Intel Threading Building Blocks. Outfitting C++ for Multi-core Processor Parallelism - Helion
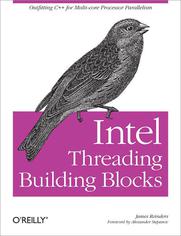
ISBN: 978-14-493-9086-0
stron: 336, Format: ebook
Data wydania: 2007-07-12
Księgarnia: Helion
Cena książki: 109,65 zł (poprzednio: 127,50 zł)
Oszczędzasz: 14% (-17,85 zł)
Multi-core chips from Intel and AMD offer a dramatic boost in speed and responsiveness, and plenty of opportunities for multiprocessing on ordinary desktop computers. But they also present a challenge: More than ever, multithreading is a requirement for good performance. This guide explains how to maximize the benefits of these processors through a portable C++ library that works on Windows, Linux, Macintosh, and Unix systems. With it, you'll learn how to use Intel Threading Building Blocks (TBB) effectively for parallel programming -- without having to be a threading expert.
Written by James Reinders, Chief Evangelist of Intel Software Products, and based on the experience of Intel's developers and customers, this book explains the key tasks in multithreading and how to accomplish them with TBB in a portable and robust manner. With plenty of examples and full reference material, the book lays out common patterns of uses, reveals the gotchas in TBB, and gives important guidelines for choosing among alternatives in order to get the best performance.
You'll learn how Intel Threading Building Blocks:
- Enables you to specify tasks instead of threads for better portability, easier programming, more understandable source code, and better performance and scalability in general
- Focuses on the goal of parallelizing computationally intensive work to deliver high-level solutions
- Is compatible with other threading packages, and doesn't force you to pick one package for your entire program
- Emphasizes scalable, data-parallel programming, which allows program performance to increase as you add processors
- Relies on generic programming, which enables you to write the best possible algorithms with the fewest constraints
Osoby które kupowały "Intel Threading Building Blocks. Outfitting C++ for Multi-core Processor Parallelism", wybierały także:
- Windows Media Center. Domowe centrum rozrywki 66,67 zł, (8,00 zł -88%)
- Ruby on Rails. Ćwiczenia 18,75 zł, (3,00 zł -84%)
- Efekt piaskownicy. Jak szefować żeby roboty nie zabrały ci roboty 59,50 zł, (11,90 zł -80%)
- Przywództwo w świecie VUCA. Jak być skutecznym liderem w niepewnym środowisku 58,64 zł, (12,90 zł -78%)
- Scrum. O zwinnym zarządzaniu projektami. Wydanie II rozszerzone 58,64 zł, (12,90 zł -78%)
Spis treści
Intel Threading Building Blocks. Outfitting C++ for Multi-core Processor Parallelism eBook -- spis treści
- Intel Threading Building Blocks
- SPECIAL OFFER: Upgrade this ebook with OReilly
- Foreword
- Note from the Lead Developer of Intel Threading Building Blocks
- Preface
- Assumptions This Book Makes
- Contents of This Book
- Conventions Used in This Book
- Informal Class Declarations
- Using Code Examples
- How to Contact Us
- Acknowledgments
- 1. Why Threading Building Blocks?
- Overview
- Benefits
- Comparison with Raw Threads and MPI
- Comparison with OpenMP
- Recursive Splitting, Task Stealing, and Algorithms
- 2. Thinking Parallel
- Elements of Thinking Parallel
- Decomposition
- Data Parallelism
- Task Parallelism
- Pipelining (Task and Data Parallelism Together)
- Mixed Solutions
- Achieving Parallelism
- Scaling and Speedup
- How Much Parallelism Is There in an Application?
- Amdahls Law
- Gustafsons observations regarding Amdahls Law
- What did they really say?
- Serial versus parallel algorithms
- How Much Parallelism Is There in an Application?
- What Is a Thread?
- Programming Threads
- Safety in the Presence of Concurrency
- Mutual Exclusion and Locks
- Correctness
- Abstraction
- Patterns
- Intuition
- 3. Basic Algorithms
- Initializing and Terminating the Library
- Loop Parallelization
- parallel_for
- Grain size
- Automatic grain size
- Notes on automatic grain size
- parallel_for with partitioner
- parallel_reduce
- Advanced example
- Parallel_reduce with partitioner
- Advanced Topic: Other Kinds of Iteration Spaces
- Notes on blocked_range2d
- parallel_scan
- Parallel_scan with partitioner
- parallel_for
- Recursive Range Specifications
- Splittable Concept
- Recursive Range Specifications
- Model Types: Splittable Ranges
- split Class
- Range Concept
- Model Types
- blocked_range<Value> Template Class
- blocked_range2d Template Class
- Partitioner Concept
- Model Types: Partitioners
- simple_partitioner Class
- auto_partitioner Class
- parallel_for<Range,Body> Template Function
- parallel_reduce<Range,Body> Template Function
- parallel_scan<Range,Body> Template Function
- pre_scan_tag and final_scan_tag Classes
- Model Types: Splittable Ranges
- Summary of Loops
- 4. Advanced Algorithms
- Parallel Algorithms for Streams
- Cook Until Done: parallel_while
- Notes on parallel_while scaling
- parallel_while Template Class
- Notes on parallel_while scaling
- Working on the Assembly Line: Pipeline
- Throughput of pipeline
- Nonlinear pipelines
- pipeline Class
- filter Class
- parallel_sort
- parallel_sort<RandomAccessIterator, Compare> Template Function
- Cook Until Done: parallel_while
