Programming C#. Building .NET Applications with C#. 4th Edition - Helion
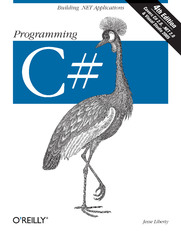
ISBN: 978-05-965-5272-5
stron: 672, Format: ebook
Data wydania: 2005-02-22
Księgarnia: Helion
Cena książki: 101,15 zł (poprzednio: 117,62 zł)
Oszczędzasz: 14% (-16,47 zł)
The programming language C# was built with the future of application development in mind. Pursuing that vision, C#'s designers succeeded in creating a safe, simple, component-based, high-performance language that works effectively with Microsoft's .NET Framework. Now the favored language among those programming for the Microsoft platform, C# continues to grow in popularity as more developers discover its strength and flexibility. And, from the start, C# developers have relied on Programming C# both as an introduction to the language and a means of further building their skills.
The fourth edition of Programming C#--the top-selling C# book on the market--has been updated to the C# ISO standard as well as changes to Microsoft's implementation of the language. It also provides notes and warnings on C# 1.1 and C# 2.0.
Aimed at experienced programmers and web developers, Programming C#, 4th Edition, doesn't waste too much time on the basics. Rather, it focuses on the features and programming patterns unique to the C# language. New C# 2005 features covered in-depth include:
- Visual Studio 2005
- Generics
- Collection interfaces and iterators
- Anonymous methods
- New ADO.NET data controls
- Fundamentals of Object-Oriented Programming
Liberty also incorporates reader suggestions from previous editions to help create the most consumer-friendly guide possible.
Osoby które kupowały "Programming C#. Building .NET Applications with C#. 4th Edition", wybierały także:
- Gray Hat C#. Język C# w kontroli i łamaniu zabezpieczeń 57,74 zł, (17,90 zł -69%)
- Programowanie asynchroniczne i równoległe w C#. Kurs video. Poziom podstawowy 69,00 zł, (31,05 zł -55%)
- Technologia LINQ. Kurs video. Warsztat pracy z danymi z różnych źródeł 59,00 zł, (26,55 zł -55%)
- Język XAML. Kurs video. Poziom pierwszy. Programowanie aplikacji w WPF 59,00 zł, (26,55 zł -55%)
- ASP.NET MVC. Kurs video. Poziom pierwszy. Programowanie aplikacji internetowych 79,00 zł, (35,55 zł -55%)
Spis treści
Programming C#. Building .NET Applications with C#. 4th Edition eBook -- spis treści
- Programming C#, 4th Edition
- SPECIAL OFFER: Upgrade this ebook with OReilly
- A Note Regarding Supplemental Files
- Preface
- About This Book
- What You Need To Use This Book
- How the Book Is Organized
- Part I, The C# Language
- Part II, Programming with C#
- Part III, The CLR and the .NET Framework
- Who This Book Is For
- C# 2.0 Versus C# 1.1
- C# Versus Visual Basic .NET
- C# Versus Java
- C# Versus C and C++
- Conventions Used in This Book
- Support
- Wed Like to Hear from You
- Safari Enabled
- Acknowledgments
- I. The C# Language
- 1. C# and the .NET Framework
- 1.1. The .NET Platform
- 1.2. The .NET Framework
- 1.3. Compilation and the MSIL
- 1.4. The C# Language
- 2. Getting Started: "Hello World"
- 2.1. Classes, Objects, and Types
- 2.1.1. Methods
- 2.1.2. Comments
- 2.1.3. Console Applications
- 2.1.4. Namespaces
- 2.1.5. The Dot Operator (.)
