iOS 15 Programming Fundamentals with Swift - Helion
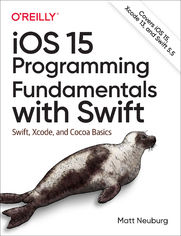
ebook
Autor: Matt NeuburgISBN: 9781098118464
stron: 788, Format: ebook
Data wydania: 2021-10-01
Księgarnia: Helion
Cena książki: 186,15 zł (poprzednio: 216,45 zł)
Oszczędzasz: 14% (-30,30 zł)
Move into iOS development by getting a firm grasp of its fundamentals, including the Xcode 13 IDE, Cocoa Touch, and the latest version of Apple's acclaimed programming language, Swift 5.5. With this thoroughly updated guide, you'll learn the Swift language, understand Apple's Xcode development tools, and discover the Cocoa framework.
- Explore Swift's object-oriented concepts
- Become familiar with built-in Swift types
- Dive deep into Swift objects, protocols, and generics
- Tour the life cycle of an Xcode project
- Learn how nibs are loaded
- Understand Cocoa's event-driven design
- Communicate with C and Objective-C
In this edition, catch up on the latest iOS programming features:
- Structured concurrency: async/await, tasks, and actors
- Swift native formatters and attributed strings
- Lazy locals and throwing getters
- Enhanced collections with the Swift Algorithms and Collections packages
- Xcode tweaks: column breakpoints, package collections, and Info.plist build settings
- Improvements in Git integration, localization, unit testing, documentation, and distribution
- And more!
Osoby które kupowały "iOS 15 Programming Fundamentals with Swift", wybierały także:
- Windows Media Center. Domowe centrum rozrywki 66,67 zł, (8,00 zł -88%)
- Ruby on Rails. Ćwiczenia 18,75 zł, (3,00 zł -84%)
- DevOps w praktyce. Kurs video. Jenkins, Ansible, Terraform i Docker 190,00 zł, (39,90 zł -79%)
- Przywództwo w świecie VUCA. Jak być skutecznym liderem w niepewnym środowisku 58,64 zł, (12,90 zł -78%)
- Scrum. O zwinnym zarządzaniu projektami. Wydanie II rozszerzone 58,64 zł, (12,90 zł -78%)
Spis treści
iOS 15 Programming Fundamentals with Swift eBook -- spis treści
- Preface
- The Scope of This Book
- From the Preface to the First Edition (Programming iOS 4)
- Versions
- Acknowledgments
- Conventions Used in This Book
- Using Code Examples
- OReilly Online Learning
- How to Contact Us
- I. Language
- 1. The Architecture of Swift
- Ground of Being
- Everything Is an Object?
- Three Flavors of Object Type
- Variables
- Functions
- The Structure of a Swift File
- Scope and Lifetime
- Object Members
- Namespaces
- Modules
- Instances
- Why Instances?
- The Keyword self
- Privacy
- Design
- 2. Functions
- Function Parameters and Return Value
- Void Return Type and Parameters
- Function Signature
- External Parameter Names
- Overloading
- Default Parameter Values
- Variadic Parameters
- Ignored Parameters
- Modifiable Parameters
- Calling Objective-C with Modifiable Parameters
- Called by Objective-C with Modifiable Parameters
- Reference Type Modifiable Parameters
- Function in Function
- Recursion
- Function as Value
- Anonymous Functions
- Using Anonymous Functions Inline
- Anonymous Function Abbreviated Syntax
- Define-and-Call
- Closures
- How Closures Improve Code
- Function Returning Function
- Closure Setting a Captured Variable
- Closure Preserving Captured Environment
- Escaping Closures
- Capture Lists
- Curried Functions
- Function References and Selectors
- Function Reference Scope
- Selectors
- Function Parameters and Return Value
- 3. Variables and Simple Types
- Variable Scope and Lifetime
- Variable Declaration
- Computed Variable Initialization
- Computed Variables
- Computed Properties
- Property Wrappers
- Setter Observers
- Lazy Initialization
- Singleton
- Lazy Initialization of Instance Properties
- Built-In Simple Types
- Bool
- Numbers
- Int
- Double
- Numeric coercion
- Other numeric types
- Arithmetic operations
- Comparison
- String
- Character and String Index
- Range
- Tuple
- Optional
- Unwrapping an Optional
- Implicitly unwrapped Optional
- The keyword nil
- Optional chains
- Optional map and flatMap
- Comparison with Optional
- Why Optionals?
