Learning C# 3.0 - Helion
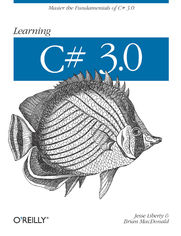
ISBN: 978-05-965-5420-0
stron: 696, Format: ebook
Data wydania: 2008-11-18
Księgarnia: Helion
Cena książki: 118,15 zł (poprzednio: 137,38 zł)
Oszczędzasz: 14% (-19,23 zł)
If you're new to C#, this popular book is the ideal way to get started. Completely revised for the latest version of the language, Learning C# 3.0 starts with the fundamentals and takes you through intermediate and advanced C# features -- including generics, interfaces, delegates, lambda expressions, and LINQ. You'll also learn how to build Windows applications and handle data with C#.
No previous programming experience is required -- in fact, if you've never written a line of code in your life, bestselling authors Jesse Liberty and Brian MacDonald will show you how it's done. Each chapter offers a self-contained lesson to help you master key concepts, with plenty of annotated examples, illustrations, and a concise summary.
With this book, you will:
- Learn how to program as you learn C#
- Grasp the principles of object-oriented programming through C#
- Discover how to use the latest features in C# 3.0 and the .NET 3.5 Framework--including LINQ and the Windows Presentation Foundation (WPF)
- Create Windows applications and data-driven applications
You'll also find a unique Test Your Knowledge section in each chapter, with practical exercises and review quizzes, so you can practice new skills and test your understanding. If you're ready to dive into C# and .NET programming, this book is a great way to quickly get up to speed.
Osoby które kupowały "Learning C# 3.0", wybierały także:
- Biologika Sukcesji Pokoleniowej. Sezon 3. Konflikty na terytorium 124,17 zł, (14,90 zł -88%)
- Windows Media Center. Domowe centrum rozrywki 66,67 zł, (8,00 zł -88%)
- Podręcznik startupu. Budowa wielkiej firmy krok po kroku 93,13 zł, (14,90 zł -84%)
- Ruby on Rails. Ćwiczenia 18,75 zł, (3,00 zł -84%)
- Scrum. O zwinnym zarz 78,42 zł, (14,90 zł -81%)
Spis treści
Learning C# 3.0. Master the fundamentals of C# 3.0 eBook -- spis treści
- Learning C# 3.0
- SPECIAL OFFER: Upgrade this ebook with OReilly
- A Note Regarding Supplemental Files
- Preface
- About This Book
- Who This Book Is For
- How This Book Is Organized
- Conventions Used in This Book
- Support: A Note from Jesse Liberty
- Using Code Examples
- Wed Like to Hear from You
- Safari Books Online
- Acknowledgments
- Jesse Liberty
- Brian MacDonald
- 1. C# and .NET Programming
- Installing C# Express
- C# 3.0 and .NET 3.5
- The .NET Platform
- The .NET Framework
- The C# Language
- Your First Program: Hello World
- The Compiler
- Examining Your First Program
- The Integrated Development Environment
- Summary
- Test Your Knowledge: Quiz
- Test Your Knowledge: Exercise
- 2. Visual Studio 2008 and C# Express 2008
- Before You Read Further
- The Start Page
- Projects and Solutions
- Project Types
- Templates
- Inside the Integrated Development Environment
- Building and Running Applications
- Menus
- The File Menu
- The Edit Menu
- The Clipboard Ring
- Find and Replace
- Go To
- Insert File As Text
- Advanced
- Incremental search
- Bookmarks
- Outlining
- IntelliSense
- The View Menu
- Class View
- Code Definition
- Error List
- Output
- Properties
- Task List
- Toolbox
- Other Windows
- The Refactor Menu
- The Project Menu
- The Build Menu
- The Debug Menu
- The Data Menu
- The Format Menu
- The Tools Menu
- Connect to Device
- Device Emulator Manager
- Connect to Database
- Connect to Server
- Code Snippets Manager
- Choose Toolbox Items
- External Tools
- Import and Export Settings
- Options
- The Window Menu
- The Help Menu
- Summary
- Test Your Knowledge: Quiz
- Test Your Knowledge: Exercises
- 3. C# Language Fundamentals
- Statements
- Types
- Numeric Types
- Nonnumeric Types: char and bool
- Types and Compiler Errors
- WriteLine( ) and Output
- Variables and Assignment
- Definite Assignment
- Implicitly Typed Variables
- Casting
- Constants
- Literal Constants
- Symbolic Constants
- Enumerations
- Strings
- Whitespace
- Summary
- Test Your Knowledge: Quiz
- Test Your Knowledge: Exercises
- 4. Operators
- Expressions
- The Assignment Operator (=)
- Mathematical Operators
- Simple Arithmetic Operators (+, , *, /)
- The Modulus Operator (%)
- Increment and Decrement Operators
- The Calculate and Reassign Operators
- Increment or Decrement by 1
- The Prefix and Postfix Operators
- Relational Operators
- Logical Operators and Conditionals