- 2.1.6. The using Keyword
- 2.1.7. Case Sensitivity
- 2.1.8. The static Keyword
- 2.2. Developing "Hello World"
- 2.2.1. Editing "Hello World"
- 2.2.2. Compiling and Running "Hello World"
- 2.3. Using the Visual Studio .NET Debugger
- 2.1. Classes, Objects, and Types
- 3. C# Language Fundamentals
- 3.1. Types
- 3.1.1. Working with Built-in Types
- 3.1.1.1. Choosing a built-in type
- 3.1.1.2. Converting built-in types
- 3.1.1. Working with Built-in Types
- 3.2. Variables and Constants
- 3.2.1. Definite Assignment
- 3.2.2. Constants
- 3.2.3. Enumerations
- 3.2.4. Strings
- 3.2.5. Identifiers
- 3.3. Expressions
- 3.4. Whitespace
- 3.5. Statements
- 3.5.1. Unconditional Branching Statements
- 3.5.2. Conditional Branching Statements
- 3.5.2.1. if...else statements
- 3.5.2.2. Nested if statements
- 3.5.2.3. switch statements: an alternative to nested ifs
- 3.5.2.4. Switch on string statements
- 3.5.3. Iteration Statements
- 3.5.3.1. The goto statement
- 3.5.3.2. The while loop
- 3.5.3.3. The do...while loop
- 3.5.3.4. The for loop
- 3.5.3.5. The foreach statement
- 3.5.3.6. The continue and break statements
- 3.6. Operators
- 3.6.1. The Assignment Operator (=)
- 3.6.2. Mathematical Operators
- 3.6.2.1. Simple arithmetical operators (+, -, *, /)
- 3.6.2.2. The modulus operator (%) to return remainders
- 3.6.3. Increment and Decrement Operators
- 3.6.3.1. Calculate and reassign operators
- 3.6.3.2. The prefix and postfix operators
- 3.6.4. Relational Operators
- 3.6.5. Use of Logical Operators with Conditionals
- 3.6.6. Operator Precedence
- 3.6.7. The Ternary Operator
- 3.7. Preprocessor Directives
- 3.7.1. Defining Identifiers
- 3.7.2. Undefining Identifiers
- 3.7.3. #if, #elif, #else, and #endif
- 3.7.4. #region
- 3.1. Types
- 4. Classes and Objects
- 4.1. Defining Classes
- 4.1.1. Access Modifiers
- 4.1.2. Method Arguments
- 4.2. Creating Objects
- 4.2.1. Constructors
- 4.2.2. Initializers
- 4.2.3. The ICloneable Interface
- 4.2.4. The this Keyword
- 4.3. Using Static Members
- 4.3.1. Invoking Static Methods
- 4.3.2. Using Static Constructors
- 4.3.3. Static Classes
- 4.3.4. Using Static Fields
- 4.4. Destroying Objects
- 4.4.1. The C# Destructor
- 4.4.2. Destructors Versus Dispose
- 4.4.3. Implementing the Close( ) Method
- 4.4.4. The using Statement
- 4.5. Passing Parameters
- 4.5.1. Passing by Reference
- 4.5.2. Overcoming Definite Assignment with out Parameters
- 4.6. Overloading Methods and Constructors
- 4.7. Encapsulating Data with Properties
- 4.7.1. The get Accessor
- 4.7.2. The set Accessor
- 4.7.3. Property Access Modifiers
- 4.8. readonly Fields
- 4.1. Defining Classes
- 5. Inheritance and Polymorphism
- 5.1. Specialization and Generalization
- 5.2. Inheritance
- 5.2.1. Implementing Inheritance
- 5.3. Polymorphism
- 5.3.1. Creating Polymorphic Types
- 5.3.2. Creating Polymorphic Methods
- 5.3.3. Calling Base Class Constructors
- 5.3.4. Controlling Access
- 5.3.5. Versioning with the new and override Keywords
- 5.4. Abstract Classes
- 5.4.1. Limitations of Abstract
- 5.4.2. Sealed Class
- 5.5. The Root of All Classes: Object
- 5.6. Boxing and Unboxing Types
- 5.6.1. Boxing Is Implicit
- 5.6.2. Unboxing Must Be Explicit
- 5.7. Nesting Classes
