Programming TypeScript. Making Your JavaScript Applications Scale - Helion
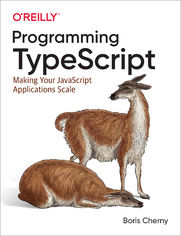
ISBN: 978-14-920-3760-6
stron: 324, Format: ebook
Data wydania: 2019-04-25
Księgarnia: Helion
Cena książki: 152,15 zł (poprzednio: 176,92 zł)
Oszczędzasz: 14% (-24,77 zł)
Any programmer working with a dynamically typed language will tell you how hard it is to scale to more lines of code and more engineers. That’s why Facebook, Google, and Microsoft invented gradual static type layers for their dynamically typed JavaScript and Python code. This practical book shows you how one such type layer, TypeScript, is unique among them: it makes programming fun with its powerful static type system.
If you’re a programmer with intermediate JavaScript experience, author Boris Cherny will teach you how to master the TypeScript language. You’ll understand how TypeScript can help you eliminate bugs in your code and enable you to scale your code across more engineers than you could before.
In this book, you’ll:
- Start with the basics: Learn about TypeScript’s different types and type operators, including what they’re for and how they’re used
- Explore advanced topics: Understand TypeScript’s sophisticated type system, including how to safely handle errors and build asynchronous programs
- Dive in hands-on: Use TypeScript with your favorite frontend and backend frameworks, migrate your existing JavaScript project to TypeScript, and run your TypeScript application in production
Osoby które kupowały "Programming TypeScript. Making Your JavaScript Applications Scale", wybierały także:
- D3.js w akcji 67,42 zł, (20,90 zł -69%)
- Tablice informatyczne. Node.js 16,86 zł, (5,90 zł -65%)
- React i GatsbyJS. Kurs video. Nowoczesne strony internetowe w oparciu o headless CMS i GraphQL 148,98 zł, (59,59 zł -60%)
- TypeScript i React. Kurs video. Tworzenie nowoczesnych komponent 129,00 zł, (51,60 zł -60%)
- Vue.js w praktyce. Kurs video. Poznaj narz 148,98 zł, (59,59 zł -60%)
Spis treści
Programming TypeScript. Making Your JavaScript Applications Scale eBook -- spis treści
- Preface
- How This Book Is Organized
- Style
- Conventions Used in This Book
- Using Code Examples
- OReilly Online Learning
- How to Contact Us
- Acknowledgments
- 1. Introduction
- 2. TypeScript: A 10_000 Foot View
- The Compiler
- The Type System
- TypeScript Versus JavaScript
- How are types bound?
- Are types automatically converted?
- When are types checked?
- When are errors surfaced?
- TypeScript Versus JavaScript
- Code Editor Setup
- tsconfig.json
- tslint.json
- index.ts
- Exercises
- 3. All About Types
- Talking About Types
- The ABCs of Types
- any
- unknown
- boolean
- number
- bigint
- string
- symbol
- Objects
- Intermission: Type Aliases, Unions, and Intersections
- Type aliases
- Union and intersection types
- Arrays
- Tuples
- Read-only arrays and tuples
- null, undefined, void, and never
- Enums
- Summary
- Exercises
- 4. Functions
- Declaring and Invoking Functions
- Optional and Default Parameters
- Rest Parameters
- call, apply, and bind
- Typing this
- Generator Functions
- Iterators
- Call Signatures
- Contextual Typing
- Overloaded Function Types
- Polymorphism
- When Are Generics Bound?
- Where Can You Declare Generics?
