Programming Excel with VBA and .NET - Helion
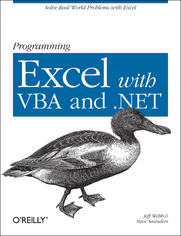
ISBN: 978-14-493-7905-6
stron: 1116, Format: ebook
Data wydania: 2006-04-25
Księgarnia: Helion
Cena książki: 143,65 zł (poprzednio: 167,03 zł)
Oszczędzasz: 14% (-23,38 zł)
Why program Excel? For solving complex calculations and presenting results, Excel is amazingly complete with every imaginable feature already in place. But programming Excel isn't about adding new features as much as it's about combining existing features to solve particular problems. With a few modifications, you can transform Excel into a task-specific piece of software that will quickly and precisely serve your needs. In other words, Excel is an ideal platform for probably millions of small spreadsheet-based software solutions.
The best part is, you can program Excel with no additional tools. A variant of the Visual Basic programming language, VB for Applications (VBA) is built into Excel to facilitate its use as a platform. With VBA, you can create macros and templates, manipulate user interface features such as menus and toolbars, and work with custom user forms or dialog boxes. VBA is relatively easy to use, but if you've never programmed before, Programming Excel with VBA and .NET is a great way to learn a lot very quickly. If you're an experienced Excel user or a Visual Basic programmer, you'll pick up a lot of valuable new tricks. Developers looking forward to .NET development will also find discussion of how the Excel object model works with .NET tools, including Visual Studio Tools for Office (VSTO).
This book teaches you how to use Excel VBA by explaining concepts clearly and concisely in plain English, and provides plenty of downloadable samples so you can learn by doing. You'll be exposed to a wide range of tasks most commonly performed with Excel, arranged into chapters according to subject, with those subjects corresponding to one or more Excel objects. With both the samples and important reference information for each object included right in the chapters, instead of tucked away in separate sections, Programming Excel with VBA and .NET covers the entire Excel object library. For those just starting out, it also lays down the basic rules common to all programming languages.
With this single-source reference and how-to guide, you'll learn to use the complete range of Excel programming tasks to solve problems, no matter what you're experience level.
Osoby które kupowały "Programming Excel with VBA and .NET", wybierały także:
- Gray Hat C#. Język C# w kontroli i łamaniu zabezpieczeń 57,74 zł, (17,90 zł -69%)
- Programowanie asynchroniczne i równoległe w C#. Kurs video. Poziom podstawowy 69,00 zł, (31,05 zł -55%)
- Technologia LINQ. Kurs video. Warsztat pracy z danymi z różnych źródeł 59,00 zł, (26,55 zł -55%)
- Język XAML. Kurs video. Poziom pierwszy. Programowanie aplikacji w WPF 59,00 zł, (26,55 zł -55%)
- ASP.NET MVC. Kurs video. Poziom pierwszy. Programowanie aplikacji internetowych 79,00 zł, (35,55 zł -55%)
Spis treści
Programming Excel with VBA and .NET eBook -- spis treści
- Programming Excel with VBA and .NET
- SPECIAL OFFER: Upgrade this ebook with OReilly
- A Note Regarding Supplemental Files
- Preface
- Learn by Doing
- Dont Force It
- Excel Versions
- Get the Samples
- What's in This Book
- Font Conventions
- Syntax Conventions
- Using Code Examples
- Safari Enabled
- How to Contact Us
- Acknowledgments
- I. Learning VBA
- 1. Becoming an Excel Programmer
- 1.1. Why Program?
- 1.2. Record and Read Code
- 1.3. Change Recorded Code
- 1.4. Fix Misteakes
- 1.4.1. Fix Syntax Errors
- 1.4.2. Fix Compile-Time Errors
- 1.4.3. Fix Runtime Errors
- 1.5. Start and Stop
- 1.6. View Results
- 1.7. Where's My Code?
- 1.8. Macros and Security
- 1.9. Write Bug-Free Code
- 1.10. Navigate Samples and Help
- 1.11. What You've Learned
- 2. Knowing the Basics
- 2.1. Parts of a Program
- 2.2. Classes and Modules
- 2.3. Procedures
- 2.3.1. Arguments and Results
- 2.3.2. Optional Arguments
- 2.3.3. Named Arguments
- 2.3.4. Properties
- 2.3.5. Events
- 2.4. Variables
- 2.4.1. Names
- 2.4.2. Declarations
- 2.4.3. Conversions
- 2.4.4. Scope and Lifetime
- 2.4.5. Scope for Procedures
- 2.4.6. Constants and Enumerations
- 2.4.7. Arrays
- 2.4.8. User-Defined Types
- 2.4.9. Objects
- 2.5. Conditional Statements
- 2.6. Loops
- 2.7. Expressions
- 2.8. Exceptions
- 2.9. What You've Learned
- 3. Tasks in Visual Basic
- 3.1. Types of Tasks
- 3.2. Interact with Users
- 3.3. Do Math
- 3.4. Work with Text
- 3.4.1. Compare Strings
- 3.4.2. Convert Strings
- 3.4.3. Change Strings
- 3.4.4. Repeat Characters
- 3.5. Get Dates and Times
- 3.6. Read and Write Files
- 3.6.1. Sequential Access
- 3.6.2. Random Access
- 3.6.3. Common Tasks
- 3.7. Check Results
- 3.8. Find Truth
- 3.9. Compare Bits
- 3.10. Run Other Applications
- 3.11. Control the Compiler
- 3.12. Not Covered Here
- 3.13. What You've Learned
- 4. Using Excel Objects
- 4.1. Objects and Their Members
- 4.2. Get Excel Objects
- 4.3. Get Objects from Collections
- 4.4. About Me and the Active Object
- 4.5. Find the Right Object
- 4.5.1. Top-Level Objects
- 4.5.2. Workbook Objects
- 4.5.3. Worksheet and Range Objects
- 4.5.4. Chart Objects
- 4.5.5. Data List and XML Objects
- 4.5.6. Database Objects
- 4.5.7. Dialog Box and Form Objects
- 4.6. Common Members
- 4.6.1. Activate Objects: Get Names and Values
- 4.6.2. Add or Delete Objects Through Collections
- 4.6.3. Change Size and Position of Objects
- 4.6.4. Print Objects
- 4.7. Respond to Events in Excel
- 4.8. The Global Object
- 4.9. The WorksheetFunction Object
- 4.10. What You've Learned
- 5. Creating Your Own Objects
- 5.1. Modules Versus Classes
- 5.1.1. Modules
- 5.1.2. Classes
- 5.2. Add Methods
- 5.3. Create Properties
- 5.3.1. Read-Only Properties
- 5.3.2. Write-Once/Write-Only Properties
- 5.4. Define Enumerations
- 5.5. Raise Events
- 5.6. Collect Objects
- 5.7. Expose Objects
- 5.8. Destroy Objects
- 5.9. Things You Can't Do
- 5.10. What You've Learned
- 5.1. Modules Versus Classes
- 6. Writing Code for Use by Others
- 6.1. Types of Applications
- 6.2. The Development Process
- 6.3. Determine Requirements
- 6.4. Design
- 6.5. Implement and Test
- 6.6. Integrate
- 6.7. Test Platforms
- 6.8. Document
- 6.9. Deploy
- 6.9.1. Protect Code
- 6.9.2. Sign Files
- 6.9.3. When Signatures Expire
- 6.9.4. Install Workbooks
- 6.9.5. Install Templates and Add-ins
- 6.10. What You've Learned
- 6.11. Resources
- 1. Becoming an Excel Programmer
- II. Excel Objects
- 7. Controlling Excel
- 7.1. Perform Tasks
- 7.1.1. Quit Excel
- 7.1.2. Lock Out User Actions
- 7.1.3. Open and Close Excel Windows
- 7.1.4. Display Dialogs
- 7.2. Control Excel Options
- 7.2.1. Set Startup Paths
- 7.2.2. View System Settings
- 7.3. Get References
- 7.4. Application Members
- [Application.]ActivateMicrosoftApp(XlMSApplication)
- [Application.]ActivePrinter [= setting]
- Application.AddChartAutoFormat(Chart, Name, [Description])
- Application.AddCustomList(ListArray, [ByRow])
- Application.AlertBeforeOverwriting [= setting]
- Application.AltStartupPath
- Application.ArbitraryXMLSupportAvailable
- Application.AskToUpdateLinks [= setting]
- Application.Assistant
- Application.AutoCorrect
- Application.AutoFormatAsYouTypeReplaceHyperlinks [= setting]
- Application.AutomationSecurity [=MsoAutomationSecurity]
- Application.AutoPercentEntry [= setting]
- Application.AutoRecover
- Application.Build
- [Application.]Calculate( )
- Application.CalculateBeforeSave [= setting]
- Application.CalculateFull( )
- Application.CalculateFullRebuild( )
- Application.Calculation [= XlCalculation]
- Application.CalculationInterruptKey [= XlCalculationInterruptKey]
- Application.CalculationState
- Application.CalculationVersion
- Application.Caller
- Application.Caption [= setting]
- Application.CellDragAndDrop [= setting]
- [Application.]Cells[(row, column)]
- Application.CentimetersToPoints(Centimeters)
- [Application.]Charts([index])
- Application.CheckAbort([KeepAbort])
- Application.CheckSpelling(Word, [CustomDictionary], [IgnoreUppercase])
- Application.ClipboardFormats
- [Application].Columns([index])
- Application.COMAddIns([index])
- Application.CommandBars([index])
- Application.CommandUnderlines [= xlCommandUnderlines]
- Application.ConstrainNumeric [= setting]
- Application.ControlCharacters [= setting]
- Application.ConvertFormula(Formula, FromReferenceStyle, [ToReferenceStyle], [ToAbsolute], [RelativeTo])
- Application.CopyObjectsWithCells [= setting]
- Application.Cursor [= XlMousePointer]
- Application.CursorMovement [= setting]
- Application.CustomListCount
- Application.CutCopyMode [= setting]
- Application.DataEntryMode [= setting]
- Application.DecimalSeparator [= setting]
- Application.DefaultFilePath [= setting]
- Application.DefaultSaveFormat [= XlFileFormat]
- Application.DefaultSheetDirection [= setting]
- Application.DefaultWebOptions
- Application.DeleteChartAutoFormat(Name)
- [Application.]DeleteCustomList(ListNum)
- Application.Dialogs(XlBuiltInDialog)
- Application.DisplayAlerts [= setting]
- Application.DisplayClipboardWindow [= setting]
- Application.DisplayCommentIndicator [=XlCommentDisplayMode]
- Application.DisplayDocumentActionTaskPane [= setting]
- Application.DisplayExcel4Menus [= setting]
- Application.DisplayFormulaBar [= setting]
- Application.DisplayFullScreen [= setting]
- Application.DisplayFunctionToolTips [= setting]
- Application.DisplayInsertOptions [= setting]
- Application.DisplayNoteIndicator [= setting]
- Application.DisplayPasteOptions [= setting]
- Application.DisplayRecentFiles [= setting]
- Application.DisplayScrollBars [= setting]
- Application.DisplayStatusBar [= setting]
- Application.DisplayXMLSourcePane ([XmlMap])
- Application.DoubleClick( )
- Application.EditDirectlyInCell [= setting]
- Application.EnableAnimations [= setting]
- [Application.]EnableAutoComplete [= setting]
- Application.EnableCancelKey [= XlEnableCancelKey]
- Application.EnableEvents [= setting]
- Application.EnableSound [= setting]
- Application.ErrorCheckingOptions
- [Application.]Evaluate(Name)
- Application.ExtendList [= setting]
- Application.FeatureInstall [= MsoFeatureInstall]
- Application.FileConverters[(Index1, Index2)]
- Application.FileDialog (MsoFileDialogType)
- Application.FileFind
- Application.FileSearch
- Application.FindFile( )
- Application.FindFormat
- Application.FixedDecimal [= setting]
- Application.FixedDecimalPlaces [= setting]
- Application.GenerateGetPivotData [= setting]
- Application.GetCustomListContents
- Application.GetCustomListNum(ListArray)
- Application.GetOpenFilename([FileFilter], [FilterIndex], [Title], [ButtonText], [MultiSelect])
- Application.GetPhonetic([Text])
- Application.GetSaveAsFilename([InitialFilename], [FileFilter], [FilterIndex], [Title], [ButtonText])
- Application.Goto([Reference], [Scroll])
- Application.Height
- Application.Help([HelpFile], [HelpContextID])
- Application.Hinstance
- Application.Hwnd
- Application.InchesToPoints(Inches)
- [Application.]InputBox(Prompt, [Title], [Default], [Left], [Top], [HelpFile], [HelpContextID], [Type])
- Application.Interactive [= setting]
- Application.International(XlApplicationInternational)
- [Application.]Intersect(Arg1, Arg2, [Argn], ...)