- The Conditional Operator
- Operator Precedence
- Summary
- Test Your Knowledge: Quiz
- Test Your Knowledge: Exercises
- 5. Branching
- Unconditional Branching Statements
- Conditional Branching Statements
- if Statements
- Single-Statement if Blocks
- Short-Circuit Evaluation
- if . . . else Statements
- Nested if Statements
- switch Statements
- Fall-Through and Jump-to Cases
- Switch on string Statements
- ReadLine( ) and Input
- Iteration (Looping) Statements
- Creating Loops with goto
- The while Loop
- The dowhile Loop
- The for Loop
- Controlling a for loop with the modulus operator
- Breaking out of a for loop
- The continue statement
- Optional for loop header elements
- Summary
- Test Your Knowledge: Quiz
- Test Your Knowledge: Exercises
- 6. Object-Oriented Programming
- Creating Models
- Classes and Objects
- Defining a Class
- Class Relationships
- The Three Pillars of Object-Oriented Programming
- Encapsulation
- Specialization
- Polymorphism
- Object-Oriented Analysis and Design
- Summary
- Test Your Knowledge: Quiz
- Test Your Knowledge: Exercises
- 7. Classes and Objects
- Defining Classes
- Instantiating Objects
- Creating a Box Class
- Access Modifiers
- Method Arguments
- Return Types
- Constructors
- Initializers
- Object Initializers
- Anonymous Types
- The this Keyword
- Static and Instance Members
- Invoking Static Methods
- Using Static Fields
- Finalizing Objects
- Memory Allocation: The Stack Versus the Heap
- Summary
- Test Your Knowledge: Quiz
- Test Your Knowledge: Exercises
- Defining Classes
- 8. Inside Methods
- Overloading Methods
- Encapsulating Data with Properties
- The get Accessor
- The set Accessor
- Automatic Properties
- Returning Multiple Values
- Passing Value Types by Reference
- out Parameters and Definite Assignment
- Summary
- Test Your Knowledge: Quiz
- Test Your Knowledge: Exercises
- 9. Basic Debugging
- Setting a Breakpoint
- Using the Debug Menu to Set Your Breakpoint
- Setting Conditions and Hit Counts
- Examining Values: The Autos and Locals Windows
- Setting Your Watch
- The Call Stack
- Stopping Debugging
- Summary
- Test Your Knowledge: Quiz
- Test Your Knowledge: Exercises
- Setting a Breakpoint
- 10. Arrays
- Using Arrays
- Declaring Arrays
- Understanding Default Values
- Accessing Array Elements
- Arrays and Loops
- The foreach Statement
- Initializing Array Elements
- The params Keyword
- Multidimensional Arrays
- Rectangular Arrays
- Jagged Arrays
- Array Methods
- Sorting Arrays
- Summary
- Test Your Knowledge: Quiz
- Test Your Knowledge: Exercises
- Using Arrays
- 11. Inheritance and Polymorphism
- Specialization and Generalization
- Inheritance
- Implementing Inheritance
- Calling the Base Class Constructor
- Hiding the Base Class Method
- Controlling Access
- Polymorphism
- Creating Polymorphic Types
- Overriding Virtual Methods
- Using Objects Polymorphically
- Versioning with new and override
- Abstract Classes
- Sealed Classes
- The Root of All Classes: Object
- Summary
- Test Your Knowlege: Quiz
- Test Your Knowledge: Exercises
- 12. Operator Overloading
- Designing the Fraction Class
- Using the operator Keyword
- Creating Useful Operators
- The Equals Operator
- Conversion Operators
- Summary
- Test Your Knowledge: Quiz
- Test Your Knowledge: Exercises
- 13. Interfaces
- What Interfaces Are
- Implementing an Interface
- Defining the Interface
- Implementing the Interface on the Client
- Implementing More Than One Interface
- Casting to an Interface
- The is and as Operators
- Extending Interfaces
- Combining Interfaces
- Overriding Interface Methods
- Explicit Interface Implementation
- Summary
- Test Your Knowledge: Quiz
- Test Your Knowledge: Exercises
- 14. Generics and Collections
- Generics
- Collection Interfaces
- Creating Your Own Collections
- Creating Indexers
- Indexers and Assignment
- Indexing on Other Values
- Generic Collection Interfaces
- The IEnumerable<T> Interface
- Framework Generic Collections
- Generic Lists: List<T>
- Sorting objects with the generic list
- Controlling sorting by implementing IComparer<T>
- Generic Queues
- Generic Stacks
- Dictionaries
- Generic Lists: List<T>
- Summary
- Test Your Knowledge: Quiz
- Test Your Knowledge: Exercises
- 15. Strings
- Creating Strings
- String Literals
- Escape Characters
- Verbatim Strings
- The ToString( ) Method
- Manipulating Strings
- Comparing Strings
- Concatenating Strings
- Copying Strings
- Testing for Equality
- Other Useful String Methods
- Finding Substrings