- 4. Object Types
- Object Type Declarations and Features
- Initializers
- How to write an initializer
- Deferred initialization of properties
- Referring to self
- Delegating initializers
- Failable initializers
- Properties
- How properties are accessed
- Property initialization and self
- Methods
- Subscripts
- Nested Object Types
- Initializers
- Enums
- Raw Values
- Associated Values
- Enum Case Iteration
- Enum Initializers
- Enum Properties
- Enum Methods
- Why Enums?
- Structs
- Struct Initializers
- Struct Properties
- Struct Methods
- Classes
- Value Types and Reference Types
- Class instances are mutable
- Class instance references are pointers
- Advantages of value types vs. reference types
- Subclass and Superclass
- Inheritance
- Additional functionality
- Overriding
- The keyword super
- Class Initializers
- Kinds of class initializer
- Subclass initializers
- Subclass initializer examples
- Required initializers
- Class Deinitializer
- Class Properties
- Static/Class Members
- Static methods vs. class methods
- Static properties vs. class properties
- Value Types and Reference Types
- Polymorphism
- Casting
- Casting Down
- Type Testing and Casting Down Safely
- Type Testing and Casting Optionals
- Bridging to Objective-C
- Type References
- From Instance to Type
- From self to Type
- Type as Value
- Summary of Type Terminology
- Comparing Types
- Protocols
- Why Protocols?
- Adopting a Library Protocol
- Protocol Type Testing and Casting
- Declaring a Protocol
- Protocol Composition
- Class Protocols
- Optional Protocol Members
- Optional properties
- Optional methods
- Implicitly Required Initializers
- Expressible by Literal
- Generics
- Generic Declarations
- Type Constraints
- Explicit Specialization
- Generic Types and Covariance
- Associated Type Chains
- Where Clauses
- Extensions
- Extending Protocols
- Extending Generics
- Umbrella Types
- Any
- AnyObject
- Suppressing type checking
- Object identity
- AnyClass
- Collection Types
- Array
- Array casting and type testing
- Array comparison
- Arrays are value types
- Array subscripting
- Nested arrays
- Basic array properties and methods
- Array enumeration and transformation
- Swift Array and Objective-C NSArray
- Dictionary
- Dictionary subscripting
- Dictionaries have no order
- Dictionary casting and comparison
- Basic dictionary properties and enumeration
- Swift Dictionary and Objective-C NSDictionary
- Set
- Option sets
- Swift Set and Objective-C NSSet
- OrderedSet and OrderedDictionary
- OrderedSet
- OrderedDictionary
- Array
- Object Type Declarations and Features
- 5. Flow Control and More
- Flow Control
- Branching
- If construct
- Conditional binding
- Switch statement
- If case
- Conditional evaluation
- Loops
- While loops
- For loops
- Jumping
- Return
- Short-circuiting and labels
- Throwing and catching errors
- Nested scopes
- Defer statement
- Aborting the whole program
- Guard
- Branching
- Privacy
- Private and Fileprivate
- Public and Open
- Privacy Rules
- Introspection
- Operators
- Memory Management
- Memory Management of Reference Types
- Weak references
- Unowned references
- Stored anonymous functions
- Memory management of protocol-typed references
- Exclusive Access to Value Types
- Memory Management of Reference Types
- Miscellaneous Swift Language Features
- Synthesized Protocol Implementations
- Equatable
- Hashable
- Comparable
- Key Paths
- Instance as Function
- Dynamic Membership
- Property Wrappers
- Custom String Interpolation
- Reverse Generics
- Result Builders
- Result
- Synthesized Protocol Implementations
