Learning iOS Programming. From Xcode to App Store. 3rd Edition - Helion
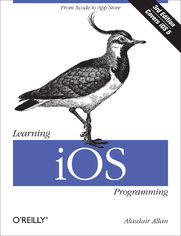
ISBN: 978-14-493-5938-6
stron: 452, Format: ebook
Data wydania: 2013-03-12
Księgarnia: Helion
Cena książki: 101,15 zł (poprzednio: 117,62 zł)
Oszczędzasz: 14% (-16,47 zł)
Get a rapid introduction to iPhone, iPad, and iPod touch programming. With this easy-to-follow guide, you’ll learn how to develop your first marketable iOS application, from opening Xcode to submitting your product to the App Store. Whether you’re a developer new to Mac programming or an experienced Mac developer ready to tackle iOS, this is your book.
You’ll learn about Objective-C and the core frameworks hands-on by writing several sample iOS applications, giving you the basic skills for building your own applications independently. Packed with code samples, this book is refreshed and updated for iOS 6 and Xcode 4.
- Discover the advantages of building native iOS apps
- Get started with Objective-C and the Cocoa Touch frameworks
- Dive deep into the table view classes for building user interfaces
- Handle data input, parse XML and JSON documents, and store data on SQLite
- Use iOS sensors, including the accelerometer, magnetometer, camera, and GPS
- Build apps that use the Core Location and MapKit frameworks
- Integrate Apple’s iCloud service into your applications
- Walk through the process of distributing your polished app to the App Store
Osoby które kupowały "Learning iOS Programming. From Xcode to App Store. 3rd Edition", wybierały także:
- Zen Steve'a Jobsa 29,67 zł, (8,90 zł -70%)
- Programowanie aplikacji mobilnych dla iOS z wykorzystaniem Xcode, Swift 3.0 i iOS 10 SDK. Kurs video. Poziom pierwszy 99,00 zł, (44,55 zł -55%)
- Podstawy języka Swift. Programowanie aplikacji dla platformy iOS 49,00 zł, (26,95 zł -45%)
- Dotknij, przesuń, potrząśnij. Od pomysłu do gry na iPhone'a i iPada 39,00 zł, (21,45 zł -45%)
- Flutter i Dart 2 dla początkujących. Przewodnik dla twórców aplikacji mobilnych 87,41 zł, (48,95 zł -44%)
Spis treści
Learning iOS Programming. From Xcode to App Store. 3rd Edition eBook -- spis treści
- Learning iOS Programming
- Preface
- Third Edition
- Notes from the Second Edition
- Who Should Read This Book?
- What Should You Already Know?
- What Will You Learn?
- Whats in This Book?
- Conventions Used in This Book
- Using Code Examples
- How to Contact Us
- Safari Books Online
- Acknowledgments
- 1. Why Go Native?
- The Pros and Cons
- Why Write Native Applications?
- The Release Cycle
- Build It and They Will Come
- The Pros and Cons
- 2. Becoming a Developer
- Registering as an iOS Developer
- Enrolling in the iOS Developer Program
- The Mac Developer Program
- Installing the iOS SDK
- What Happens When There Is a Beta?
- Preparing Your iOS Device
- Creating a Development Certificate
- Getting the UDID of Your Development Device
- Creating an App ID
- Creating a Mobile Provisioning Profile
- Making Your Device Available for Development
- 3. Your First iOS App
- Objective-C Basics
- Object-Oriented Programming
- The Objective-C Object Model
- The Basics of Objective-C Syntax
- Creating a Project
- Exploring the Project in Xcode
- Overview of an iPhone application
- The application delegate
- The view controller
- Our Project in Interface Builder
- Building the User Interface
- Connecting the User Interface to the Code
- Running the Application in the Simulator
- Putting the Application on Your iPhone
- Exploring the Project in Xcode
- Objective-C Basics
- 4. Coding in Objective-C
- Declaring and Defining Classes
- Declaring a Class with the Interface
- Defining a Class with the Implementation
- Object Typing
- Properties
- Synthesizing Properties
- The Dot Syntax
- Declaring Methods
- Calling Methods
- Calling Methods on nil
- Memory Management
- Creating Objects
- The Autorelease Pool
- The alloc, retain, copy, and release Cycle
- Automatic Reference Counting
- The dealloc Method
- Responding to Memory Warnings
- Fundamental iOS Design Patterns
- The Model-View-Controller Pattern
- Views and View Controllers
- The Delegates and DataSource Pattern
- Conclusion
- Declaring and Defining Classes
- 5. Table View-Based Applications
- Creating the Project
- Creating a Table View
- Running the Code
- Populating the Table View
- Building a Model
- Adding Cities to the Guide
- Adding Images to Your Projects
- Connecting the Controller to the Model
- Mocking Up Functionality with Alert Windows
- Adding Navigation Controls to the Application
- Adding a City View
- Edit Mode
- Deleting a City Entry
- Adding a City Entry
- The Add New City Interface