- Application.Iteration [= setting]
- Application.LanguageSettings
- Application.LargeButtons [= setting]
- Application.Left [= setting]
- Application.LibraryPath
- Application.MacroOptions([Macro], [Description], [HasMenu], [MenuText], [HasShortcutKey], [ShortcutKey], [Category], [StatusBar], [HelpContextId], [HelpFile])
- Application.MailLogoff( )
- Application.MailLogon([Name], [Password], [DownloadNewMail])
- Application.MailSession
- Application.MailSystem
- Application.MapPaperSize [= setting]
- Application.MaxChange [= setting]
- Application.MaxIterations [= setting]
- Application.MoveAfterReturn [= setting]
- Application.MoveAfterReturnDirection [=XlDirection]
- Application.Names([index])
- Application.NetworkTemplatesPath
- Application.NewWorkbook
- Application.NextLetter( )
- Application.ODBCErrors
- Application.ODBCTimeout [= setting]
- Application.OLEDBErrors
- Application.OnKey(Key, [Procedure])
- Application.OnRepeat(Text, Procedure)
- Application.OnTime(EarliestTime, Procedure, [LatestTime], [Schedule])
- Application.OnUndo(Text, Procedure)
- Application.OnWindow [= setting]
- Application.OperatingSystem
- Application.OrganizationName
- Application.Path
- Application.PathSeparator
- Application.PivotTableSelection [= setting]
- Application.PreviousSelections([index])
- Application.ProductCode
- Application.PromptForSummaryInfo [= setting]
- Application.Quit( )
- [Application.]Range([cell1],[cell2])
- Application.Ready
- Application.RecentFiles([index])
- Application.RecordMacro([BasicCode], [XlmCode])
- Application.RecordRelative [= setting]
- Application.ReferenceStyle [=XlReferenceStyle]
- Application.RegisteredFunctions
- Application.RegisterXLL(Filename)
- Application.Repeat( )
- Application.ReplaceFormat [= setting]
- Application.RollZoom [= setting]
- [Application.]Rows([index])
- Application.RTD
- Application.Run([Macro], [Args])
- Application.SaveWorkspace([Filename])
- Application.ScreenUpdating [= setting]
- Application.Selection
- Application.SendKeys(Keys, [Wait])
- Application.SetDefaultChart([FormatName], [Gallery])
- [Application.]Sheets([index])
- Application.SheetsInNewWorkbook [= setting]
- Application.ShowChartTipNames [= setting]
- Application.ShowChartTipValues [= setting]
- Application.ShowStartupDialog [= setting]
- Application.ShowToolTips [= setting]
- Application.ShowWindowsInTaskbar [= setting]
- Application.SmartTagRecognizers
- Application.Speech
- Application.SpellingOptions
- Application.StandardFont [= setting]
- Application.StandardFontSize [= setting]
- Application.StartupPath
- Application.StatusBar [= setting]
- Application.TemplatesPath
- Application.ThisCell
- Application.ThisWorkbook
- Application.ThousandsSeparator [= setting]
- Application.Top [= setting]
- Application.Undo( )
- [Application.]Union(Arg1, Arg2, [Argn])
- Application.UsableHeight
- Application.UsableWidth
- Application.UsedObjects
- Application.UserControl
- Application.UserLibraryPath
- Application.UserName [= setting]
- Application.UseSystemSeparators [= setting]
- Application.VBE
- Application.Version
- Application.Visible [= setting]
- Application.Volatile([Volatile])
- Application.Wait(Time)
- Application.Watches([index])
- Application.Width [= setting]
- Application.Windows([index])
- Application.WindowsForPens
- Application.WindowState [= XlWindowState]
- [Application.]Workbooks([index])
- [Application.]WorksheetFunction
- [Application.]Worksheets([index])
- 7.5. AutoCorrect Members
- AutoCorrect.AddReplacement(What, Replacement)
- AutoCorrect.DeleteReplacement(What)
- AutoCorrect.ReplacementList
- 7.6. AutoRecover Members
- AutoRecover.Enabled [ = setting ]
- AutoRecover.Path [ = setting ]
- AutoRecover.Time [ = setting ]
- 7.7. ErrorChecking Members
- 7.8. SpellingOptions Members
- 7.9. Window and Windows Members
- window.Activate( )
- window.ActivateNext( )
- window.ActivatePrevious( )
- windows.Arrange([ArrangeStyle], [ActiveWorkbook], [SyncHorizontal], [SyncVertical])
- windows.BreakSideBySide( )
- window.Close([SaveChanges], [Filename], [RouteWorkbook])
- windows.CompareSideBySideWith(WindowName)
- window.DisplayFormulas [= setting]
- window.DisplayGridlines [= setting]
- window.DisplayHeadings [= setting]
- window.DisplayHorizontalScrollBar [= setting]
- window.DisplayOutline [= setting]
- window.DisplayRightToLeft [= setting]
- window.DisplayVerticalScrollBar [= setting]
- window.DisplayWorkbookTabs [= setting]
- window.DisplayZeros [= setting]
- window.EnableResize [= setting]
- window.FreezePanes [= setting]
- window.GridlineColor [= setting]
- window.GridlineColorIndex [=xlColorIndexAutomatic]
- window.LargeScroll([Down], [Up], [ToRight], [ToLeft])
- window.Panes
- window.PointsToScreenPixelsX(Points)
- window.PointsToScreenPixelsY(Points)
- window.RangeFromPoint(x, y)
- window.RangeSelection
- windows.ResetPositionsSideBySide( )
- window.ScrollColumn [= setting]
- window.ScrollIntoView(Left, Top, Width, Height, [Start])
- window.ScrollRow [= setting]
- window.ScrollWorkbookTabs([Sheets], [Position])
- window.SelectedSheets
- window.Selection
- window.SmallScroll([Down], [Up], [ToRight], [ToLeft])
- window.Split [= setting]
- window.SplitColumn [= setting]
- window.SplitHorizontal [= setting]
- window.SplitRow [= setting]
- window.SplitVertical [= setting]
- windows.SyncScrollingSideBySide [= setting]
- window.TabRatio [= setting]
- window.View [= XlWindowView]
- window.VisibleRange
- window.WindowNumber [= setting]
- window.WindowState [= XlWindowState]
- window.Zoom [= setting]
- 7.10. Pane and Panes Members
- 7.1. Perform Tasks
- 8. Opening, Saving, and Sharing Workbooks
- 8.1. Add, Open, Save, and Close
- 8.1.1. Templates
- 8.1.2. Open as Read-Only or with Passwords
- 8.1.3. Open Text Files
- 8.1.4. Open XML Files
- 8.1.5. Close Workbooks
- 8.2. Share Workbooks
- 8.3. Program with Shared Workbooks
- 8.4. Program with Shared Workspaces
- 8.4.1. Open Workbooks from a Shared Workspace
- 8.4.2. Link a Workbook to a Workspace
- 8.4.3. Remove Sharing
- 8.5. Respond to Actions
- 8.6. Workbook and Workbooks Members
- workbook.AcceptAllChanges([When], [Who], [Where])
- workbook.AcceptLabelsInFormulas [= setting]
- workbook.Activate( )
- workbook.ActiveChart
- workbook.ActiveSheet
- workbooks.Add([Template])
- workbook.AddToFavorites
- workbook.Author [= setting]
- workbook.AutoUpdateFrequency [= setting]
- workbook.AutoUpdateSaveChanges [= setting]
- workbook.BreakLink(Name, Type)
- workbook.BuiltinDocumentProperties
- workbook.CalculationVersion
- workbook.CanCheckIn
- workbooks.CanCheckOut(Filename)
- workbook.ChangeFileAccess(Mode, [WritePassword], [Notify])
- workbook.ChangeHistoryDuration [= setting]
- workbook.ChangeLink(Name, NewName, [Type])
- workbook.Charts
- workbook.CheckIn([SaveChanges], [Comments], [MakePublic])
- workbooks.CheckOut(Filename)
- workbook.Close([SaveChanges], [Filename], [RouteWorkbook])
- workbook.CodeName
- workbook.Colors [= setting]
- workbook.CommandBars
- workbook.Comments [= setting]
- workbook.ConflictResolution [= setting]
- workbook.Container
- workbook.CreateBackup [= setting]
- workbook.CustomDocumentProperties
- workbook.CustomViews
- workbook.DeleteNumberFormat(NumberFormat)
- workbook.DisplayDrawingObjects [= setting]
- workbook.DisplayInkComments [= setting]
- workbook.DocumentLibraryVersions
- workbook.EnableAutoRecover [= setting]
- workbook.EndReview
- workbook.EnvelopeVisible [= setting]
- workbook.ExclusiveAccess
- workbook.FileFormat
- workbook.FollowHyperlink(Address, [SubAddress], [NewWindow], [AddHistory], [ExtraInfo], [Method], [HeaderInfo])
- workbook.ForwardMailer( )
- workbook.FullName
- workbook.FullNameURLEncoded
- workbook.HasMailer
- workbook.HasPassword
- workbook.HasRoutingSlip [= setting]
- workbook.HighlightChangesOnScreen [= setting]
- workbook.HighlightChangesOptions([When], [Who], [Where])
- workbook.HTMLProject
- workbook.InactiveListBorderVisible [= setting]
- workbook.IsAddin [= setting]
- workbook.IsInplace
- workbook.KeepChangeHistory [= setting]
- workbook.Keywords [= setting]
- workbook.LinkInfo(Name, LinkInfo, [Type], [EditionRef])
- workbook.LinkSources([Type])
- workbook.ListChangesOnNewSheet [= setting]
- workbook.Mailer
- workbook.MergeWorkbook(Filename)
- workbook.MultiUserEditing
- workbook.Names
- workbook.NewWindow
- workbooks.Open(Filename, [UpdateLinks], [ReadOnly], [Format], [Password], [WriteResPassword], [IgnoreReadOnlyRecommended], [Origin], [Delimiter], [Editable], [Notify], [Converter], [AddToMru], [Local], [CorruptLoad])
- workbooks.OpenDatabase(Filename, [CommandText], [CommandType], [BackgroundQuery], [ImportDataAs])
- workbook.OpenLinks(Name, [ReadOnly], [Type])
- workbooks.OpenText(Filename, [Origin], [StartRow], [DataType], [TextQualifier], [ConsecutiveDelimiter], [Tab], [Semicolon], [Comma], [Space], [Other], [OtherChar], [FieldInfo], [TextVisualLayout], [DecimalSeparator], [ThousandsSeparator], [TrailingMinusNumbers], [Local])
- workbooks.OpenXML(Filename, [Stylesheets], [LoadOption])
- workbook.Path
- workbook.PersonalViewListSettings [= setting]
- workbook.PersonalViewPrintSettings [= setting]
- workbook.PivotCaches
- workbook.PivotTableWizard([SourceType], [SourceData], [TableDestination], [TableName], [RowGrand], [ColumnGrand], [SaveData], [HasAutoFormat], [AutoPage], [Reserved], [BackgroundQuery], [OptimizeCache], [PageFieldOrder], [PageFieldWrapCount], [ReadData], [Connection])
- workbook.Post([DestName])
- workbook.PrecisionAsDisplayed [= setting]
- workbook.PrintOut([From], [To], [Copies], [Preview], [ActivePrinter], [PrintToFile], [Collate], [PrToFileName])
- workbook.PrintPreview([EnableChanges])
- workbook.PublishObjects
- workbook.PurgeChangeHistoryNow(Days, [SharingPassword])
- workbook.ReadOnly
- workbook.ReadOnlyRecommended
- workbook.RecheckSmartTags
- workbook.RefreshAll
- workbook.RejectAllChanges([When], [Who], [Where])
- workbook.ReloadAs(Encoding)
- workbook.RemovePersonalInformation [= setting]
- workbook.RemoveUser(Index)
- workbook.Reply
- workbook.ReplyAll
- workbook.ReplyWithChanges([ShowMessage])
- workbook.ResetColors
- workbook.RevisionNumber
- workbook.Route
- workbook.Routed
- workbook.RoutingSlip
- workbook.RunAutoMacros(Which)
- workbook.Save
- workbook.SaveAs([Filename], [FileFormat], [Password], [WriteResPassword], [ReadOnlyRecommended], [CreateBackup], [AccessMode], [ConflictResolution], [AddToMru], [TextCodepage], [TextVisualLayout], [Local])
- workbook.SaveAsXMLData(Filename, Map)
- workbook.SaveCopyAs([Filename])
- workbook.Saved [= setting]
- workbook.SaveLinkValues [= setting]