- 6. Operator Overloading
- 6.1. Using the operator Keyword
- 6.2. Supporting Other .NET Languages
- 6.3. Creating Useful Operators
- 6.4. Logical Pairs
- 6.5. The Equality Operator
- 6.6. Conversion Operators
- 7. Structs
- 7.1. Defining Structs
- 7.2. Creating Structs
- 7.2.1. Structs as Value Types
- 7.2.2. Creating Structs Without new
- 8. Interfaces
- 8.1. Defining and Implementing an Interface
- 8.1.1. Implementing More Than One Interface
- 8.1.2. Extending Interfaces
- 8.1.3. Combining Interfaces
- 8.1.3.1. Casting to extended interfaces
- 8.2. Accessing Interface Methods
- 8.2.1. Casting to an Interface
- 8.2.2. The is Operator
- 8.2.3. The as Operator
- 8.2.4. The is Operator Versus the as Operator
- 8.2.5. Interface Versus Abstract Class
- 8.3. Overriding Interface Implementations
- 8.4. Explicit Interface Implementation
- 8.4.1. Selectively Exposing Interface Methods
- 8.4.2. Member Hiding
- 8.4.3. Accessing Sealed Classes and Value Types
- 8.1. Defining and Implementing an Interface
- 9. Arrays, Indexers, and Collections
- 9.1. Arrays
- 9.1.1. Declaring Arrays
- 9.1.2. Understanding Default Values
- 9.1.3. Accessing Array Elements
- 9.2. The foreach Statement
- 9.2.1. Initializing Array Elements
- 9.2.2. The params Keyword
- 9.2.3. Multidimensional Arrays
- 9.2.3.1. Rectangular arrays
- 9.2.3.2. Jagged arrays
- 9.2.4. Array Bounds
- 9.2.5. Array Conversions
- 9.2.6. Sorting Arrays
- 9.3. Indexers
- 9.3.1. Indexers and Assignment
- 9.3.2. Indexing on Other Values
- 9.4. Collection Interfaces
- 9.4.1. The IEnumerable<T> Interface
- 9.5. Constraints
- 9.6. List<T>
- 9.6.1. Implementing IComparable
- 9.6.2. Implementing IComparer
- 9.7. Queues
- 9.8. Stacks
- 9.9. Dictionaries
- 9.9.1. IDictionary<K,V>
- 9.1. Arrays
- 10. Strings and Regular Expressions
- 10.1. Strings
- 10.1.1. Creating Strings
- 10.1.2. The ToString() Method
- 10.1.3. Manipulating Strings
- 10.1.4. Finding Substrings
- 10.1.5. Splitting Strings
- 10.1.6. Manipulating Dynamic Strings
- 10.2. Regular Expressions
- 10.2.1. Using Regular Expressions: Regex
- 10.2.2. Using Regex Match Collections
- 10.2.3. Using Regex Groups
- 10.2.4. Using CaptureCollection
- 10.1. Strings
- 11. Handling Exceptions
- 11.1. Throwing and Catching Exceptions
- 11.1.1. The throw Statement
- 11.1.2. The catch Statement
- 11.1.2.1. Taking corrective action
- 11.1.2.2. Unwinding the call stack
- 11.1.2.3. Creating dedicated catch statements
- 11.1.3. The finally Statement
- 11.2. Exception Objects
- 11.3. Custom Exceptions
- 11.4. Rethrowing Exceptions
- 11.1. Throwing and Catching Exceptions
- 12. Delegates and Events
- 12.1. Delegates
- 12.1.1. Using Delegates to Specify Methods at Runtime
- 12.1.2. Delegates and Instance Methods
- 12.1.3. Static Delegates
- 12.1.4. Delegates as Properties
- 12.2. Multicasting
- 12.3. Events
- 12.3.1. Publishing and Subscribing
- 12.3.2. Events and Delegates
- 12.3.3. Solving Delegate Problems with Events
- 12.3.4. The event Keyword
- 12.4. Using Anonymous Methods
- 12.5. Retrieving Values from Multicast Delegates
- 12.5.1. Invoking Events Asynchronously
- 12.5.2. Callback Methods
- 12.1. Delegates
- 1. C# and the .NET Framework
- II. Programming with C#