- Capturing the City Data
- 6. Other View Controllers
- Utility Applications
- Making the Battery Monitoring Application
- Building the interface
- Writing the code
- Making the Battery Monitoring Application
- Tab Bar Applications
- Adding Another Tab Bar Item
- Combining View Controllers
- Modal View Controllers
- Modifying the City Guide Application
- The Image Picker View Controller
- Adding the Image Picker to the City Guide Application
- Master-Detail Applications
- Creating a Universal Application
- Popover Controllers
- Utility Applications
- 7. Connecting to the Network
- Detecting Network Status
- Apples Reachability Class
- Reusing the Reachability class
- Synchronous Reachability
- Asynchronous reachability
- Using Reachability directly
- Updating the Reachability project
- Apples Reachability Class
- Embedding a Web Browser in Your App
- A Simple Web View Controller
- Displaying Static HTML Files
- Getting Data Out of a UIWebView
- Sending Email
- Getting Data from the Internet
- Synchronous Requests
- Asynchronous Requests
- Using Web Services
- The Weather Underground service
- Building an application
- Parsing the XML document
- Populating the UI
- Tidying up
- Detecting Network Status
- 8. Handling Data
- Data Entry
- UITextField and Its Delegate
- UITextView and Its Delegate
- Dismissing the UITextView
- Parsing XML
- Parsing XML with libxml2
- Parsing XML with NSXMLParser
- Parsing JSON
- NSJSONSerialization
- Parsing JSON documents
- Creating JSON documents
- The JSON Framework
- Retrieving Twitter Trends
- Using the Social Framework
- The Twitter Trends Application
- Retrieving the trends
- Building a UI
- Parsing the JSON document
- Tidying up
- NSJSONSerialization
- Regular Expressions
- Introduction to Regular Expressions
- NSRegularExpression
- RegexKitLite
- Faking regex support with the built-in NSPredicate
- Introduction to Regular Expressions
- Storing Data
- Using Flat Files
- Reading and writing text content
- Creating temporary files
- Other file manipulation
- Storing Information in a SQL Database
- Adding a database to your project
- Data persistence for the City Guide application
- Refactoring and rethinking
- Core Data
- Using Flat Files
- Data Entry
- 9. Using Sensors
- Hardware Support
- Network Availability
- Camera Availability
- Audio Input Availability
- GPS Availability
- Magnetometer Availability
- Setting Required Hardware Capabilities
- Persistent WiFi
- Background Modes
- Differences Between iPhone and iPad
- Using the Camera
- The Core Motion Framework
- Pulling Motion Data
- Pushing Motion Data
- The Accelerometer
- Using the accelerometer directly
- The Gyroscope
- Using the gyroscope directly
- The Magnetometer
- Using the magnetometer directly
- Accessing the Proximity Sensor
- Using Vibration
- Hardware Support
- 10. Geolocation and Mapping
- The Core Location Framework
- Device Heading
- Location-Dependent Weather
- Reverse Geocoding
- Forward Geocoding
- CLPlacemark Objects
- Modifying the Weather Application
- User Location and MapKit
- Annotating Maps
- The Core Location Framework
- 11. Introduction to iCloud
- How Can I Use iCloud?
- iCloud Backup
- Provisioning Your Application for iCloud
- Using Key-Value Storage
- Wrapping Up
- How Can I Use iCloud?
- 12. Integrating Your Application
- Application Preferences
- The Accounts Framework
- The Social Framework
- Sending Tweets
- Making Posts to Facebook
- Custom URL Schemes
- Using Custom Schemes
- Making a telephone call
- Opening the Settings application
- Registering Custom Schemes
- Using Custom Schemes
- Media Playback
- Using the Address Book
- Interactive People Picking
- Programmatic People Picking
- Sending Text Messages
- 13. Distributing Your Application
- Adding Missing Features
- Adding an Icon
- Adding a Launch Image
- Changing the Display Name
- Enabling Rotation
- Building and Signing
- Ad Hoc Distribution
- Obtaining a distribution certificate
- Registering devices
- Creating a provisioning profile
- Building your application for ad hoc distribution
- Distributing an ad hoc build
- Developer-to-Developer Distribution
- App Store Distribution
- Ad Hoc Distribution
- Submitting to the App Store
- Building Your Application for App Store Distribution
- The App Store Resource Center
- Reasons for Rejection
- Adding Missing Features
- 14. Going Further
- Cocoa and Objective-C
- The iOS SDK
- Web Applications
- PhoneGap
- Core Data
- In-App Purchase
- MKStoreKit
- Core Animation
- Game Kit
- Writing Games
- Look and Feel
- Hardware Accessories
- Cocoa and Objective-C
- Index
- About the Author
- Colophon
- Copyright