- workbook.SendFaxOverInternet([Recipients], [Subject], [ShowMessage])
- workbook.SendForReview([Recipients], [Subject], [ShowMessage], [IncludeAttachment])
- workbook.SendMail(Recipients, [Subject], [ReturnReceipt])
- workbook.SendMailer([FileFormat], [Priority])
- workbook.SetLinkOnData(Name, [Procedure])
- workbook.SharedWorkspace
- workbook.Sheets
- workbook.ShowConflictHistory [= setting]
- workbook.ShowPivotTableFieldList [= setting]
- workbook.SmartDocument
- workbook.SmartTagOptions
- workbook.Styles [= setting]
- workbook.Subject [= setting]
- workbook.TemplateRemoveExtData [= setting]
- workbook.Title [= setting]
- workbook.ToggleFormsDesign [= setting]
- workbook.UpdateFromFile
- workbook.UpdateLink([Name], [Type])
- workbook.UpdateLinks [= setting]
- workbook.UpdateRemoteReferences [= setting]
- workbook.UserStatus
- workbook.VBASigned
- workbook.VBProject
- workbook.WebOptions
- workbook.WebPagePreview
- workbook.Windows [= setting]
- workbook.Worksheets
- workbook.XmlImport(Url, ImportMap, [Overwrite], [Destination])
- workbook.XmlImportXml(Data, ImportMap, [Overwrite], [Destination])
- workbook.XmlMaps
- workbook.XmlNamespaces
- 8.7. RecentFile and RecentFiles Members
- recentfiles.Add(Name)
- recentfile.Delete( )
- recentfiles.Maximum [= setting]
- recentfile.Name
- recentfile.Open
- recentfile.Path
- 8.1. Add, Open, Save, and Close
- 9. Working with Worksheets and Ranges
- 9.1. Work with Worksheet Objects
- 9.1.1. Get Cells in a Worksheet
- 9.2. Worksheets and Worksheet Members
- worksheet.Activate( )
- worksheets.Add(Before, After, Count, Type)
- worksheet.Calculate( )
- worksheet.Cells
- worksheet.CheckSpelling(CustomDictionary, IgnoreUppercase, AlwaysSuggest, SpellLang)
- worksheet.Columns([Index])
- worksheet.Comments
- worksheet.Copy(Before, After)
- worksheet.DisplayPageBreaks
- worksheet.EnableCalculation [= setting]
- worksheet.EnableOutlining [= setting]
- worksheet.EnablePivotTable [= setting]
- worksheet.EnableSelection [= setting]
- worksheet.Hyperlinks
- worksheet.Move(Before, After)
- worksheet.Outline
- worksheet.PageSetup
- worksheet.Paste([Destination], [Link])
- worksheet.PasteSpecial([Format], [Link], [DisplayAsIcon], [IconFileName], [IconIndex], [IconLabel], [NoHTMLFormatting])
- worksheet.Protect([Password], [DrawingObjects], [Contents], [Scenarios], [UserInterfaceOnly], [AllowFormattingCells], [AllowFormattingColumns], [AllowFormattingRows], [ AllowInsertingColumns], [ AllowInsertingRows], [AllowInsertingHyperlinks], [ AllowDeletingColumns], [ AllowDeletingRows], [AllowSorting], [AllowFiltering], [AllowUsingPivotTables])
- worksheet.ProtectContents
- worksheet.ProtectDrawingObjects
- worksheet.Protection
- worksheet.ProtectionMode
- worksheet.ProtectScenarios
- worksheet.QueryTables
- worksheet.Range([Cell1], [Cell2])
- worksheet.Rows([Index])
- worksheet.Scenarios([Index])
- worksheet.ScrollArea
- worksheet.SetBackgroundPicture([Filename])
- worksheet.Shapes
- worksheet.StandardHeight
- worksheet.StandardWidth
- worksheet.Type [= setting]
- worksheet.Unprotect([Password])
- worksheet.UsedRange
- 9.3. Sheets Members
- Sheets.Copy(Before, After)
- Sheets.FillAcrossSheets(Range, Type)
- Sheets.Move(Before, After)
- 9.4. Work with Outlines
- 9.5. Outline Members
- outline.AutomaticStyles
- outline.ShowLevels(RowLevels, ColumnLevels)
- outline.SummaryColumn
- outline.SummaryRow
- 9.6. Work with Ranges
- 9.6.1. Find and Replace Text in a Range
- 9.6.2. Use Named Ranges
- 9.6.3. Format and Change Text
- 9.7. Range Members
- range.Activate( )
- range.AddComment( )
- range.AddIndent[= setting]
- range.Address([RowAbsolute], [ColumnAbsolute], [ReferenceStyle], [External], [RelativeTo])
- range.AllowEdit
- range.Areas([Index])
- range.AutoFill(Destination, [Type])
- range.AutoFit
- range.BorderAround([LineStyle], [Weight], [ColorIndex], [Color])
- range.Borders([Index])
- range.Calculate( )
- range.Cells([RowIndex], [ColumnIndex])
- range.Characters([Start], [Length])
- range.CheckSpelling([CustomDictionary], [IgnoreUppercase], [AlwaysSuggest], [SpellLang])
- range.Clear( )
- range.ClearContents( )
- range.ClearFormats( )
- range.Column
- range.Columns([Index])
- range.ColumnWidth
- range.Copy([Destination])
- range.CopyFromRecordset([Data, MaxRows, MaxColumns])
- range.Cut([Destination])
- range.Delete([Shift])
- range.Dependents
- range.DirectDependents
- range.DirectPrecedents
- range.End([Direction])
- range.EntireColumn
- range.EntireRow
- range.FillDown
- range.FillLeft
- range.FillRight
- range.FillUp
- range.Find(What, [After], [LookIn], [LookAt]), [SearchOrder], [SearchDirection], [MatchCase], [MatchByte], [SearchFormat])
- range.FindNext([After])
- range.FindPrevious([After])
- range.Font
- range.Formula
- range.FormulaR1C1
- range.Hidden
- range.HorizontalAlignment
- range.Hyperlinks
- range.Insert([Shift])
- range.Interior
- range.Item(RowIndex, [ColumnIndex])
- range.Justify
- range.Locked
- range.Merge([Across])
- range.MergeArea
- range.MergeCells
- range.Next
- range.NoteText([Text], [Start], [Length])
- range.NumberFormat
- range.NumberFormatLocal
- range.Offset([RowOffset], [ColumnOffset])
- range.PageBreak
- range.PasteSpecial([Paste], [Operation], [SkipBlanks], [Transpose])
- range.Precedents
- range.Previous
- range.PrintOut([From], [To], [Copies], [Preview], [ActivePrinter], [PrintToFile], [Collate], [PrToFileName])
- range.PrintPreview
- range.Replace(What, Replacement, [LookAt]), [SearchOrder], [MatchCase], [MatchByte], [SearchFormat], [ReplaceFormat])
- range.Resize([RowSize]), [ColumnSize])
- range.Row
- range.RowDifferences(Comparison)
- range.RowHeight
- range.Rows([Index])
- range.Select
- range.Show
- range.ShowDependents([Remove])
- range.ShowDetail [= setting]
- range.ShowErrors( )
- range.ShowPrecedents([Remove])
- range.ShrinkToFit [= setting]
- range.Sort([Key1]), [Order1], [Key2], [Type], [Order2], [Key3], [Order3], [Header], [OrderCustom], [MatchCase], [Orientation], [SortMethod], [DataOption1], [DataOption2], [DataOption3])
- range.SpecialCells(Type, [Value])
- range.Style
- range.Table([RowInput], [ColumnInput])
- range.Text
- range.TextToColumns([Destination]), [DataType], [TextQualifier], [ConsecutiveDelimiter], [Tab], [Semicolon], [Comma], [Space], [Other], [OtherChar], [FieldInfo], [DecimalSeparator], [ThousandsSeparator], [TrailingMinusNumbers])
- range.UnMerge
- range.UseStandardHeight [= setting]
- range.UseStandardWidth [= setting]
- range.Value([RangeValueDataType]) [= setting]
- range.VerticalAlignment
- range.Worksheet
- range.WrapText[= setting]
- 9.8. Work with Scenario Objects
- 9.9. Scenario and Scenarios Members
- scenario.ChangeScenario(ChangingCells, [Values])
- scenario.ChangingCells
- scenario.Comment [= setting]
- scenario.Hidden [= setting]
- scenario.Locked [= setting]
- scenario.Show
- scenario.Values
- 9.10. Resources
- 9.1. Work with Worksheet Objects
- 10. Linking and Embedding
- 10.1. Add Comments
- 10.2. Use Hyperlinks
- 10.3. Link and Embed Objects
- 10.3.1. Embed Controls
- 10.3.2. Use OleObjects in Code
- 10.4. Speak
- 10.5. Comment and Comments Members
- comment.Author
- comment.Delete
- comment.Shape
- comment.Text([Text], [Start], [Overwrite])
- 10.6. Hyperlink and Hyperlinks Members
- hyperlinks.Add(Anchor, Address, [SubAddress], [ScreenTip], [TextToDisplay])
- hyperlink.Address [= setting]
- hyperlink.AddToFavorites( )
- hyperlink.CreateNewDocument(Filename, EditNow, Overwrite)
- hyperlink.EmailSubject [= setting]
- hyperlink.Follow([NewWindow], [AddHistory], [ExtraInfo], [Method], [HeaderInfo])
- hyperlink.Range
- hyperlink.ScreenTip [= setting]
- hyperlink.Shape
- hyperlink.SubAddress [= setting]
- hyperlink.TextToDisplay [= setting]
- hyperlink.Type
- 10.7. OleObject and OleObjects Members
- oleobject.Add([ClassType], [Filename], [Link], [DisplayAsIcon], [IconFileName], [IconIndex], [IconLabel], [Left], [Top], [Width], [Height])
- oleobject.AutoLoad [= setting]
- oleobject.AutoUpdate [= setting]
- oleobject.BottomRightCell
- oleobject.BringToFront( )
- oleobject.Copy( )
- oleobject.CopyPicture([Appearance], [Format])
- oleobject.Duplicate( )
- oleobjects.Group( )
- oleobject.LinkedCell
- oleobject.ListFillRange [= setting]
- oleObject.Object
- oleobject.OLEType
- oleobject.OnAction [= setting]
- oleobject.Placement [= xlPlacement]
- oleobject.progID [= setting]
- oleobject.Shadow [= setting]
- oleobject.ShapeRange
- oleobject.SourceName
- oleobject.Update( )
- oleobject.Verb([Verb])
- oleobject.ZOrder
- 10.8. OLEFormat Members
- 10.9. Speech Members
- speech.Direction [= XlSpeakDirection]
- speech.Speak(Text, [SpeakAsync], [SpeakXML], [Purge])
- speech.SpeakCellOnEnter [= setting]
- 10.10. UsedObjects Members
- 11. Printing and Publishing
- 11.1. Print and Preview
- 11.2. Control Paging
- 11.3. Change Printer Settings
- 11.4. Filter Ranges
- 11.5. Save and Display Views
- 11.6. Publish to the Web
- 11.7. AutoFilter Members
- autofilter.Filters(index)
- 11.8. Filter and Filters Members
- filter.Criteria1
- filter.Criteria2
- filter.On
- filter.Operator
- 11.9. CustomView and CustomViews Members
- customviews.Add(ViewName, [PrintSettings], [RowColSettings])
- customview.PrintSettings
- customview.RowColSettings
- customview.Show
- 11.10. HPageBreak, HPageBreaks, VPageBreak, VPageBreaks Members
- pagebreaks.Add(Before)
- pagebreak.DragOff(Direction, RegionIndex)
- pagebreak.Extent
- pagebreak.Location
- pagebreak.Type
- 11.11. PageSetup Members
- pagesetup.BlackAndWhite [= setting]
- pagesetup.BottomMargin [= setting]
- pagesetup.CenterFooter [= setting]
- pagesetup.CenterFooterPicture
- pagesetup.CenterHeader [= setting]
- pagesetup.CenterHeaderPicture
- pagesetup.CenterHorizontally [= setting]
- pagesetup.CenterVertically [= setting]
- pagesetup.ChartSize [= setting]
- pagesetup.Draft [= setting]
- pagesetup.FirstPageNumber [= setting]
- pagesetup.FitToPagesTall [= setting]
- pagesetup.FitToPagesWide [= setting]
- pagesetup.FooterMargin [= setting]
- pagesetup.HeaderMargin [= setting]
- pagesetup.LeftFooter [= setting]
- pagesetup.LeftFooterPicture
- pagesetup.LeftHeader [= setting]
- pagesetup.LeftHeaderPicture
- pagesetup.LeftMargin [= setting]
- pagesetup.Order [= setting]
- pagesetup.Orientation [= setting]
- pagesetup.PaperSize [= setting]
- pagesetup.PrintArea [= setting]
- pagesetup.PrintComments [= setting]
- pagesetup.PrintErrors [= setting]
- pagesetup.PrintGridlines [= setting]
- pagesetup.PrintHeadings [= setting]
- pagesetup.PrintNotes [= setting]
- pagesetup.PrintQuality(index) [= setting]
- pagesetup.PrintTitleColumns [= setting]
- pagesetup.PrintTitleRows [= setting]
