Learning Vue - Helion
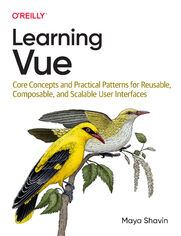
ISBN: 9781492098782
stron: 350, Format: ebook
Data wydania: 2023-12-01
Księgarnia: Helion
Cena książki: 203,15 zł (poprzednio: 236,22 zł)
Oszczędzasz: 14% (-33,07 zł)
Learn the core concepts of Vue.js, the modern JavaScript framework for building frontend applications and interfaces from scratch. With concise, practical, and clear examples, this book takes web developers step-by-step through the tools and libraries in the Vue.js ecosystem and shows them how to create complete applications for real-world web projects.
You’ll learn how to handle data communication between components with Pinia architecture, develop a manageable routing system for a frontend project to control the application flow, and produce basic animation effects to create a better user experience.
This book also shows you how to:
- Create reusable and lightweight component systems using Vue.js
- Bring reactivity to your existing static application
- Set up a project using Vite.js, a build tool for frontend project code management
- Build an interactive state management system for a frontend application with Pinia
- Connect external data from the server to your Vue application
- Control the application flow with static and dynamic routing using Vue Router
- Fully test your application using Vitest and Playwright
Osoby które kupowały "Learning Vue", wybierały także:
- Windows Media Center. Domowe centrum rozrywki 66,67 zł, (8,00 zł -88%)
- Ruby on Rails. Ćwiczenia 18,75 zł, (3,00 zł -84%)
- DevOps w praktyce. Kurs video. Jenkins, Ansible, Terraform i Docker 190,00 zł, (39,90 zł -79%)
- Przywództwo w świecie VUCA. Jak być skutecznym liderem w niepewnym środowisku 58,64 zł, (12,90 zł -78%)
- Scrum. O zwinnym zarządzaniu projektami. Wydanie II rozszerzone 58,64 zł, (12,90 zł -78%)
Spis treści
Learning Vue eBook -- spis treści
- Preface
- Conventions Used in This Book
- Using Code Examples
- OReilly Online Learning
- How to Contact Us
- Acknowledgments
- 1. Welcome to the Vue.js World!
- What Is Vue.js?
- The Benefits of Vue in Modern Web Development
- Installing Node.js
- NPM
- Yarn
- Vue Developer Tools
- Vite.js as a Builder Management Tool
- Create a New Vue Application
- File Repository Structure
- Summary
- 2. How Vue Works: The Basics
- Virtual DOM Under the Hood
- The Layout Update Problem
- What Is Virtual DOM?
- How Virtual DOM Works in Vue
- The Vue App Instance and Options API
- Exploring the Options API
- The Template Syntax
- Creating Local State with Data Properties
- How Reactivity in Vue Works
- Two-Way Binding with v-model
- Using v-model.lazy Modifier
- Binding Reactive Data and Passing Props Data with v-bind
- Binding to Class and Style Attributes
- Iterating over Data Collection Using v-for
- Iterating Through Object Properties
- Make the Element Binding Unique with Key Attribute
- Adding Event Listener to Elements with v-on
- Handling Events with v-on Event Modifiers
- Detecting Keyboard Events with Key Code Modifiers
- Conditional Rendering Elements with v-if, v-else, and v-else-if
- Conditional Displaying Elements with v-show
- Dynamically Displaying HTML Code with v-html
- Displaying Text Content with v-text
- Optimizing Renders with v-once and v-memo
- Registering a Component Globally
- Summary
- Virtual DOM Under the Hood
- 3. Composing Components
- Vue Single File Component Structure
- Using defineComponent() for TypeScript Support
- Component Lifecycle Hooks
- setup
- beforeCreate
- created
- beforeMount
- mounted
- beforeUpdate
- updated
- beforeUnmount
- unmounted
- Methods
- Computed Properties
- Watchers
- Observing for Changes in Nested Properties
- Using the this.$watch() Method
- The Power of Slots
- Using Named Slots with Template Tag and v-slot Attribute
- Understanding Refs
- Sharing Component Configuration with Mixins
- Scoped Styling Components
- Applying CSS to a Child Component in Scoped Styles
- Applying Scoped Styles to Slot Content
- Accessing a Components Data Value in Style Tag with v-bind() Pseudo-Class
- Styling Components with CSS Modules
- Summary
- 4. Interactions Between Components
- Nested Components and Data Flow in Vue
- Using Props to Pass Data to Child Components
- Declaring Prop Types with Validation and Default Values
- Declaring Props with Custom Type Checking
- Declaring Props Using defineProps() and withDefaults()
- Communication Between Components with Custom Events
- Defining Custom Events Using defineEmits()
- Communicate Between Components with provide/inject Pattern
- Using provide to Pass Data
- Using inject to Receive Data
- Teleport API
- Implementing a Modal with Teleport and the <dialog> Element
- Rendering Problem Using Teleport
- Summary
- Nested Components and Data Flow in Vue
- 5. Composition API
- Setting Up Components with Composition API
- Handling Data with ref() and reactive()
- Using ref()
- Using reactive()
- Using the Lifecycle Hooks
- Understanding Watchers in Composition API
- Using computed()
- Creating Your Reusable Composables
- Summary
- 6. Incorporating External Data
- What Is Axios?