- Flow Control
- 6. Structured Concurrency
- Multithreading
- The Main Thread
- Background Threads
- Asynchronous Code
- What asynchronous code looks like
- Returning a value
- Throwing an error
- Summary
- Structured Concurrency Syntax
- async/await
- Async Contexts
- Tasks
- Wrapping a Completion Handler
- Multiple Concurrent Tasks
- async let
- Task Groups
- Asynchronous Sequences
- Built-in Asynchronous Sequences
- Making an Asynchronous Sequence
- Asynchronous Sequence Methods
- Actors
- Actor Isolation
- Actor Serialization
- The Main Actor
- Context Switching
- Implicit Context Switching
- Explicit Context Switching
- More About Tasks
- Task Priority
- The Current Task
- Sleeping
- Yielding
- Cancellation
- Cancellation strategies
- Cancelling subtasks
- More About Actors
- Reentrancy
- The Keyword nonisolated
- The Keyword isolated
- Global Actors
- Sendable
- Multithreading
- 1. The Architecture of Swift
- II. IDE
- 7. Anatomy of an Xcode Project
- New Project
- The Project Window
- The Navigator Pane
- The Inspector Pane
- The Editor
- Editor panes
- Assistant panes
- Tabs and windows
- Project File and Dependents
- Contents of the Project Folder
- Groups
- The Target
- Build Phases
- Build Settings
- Configurations
- Schemes and Destinations
- From Project to Built App
- Build Settings
- Property List Settings
- Nib Files
- Resources
- Resources in the Project navigator
- Resources in an asset catalog
- Code Files
- Frameworks and SDKs
- Swift Packages
- Adding a package
- Creating a package
- Customizing a package
- Sharing a package
- The App Launch Process
- The Entry Point
- How an App Gets Going
- App Without a Storyboard
- Renaming Parts of a Project
- 8. Nib Files
- The Nib Editor Interface
- Document Outline
- Canvas
- Inspectors
- Loading a Nib
- Loading a View Controller Nib
- Loading a Main View Nib
- Loading a View Nib Manually
- Connections
- Outlets
- The Nib Owner
- Automatically Configured Nibs
- Misconfigured Outlets
- Outletproperty name mismatch
- No outlet in the nib
- No view outlet
- Deleting an Outlet
- More Ways to Create Outlets
- Outlet Collections
- Action Connections
- More Ways to Create Actions
- Misconfigured Actions
- Connections Between Nibs Not!
- Additional Configuration of Nib-Based Instances
- The Nib Editor Interface
- 9. Documentation
- The Documentation Window
- Class Documentation Pages
- Quick Help
- Documenting Frameworks and Packages
- Symbol Declarations
- Header Files
- Sample Code
- Internet Resources
- 10. Life Cycle of a Project
- Environmental Dependencies
- Conditional Compilation
- Build Action
- Permissible Runtime Environment
- Backward Compatibility
- Device Type
- Arguments and Environment Variables
- Version Control
- Editing and Navigating Your Code
- Text Editing Preferences
- Display
- Editing
- Indentation
- Multiple Selection
- Code Completion and Placeholders
- Snippets
- Refactoring and Code Actions
- Fix-it and Live Syntax Checking
- Navigation
- Finding
- Text Editing Preferences
- Running in the Simulator
- Debugging
- Caveman Debugging
- Dump
- Logger
- Aborting
- The Xcode Debugger
- Breakpoints
- Paused at a breakpoint
- Caveman Debugging
- Testing
- Unit Tests
- Interface Tests
- Persisting screenshots
- Interface testing and accessibility
- Test Plans
- Massaging the Report
- Clean
- Running on a Device
- Obtaining a Developer Program Membership
- Signing an App
- Automatic Signing
- Manual Signing
- Running the App
- Managing Development Certificates and Devices
- Profiling
- Gauges
- Memory Debugging
- Instruments
- Localization