- Splitting Strings
- The StringBuilder Class
- Regular Expressions
- The Regex Class
- Summary
- Test Your Knowledge: Quiz
- Test Your Knowledge: Exercises
- Creating Strings
- 16. Throwing and Catching Exceptions
- Bugs, Errors, and Exceptions
- Throwing Exceptions
- Searching for an Exception Handler
- The throw Statement
- The try and catch Statements
- How the Call Stack Works
- Creating Dedicated catch Statements
- The finally Statement
- Exception Class Methods and Properties
- Custom Exceptions
- Summary
- Test Your Knowledge: Quiz
- Test Your Knowledge: Exercises
- 17. Delegates and Events
- Delegates
- Events
- Publishing and Subscribing
- Events and Delegates
- Solving Delegate Problems with Events
- The event Keyword
- Using Anonymous Methods
- Lambda Expressions
- Summary
- Test Your Knowledge: Quiz
- Test Your Knowledge: Exercises
- Delegates
- 18. Creating Windows Applications
- Creating a Simple Windows Form
- Using the Visual Studio Designer
- Creating a Real-World Application
- Creating the Basic UI Form
- Populating the TreeView Controls
- TreeNode objects
- Recursing through the subdirectories
- Getting the files in the directory
- Handling the TreeView Events
- Clicking the source TreeView
- Expanding a directory
- Clicking the target TreeView
- Handling the Button Events
- Handling the Clear button event
- Implementing the Copy button event
- Handling the Delete button event
- Handling the Cancel button event
- Source Code
- Summary
- Test Your Knowledge: Quiz
- Test Your Knowledge: Exercises
- Creating a Simple Windows Form
- 19. Windows Presentation Foundation
- Your First WPF Application
- WPF Differences from Windows Forms
- Using Resources
- Animations
- Triggers and Storyboards
- Animations As Resources
- C# and WPF
- Grids and Stack Panels
- Defining ListBox styles
- Triggers and animations
- Adding Data
- Instantiating objects declaratively
- Using the Data in the XAML
- Defining the ListBox
- Event Handling
- The Complete XAML File
- Grids and Stack Panels
- Summary
- Test Your Knowledge: Quiz
- Test Your Knowledge: Exercises
- 20. ADO.NET and Relational Databases
- Relational Databases and SQL
- Installing the Northwind Database
- Tables, Records, and Columns
- Normalization
- Declarative Referential Integrity
- SQL
- The ADO.NET Object Model
- DataTables and DataColumns
- DataRelations
- Rows
- DataAdapter
- DbCommand and DbConnection
- DataReader
- Getting Started with ADO.NET
- Summary
- Test Your Knowledge: Quiz
- Test Your Knowledge: Exercises
- Relational Databases and SQL
- 21. LINQ
- Querying In-Memory Data
- Anonymous Types and Implicitly Typed Variables
- Lambda Expressions
- Ordering and Joining
- Using LINQ with SQL
- Using the Object Relational Designer
- Summary
- Test Your Knowledge: Quiz
- Test Your Knowledge: Exercises
- A. Answers to Quizzes and Exercises
- Chapter 1: C# and .NET Programming
- Quiz Solutions
- Exercise Solution
- Chapter 2: Visual Studio 2008 and C# Express 2008
- Quiz Solutions
- Exercise Solutions
- Chapter 3: C# Language Fundamentals
- Quiz Solutions
- Exercise Solutions
- Chapter 4: Operators
- Quiz Solutions
- Exercise Solutions
- Chapter 5: Branching
- Quiz Solutions
- Exercise Solutions
- Chapter 6: Object-Oriented Programming
- Quiz Solutions
- Exercise Solutions
- Chapter 7: Classes and Objects
- Quiz Solutions
- Exercise Solutions
- Chapter 8: Inside Methods
- Quiz Solutions
- Exercise Solutions
- Chapter 9: Basic Debugging
- Quiz Solutions
- Exercise Solutions
- Chapter 10: Arrays
- Quiz Solutions
- Exercise Solutions
- Chapter 11: Inheritance and Polymorphism
- Quiz Solutions
- Exercise Solutions
- Chapter 12: Operator Overloading
- Quiz Solutions
- Exercise Solutions
- Chapter 13: Interfaces
- Quiz Solutions
- Exercise Solutions
- Chapter 14: Generics and Collections
- Quiz Solutions
- Exercise Solutions
- Chapter 15: Strings
- Quiz Solutions
- Exercise Solutions
- Chapter 16: Throwing and Catching Exceptions
- Quiz Solutions
- Exercise Solutions
- Chapter 17: Delegates and Events
- Quiz Solutions
- Exercise Solutions
- Chapter 18: Creating Windows Applications
- Quiz Solutions
- Exercise Solutions
- Chapter 19: Windows Presentation Foundation
- Quiz Solutions
- Exercise Solutions
- Chapter 20: ADO.NET and Relational Databases
- Quiz Solutions
- Exercise Solutions
- Chapter 21: LINQ
- Quiz Solutions
- Exercise Solutions
- Chapter 1: C# and .NET Programming
- Index
- About the Authors
- Colophon
- SPECIAL OFFER: Upgrade this ebook with OReilly