- pagesetup.RightFooter [= setting]
- pagesetup.RightFooterPicture
- pagesetup.RightHeader [= setting]
- pagesetup.RightHeaderPicture
- pagesetup.RightMargin [= setting]
- pagesetup.TopMargin [= setting]
- pagesetup.Zoom [= setting]
- 11.12. Graphic Members
- graphic.Filename [= setting]
- graphic.LockAspectRatio [= setting]
- 11.13. PublishObject and PublishObjects Members
- publishobjects.Add(SourceType, Filename, [Sheet], [Source], [HtmlType], [DivID], [Title])
- publishobject.AutoRepublish [= setting]
- publishobject.DivID
- publishobject.Filename [= setting]
- publishobject.HtmlType [= setting]
- publishobjects.Publish([Create])
- publishobject.Sheet
- publishobject.Source
- publishobject.SourceType
- publishobject.Title [= setting]
- 11.14. WebOptions and DefaultWebOptions Members
- options.AllowPNG [= setting]
- defaultweboptions.AlwaysSaveInDefaultEncoding [= setting]
- defaultweboptions.CheckIfOfficeIsHTMLEditor [= setting]
- options.DownloadComponents [= setting]
- options.Encoding [= msoEncoding]
- options.FolderSuffix
- defaultweboptions.Fonts
- defaultweboptions.LoadPictures [= setting]
- options.LocationOfComponents [= setting]
- options.OrganizeInFolder [= setting]
- options.RelyOnCSS [= setting]
- options.RelyOnVML [= setting]
- defaultweboptions.SaveHiddenData [= setting]
- defaultweboptions.SaveNewWebPagesAsWebArchives [= setting]
- options.ScreenSize [= msoScreenSize]
- options.TargetBrowser [= msoTargetBrowser]
- defaultweboptions.UpdateLinksOnSave [= setting]
- options.UseLongFileNames [= setting]
- 12. Loading and Manipulating Data
- 12.1. Working with QueryTable Objects
- 12.2. QueryTable and QueryTables Members
- querytables.Add(Connection, Destination, [Sql])
- querytable.AdjustColumnWidth [= setting]
- querytable.BackgroundQuery[= setting]
- querytable.CancelRefresh
- querytable.CommandText[= setting]
- querytable.CommandType[= setting]
- querytable.Connection[= setting]
- querytable.Delete
- querytable.Destination
- querytable.EnableEditing[= setting]
- querytable.EnableRefresh[= setting]
- querytable.FetchedRowOverflow[= setting]
- querytable.FieldNames[= setting]
- querytable.FillAdjacentFormulas[= setting]
- querytable.MaintainConnection[= setting]
- querytable.Parameters
- querytable.PreserveColumnInfo[= setting]
- querytable.PreserveFormatting[= setting]
- querytable.QueryType[= setting]
- querytable.Recordset[= setting]
- querytable.Refresh([BackgroundQuery])
- querytable.Refreshing
- querytable.RefreshOnFileOpen[= setting]
- querytable.RefreshPeriod[= setting]
- querytable.RefreshStyle[= setting]
- querytable.ResetTimer
- querytable.ResultRange
- querytable.RowNumbers[= setting]
- querytable.SavePassword[= setting]
- querytable.TextFileColumnDataTypes[= setting]
- querytable.TextFileCommaDelimiter[= setting]
- querytable.TextFileConsecutiveDelimiter[= setting]
- querytable.TextFileDecimalSeparator[= setting]
- querytable.TextFileFixedColumnWidths[= setting]
- querytable.TextFileOtherDelimiter[= setting]
- querytable.TextFileParseType[= setting]
- querytable.TextFilePlatform[= setting]
- querytable.TextFilePromptOnRefresh[= setting]
- querytable.TextFileSemicolonDelimiter[= setting]
- querytable.TextFileSpaceDelimiter[= setting]
- querytable.TextFileTabDelimiter[= setting]
- querytable.TextFileTextQualifier[= setting]
- querytable.TextFileThousandsSeparator[= setting]
- querytable.TextFileTrailingMinusNumbers[= setting]
- querytable.TextFileVisualLayout[= setting]
- 12.3. Working with Parameter Objects
- 12.4. Parameter Members
- parameters.Add(Name, [iDataType])
- parameter.DataType [= setting]
- parameter.Delete
- parameter.PromptString[= setting]
- parameter.RefreshOnChange[= setting]
- parameter.SetParam[= setting]
- parameter.SourceRange[= setting]
- parameter.Type[= setting]
- parameter.Value[= setting]
- 12.5. Working with ADO and DAO
- 12.6. ADO Objects and Members
- 12.6.1. ADO.Command Members
- command.ActiveConnection[= setting]
- command.CommandText[= setting]
- command.CommandType[= setting]
- command.CreateParameter
- command.Execute
- command.Name[= setting]
- 12.6.2. ADO.Connection Members
- connection.BeginTrans
- connection.Cancel
- connection.CommandTimeout[= setting]
- connection.CommitTrans
- connection.ConnectionString[= setting]
- connection.ConnectionTimeout[= setting]
- connection.Open
- connection.RollbackTrans
- connection.Version[= setting]
- 12.6.3. ADO.Field and ADO.Fields Members
- field.ActualSize[= setting]
- field.AppendChunk
- fields.CancelUpdate
- field.DefinedSize[= setting]
- field.GetChunk(Size)
- field.NumericScale[= setting]
- field.OriginalValue[= setting]
- field.UnderlyingValue[= setting]
- field.Value[= setting]
- 12.6.4. ADO.Parameter and ADO.Parameters Members
- Parameter.AppendChunk
- Parameter.Name[= setting]
- Parameter.NumericScale[= setting]
- Parameter.Precision[= setting]
- parameter.Size[= setting]
- parameter.Value[= setting]
- 12.6.5. ADO.Record Members
- record.ActiveConnection[= setting]
- record.Cancel
- record.GetChildren
- record.Open([Source], [ActiveConnection], [Mode]), [CreateOptions], [Options], [UserName], [Password])
- record.RecordType
- record.Source[= setting]
- record.State
- 12.6.6. ADO.Recordset Members
- recordset.AbsolutePosition[= setting]
- recordset.ActiveCommand[= setting]
- recordset.ActiveConnection [= setting]
- recordset.AddNew([FieldList], [Values])
- recordset.BOF[= setting]
- recordset.Cancel
- recordset.CancelUpdate
- recordset.Delete([AffectRecords])
- recordset.EOF[= setting]
- recordset.Filter[= setting]
- recordset.MoveFirst
- recordset.MoveLast
- recordset.MoveNext
- recordset.MovePrevious
- recordset.Open([Source], [ActiveConnection], [CursorType] , [LockType] , [Options])
- recordset.RecordCount[= setting]
- recordset.Requery
- recordset.Source[= setting]
- recordset.Update([Fields], [Value])
- 12.6.1. ADO.Command Members
- 12.7. DAO Objects and Members
- 12.8. DAO.Database and DAO.Databases Members
- Reference Section
- database.Connection
- Reference Section
- database.Execute(Source, [Options])
- Reference Section
- database.OpenRecordset(Source, [Type], [Options]), [LockEdits])
- 12.8.1. DAO.DbEngine Members
- dbengine.CompactDatabase(olddb, newdb, [locale]), [options] , [password])
- dbengine.OpenDatabase(dbname, [options], [read-only], [connect])
- Reference Section
- 12.9. DAO.Document and DAO.Documents Members
- Document.Container
- Document.Name
- 12.10. DAO.QueryDef and DAO.QueryDefs Members
- querydef.Execute([Options])
- querydef.MaxRecords[= setting]
- querydef.OpenRecordset([Type], [Options]), [LockEdits])
- querydef.SQL[= setting]
- 12.11. DAO.Recordset and DAO.Recordsets Members
- recordset.AddNew
- recordset.BOF[= setting]
- recordset.EOF[= setting]
- recordset.MoveFirst
- recordset.MoveLast
- recordset.MoveNext
- recordset.MovePrevious
- 13. Analyzing Data with Pivot Tables
- 13.1. Quick Guide to Pivot Tables
- 13.1.1. Create a Pivot Table
- 13.1.2. Apply Formatting
- 13.1.3. Change Totals
- 13.1.4. Chart the Data
- 13.1.5. Change the Layout
- 13.1.6. Connect to an External Data Source
- 13.1.7. Create OLAP Cubes
- 13.2. Program Pivot Tables
- 13.2.1. Create Pivot Tables
- 13.2.2. Refresh Pivot Tables and Charts
- 13.2.3. Connect to External Data
- 13.2.4. OLAP Data Cubes
- 13.3. PivotTable and PivotTables Members
- pivottables.Add(PivotCache, TableDestination, [TableName], [ReadData], [DefaultVersion])
- pivottable.AddDataField(Field, [Caption], [Function])
- pivottable.AddFields([RowFields], [ColumnFields], [PageFields], [AddToTable])
- pivottable.CacheIndex [= setting]
- pivottable.CalculatedFields( )
- pivottable.CalculatedMembers
- pivottable.ColumnFields
- pivottable.ColumnGrand [= setting]
- pivottable.ColumnRange
- pivottable.CreateCubeFile(File, [Measures], [Levels], [Members], [Properties])
- pivottable.CubeFields
- pivottable.DataBodyRange
- pivottable.DataFields
- pivottable.DataLabelRange
- pivottable.DataPivotField
- pivottable.DisplayEmptyColumn [= setting]
- pivottable.DisplayEmptyRow [= setting]
- pivottable.DisplayErrorString [= setting]
- pivottable.DisplayImmediateItems [= setting]
- pivottable.DisplayNullString [= setting]
- pivottable.EnableDataValueEditing [= setting]
- pivottable.EnableDrilldown [= setting]
- pivottable.EnableFieldDialog [= setting]
- pivottable.EnableFieldList [= setting]
- pivottable.EnableWizard [= setting]
- pivottable.ErrorString [= setting]
- pivottable.Format(Format)
- pivottable.GetData(Name)
- pivottable.GetPivotData([DataField], [Field1], [Item1], [Fieldn], [Itemn])
- pivottable.GrandTotalName [= setting]
- pivottable.HasAutoFormat [= setting]
- pivottable.HiddenFields
- pivottable.InnerDetail [= setting]
- pivottable.ListFormulas( )
- pivottable.ManualUpdate [= setting]
- pivottable.MDX
- pivottable.MergeLabels [= setting]
- pivottable.NullString [= setting]
- pivottable.PageFieldOrder [= xlOrder]
- pivottable.PageFields
- pivottable.PageFieldWrapCount [= setting]
- pivottable.PageRange
- pivottable.PageRangeCells
- pivottable.PivotCache( )
- pivottable.PivotFields
- pivottable.PivotFormulas
- pivottable.PivotSelect(Name, [Mode], [UseStandardName])
- pivottable.PivotSelection [= setting]
- pivottable.PivotSelectionStandard [= setting]
- pivottable.PivotTableWizard([SourceType], [SourceData], [TableDestination], [TableName], [RowGrand], [ColumnGrand], [SaveData], [HasAutoFormat], [AutoPage], [Reserved], [BackgroundQuery], [OptimizeCache], [PageFieldOrder], [PageFieldWrapCount], [ReadData], [Connection])
- pivottable.PreserveFormatting [= setting]
- pivottable.PrintTitles [= setting]
- pivottable.RefreshDate
- pivottable.RefreshName
- pivottable.RefreshTable( )
- pivottable.RepeatItemsOnEachPrintedPage [= setting]
- pivottable.RowFields
- pivottable.RowGrand [= setting]
- pivottable.RowRange
- pivottable.SaveData [= setting]
- pivottable.SelectionMode [= xlPTSelectionMode]
- pivottable.ShowCellBackgroundFromOLAP [= setting]
- pivottable.ShowPageMultipleItemLabel [= setting]
- pivottable.ShowPages([PageField])
- pivottable.SourceData
- pivottable.SubtotalHiddenPageItems [= setting]
- pivottable.TableRange1
- pivottable.TableRange2
- pivottable.TableStyle [= setting]
- pivottable.TotalsAnnotation [= setting]
- pivottable.Update( )
- pivottable.VacatedStyle [= setting]
- pivottable.Value [= setting]
- pivottable.Version
- pivottable.ViewCalculatedMembers [= setting]
- pivottable.VisibleFields
- pivottable.VisualTotals [= setting]
- 13.4. PivotCache and PivotCaches Members
- pivotcaches.Add(SourceType, [SourceData])
- pivotcache.ADOConnection
- pivotcache.BackgroundQuery [= setting]