- 13. Building Windows Applications
- 13.1. Creating a Simple Windows Form
- 13.1.1. Using the Visual Studio Designer
- 13.2. Creating a Windows Forms Application
- 13.2.1. Creating the Basic UI Form
- 13.2.2. Populating the TreeView Controls
- 13.2.2.1. TreeNode objects
- 13.2.2.2. Recursing through the subdirectories
- 13.2.2.3. Getting the files in the directory
- 13.2.3. Handling TreeView Events
- 13.2.3.1. Clicking the source TreeView
- 13.2.3.2. Expanding a directory
- 13.2.3.3. Clicking the target TreeView
- 13.2.3.4. Handling the Clear button event
- 13.2.4. Implementing the Copy Button Event
- 13.2.4.1. Getting the selected files
- 13.2.4.2. Sorting the list of selected files
- 13.2.5. Handling the Delete Button Event
- 13.3. XML Documentation Comments
- 13.1. Creating a Simple Windows Form
- 14. Accessing Data with ADO.NET
- 14.1. Relational Databases and SQL
- 14.1.1. Tables, Records, and Columns
- 14.1.2. Normalization
- 14.1.3. Declarative Referential Integrity
- 14.1.4. SQL
- 14.2. The ADO.NET Object Model
- 14.2.1. DataTables and DataColumns
- 14.2.2. DataRelations
- 14.2.3. Rows
- 14.2.4. Data Adapter
- 14.2.5. DBCommand and DBConnection
- 14.2.6. DataAdapter
- 14.2.7. DataReader
- 14.3. Getting Started with ADO.NET
- 14.4. Using OLE DB Managed Providers
- 14.5. Working with Data-Bound Controls
- 14.5.1. Populating a DataGrid Programmatically
- 14.5.2. Customizing the DataSet
- 14.1. Relational Databases and SQL
- 15. Programming ASP.NET Applications and Web Services
- 15.1. Understanding Web Forms
- 15.1.1. Web Form Events
- 15.1.1.1. Postback versus nonpostback events
- 15.1.1.2. State
- 15.1.2. Web Form Life Cycle
- 15.1.1. Web Form Events
- 15.2. Creating a Web Form
- 15.2.1. Code-Behind Files
- 15.3. Adding Controls
- 15.3.1. Server Controls
- 15.4. Data Binding
- 15.4.1. Examining the Code
- 15.4.2. Adding Controls and Events
- 15.5. Web Services
- 15.6. SOAP, WSDL, and Discovery
- 15.6.1. Server-Side Support
- 15.6.2. Client-Side Support
- 15.7. Building a Web Service
- 15.7.1. Testing Your Web Service
- 15.7.2. Viewing the WSDL Contract
- 15.8. Creating the Proxy
- 15.8.1. Testing the Web Service
- 15.1. Understanding Web Forms
- 16. Putting It All Together
- 16.1. The Overall Design
- 16.2. Creating the Web Services Client
- 16.2.1. Creating the Amazon proxy
- 16.2.1.1. Creating the desktop application
- 16.2.1. Creating the Amazon proxy
- 16.3. Displaying the Output
- 16.3.1. Implementing the Grid
- 16.3.2. Handling the RowDataBound Event
- 16.4. Searching by Category
- 13. Building Windows Applications
- III. The CLR and the .NET Framework
- 17. Assemblies and Versioning
- 17.1. PE Files
- 17.2. Metadata
- 17.3. Security Boundary
- 17.4. Manifests
- 17.5. Multimodule Assemblies
- 17.5.1. Building a Multimodule Assembly
- 17.5.1.1. Testing the assembly
- 17.5.1.2. Loading the assembly
- 17.5.1. Building a Multimodule Assembly
- 17.6. Private Assemblies
- 17.7. Shared Assemblies
- 17.7.1. The End of DLL Hell
- 17.7.2. Versions
- 17.7.3. Strong Names
- 17.7.4. The Global Assembly Cache
- 17.7.5. Building a Shared Assembly
- 17.7.5.1. Step 1: Create a strong name
- 17.7.5.2. Step 2: Put the shared assembly in the GAC