- Generic Type Inference
- Generic Type Aliases
- Bounded Polymorphism
- Bounded polymorphism with multiple constraints
- Using bounded polymorphism to model arity
- Generic Type Defaults
- Type-Driven Development
- Summary
- Exercises
- Declaring and Invoking Functions
- 5. Classes and Interfaces
- Classes and Inheritance
- super
- Using this as a Return Type
- Interfaces
- Declaration Merging
- Implementations
- Implementing Interfaces Versus Extending Abstract Classes
- Classes Are Structurally Typed
- Classes Declare Both Values and Types
- Polymorphism
- Mixins
- Decorators
- Simulating final Classes
- Design Patterns
- Factory Pattern
- Builder Pattern
- Summary
- Exercises
- 6. Advanced Types
- Relationships Between Types
- Subtypes and Supertypes
- Variance
- Shape and array variance
- Function variance
- Assignability
- Type Widening
- The const type
- Excess property checking
- Refinement
- Discriminated union types
- Totality
- Advanced Object Types
- Type Operators for Object Types
- The keying-in operator
- The keyof operator
- The Record Type
- Mapped Types
- Built-in mapped types
- Companion Object Pattern
- Type Operators for Object Types
- Advanced Function Types
- Improving Type Inference for Tuples
- User-Defined Type Guards
- Conditional Types
- Distributive Conditionals
- The infer Keyword
- Built-in Conditional Types
- Escape Hatches
- Type Assertions
- Nonnull Assertions
- Definite Assignment Assertions
- Simulating Nominal Types
- Safely Extending the Prototype
- Summary
- Exercises
- Relationships Between Types
- 7. Handling Errors
- Returning null
- Throwing Exceptions
- Returning Exceptions
- The Option Type
- Summary
- Exercises
- 8. Asynchronous Programming, Concurrency, and Parallelism
- JavaScripts Event Loop
- Working with Callbacks
- Regaining Sanity with Promises
- async and await
- Async Streams
- Event Emitters
- Typesafe Multithreading
- In the Browser: With Web Workers
- Typesafe protocols
- In NodeJS: With Child Processes
- In the Browser: With Web Workers
- Summary
- Exercises
- 9. Frontend and Backend Frameworks
- Frontend Frameworks
- React
- A JSX primer
- TSX = JSX + TypeScript
- Using TSX with React
- Angular 6/7
- Scaffolding
- Components
- Services
- React
- Typesafe APIs
- Backend Frameworks
- Summary
- Frontend Frameworks
- 10. Namespaces.Modules
- A Brief History of JavaScript Modules
- import, export
- Dynamic Imports
- Using CommonJS and AMD Code
- Module Mode Versus Script Mode
- Namespaces
- Collisions
- Compiled Output
- Declaration Merging
- Summary
- Exercise
- 11. Interoperating with JavaScript
- Type Declarations
- Ambient Variable Declarations
- Ambient Type Declarations
- Ambient Module Declarations
- Gradually Migrating from JavaScript to TypeScript
- Step 1: Add TSC
- Step 2a: Enable Typechecking for JavaScript (Optional)
- Step 2b: Add JSDoc Annotations (Optional)
- Step 3: Rename Your Files to .ts
- Step 4: Make It strict
- Type Lookup for JavaScript
- Using Third-Party JavaScript
- JavaScript That Comes with Type Declarations
- JavaScript That Has Type Declarations on DefinitelyTyped
- JavaScript That Doesnt Have Type Declarations on DefinitelyTyped
- Summary
- Type Declarations
- 12. Building and Running TypeScript
- Building Your TypeScript Project
- Project Layout
- Artifacts
- Dialing In Your Compile Target
- target
- lib
- Enabling Source Maps
- Project References
- Error Monitoring
- Running TypeScript on the Server
- Running TypeScript in the Browser
- Publishing Your TypeScript Code to NPM
- Triple-Slash Directives
- The types Directive
- The amd-module Directive
- Summary
- Building Your TypeScript Project
- 13. Conclusion
- A. Type Operators
- B. Type Utilities
- C. Scoped Declarations
- Does It Generate a Type?
- Does It Merge?
- D. Recipes for Writing Declaration Files for Third-Party JavaScript Modules
- Types of Exports
- Globals
- ES2015 Exports
- CommonJS Exports
- UMD Exports
- Extending a Module
- Globals
- Modules
- Types of Exports
- E. Triple-Slash Directives
- Internal Directives
- Deprecated Directives
- F. TSC Compiler Flags for Safety
- G. TSX
- Index