- pivotcache.CommandText [= setting]
- pivotcache.CommandType [= xlCmdType]
- pivotcache.Connection [= setting]
- pivotcache.CreatePivotTable(TableDestination, [TableName], [ReadData], [DefaultVersion])
- pivotcache.EnableRefresh [= setting]
- pivotcache.IsConnected
- pivotcache.LocalConnection [= setting]
- pivotcache.MaintainConnection [= setting]
- pivotcache.MakeConnection( )
- pivotcache.MemoryUsed
- pivotcache.MissingItemsLimit [= setting]
- pivotcache.OLAP
- pivotcache.OptimizeCache [= setting]
- pivotcache.QueryType
- pivotcache.RecordCount
- pivotcache.Recordset [= setting]
- pivotcache.Refresh( )
- pivotcache.RefreshOnFileOpen [= setting]
- pivotcache.RefreshPeriod [= setting]
- pivotcache.ResetTimer( )
- pivotcache.RobustConnect [= xlRobustConnect]
- pivotcache.SaveAsODC(ODCFileName, [Description], [Keywords])
- pivotcache.SavePassword [= setting]
- pivotcache.SourceConnectionFile [= setting]
- pivotcache.SourceDataFile
- pivotcache.SourceType
- pivotcache.Sql [= setting]
- pivotcache.UseLocalConnection [= setting]
- 13.5. PivotField and PivotFields Members
- pivotfield.AddPageItem(Item, [ClearList])
- pivotfield.AutoShow(Type, Range, Count, Field)
- pivotfield.AutoShowCount
- pivotfield.AutoShowField
- pivotfield.AutoShowRange
- pivotfield.AutoShowType
- pivotfield.AutoSort(Order, Field)
- pivotfield.AutoSortField
- pivotfield.AutoSortOrder
- pivotfield.BaseField [= setting]
- pivotfield.BaseItem [= setting]
- pivotfield.CalculatedItems( )
- pivotfield.Calculation [= xlPivotFieldCalculation]
- pivotfield.Caption [= setting]
- pivotfield.ChildField
- pivotfield.ChildItems
- pivotfield.CubeField
- pivotfield.CurrentPage [= setting]
- pivotfield.CurrentPageList [= setting]
- pivotfield.CurrentPageName [= setting]
- pivotfield.DatabaseSort [= setting]
- pivotfield.DataRange
- pivotfield.DataType
- pivotfield.Delete( )
- pivotfield.DragToColumn [= setting]
- pivotfield.DragToData [= setting]
- pivotfield.DragToHide [= setting]
- pivotfield.DragToPage [= setting]
- pivotfield.DragToRow [= setting]
- pivotfield.DrilledDown [= setting]
- pivotfield.EnableItemSelection [= setting]
- pivotfield.Formula [= setting]
- pivotfield.Function [= xlConsolidationFunction]
- pivotfield.GroupLevel
- pivotfield.HiddenItems
- pivotfield.HiddenItemsList [= setting]
- pivotfield.IsCalculated
- pivotfield.IsMemberProperty
- pivotfield.LabelRange
- pivotfield.LayoutBlankLine [= setting]
- pivotfield.LayoutForm [= xlLayoutFormType]
- pivotfield.LayoutPageBreak [= setting]
- pivotfield.LayoutSubtotalLocation [= XlSubtototalLocationType]
- pivotfield.NumberFormat [= setting]
- pivotfield.Orientation [= xlPivotFieldOrientation]
- pivotfield.ParentField
- pivotfield.PivotItems([Index])
- pivotfield.Position [= setting]
- pivotfield.PropertyOrder [= setting]
- pivotfield.PropertyParentField
- pivotfield.ServerBased [= setting]
- pivotfield.ShowAllItems [= setting]
- pivotfield.SourceName
- pivotfield.StandardFormula [= setting]
- pivotfield.SubtotalName [= setting]
- pivotfield.Subtotals [= setting]
- pivotfield.TotalLevels
- pivotfield.VisibleItems
- 13.6. CalculatedFields Members
- calculatedfields.Add(Name, Formula, [UseStandardFormula])
- 13.7. CalculatedItems Members
- pivotitem.Add(Name, Formula, [UseStandardFormula])
- 13.8. PivotCell Members
- 13.9. PivotFormula and PivotFormulas Members
- 13.10. PivotItem and PivotItems Members
- 13.11. PivotItemList Members
- 13.12. PivotLayout Members
- 13.13. CubeField and CubeFields Members
- cubefield.AddMemberPropertyField(Property, [PropertyOrder])
- cubefields.AddSet(Name, Caption)
- cubefield.CubeFieldType
- cubefield.EnableMultiplePageItems [= setting]
- cubefield.HasMemberProperties
- cubefield.HiddenLevels [= setting]
- cubefield.ShowInFieldList [= setting]
- cubefield.TreeviewControl
- 13.14. CalculatedMember and CalculatedMembers Members
- calculatedmembers.Add(Name, Formula, [SolveOrder], [Type])
- 13.1. Quick Guide to Pivot Tables
- 14. Sharing Data Using Lists
- 14.1. Use Lists
- 14.1.1. Supported Data Types
- 14.1.2. Resolve Conflicts
- 14.1.3. Authorization and Authentication in Shared Lists
- 14.1.4. Create a List in Code
- 14.1.5. Share a List
- 14.1.6. Insert a Shared List
- 14.1.7. Refresh and Update
- 14.1.8. Unlink, Unlist, and Delete
- 14.2. ListObject and ListObjects Members
- Reference Section
- listobjects.Add([SourceType], [Source], [LinkSource], [XlListObjectHasHeaders], [Destination])
- 14.2.1.
- 14.2.1.1. Create a list from a range
- 14.2.1.2. Insert a shared list
- listobject.DataBodyRange
- listobject.Delete( )
- listobject.Publish(Target, LinkSource)
- listobject.SharePointURL
- listobject.ShowTotals [= setting]
- listobject.Unlink( )
- listobject.Unlist( )
- listobject.UpdateChanges([iConflictType])
- listobject.XMLMap
- Reference Section
- 14.3. ListRow and ListRows Members
- listrows.Add([Position])
- listrow.Delete
- listrow.InvalidData
- listrow.Range
- 14.4. ListColumn and ListColumns Members
- listcolumn.ListDataFormat
- listcolumn.SharePointFormula
- listcolumn.TotalsCalculation [= setting]
- listcolumn.XPath
- 14.5. ListDataFormat Members
- listdataformat.AllowFillIn
- listdataformat.Choices
- listdataformat.DecimalPlaces
- listdataformat.DefaultValue
- listdataformat.IsPercent
- listdataformat.lcid
- listdataformat.MaxCharacters
- listdataformat.MaxNumber
- listdataformat.MinNumber
- listdataformat.ReadOnly
- listdataformat.Required
- listdataformat.Type
- 14.6. Use the Lists Web Service
- 14.6.1. Authentication and Authorization
- 14.6.2. Debugging Tip
- 14.6.3. Add Attachments to a List
- 14.6.4. Retrieve Attachments
- 14.6.5. Delete Attachments
- 14.6.6. Delete a SharePoint List
- 14.6.7. Look Up a List GUID
- 14.6.8. Perform Queries
- 14.7. Lists Web Service Members
- wslists.AddAttachment (listName, listItemID, fileName, attachment)
- wslists.AddList (listName, description, templateID)
- wslists.DeleteAttachment (listName, listItemID, url)
- wslists.DeleteList (listName)
- wslists.GetAttachmentCollection (listName, listItemID)
- wslists.GetList (listName)
- wslists.GetListAndView (listName, viewName)
- wslists.GetListCollection ( )
- wslists.GetListItemChanges (listName, viewFields, since, contains)
- wslists.GetListItems (listName, viewName, query, viewFields, rowLimit, queryOptions)
- wslists.UpdateList (listName, listProperties, newFields, updateFields, deleteFields, listVersion)
- wslists.UpdateListItems (listName, updates)
- 14.8. Resources
- 14.1. Use Lists
- 15. Working with XML
- 15.1. Understand XML
- 15.2. Save Workbooks as XML
- 15.2.1. Data Excel Omits from XML
- 15.2.2. Transform XML Spreadsheets
- 15.2.3. Create XSLT for an XML Spreadsheet
- 15.2.4. Transform in Code
- 15.2.5. Transform from the Command Line
- 15.2.6. Transform with Processing Instructions
- 15.2.7. Transform XML into an XML Spreadsheet
- 15.3. Use XML Maps
- 15.3.1. Limitations of XML Maps
- 15.3.2. Use Schemas with XML Maps
- 15.3.3. Export XML Data Through XML Maps
- 15.3.4. Approaches to Using XML Maps
- 15.3.4.1. Avoid lists of lists
- 15.3.4.2. Avoid denormalized data
- 15.3.4.3. Create an XML schema
- 15.3.4.4. Include all nodes if exporting
- 15.3.4.5. Other things to avoid
- 15.3.5. Respond to XML Events
- 15.4. Program with XML Maps
- 15.4.1. Add or Delete XML Maps
- 15.4.2. Export and Import XML
- 15.4.3. Refresh, Change, or Clear the Data Binding
- 15.4.4. View the Schema
- 15.5. XmlMap and XmlMaps Members
- xmlmaps.Add(Schema, [RootElementName])
- xmlmap.AdjustColumnWidth [= setting]
- xmlmap.AppendOnImport [= setting]
- xmlmap.DataBinding
- xmlmap.Delete
- xmlmap.Export(Url, [Overwrite])
- xmlmap.ExportXml(Data)
- xmlmap.Import(Url, [Overwrite])
- xmlmap.ImportXml(Data, [Overwrite])
- xmlmap.IsExportable
- xmlmap.PreserveColumnFilter [= setting]
- xmlmap.PreserveNumberFormatting [= setting]
- xmlmap.RootElementName
- xmlmap.RootElementNamespace
- xmlmap.SaveDataSourceDefinition [= setting]
- xmlmap.Schemas
- xmlmap.ShowImportExportValidationErrors [= setting]
- 15.6. XmlDataBinding Members
- xmldatabinding.ClearSettings
- xmldatabinding.LoadSettings(Url)
- xmldatabinding.Refresh
- xmldatabinding.SourceUrl
- 15.7. XmlNamespace and XmlNamespaces Members
- xmlnamespaces.InstallManifest(Path, [InstallForAllUsers])
- xmlnamespace.Prefix
- xmlnamespace.Uri
- xmlnamespaces.Value
- 15.8. XmlSchema and XmlSchemas Members
- xmlschema.Namespace
- xmlschema.XML
- 15.9. Get an XML Map from a List or Range
- 15.9.1. Map XML to a List Column
- 15.9.2. Remove a Mapping
- 15.10. XPath Members
- xpath.Clear
- xpath.Map
- xpath.Repeating
- xpath.SetValue(Map, XPath, [SelectionNamespace], [Repeating])
- xpath.Value
- 15.11. Resources
- 16. Charting
- 16.1. Navigate Chart Objects
- 16.2. Create Charts Quickly
- 16.3. Embed Charts
- 16.4. Create More Complex Charts
- 16.5. Choose Chart Type
- 16.6. Create Combo Charts
- 16.7. Add Titles and Labels
- 16.8. Plot a Series
- 16.9. Respond to Chart Events
- 16.10. Chart and Charts Members
- chart.Add([Before], [After], [Count])
- chart.ApplyCustomType(ChartType, [TypeName])
- chart.ApplyDataLabels([Type], [LegendKey], [AutoText], [HasLeaderLines], [ShowSeriesName], [ShowCategoryName], [ShowValues], [ShowPercentage], [ShowBubbleSize], [Separator])
- chart.Area3DGroup
- chart.AreaGroups([Index])
- chart.AutoFormat(Gallery, [Format])
- chart.AutoScaling [= setting]
- chart.Axes([Type], [AxisGroup])
- chart.Bar3DGroup
- chart.BarGroups([Index])
- chart.BarShape [= xlBarShape]
- chart.ChartArea
- chart.ChartGroups([Index])
- chart.ChartObjects([Index])
- chart.ChartTitle
- chart.ChartType [= xlChartType]
- chart.ChartWizard([Source], [Gallery], [Format], [PlotBy], [CategoryLabels], [SeriesLabels], [HasLegend], [Title], [CategoryTitle], [ValueTitle], [ExtraTitle])
- chart.CodeName
- chart.Column3DGroup
- chart.ColumnGroups([Index])
- chart.CopyPicture([Appearance], [Format], [Size])
- chart.Corners
- chart.CreatePublisher([Edition], [Appearance], [Size], [ContainsPICT], [ContainsBIFF], [ContainsRTF], [ContainsVALU])
- chart.DataTable
- chart.DepthPercent [= setting]
- chart.Deselect( )
- chart.DisplayBlanksAs [= xlDisplayBlanksAs]
- chart.DoughnutGroups([Index])
- chart.Elevation [= setting]
- chart.Floor
- chart.GapDepth [= setting]
- chart.GetChartElement(x, y, ElementID, Arg1, Arg2)
- chart.HasAxis(xlAxisGroup, xlAxisType) [= setting]
- chart.HasDataTable [= setting]
- chart.HasLegend [= setting]
- chart.HasPivotFields [= setting]
- chart.HasTitle [= setting]
- chart.HeightPercent [= setting]
- chart.Legend
- chart.Line3DGroup
- chart.LineGroups([Index])
- chart.Location(Where, [Name])
