JavaScript Pocket Reference. 3rd Edition - Helion
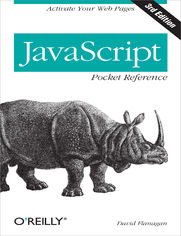
ISBN: 978-14-493-3599-1
stron: 280, Format: ebook
Data wydania: 2012-04-09
Księgarnia: Helion
Cena książki: 46,74 zł (poprzednio: 54,99 zł)
Oszczędzasz: 15% (-8,25 zł)
JavaScript is the ubiquitous programming language of the Web, and for more than 15 years, JavaScript: The Definitive Guide has been the bible of JavaScript programmers around the world. Ideal for JavaScript developers at any level, this book is an all-new excerpt of The Definitive Guide, collecting the essential parts of that hefty volume into this slim yet dense pocket reference.
The first 9 chapters document the latest version (ECMAScript 5) of the core JavaScript language, covering:
- Types, values, and variables
- Operators, expressions, and statements
- Objects and arrays
- Functions and classes
The next 5 chapters document the fundamental APIs for using JavaScript with HTML5 and explain how to:
- Interact with web browser windows
- Script HTML documents and document elements
- Modify and apply CSS styles and classes
- Respond to user input events
- Communicate with web servers
- Store data locally on the user's computer
This book is a perfect companion to jQuery Pocket Reference.
Osoby które kupowały "JavaScript Pocket Reference. 3rd Edition", wybierały także:
- Windows Media Center. Domowe centrum rozrywki 66,67 zł, (8,00 zł -88%)
- Ruby on Rails. Ćwiczenia 18,75 zł, (3,00 zł -84%)
- DevOps w praktyce. Kurs video. Jenkins, Ansible, Terraform i Docker 190,00 zł, (39,90 zł -79%)
- Przywództwo w świecie VUCA. Jak być skutecznym liderem w niepewnym środowisku 58,64 zł, (12,90 zł -78%)
- Scrum. O zwinnym zarządzaniu projektami. Wydanie II rozszerzone 58,64 zł, (12,90 zł -78%)
Spis treści
JavaScript Pocket Reference. Activate Your Web Pages. 3rd Edition eBook -- spis treści
- JavaScript Pocket Reference
- Preface
- 1. Lexical Structure
- Comments
- Identifiers and Reserved Words
- Optional Semicolons
- 2. Types, Values, and Variables
- Numbers
- Text
- String Literals
- Boolean Values
- null and undefined
- The Global Object
- Type Conversions
- Variable Declaration
- 3. Expressions and Operators
- Expressions
- Initializers
- Property Access
- Function Definition
- Invocation
- Object Creation
- Operators
- Arithmetic Operators
- Relational Operators
- Logical Expressions
- Assignment Expressions
- Evaluation Expressions
- Miscellaneous Operators
- The Conditional Operator (?:)
- The typeof Operator
- The delete Operator
- The void Operator
- The Comma Operator (,)
- Expressions
- 4. Statements
- Expression Statements
- Compound and Empty Statements
- Declaration Statements
- var
- function
- Conditionals
- if
- else if
- switch
- Loops
- while
- do/while
- for
- for/in
- Jumps
- Labeled Statements
- break
- continue
- return
- throw
- try/catch/finally
- Miscellaneous Statements
- with
- debugger
- use strict
- 5. Objects
- Creating Objects
- Object Literals
- Creating Objects with new
- Prototypes
- Object.create()
- Properties
- Querying and Setting Properties
- Property Inheritance
- Deleting Properties
- Testing Properties
- Enumerating Properties
- Serializing Properties and Objects
- Property Getters and Setters
- Property Attributes
- Object Attributes
- The prototype Attribute
- The class Attribute
- The extensible Attribute
- Creating Objects
- 6. Arrays
- Creating Arrays
- Array Elements and Length
- Iterating Arrays
- Multidimensional Arrays
- Array Methods
- join()
- reverse()
- sort()
- concat()
- slice()
- splice()
- push() and pop()
- unshift() and shift()
- toString()
- ECMAScript 5 Array Methods
- forEach()
- map()
- filter()
- every() and some()
- reduce(), reduceRight()
- indexOf() and lastIndexOf()
- Array Type
- Array-Like Objects
- Strings as Arrays
- 7. Functions
- Defining Functions
- Nested Functions
- Invoking Functions
- Function Invocation
- Method Invocation
- Constructor Invocation
- Indirect Invocation
- Function Arguments and Parameters
- Optional Parameters
- Variable-Length Argument Lists: The Arguments Object
- Functions as Namespaces
- Closures
- Function Properties, Methods, and Constructor
- The length Property
- The prototype Property
- The bind() Method
- The toString() Method
- The Function() Constructor
- Defining Functions
- 8. Classes
- Classes and Prototypes
- Classes and Constructors
- Constructors and Class Identity
- The constructor Property
- Java-Style Classes in JavaScript
- Immutable Classes
- Subclasses
- Augmenting Classes
- 9. Regular Expressions
- Describing Patterns with Regular Expressions
- Literal Characters
- Character Classes
- Repetition
- Nongreedy repetition
- Alternation, Grouping, and References
- Specifying Match Position
- Flags
- Matching Patterns with Regular Expressions
- String Methods for Pattern-Matching
- RegExp Properties and Methods
- Describing Patterns with Regular Expressions
- 10. Client-Side JavaScript
- Embedding JavaScript in HTML
- Event-Driven Programming
- The Window Object
- Timers
- Browser Location and Navigation
- Browsing History
- Browser and Screen Information
- Dialog Boxes
- Document Elements as Window Properties
- Multiple Windows and Frames
- Relationships Between Frames
- JavaScript in Interacting Windows
- The Same-Origin Policy
- 11. Scripting Documents
- Overview of the DOM
- Selecting Document Elements
- Selecting Elements by ID
- Selecting Elements by Name
- Selecting Elements by Type
- Selecting Elements by CSS Class
- Selecting Elements with CSS Selectors
- Document Structure and Traversal
- Attributes
- Element Content
- Element Content as HTML
- Element Content as Plain Text
- Element Content as Text Nodes
- Creating, Inserting, and Deleting Nodes
- Element Style
- Geometry and Scrolling
- 12. Handling Events
- Types of Events
- Form Events
- Window Events
- Mouse Events
- Key Events
- HTML5 Events
- Touchscreen and Mobile Events
- Registering Event Handlers
- Setting Event Handler Properties
- Setting Event Handler Attributes
- addEventListener()
- Event Handler Invocation
- Event Handler Argument
- Event Handler Context
- Event Handler Scope
- Handler Return Value
- Event Propagation
- Event Cancellation
- Types of Events
- 13. Networking
- Using XMLHttpRequest
- Specifying the Request
- Retrieving the Response
- HTTP Progress Events
- Cross-Origin HTTP Requests
- HTTP by <script>: JSONP
- Server-Sent Events
- WebSockets
- Using XMLHttpRequest
- 14. Client-Side Storage
- localStorage and sessionStorage
- Storage Lifetime and Scope
- Storage API
- Storage Events
- Cookies
- Cookie Attributes: Lifetime and Scope
- Setting Cookies
- Reading Cookies
- Cookie Limitations
- localStorage and sessionStorage
- Index
- About the Author
- Colophon
- Copyright