- Installing Axios
- Load Data with Lifecycle Hooks and Axios
- Async Data Requests in Run-Time: the Challenge
- Creating Your Reusable Fetch Component
- Connect Your Application with an External Database
- Summary
- 7. Advanced Rendering, Dynamic Components, and Plugin Composition
- The Render Function and JSX
- Using the Render Function
- Using the h Function to Create a VNode
- Writing JavaScript XML in the Render Function
- Functional Component
- Defining Props and Emits for Functional Component
- Adding Custom Functionality Globally with Vue Plugins
- Dynamic Rendering with the <component> Tag
- Keeping Component Instance Alive with <keep-alive>
- Summary
- The Render Function and JSX
- 8. Routing
- What is Routing?
- Using Vue Router
- Installing Vue Router
- Defining Routes
- Creating a Router Instance
- Plugging the Router Instance Into the Vue Application
- Rendering the Current Page with the RouterView Component
- Build a Navigation Bar with the RouterLink Component
- Passing Data Between Routes
- Decoupling Route Parameters Using Props
- Understanding Navigation Guards
- Global Navigation Guards
- Route-Level Navigation Guards
- Component-Level Router Guards
- Creating Nesting Routes
- Creating Dynamic Routes
- Going Back and Forward with the Router Instance
- Handling Unknown Routes
- Summary
- 9. State Management with Pinia
- Understanding State Management in Vue
- Understanding Pinia
- Creating a Pizzas Store for Pizza House
- Creating a Cart Store for Pizza House
- Using the Cart Store in a Component
- Adding Items to the Cart from the Pizzas Gallery
- Displaying Cart Items with Actions
- Removing Items from the Cart Store
- Unit Testing Pinia Stores
- Subscribing Side Effects on Store Changes
- Summary
- 10. Transitioning and Animation in Vue
- Understanding CSS Transitions and CSS Animations
- Transition Component in Vue.js
- Using Custom Transition Class Attributes
- Adding Transition Effect on the Initial Render with appear
- Building Transition for a Group of Elements
- Creating Route Transitions
- Using Transition Events to Control Animation
- Summary
- 11. Testing in Vue
- Introduction to Unit Testing and E2E Testing
- Vitest as a Unit Testing Tool
- Configuring Vitest Using Parameters and Config File
- Writing Your First Test
- Testing Non-Lifecycle Composables
- Testing Composables with Lifecycle Hook
- Testing Components Using Vue Test Utils
- Testing Interaction and Events of a Component
- Using Vitest with a GUI
- Using Vitest with a Coverage Runner
- End-to-End Testing with PlaywrightJS
- Debugging E2E Tests Using Playwright Test Extension for VSCode
- Summary
- 12. Continuous Integration/Continuous Deployment of Vue.Js Applications
- CI/CD in Software Development
- Continuous Integration
- Continuous Delivery
- Continuous Deployment
- CI/CD Pipeline with GitHub Actions
- Continuous Deployment with Netlify
- Deploying with Netlify CLI
- Summary
- CI/CD in Software Development
- 13. Server-Side Rendering with Vue
- Client-Side Rendering in Vue
- Server-Side Rendering (SSR)
- Server-Side Rendering with Nuxt.Js
- Static Side Generator (SSG)
- Last Words
- Index