- chart.Perspective [= setting]
- chart.Pie3DGroup
- chart.PieGroups([Index])
- chart.PivotLayout
- chart.PlotArea
- chart.PlotBy [= xlRowCol]
- chart.PlotVisibleOnly [= setting]
- chart.Refresh( )
- chart.RightAngleAxes [= setting]
- chart.Rotation [= setting]
- chart.Select([Replace])
- chart.SeriesCollection([Index])
- chart.SetBackgroundPicture(Filename)
- chart.SetSourceData(Source, [PlotBy])
- chart.ShowWindow [= setting]
- chart.SizeWithWindow [= setting]
- chart.SurfaceGroup
- chart.Walls
- chart.WallsAndGridlines2D [= setting]
- chart.XYGroups([Index])
- 16.11. ChartObject and ChartObjects Members
- chartobjects.Add(Left, Top, Width, Height)
- chartobject.BottomRightCell
- chartobject.Chart
- chartobjects.RoundedCorners [= setting]
- chartobject.TopLeftCell
- 16.12. ChartGroup and ChartGroups Members
- chartgroup.BubbleScale [= setting]
- chartgroup.DoughnutHoleSize [= setting]
- chartgroup.DownBars
- chartgroup.DropLines
- chartgroup.FirstSliceAngle [= setting]
- chartgroup.GapWidth [= setting]
- chartgroup.Has3DShading [= setting]
- chartgroup.HasDropLines [= setting]
- chartgroup.HasHiLoLines [= setting]
- chartgroup.HasRadarAxisLabels [= setting]
- chartgroup.HasSeriesLines [= setting]
- chartgroup.HasUpDownBars [= setting]
- chartgroup.HiLoLines
- chartgroup.Overlap [= setting]
- chartgroup.RadarAxisLabels
- chartgroup.SecondPlotSize [= setting]
- chartgroup.SeriesLines
- chartgroup.ShowNegativeBubbles [= setting]
- chartgroup.SizeRepresents [= xlSizeRepresents]
- chartgroup.SplitType [= xlSplitType]
- chartgroup.SplitValue [= setting]
- chartgroup.UpBars
- chartgroup.VaryByCategories [= setting]
- 16.13. SeriesLines Members
- 16.14. Axes and Axis Members
- axis.AxisBetweenCategories [= setting]
- axis.AxisGroup
- axis.AxisTitle
- axis.BaseUnit [= setting]
- axis.BaseUnitIsAuto [= setting]
- axis.CategoryNames [= setting]
- axis.CategoryType [= xlCategoryType]
- axis.Crosses [= xlAxisCrosses]
- axis.CrossesAt [= setting]
- axis.DisplayUnit [= xlDisplayUnit]
- axis.DisplayUnitCustom [= setting]
- axis.DisplayUnitLabel
- axis.HasDisplayUnitLabel [= setting]
- axis.HasMajorGridlines [= setting]
- axis.HasMinorGridlines [= setting]
- axis.HasTitle [= setting]
- axes.[Item](Type, [AxisGroup])
- axis.MajorGridlines
- axis.MajorTickMark [= xlTickMark]
- axis.MajorUnit [= setting]
- axis.MajorUnitIsAuto [= setting]
- axis.MajorUnitScale [= xlTimeUnit]
- axis.MaximumScale [= setting]
- axis.MaximumScaleIsAuto [= setting]
- axis.MinimumScale [= setting]
- axis.MinimumScaleIsAuto [= setting]
- axis.MinorGridlines
- axis.MinorTickMark [= xlTickMark]
- axis.MinorUnit [= setting]
- axis.MinorUnitIsAuto [= setting]
- axis.MinorUnitScale [= xlTimeUnit]
- axis.ReversePlotOrder [= setting]
- axis.ScaleType [= xlScaleType]
- axis.TickLabelPosition [= xlTickLabelPosition]
- axis.TickLabels
- axis.TickLabelSpacing [= setting]
- axis.TickMarkSpacing [= setting]
- axis.Type
- 16.15. DataTable Members
- 16.16. Series and SeriesCollection Members
- seriescollection.Add(Source, [Rowcol], [SeriesLabels], [CategoryLabels], [Replace])
- series.ApplyCustomType(ChartType)
- series.ApplyDataLabels([Type], [LegendKey], [AutoText], [HasLeaderLines], [ShowSeriesName], [ShowCategoryName], [ShowValues], [ShowPercentage], [ShowBubbleSize], [Separator])
- series.ApplyPictToEnd [= setting]
- series.ApplyPictToFront [= setting]
- series.ApplyPictToSides [= setting]
- series.AxisGroup [= xlAxisGroup]
- series.ChartType [= xlChartType]
- series.ClearFormats( )
- series.DataLabels([Index])
- series.ErrorBar(Direction, Include, Type, [Amount], [MinusValues])
- series.ErrorBars
- series.Explosion [= setting]
- seriescollection.Extend(Source, [Rowcol], [CategoryLabels])
- series.Fill
- series.Formula [= setting]
- End Function series.FormulaLocal [= setting]
- series.FormulaR1C1 [= setting]
- series.FormulaR1C1Local [= setting]
- series.Has3DEffect [= setting]
- series.HasDataLabels [= setting]
- series.HasErrorBars [= setting]
- series.HasLeaderLines [= setting]
- series.InvertIfNegative [= setting]
- series.LeaderLines
- series.MarkerBackgroundColor [= setting]
- series.MarkerBackgroundColorIndex [= setting]
- series.MarkerForegroundColor [= setting]
- series.MarkerForegroundColorIndex [= setting]
- series.MarkerSize [= setting]
- series.MarkerStyle [= xlMarkerStyle]
- seriescollection.NewSeries( )
- seriescollection.Paste([Rowcol], [SeriesLabels], [CategoryLabels], [Replace], [NewSeries])
- series.PictureType [= xlChartPictureType]
- series.PictureUnit [= setting]
- series.PlotOrder [= setting]
- series.Points([Index])
- series.Smooth [= setting]
- series.Trendlines([Index])
- series.Values [= setting]
- series.XValues [= setting]
- 16.17. Point and Points Members
- point.SecondaryPlot [= setting]
- 17. Formatting Charts
- 17.1. Format Titles and Labels
- 17.2. Change Backgrounds and Fonts
- 17.3. Add Trendlines
- 17.4. Add Series Lines and Bars
- 17.5. ChartTitle, AxisTitle, and DisplayUnitLabel Members
- title.Caption [= setting]
- title.Characters
- title.Fill
- title.Font
- title.HorizontalAlignment [= setting]
- title.Orientation [= setting]
- title.ReadingOrder [= setting]
- title.VerticalAlignment [= setting]
- 17.6. DataLabel and DataLabels Members
- datalabel AutoText [= setting]
- datalabels.NumberFormat [= setting]
- datalabel.NumberFormatLinked [= setting]
- datalabel.NumberFormatLocal [= setting]
- datalabel.Position [= xlDataLabelPosition]
- datalabel.Separator [= setting]
- datalabel.ShowBubbleSize [= setting]
- datalabel.ShowCategoryName [= setting]
- datalabel.ShowLegendKey [= setting]
- datalabel.ShowPercentage [= setting]
- datalabel.ShowSeriesName [= setting]
- datalabel.ShowValue [= setting]
- 17.7. LeaderLines Members
- 17.8. ChartArea Members
- chartarea.AutoScaleFont [= setting]
- chartarea.Clear( )
- chartarea.ClearContents( )
- chartarea.ClearFormats( )
- chartarea.Fill
- chartarea.Font
- chartarea.Interior
- chartarea.Shadow [= setting]
- 17.9. ChartFillFormat Members
- chartfillformat.BackColor
- chartfillformat.ForeColor
- chartfillformat.GradientColorType
- chartfillformat.GradientDegree
- chartfillformat.GradientStyle
- chartfillformat.GradientVariant
- chartfillformat.OneColorGradient(Style, Variant, Degree)
- chartfillformat.Pattern
- chartfillformat.Patterned(Pattern)
- chartfillformat.PresetGradient(Style, Variant, PresetGradientType)
- chartfillformat.PresetGradientType
- chartfillformat.PresetTexture
- chartfillformat.PresetTextured(PresetTexture)
- chartfillformat.Solid( )
- chartfillformat.TextureName
- chartfillformat.TextureType
- chartfillformat.TwoColorGradient(Style, Variant)
- chartfillformat.UserPicture(PictureFile)
- chartfillformat.UserTextured(TextureFile)
- 17.10. ChartColorFormat Members
- 17.11. DropLines and HiLoLines Members
- 17.12. DownBars and UpBars Members
- 17.13. ErrorBars Members
- errorbars.ClearFormats( )
- errorbars.EndStyle [=xlEndStyleCap]
- 17.14. Legend Members
- legend.LegendEntries([Index])
- legend.Position [= xlLegendPosition]
- 17.15. LegendEntry and LegendEntries Members
- legendentry.LegendKey
- 17.16. LegendKey Members
- 17.17. Gridlines Members
- 17.18. TickLabels Members
- ticklabels.Alignment [= setting]
- ticklabels.Depth
- ticklabels.Font
- ticklabels.NumberFormat [= setting]
- ticklabels.NumberFormatLinked [= setting]
- ticklabels.NumberFormatLocal [= setting]
- ticklabels.Offset [= setting]
- ticklabels.Orientation [= xlTickLabelOrientation]
- ticklabels.ReadingOrder [= setting]
- 17.19. Trendline and Trendlines Members
- trendlines.Add([Type], [Order], [Period], [Forward], [Backward], [Intercept], [DisplayEquation], [DisplayRSquared], [Name])
- trendline.Backward [= setting]
- trendline.ClearFormats( )
- trendline.DataLabel
- trendline.DisplayEquation [= setting]
- trendline.DisplayRSquared [= setting]
- trendline.Forward [= setting]
- trendline.Intercept [= setting]
- trendline.InterceptIsAuto [= setting]
- trendline.Name [= setting]
- trendline.NameIsAuto [= setting]
- trendline.Order [= setting]
- trendline.Period [= setting]
- 17.20. PlotArea Members
- plotarea.ClearFormats( )
- plotarea.Fill
- plotarea.InsideHeight
- plotarea.InsideLeft
- plotarea.InsideTop
- plotarea.InsideWidth
- 17.21. Floor Members
- 17.22. Walls Members
- 17.23. Corners Members
- 18. Drawing Graphics
- 18.1. Draw in Excel
- 18.2. Create Diagrams
- 18.3. Program with Drawing Objects
- 18.3.1. Draw Simple Shapes
- 18.3.2. Add Text
- 18.3.3. Connect Shapes
- 18.3.4. Insert Pictures
- 18.3.5. Insert Other Objects
- 18.3.6. Group Shapes
- 18.4. Program Diagrams
- 18.5. Shape, ShapeRange, and Shapes Members
- shapes.AddCallout(Type, Left, Top, Width, Height)
- shapes.AddConnector(Type, BeginX, BeginY, EndX, EndY)
- shapes.AddCurve(SafeArrayOfPoints)
- shapes.AddLabel(Orientation, Left, Top, Width, Height)
- shapes.AddLine(BeginX, BeginY, EndX, EndY)
- shapes.AddPicture(Filename, LinkToFile, SaveWithDocument, Left, Top, Width, Height)
- shapes.AddPolyline(SafeArrayOfPoints)
- shapes.AddShape(Type, Left, Top, Width, Height)
- shapes.AddTextbox(Orientation, Left, Top, Width, Height)
- shapes.AddTextEffect(PresetTextEffect, Text, FontName, FontSize, FontBold, FontItalic, Left, Top)
- shape.Adjustments
- shaperange.Align(AlignCmd, RelativeTo)
- shape.AlternativeText [= setting]
- shape.Apply( )
- shape.AutoShapeType [= msoAutoShapeType]
- shape.BlackWhiteMode [= msoBlackWhiteMode]
- shapes.BuildFreeform(EditingType, X1, Y1)
- shape.Callout
- shape.ConnectionSiteCount
- shape.Connector
- shape.ConnectorFormat
- shape.ControlFormat
- shaperange.Distribute(DistributeCmd, RelativeTo)
- shape.Duplicate( )
- shape.Fill
- shape.Flip(FlipCmd)
- shape.FormControlType
- shaperange.Group( )
- shape.GroupItems
- shape.HorizontalFlip
- shape.Hyperlink
- shape.ID
- shape.IncrementLeft(Increment)
- shape.IncrementRotation(Increment)
- shape.IncrementTop(Increment)
- shape.Line
- shape.LinkFormat
- shape.LockAspectRatio [= setting]
- shape.Locked [= setting]
- shape.ParentGroup
- shape.PickUp( )
- shape.PictureFormat
- shape.Placement [= xlPlacement]
- shapes.Range(Index)
- shaperange.Regroup( )
- shape.RerouteConnections( )
- shape.Rotation [= setting]
- shapes.SelectAll( )
- shape.SetShapesDefaultProperties( )
- shape.Shadow
- shape.TextEffect
- shape.TextFrame
- shape.ThreeD
- shape.Type
- shape.Ungroup( )
- shape.VerticalFlip
- shape.Vertices
- 18.6. Adjustments Members
- 18.7. CalloutFormat Members
- callout.Accent [= setting]
- callout.Angle [= msoCalloutAngleType]
- callout.AutoAttach [= setting]
- callout.AutoLength
- callout.AutomaticLength( )