- Creating Localized Content
- Exporting
- Editing
- Importing
- Testing Localization
- Creating Localized Content
- Distribution
- Making an Archive
- The Distribution Certificate
- The Distribution Profile
- Distribution for Testing
- Ad Hoc distribution
- TestFlight distribution
- Final App Preparations
- Icons in the app
- Marketing icon
- Launch images
- Screenshots and Video Previews
- Property List Settings
- Submission to the App Store
- Environmental Dependencies
- 7. Anatomy of an Xcode Project
- III. Cocoa
- 11. Cocoa Classes
- Subclassing
- Categories and Extensions
- How Swift Uses Extensions
- How You Use Extensions
- How Cocoa Uses Categories
- Protocols
- Optional Members
- Informal Protocols
- Some Foundation Classes
- NSRange
- NSNotFound
- NSString and Friends
- NSDate and Friends
- NSNumber
- NSValue
- NSData
- NSMeasurement and Friends
- Equality, Hashability, and Comparison
- NSArray and NSMutableArray
- NSDictionary and NSMutableDictionary
- NSSet and Friends
- NSIndexSet
- NSNull
- Immutable and Mutable
- Property Lists
- Codable
- Accessors, Properties, and KeyValue Coding
- Swift Accessors
- KeyValue Coding
- How Outlets Work
- Cocoa Key Paths
- Uses of KeyValue Coding
- KeyPath Notation
- The Secret Life of NSObject
- 12. Cocoa Events
- Reasons for Events
- Subclassing
- Notifications
- Receiving a Notification
- Selector-based registration
- Function-based registration
- Unregistering
- Subscribing to a Notification
- Combine framework
- Structured concurrency
- Posting a Notification
- Timer
- Receiving a Notification
- Delegation
- Cocoa Delegation
- Implementing Delegation
- Data Sources
- Actions
- The Responder Chain
- Nil-Targeted Actions
- KeyValue Observing
- Registration and Notification
- Unregistering
- KeyValue Observing Example
- Swamped by Events
- Delayed Performance
- 13. Memory Management
- Principles of Cocoa Memory Management
- Rules of Cocoa Memory Management
- What ARC Is and What It Does
- How Cocoa Objects Manage Memory
- Autorelease Pool
- Memory Management of Instance Properties
- Retain Cycles and Weak References
- Unusual Memory Management Situations
- Notification Observers
- KVO Observers
- Timers
- Other Unusual Situations
- Memory Management of CFTypeRefs
- Property Memory Management Policies
- Debugging Memory Management Mistakes
- 14. Communication Between Objects
- Visibility Through an Instance Property
- Visibility by Instantiation
- Getting a Reference
- Visibility by Relationship
- Global Visibility
- Notifications and KeyValue Observing
- The Combine Framework
- Alternative Architectures
- ModelViewController
- Router and Data Space
- ModelViewPresenter
- Protocols and Reactive Programming
- SwiftUI
- Result Builders and Modifiers
- State Properties
- Bindings
- Passing Data Downhill
- Passing Data Uphill
- Custom State Objects
- A. C, Objective-C, and Swift
- The C Language
- C Data Types
- C Enums
- Old-fashioned C enum
- NS_ENUM
- NS_OPTIONS
- Global string constants
- C Structs
- C Pointers
- C Arrays
- C Strings
- C Functions
- Struct functions
- Pointer-to-function
- Objective-C
- Objective-C Objects and C Pointers
- Objective-C Objects and Swift Objects
- Exposure of Swift to Objective-C
- Bridged Types and Boxed Types
- Objective-C Methods
- Renamification
- Internal parameter names
- Reverse renamification
- Overloading
- Variadics
- Initializers and factories
- Error pointers
- Async methods
- Selectors
- CFTypeRefs
- Blocks
- API Markup
- Nullability
- Lightweight generics
- Bilingual Targets
- The C Language
- 11. Cocoa Classes
- Index