- Parallel Algorithms for Streams
- 5. Containers
- concurrent_queue
- Iterating over a concurrent_queue for Debugging
- When Not to Use Queues
- Template Class
- concurrent_vector
- concurrent_vector Template Class
- concurrent_vector
- Whole Vector Operations
- Concurrent Operations
- Parallel Iteration
- Capacity
- Iterators
- concurrent_hash_map
- More on HashCompare
- Template Class
- Whole-Table Operations
- Concurrent Access
- const_accessor
- accessor class
- Concurrent Operations: find, insert, erase
- Parallel Iteration
- Capacity
- Iterators
- More on HashCompare
- concurrent_queue
- 6. Scalable Memory Allocation
- Limitations
- Problems in Memory Allocation
- Memory Allocators
- Which Library to Link into Your Application
- Using the Allocator Argument to C++ STL Template Classes
- Replacing malloc, new, and delete
- Replace malloc, free, realloc, and calloc
- Replace new and delete
- Allocator Concept
- Model Types
- scalable_allocator<T> Template Class
- cache_aligned_allocator<T> Template Class
- aligned_space Template Class
- 7. Mutual Exclusion
- When to Use Mutual Exclusion
- Mutexes
- Mutex Flavors
- Reader-Writer Mutexes
- Upgrade/Downgrade
- Lock Pathologies
- Deadlock
- Convoying and priority inversion
- Mutexes
- Mutex Concept
- mutex Class
- spin_mutex Class
- queuing_mutex Class
- ReaderWriterMutex Concept
- Model Types
- spin_rw_mutex Class
- queuing_rw_mutex Class
- Model Types
- Mutex Concept
- Atomic Operations
- Why atomic<T> Has No Constructors
- Memory Consistency and Fences
- atomic<T> Template Class
- 8. Timing
- tick_count Class
- tick_count::interval_t Class
- 9. Task Scheduler
- When Task-Based Programming Is Inappropriate
- Much Better Than Raw Native Threads
- Oversubscription
- Fair Scheduling
- High Coding Overhead
- Load Imbalance
- Portability
- Initializing the Library Is Your Job
- Example Program for Fibonacci Numbers
- Task Scheduling Overview
- How Task Scheduling Works
- Recommended Task Recurrence Patterns
- Blocking Style with Children
- Continuation-Passing Style with Children
- Recycling the parent as the continuation
- Recycling the parent as a child
- Making Best Use of the Scheduler
- Recursive Chain Reaction
- Continuation Passing
- Scheduler bypass
- Recycling
- Empty tasks
- Lazy copying
- Task Scheduler Interfaces
- task_scheduler_init Class
- task Class
- empty_task Class
- task_list Class
- Task Scheduler Summary
- 10. Keys to Success
- Key Steps to Success
- Relaxed Sequential Execution
- Safe Concurrency for Methods and Libraries
- Debug Versus Release
- For Efficiencys Sake
- Enabling Debugging Features
- The TBB_DO_ASSERT Macro
- Do Not Ship Your Program Built with TBB_DO_ASSERT
- The TBB_DO_THREADING_TOOLS Macro
- Debug Versus Release Libraries
- Mixing with Other Threading Packages
- Naming Conventions
- The tbb Namespace
- The tbb::internal Namespace
- The _ _TBB Prefix
- 11. Examples
- The Aha! Factor
- A Few Other Key Points
- parallel_for Examples
- ParallelAverage
- Seismic
- Matrix Multiply
- ParallelMerge
- SubstringFinder
- The Game of Life
- Implementation
- Automaton
- Automata: Implementation
- Extending the Application
- Futher Reading
- Parallel_reduce Examples
- ParallelSum
- ParallelSum without Having to Specify a Grain Size
- ParallelPrime
- CountStrings: Using concurrent_hash_map
- CountStrings: Using concurrent_hash_map
- Switching from an STL map
- CountStrings: Using concurrent_hash_map
- Quicksort: Visualizing Task Stealing
- A Better Matrix Multiply (Strassen)
- Advanced Task Programming
- Start a Large Task in Parallel with the Main Program
- Two Mouths: Feeding Two from the Same Task in a Pipeline
- Packet Processing Pipeline
- Parallel Programming for an Internet Device
- Local Network Router Example
- Pipelined Components for the Local Network Router
- Network address translation (NAT)
- Application Layer Gateway (ALG)
- Packet forwarding
- Our example
- Implementation
- The Threading Building Blocks pipeline
- Synchronization with the pipeline item and concurrent hash maps
- Filter Classes
- Class get_next_packet : public tbb::filter
- Class output_packet : public tbb::filter
- Class translator : public tbb::filter
- Class gateway : public tbb::filter
- Class forwarding : public tbb::filter
- Additional reading
- Memory Allocation
- Replacing new and delete
- Replacing malloc, calloc, realloc, and free
- Game Threading Example
- Threading Architecture: Physics + Rendering
- Overview of Keys to Scalability
- A Frame Loop
- Domain Decomposition Data Structure Needs
- Think Tasks, Not Threads
- Load Balancing Versus Task Stealing
- Synchronization Between Physics Threads
- Integrating the Example into a Real Game
- How to Measure Performance
- Physics Interaction and Update Code
- Open Dynamics Engine
- Look for Hotspots
- Improving on the First Solution
- The Code
- 12. History and Related Projects
- Libraries
- Languages
- Pragmas
- Generic Programming
- Concepts in Generic Programming
- Pseudosignatures in Generic Programming
- Models in Generic Programming
- Caches
- Costs of Time Slicing
- Quick Introduction to Lambda Functions
- Further Reading
- Index
- About the Author
- Colophon
- SPECIAL OFFER: Upgrade this ebook with OReilly