- 17.7.6. Other Required Assemblies
- 18. Attributes and Reflection
- 18.1. Attributes
- 18.1.1. Attributes
- 18.1.1.1. Attribute targets
- 18.1.1.2. Applying attributes
- 18.1.2. Custom Attributes
- 18.1.2.1. Declaring an attribute
- 18.1.2.2. Naming an attribute
- 18.1.2.3. Constructing an attribute
- 18.1.2.4. Using an attribute
- 18.1.1. Attributes
- 18.2. Reflection
- 18.2.1. Viewing Metadata
- 18.2.2. Type Discovery
- 18.2.3. Reflecting on a Type
- 18.2.3.1. Finding all type members
- 18.2.3.2. Finding type methods
- 18.2.3.3. Finding particular type members
- 18.2.4. Late Binding
- 18.1. Attributes
- 19. Marshaling and Remoting
- 19.1. Application Domains
- 19.1.1. Creating and Using App Domains
- 19.1.2. Marshaling Across App Domain Boundaries
- 19.1.2.1. Understanding marshaling with proxies
- 19.1.2.2. Specifying the marshaling method
- 19.2. Context
- 19.2.1. Context-Bound and Context-Agile Objects
- 19.2.2. Marshaling Across Context Boundaries
- 19.3. Remoting
- 19.3.1. Understanding Server Object Types
- 19.3.2. Specifying a Server with an Interface
- 19.3.3. Building a Server
- 19.3.4. Building the Client
- 19.3.5. Using SingleCall
- 19.3.6. Understanding RegisterWellKnownServiceType
- 19.3.7. Understanding Endpoints
- 19.1. Application Domains
- 20. Threads and Synchronization
- 20.1. Threads
- 20.1.1. Starting Threads
- 20.1.2. Joining Threads
- 20.1.3. Blocking Threads with Sleep
- 20.1.4. Killing Threads
- 20.2. Synchronization
- 20.2.1. Using Interlocked
- 20.2.2. Using Locks
- 20.2.3. Using Monitors
- 20.3. Race Conditions and Deadlocks
- 20.3.1. Race Conditions
- 20.3.2. Deadlock
- 20.1. Threads
- 21. Streams
- 21.1. Files and Directories
- 21.1.1. Working with Directories
- 21.1.2. Creating a DirectoryInfo Object
- 21.1.3. Working with Files
- 21.1.4. Modifying Files
- 21.2. Reading and Writing Data
- 21.2.1. Binary Files
- 21.2.2. Buffered Streams
- 21.2.3. Working with Text Files
- 21.3. Asynchronous I/O
- 21.4. Network I/O
- 21.4.1. Creating a Network Streaming Server
- 21.4.2. Creating a Streaming Network Client
- 21.4.3. Handling Multiple Connections
- 21.4.4. Asynchronous Network File Streaming
- 21.5. Web Streams
- 21.6. Serialization
- 21.6.1. Using a Formatter
- 21.6.2. Working with Serialization
- 21.6.2.1. Serializing the object
- 21.6.2.2. Deserializing the object
- 21.6.3. Handling Transient Data
- 21.7. Isolated Storage
- 21.1. Files and Directories
- 22. Programming .NET and COM
- 22.1. Importing ActiveX Controls
- 22.1.1. Creating an ActiveX Control
- 22.1.2. Importing a Control in .NET
- 22.1.2.1. Importing a control
- 22.1.2.2. Manually importing the control
- 22.1.2.3. Adding the control to the form
- 22.2. Importing COM Components
- 22.2.1. Coding the COMTestForm Program
- 22.2.2. Importing the COM .DLL to .NET
- 22.2.3. Importing the Type Library
- 22.2.4. Importing Manually
- 22.2.5. Creating a Test Program
- 22.2.6. Using Late Binding and Reflection
- 22.3. Exporting .NET Components
- 22.3.1. Creating a Type Library
- 22.4. P/Invoke
- 22.5. Pointers
- 22.1. Importing ActiveX Controls
- 17. Assemblies and Versioning
- A. C# Keywords
- Index
- Colophon
- SPECIAL OFFER: Upgrade this ebook with OReilly