- callout.Border [= setting]
- callout.CustomDrop(Drop)
- callout.CustomLength(Length)
- callout.Drop
- callout.DropType
- callout.Gap [= setting]
- callout.Length
- callout.PresetDrop(DropType)
- callout.Type [= msoCalloutType]
- 18.8. ColorFormat Members
- colorformat.TintAndShade [= setting]
- 18.9. ConnectorFormat Members
- connectorformat.BeginConnect(ConnectedShape, ConnectionSite)
- connectorformat.BeginConnected
- connectorformat.BeginConnectedShape
- connectorformat.BeginConnectionSite
- connectorformat.BeginDisconnect( )
- connectorformat.EndConnect(ConnectedShape, ConnectionSite)
- connectorformat.EndConnected
- connectorformat.EndConnectedShape
- connectorformat.EndConnectionSite
- connectorformat.EndDisconnect( )
- connectorformat.Type [= msoConnectorType]
- 18.10. ControlFormat Members
- 18.11. FillFormat Members
- fillformat.BackColor
- fillformat.ForeColor
- fillformat.Transparency [= setting]
- 18.12. FreeFormBuilder
- freeformbuilder.AddNodes(SegmentType, EditingType, X1, Y1, [X2], [Y2], [X3], [Y3])
- freeformbuilder.ConvertToShape( )
- 18.13. GroupShapes Members
- 18.14. LineFormat Members
- 18.15. LinkFormat Members
- 18.16. PictureFormat Members
- pictureformat.Brightness [= setting]
- pictureformat.ColorType [= msoPictureColorType]
- pictureformat.Contrast [= setting]
- pictureformat.CropBottom [= setting]
- pictureformat.CropLeft [= setting]
- pictureformat.CropRight [= setting]
- pictureformat.CropTop [= setting]
- pictureformat.IncrementBrightness(Increment)
- pictureformat.IncrementContrast(Increment)
- pictureformat.TransparencyColor [= setting]
- pictureformat.TransparentBackground [= setting]
- 18.17. ShadowFormat
- 18.18. ShapeNode and ShapeNodes Members
- 18.19. TextFrame
- textframe.AutoMargins [= setting]
- textframe.AutoSize [= setting]
- textframe.Characters([Start], [Length])
- textframe.HorizontalAlignment [= xlHAlign]
- textframe.MarginBottom [= setting]
- textframe.MarginLeft [= setting]
- textframe.MarginRight [= setting]
- textframe.MarginTop [= setting]
- textframe.Orientation [= msoTextOrientation]
- textframe.VerticalAlignment [= xlVAlign]
- 18.20. TextEffectFormat
- shape.Alignment [= msoTextEffectAlignment]
- shape.FontBold [= setting]
- shape.FontItalic [= setting]
- shape.FontName [= setting]
- shape.FontSize [= setting]
- shape.KernedPairs [= setting]
- shape.NormalizedHeight [= setting]
- shape.PresetShape [= msoPresetTextEffectShape]
- shape.PresetTextEffect [= msoPresetTextEffect]
- shape.RotatedChars [= setting]
- shape.Text [= setting]
- shape.ToggleVerticalText( )
- shape.Tracking [= setting]
- 18.21. ThreeDFormat
- 19. Adding Menus and Toolbars
- 19.1. About Excel Menus
- 19.2. Build a Top-Level Menu
- 19.2.1. Change Existing Menus
- 19.2.2. Assign Accelerator and Shortcut Keys
- 19.2.3. Save and Distribute Menus
- 19.3. Create a Menu in Code
- 19.3.1. Remove the Menu on Close
- 19.3.2. Change an Existing Menu
- 19.3.3. Reset an Existing Menu
- 19.4. Build Context Menus
- 19.4.1. Change Context Menu Items
- 19.4.2. Restore Context Menus
- 19.4.3. Create New Context Menus
- 19.5. Build a Toolbar
- 19.5.1. Create Menus Using Toolbars
- 19.5.2. Save and Distribute Toolbars
- 19.6. Create Toolbars in Code
- 19.6.1. Add Edit Controls to Toolbars
- 19.6.2. Delete Toolbars
- 19.7. CommandBar and CommandBars Members
- commandbars.ActionControl
- commandbars.ActiveMenuBar
- commandbars.AdaptiveMenus [= setting]
- commandbars.Add([Name], [Position], [MenuBar], [Temporary])
- commandbar.BuiltIn
- commandbar.Controls
- commandbar.Delete( )
- commandbars.DisableAskAQuestionDropdown [= setting]
- commandbars.DisableCustomize [= setting]
- commandbars.DisplayFonts [= setting]
- commandbar.DisplayKeysInTooltips [= setting]
- commandbars.DisplayTooltips [= setting]
- commandbar.Enabled [= setting]
- commandbar.FindControl([Type], [Id], [Tag], [Visible], [Recursive])
- commandbars.FindControls([Type], [Id], [Tag], [Visible])
- commandbar.Id
- commandbars.LargeButtons [= setting]
- commandbars.MenuAnimationStyle [= msoMenuAnimation]
- commandbar.Name [= setting]
- commandbar.NameLocal [= setting]
- commandbar.Position [= msoBarPosition]
- commandbar.Protection [= msoBarProtection]
- CommandBars.ReleaseFocus( )
- commandbar.Reset( )
- commandbar.RowIndex [= msoBarRow]
- commandbar.ShowPopup([x], [y])
- commandbar.Type
- 19.8. CommandBarControl and CommandBarControls Members
- commandbarcontrols.Add([Type], [Id], [Parameter], [Before], [Temporary])
- commandbarcontrol.BeginGroup [= setting]
- commandbarcontrol.BuiltIn
- commandbarcontrol.Caption [= setting]
- commandbarcontrol.Copy([Bar], [Before])
- commandbarcontrol.Delete([Temporary])
- commandbarcontrol.DescriptionText [= setting]
- commandbarcontrol.Enabled [= setting]
- commandbarcontrol.Execute( )
- commandbarcontrol.HelpContextId [= setting]
- commandbarcontrol.HelpFile [= setting]
- commandbarcontrol.Id
- commandbarcontrol.IsPriorityDropped
- commandbarcontrol.Move([Bar], [Before])
- commandbarcontrol.OLEUsage [= msoControlOLEUsage]
- commandbarcontrol.OnAction [= setting]
- commandbarcontrol.Parameter [= setting]
- commandbarcontrol.Priority [= setting]
- commandbarcontrol.Reset( )
- commandbarcontrol.SetFocus( )
- commandbarcontrol.Tag [= setting]
- commandbarcontrol.TooltipText [= setting]
- commandbarcontrol.Type
- 19.9. CommandBarButton Members
- commandbarbutton.CopyFace( )
- commandbarbutton.FaceId [= setting]
- commandbarbutton.HyperlinkType [= msoCommandBarButtonHyperlinkType]
- commandbarbutton.Mask
- commandbarbutton.PasteFace( )
- commandbarbutton.Picture
- commandbarbutton.ShortcutText [= setting]
- commandbarbutton.State [= msoButtonState]
- commandbarbutton.Style [= msoButtonStyle]
- 19.10. CommandBarComboBox Members
- commandbarcombobox.AddItem(Text, [Index])
- commandbarcombobox.Clear( )
- commandbarcombobox.DropDownLines [= setting]
- commandbarcombobox.DropDownWidth [= setting]
- commandbarcombobox.List(Index)
- commandbarcombobox.ListCount
- commandbarcombobox.ListHeaderCount [= setting]
- commandbarcombobox.ListIndex [= setting]
- commandbarcombobox.RemoveItem(Index)
- commandbarcombobox.Style [= msoComboStyle]
- commandbarcombobox.Text [= setting]
- 19.11. CommandBarPopup Members
- commandbarpopup.Controls
- commandbarpopup.OLEMenuGroup [= msoOLEMenuGroup]
- 20. Building Dialog Boxes
- 20.1. Types of Dialogs
- 20.2. Create Data-Entry Forms
- 20.2.1. Advanced Validation
- 20.2.2. Data Forms from Code
- 20.3. Design Your Own Forms
- 20.3.1. Respond to Form Events
- 20.3.2. Show a Form
- 20.3.3. Separate Work Code from UI Code
- 20.3.4. Enable and Disable Controls
- 20.3.5. Create Tabbed Dialogs
- 20.3.6. Provide Keyboard Access to Controls
- 20.3.7. Choose the Right Control
- 20.4. Use Controls on Worksheets
- 20.4.1. Add a Simple Button
- 20.4.2. Use Controls from the Worksheet Class
- 20.4.3. Controls on a Worksheet Versus Controls on a Form
- 20.5. UserForm and Frame Members
- form.ActiveControl
- form.BackColor [= rgb]
- form.BorderColor [= rgb]
- form.BorderStyle [= fmBorderStyle]
- form.CanPaste
- form.CanRedo
- form.CanUndo
- form.Caption [= setting]
- form.Controls
- form.Copy( )
- form.Cut( )
- form.Cycle [= fmCycle]
- form.DrawBuffer [= setting]
- form.Enabled [= setting]
- form.Font [= setting]
- form.ForeColor [= rgb]
- form.InsideHeight
- form.InsideWidth
- form.KeepScrollBarsVisible [= fmScrollBars]
- form.MouseIcon [= setting]
- form.MousePointer [= fmMousePointer]
- form.Paste( )
- form.Picture [= setting]
- form.PictureAlignment [= fmPictureAlignment]
- form.PictureSizeMode [= fmPictureSizeMode]
- form.PictureTiling [= setting]
- form.PrintForm
- form.RedoAction( )
- form.Repaint( )
- form.Scroll([ActionX] [, ActionY])
- form.ScrollBars [= fmScrollBars]
- form.ScrollHeight [= setting]
- form.ScrollLeft [= setting]
- form.ScrollTop [= setting]
- form.ScrollWidth [= setting]
- form.SetDefaultTabOrder( )
- form.SpecialEffect [= fmButtonEffect]
- form.UndoAction( )
- form.VerticalScrollBarSide [= fmVerticalScrollbarSide]
- form.Zoom [= setting]
- 20.6. Control and Controls Members
- controls.Add(ProgID [, Name] [, Visible])
- control.Cancel [= setting]
- controls .Clear( )
- control.ControlSource [= setting]
- control.ControlTipText [= setting]
- control.Default [= setting]
- control.LayoutEffect
- controls.Move ([Left ][, Top ][, Width ][, Height ][, Layout])
- control.Object
- control.OldHeight
- control.OldLeft
- control.OldTop
- control.OldWidth
- control.Remove(Index)
- control.RowSource [= setting]
- control.SetFocus( )
- control.TabIndex [= setting]
- control.TabStop [= setting]
- control.Tag [= setting]
- control.ZOrder([zPosition])
- 20.7. Font Members
- 20.8. CheckBox, OptionButton, ToggleButton Members
- control.AutoSize [= setting]
- control.BackStyle [= fmBackStyle]
- control.GroupName [= setting]
- control.Locked [= setting]
- control.TripleState [= setting]
- 20.9. ComboBox Members
- control.AddItem(Item[, Index])
- control.AutoTab [= setting]
- control.AutoWordSelect [= setting]
- control.BoundColumn [= setting]
- control.Clear( )
- control.Column([Column][, Row])
- control.ColumnCount [= setting]
- control.ColumnHeads [= setting]
- control.ColumnWidths [= setting]
- control.CurTargetX
- control.CurX [= setting]
- control.DragBehavior [= fmDragBehavior]
- control.DropButtonStyle [= fmDropButtonStyle]
- control.DropDown( )
- control.EnterFieldBehavior [= fmEnterFieldBehavior]
- control.HideSelection [= setting]
- control.IMEMode [= fmIMEMode]
- control.LineCount
- control.List([Row, Column]) [= setting]
- control.ListCount
- control.ListIndex [= setting]
- control.ListRows [= setting]
- control.ListStyle [= fmListStyle]
- control.ListWidth [= setting]
- control.MatchEntry [= fmMatchEntry]
- control.MatchFound
- control.MatchRequired [= setting]
- control.MaxLength [= setting]
- control.RemoveItem(Index)
- control.SelectionMargin [= setting]
- control.SelLength [= setting]
- control.SelStart [= setting]
- control.SelText [= setting]
- control.ShowDropButtonWhen [= fmShowDropButtonWhen]
- control.Style [= fmStyle]
- control.Text [= setting]
- control.TextAlign [= fmTextAlign]
- control.TextColumn [= setting]
- control.TextLength
- control.TopIndex [= setting]
- 20.10. CommandButton Members
- control.Picture [= setting]
- control.PicturePosition [= fmPicturePosition]
- control.TakeFocusOnClick [= setting]
- 20.11. Image Members
- control.Picture [= setting]
- control.PictureAlignment [= fmPictureAlignment]
- control.PictureSizeMode [= fmPictureSizeMode]
- control.PictureTiling [= setting]
- 20.12. Label Members
- 20.13. ListBox Members
