problem
- Solution
- Discussion
- Add Notifications
- Problem
- Solution
- Discussion
- Make Offline Changes with Background Sync
- Problem
- Solution
- Discussion
- Add a Custom Installation UI
- Problem
- Solution
- Discussion
- Provide Offline Responses
- Problem
- Solution
- Discussion
- Create Service Workers with Workbox
- Index