- control.IntegralHeight [= setting]
- control.MultiSelect [= fmMultiSelect]
- 20.14. MultiPage Members
- control.MultiRow [= setting]
- control.Pages
- control.SelectedItem
- control.Style [= fmTabStyle]
- control.TabFixedHeight [= setting]
- control.TabFixedWidth [= setting]
- control.TabOrientation [= fmTabOrientation]
- 20.15. Page Members
- control.TransitionEffect [= fmTransitionEffect]
- control.TransitionPeriod [= setting]
- 20.16. ScrollBar and SpinButton Members
- control.LargeChange [= setting]
- control.Max [= setting]
- control.Min [= setting]
- control.Orientation [= fmOrientation]
- control.ProportionalThumb [= setting]
- control.SmallChange [= setting]
- 20.17. TabStrip Members
- control.ClientHeight
- control.ClientLeft
- control.ClientTop
- control.ClientWidth
- control.Tabs
- 20.18. TextBox and RefEdit Members
- control.CurLine [= setting]
- control.EnterKeyBehavior [= fmEnterFieldBehavior]
- control.IntegralHeight [= setting]
- control.LineCount
- control.MultiLine [= setting]
- control.PasswordChar [= setting]
- control.ScrollBars [= fmScrollBars]
- control.TabKeyBehavior [= setting]
- control.WordWrap [= setting]
- 21. Sending and Receiving Workbooks
- 21.1. Send Mail
- 21.2. Work with Mail Items
- 21.3. Collect Review Comments
- 21.4. Route Workbooks
- 21.5. Read Mail
- 21.6. MsoEnvelope Members
- mailenvelope.Introduction [= setting]
- mailenvelope.Item
- 21.7. MailItem Members
- mailitem.Attachments
- mailitem.BCC [= setting]
- mailitem.Body [= setting]
- mailitem.CC [= setting]
- mailitem.Close(SaveMode)
- mailitem.DeferredDeliveryTime [= setting]
- mailitem.DeleteAfterSubmit [= setting]
- mailitem.Display( )
- mailitem.ExpiryTime [= setting]
- mailitem.HTMLBody [= setting]
- mailitem.Importance [= setting]
- mailitem.PrintOut( )
- mailitem.ReadReceiptRequested [= setting]
- mailitem.Recipients
- mailitem.Save( )
- mailitem.SaveAs(Path, Type)
- mailitem.SaveSentMessageFolder [= setting]
- mailitem.Send( )
- mailitem.SenderEmailAddress
- mailitem.SenderName
- mailitem.Sensitivity [= olSensitivity]
- mailitem.Subject [= setting]
- mailitem.To [= setting]
- 21.8. RoutingSlip Members
- routingslip.Delivery [= xlRoutingSlipDelivery]
- routingslip.Message [= setting]
- routingslip.Recipients [= setting]
- routingslip.Reset( )
- routingslip.ReturnWhenDone [= setting]
- routingslip.Status
- routingslip.Subject [= setting]
- routingslip.TrackStatus [= setting]
- 7. Controlling Excel
- III. Extending Excel
- 22. Building Add-ins
- 22.1. Types of Add-ins
- 22.2. Code-Only Add-ins
- 22.2.1. Save Add-ins
- 22.2.2. Create a Test Workbook
- 22.2.3. Use the Add-in from Code
- 22.2.4. Change the Add-in
- 22.2.5. Programming Tips
- 22.3. Visual Add-ins
- 22.3.1. Add a Menu Item
- 22.3.2. Add a Toolbar
- 22.3.3. Respond to Application Events
- 22.4. Set Add-in Properties
- 22.5. Sign the Add-in
- 22.6. Distribute the Add-in
- 22.7. Work with Add-ins in Code
- 22.8. AddIn and AddIns Members
- addins.Add(Filename, [CopyFile])
- addin.Author
- addin.Comments
- addin.FullName
- addin.Installed [= setting]
- addin.Keywords
- addin.Path
- addin.Subject
- addin.Title
- 23. Integrating DLLs and COM
- 23.1. Use DLLs
- 23.1.1. Find the Right Function
- 23.1.2. Declare and Use DLL Functions
- 23.1.3. Use Flags and Constants
- 23.1.4. Work with Strings
- 23.1.5. Handle Exceptions
- 23.2. Use COM Applications
- 23.2.1. Program Other Office Applications
- 23.2.2. Integrate Word
- 23.2.3. Automate PowerPoint
- 23.2.4. Handle Exceptions
- 23.2.5. Get Help on Objects
- 23.1. Use DLLs
- 24. Getting Data from the Web
- 24.1. Perform Web Queries
- 24.1.1. Modify a Web Query
- 24.1.2. Perform Periodic Updates
- 24.1.3. Trap QueryTable Events
- 24.1.4. Manage Web Queries
- 24.1.5. Limitations of Web Queries
- 24.2. QueryTable and QueryTables Web Query Members
- querytables.Add(Connection, Destination, [Sql])
- querytable.AdjustColumnWidth [= setting]
- querytable.BackgroundQuery [= setting]
- querytable.CancelRefresh
- querytable.Connection [= setting]
- querytable.Delete
- querytable.Destination
- querytable.EditWebPage [= setting]
- querytable.EnableEditing [= setting]
- querytable.EnableRefresh [= setting]
- querytable.FetchedRowOverflow
- querytable.FillAdjacentFormulas [= setting]
- querytable.PostText [= setting]
- querytable.PreserveFormatting [= setting]
- querytable.QueryType [= xlQueryType]
- querytable.Refresh([BackgroundQuery])
- querytable.Refreshing
- querytable.RefreshOnFileOpen [= setting]
- querytable.RefreshPeriod [= setting]
- querytable.RefreshStyle [= xlCellInsertionMode]
- querytable.ResetTimer
- querytable.ResultRange
- querytable.TablesOnlyFromHTML [= setting]
- querytable.WebConsecutiveDelimitersAsOne [= setting]
- querytable.WebDisableDateRecognition [= setting]
- querytable.WebDisableRedirections [= setting]
- querytable.WebFormatting [= setting]
- querytable.WebPreFormattedTextToColumns [= setting]
- querytable.WebSelectionType [= xlWebSelectionType]
- querytable.WebSingleBlockTextImport [= setting]
- querytable.WebTables [= setting]
- 24.3. Use Web Services
- 24.3.1. Use the Web Services Toolkit
- 24.3.2. Use Web Services Through XML
- 24.3.3. Call a Web Service Asynchronously
- 24.3.4. Reformat XML Results for Excel
- 24.4. Resources
- 24.1. Perform Web Queries
- 25. Programming Excel with .NET
- 25.1. Approaches to Working with .NET
- 25.2. Create .NET Components for Excel
- 25.3. Use .NET Components in Excel
- 25.3.1. Respond to Errors and Events from .NET Objects
- 25.3.2. Debug .NET Components
- 25.3.3. Distribute .NET Components
- 25.4. Use Excel as a Component in .NET
- 25.4.1. Work with Excel Objects in .NET
- 25.4.2. Respond to Excel Events in .NET
- 25.4.3. Respond to Excel Exceptions in .NET
- 25.4.4. Distribute .NET Applications That Use Excel
- 25.5. Create Excel Applications in .NET
- 25.5.1. Set .NET Security Policies
- 25.5.2. Respond to Events in .NET Applications
- 25.5.3. Debug Excel .NET Applications
- 25.5.4. Display Forms
- 25.5.5. Distribute Excel .NET Applications
- 25.5.6. Migrate to .NET
- 25.5.6.1. Be explicit
- 25.5.6.2. Pass arguments by value
- 25.5.6.3. Collections start at zero
- 25.5.6.4. Data access is through ADO.NET
- 25.6. Resources
- 26. Exploring Security in Depth
- 26.1. Security Layers
- 26.2. Understand Windows Security
- 26.2.1. Set File Permissions in Windows XP
- 26.2.2. View Users and Groups in XP
- 26.3. Password-Protect and Encrypt Workbooks
- 26.4. Program with Passwords and Encryption
- 26.5. Workbook Password and Encryption Members
- workbook.HasPassword
- workbook.Password [= setting]
- workbook.PasswordEncryptionAlgorithm
- workbook.PasswordEncryptionFileProperties
- workbook.PasswordEncryptionKeyLength
- workbook.PasswordEncryptionProvider
- workbook.SetPasswordEncryptionOptions(PasswordEncryptionProvider, PasswordEncryptionAlgorithm, PasswordEncryptionKeyLength, PasswordEncryptionFileProperties)
- workbook.WritePassword [= setting]
- workbook.WriteReserved
- workbook.WriteReservedBy
- 26.6. Excel Password Security
- 26.7. Protect Items in a Workbook
- 26.8. Program with Protection
- 26.9. Workbook Protection Members
- workbook.Protect([Password], [Structure], [Windows])
- workbook.ProtectSharing([Filename], [Password], [WriteResPassword], [ReadOnlyRecommended], [CreateBackup], [SharingPassword])
- workbook.ProtectStructure
- workbook.ProtectWindows
- workbook.Unprotect([Password])
- workbook.UnprotectSharing([SharingPassword])
- 26.10. Worksheet Protection Members
- worksheet.Protect([Password], [DrawingObjects], [Contents], [Scenarios], [UserInterfaceOnly], [AllowFormattingCells], [AllowFormattingColumns], [AllowFormattingRows], [AllowInsertingColumns], [AllowInsertingRows], [AllowInsertingHyperlinks], [AllowDeletingColumns], [AllowDeletingRows], [AllowSorting], [AllowFiltering], [AllowUsingPivotTables])
- worksheet.ProtectContents
- worksheet.ProtectDrawingObjects
- worksheet.Protection
- worksheet.ProtectionMode
- worksheet.ProtectScenarios
- worksheet.Unprotect([Password])
- 26.11. Chart Protection Members
- chart.Protect([Password], [DrawingObjects], [Contents], [Scenarios], [UserInterfaceOnly])
- chart.ProtectData [= setting]
- chart.ProtectGoalSeek [= setting]
- chart.ProtectSelection [= setting]
- chart.UnProtect([Password])
- 26.12. Protection Members
- protection.AllowDeletingColumns
- protection.AllowDeletingRows
- protection.AllowEditRanges
- protection.AllowFiltering
- protection.AllowFormattingCells
- protection.AllowFormattingColumns
- protection.AllowFormattingRows
- protection.AllowInsertingColumns
- protection.AllowInsertingHyperlinks
- protection.AllowInsertingRows
- protection.AllowSorting
- protection.AllowUsingPivotTables
- 26.13. AllowEditRange and AllowEditRanges Members
- alloweditranges.Add(Title, Range, [Password])
- alloweditrange.ChangePassword(Password)
- alloweditrange.Delete( )
- alloweditrange.Range
- alloweditrange.Title
- alloweditrange.Unprotect([Password])
- alloweditrange.Users
- 26.14. UserAccess and UserAccessList Members
- useraccesslist.Add(Name, AllowEdit)
- useraccess.AllowEdit [= setting]
- useraccess.Delete( )
- useraccesslist.DeleteAll( )
- 26.15. Set Workbook Permissions
- 26.16. Program with Permissions
- 26.17. Permission and UserPermission Members
- permission.Add(UserId, [Permission], [ExpirationDate])
- permission.ApplyPolicy(FileName)
- permission.DocumentAuthor [= setting]
- permission.Enabled [= setting]
- permission.EnableTrustedBrowser [= setting]
- userpermission.ExpirationDate [= setting]
- userpermission.Permission [= setting]
- permission.PermissionFromPolicy
- permission.PolicyDescription
- permission.PolicyName
- userpermission.Remove( )
- permission.RemoveAll( )
- permission.RequestPermissionURL [= setting]
- permission.StoreLicenses [= setting]
- userpermission.UserId
- 26.18. Add Digital Signatures
- 26.19. Set Macro Security
- 26.20. Set ActiveX Control Security
- 26.21. Distribute Security Settings
- 26.21.1. Change Security Settings
- 26.21.2. Distribute Certificates
- 26.22. Using the Anti-Virus API
- 26.23. Common Tasks
- 26.23.1. Get Rid of the Macro Security Warning
- 26.23.2. Prevent Someone from Running Any Macros
- 26.23.3. Make a File Truly Secure
- 26.23.4. Add a Trusted Publisher for a Group of Users
- 26.24. Resources
- 22. Building Add-ins
- IV. Appendixes
- A. Reference Tables
- A.1. Dialogs Collection Constants
- A.2. Common Programmatic IDs
- B. Version Compatibility
- B.1. Summary of Version Changes
- B.2. Macintosh Compatibility
- A. Reference Tables
- About the Authors
- Colophon
- SPECIAL OFFER: Upgrade this ebook with OReilly