Robust Python - Helion
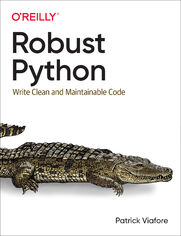
ISBN: 9781098100612
stron: 380, Format: ebook
Data wydania: 2021-07-12
Księgarnia: Helion
Cena książki: 169,15 zł (poprzednio: 196,69 zł)
Oszczędzasz: 14% (-27,54 zł)
Does it seem like your Python projects are getting bigger and bigger? Are you feeling the pain as your codebase expands and gets tougher to debug and maintain? Python is an easy language to learn and use, but that also means systems can quickly grow beyond comprehension. Thankfully, Python has features to help developers overcome maintainability woes.
In this practical book, author Patrick Viafore shows you how to use Python's type system to the max. You'll look at user-defined types, such as classes and enums, and Python's type hinting system. You'll also learn how to make Python extensible and how to use a comprehensive testing strategy as a safety net. With these tips and techniques, you'll write clearer and more maintainable code.
- Learn why types are essential in modern development ecosystems
- Understand how type choices such as classes, dictionaries, and enums reflect specific intents
- Make Python extensible for the future without adding bloat
- Use popular Python tools to increase the safety and robustness of your codebase
- Evaluate current code to detect common maintainability gotchas
- Build a safety net around your codebase with linters and tests
Osoby które kupowały "Robust Python", wybierały także:
- Windows Media Center. Domowe centrum rozrywki 66,67 zł, (8,00 zł -88%)
- Ruby on Rails. Ćwiczenia 18,75 zł, (3,00 zł -84%)
- DevOps w praktyce. Kurs video. Jenkins, Ansible, Terraform i Docker 190,00 zł, (39,90 zł -79%)
- Przywództwo w świecie VUCA. Jak być skutecznym liderem w niepewnym środowisku 58,64 zł, (12,90 zł -78%)
- Scrum. O zwinnym zarządzaniu projektami. Wydanie II rozszerzone 58,64 zł, (12,90 zł -78%)
Spis treści
Robust Python eBook -- spis treści
- Preface
- Who Should Read This Book
- About This Book
- Conventions Used in This Book
- Using Code Examples
- OReilly Online Learning
- How to Contact Us
- Acknowledgments
- 1. Introduction to Robust Python
- Robustness
- Why Does Robustness Matter?
- Whats Your Intent?
- Asynchronous Communication
- Examples of Intent in Python
- Collections
- Iteration
- Law of Least Surprise
- Closing Thoughts
- Robustness
- I. Annotating Your Code with Types
- 2. Introduction to Python Types
- Whats in a Type?
- Mechanical Representation
- Semantic Representation
- Typing Systems
- Strong Versus Weak
- Dynamic Versus Static
- Duck Typing
- Closing Thoughts
- Whats in a Type?
- 3. Type Annotations
- What Are Type Annotations?
- Benefits of Type Annotations
- Autocomplete
- Typecheckers
- Exercise: Spot the Bug
- When to Use Type Annotations
- Closing Thoughts
- 4. Constraining Types
- Optional Type
- Union Types
- Product and Sum Types
- Literal Types
- Annotated Types
- NewType
- Final Types
- Closing Thoughts
- 5. Collection Types
- Annotating Collections
- Homogeneous Versus Heterogeneous Collections
- TypedDict
- Creating New Collections
- Generics
- Modifying Existing Types
- As Easy as ABC
- Closing Thoughts
- 6. Customizing Your Typechecker
- Configuring Your Typechecker
- Configuring mypy
- Catching dynamic behavior
- Requiring types
- Handling None/Optional
- Mypy Reporting
- Speeding Up mypy
- Configuring mypy
- Alternative Typecheckers
- Pyre
- Codebase querying
- Python Static Analyzer (Pysa)
- Pyright
- Pyre
- Closing Thoughts
- Configuring Your Typechecker
- 7. Adopting Typechecking Practically
- Trade-offs
- Breaking Even Earlier
- Find Your Pain Points
- Target Code Strategically
- Type annotate new code only
- Type annotate from the bottom up
- Type annotate your moneymakers
- Type annotate the churners
- Type annotate the complex
- Lean on Your Tooling
- MonkeyType
- Pytype
- Closing Thoughts
- II. Defining Your Own Types
- 8. User-Defined Types: Enums
- User-Defined Types
- Enumerations
- Enum
- When Not to Use
- Advanced Usage
- Automatic Values
- Flags
- Integer Conversion
- Unique
- Closing Thoughts
- 9. User-Defined Types: Data Classes
- Data Classes in Action
- Usage
- String Conversion
- Equality
- Relational Comparison
- Immutability
- Comparison to Other Types
- Data Classes Versus Dictionaries
- Data Classes Versus TypedDict
- Data Classes Versus namedtuple
- Closing Thoughts
- 10. User-Defined Types: Classes
- Class Anatomy
- Constructors
- Invariants
- Avoiding Broken Invariants
- Why Are Invariants Beneficial?
- Communicating Invariants
- Consuming Your Class
- What About Maintainers?
- Encapsulation and Maintaining Invariants
- Encapsul-what, Now?
- Protecting Data Access
- Operations
- Closing Thoughts
- Class Anatomy
- 11. Defining Your Interfaces
- Natural Interface Design
- Thinking Like a User
- Test-driven development
- README-driven development
- Usability testing
- Thinking Like a User
- Natural Interactions
- Natural Interfaces in Action
- Magic Methods
- Context Managers
- Closing Thoughts
- Natural Interface Design
- 12. Subtyping
- Inheritance
- Substitutability
- Design Considerations
- Composition
- Closing Thoughts
- 13. Protocols
- Tension Between Typing Systems
- Leave the Type Blank or Use Any
- Use a Union
- Use Inheritance
- Use Mixins
- Protocols
- Defining a Protocol
- Advanced Usage
- Composite Protocols
- Runtime Checkable Protocols
- Modules Satisfying Protocols
- Closing Thoughts
- Tension Between Typing Systems
- 14. Runtime Checking With pydantic
- Dynamic Configuration
- pydantic
- Validators
- Validation Versus Parsing
- Closing Thoughts
- III. Extensible Python
- 15. Extensibility
- What Is Extensibility?
- The Redesign
- Open-Closed Principle
- Detecting OCP Violations
- Drawbacks
- Closing Thoughts
- What Is Extensibility?
- 16. Dependencies
- Relationships
- Types of Dependencies
- Physical Dependencies
- Logical Dependencies
- Temporal Dependencies
- Visualizing Your Dependencies
- Visualizing Packages
- Visualizing Imports
- Visualizing Function Calls
- Interpreting Your Dependency Graph
- Closing Thoughts
- 17. Composability
- Composability
- Policy Versus Mechanisms
- Composing on a Smaller Scale
- Composing Functions
- Decorators
- Composing Algorithms
- Composing Functions
- Closing Thoughts
- 18. Event-Driven Architecture
- How It Works
- Drawbacks
- Simple Events
- Using a Message Broker
- The Observer Pattern
- Streaming Events
- Closing Thoughts
- How It Works
- 19. Pluggable Python
- The Template Method Pattern
- The Strategy Pattern
- Plug-in Architectures
- Closing Thoughts
- IV. Building a Safety Net
- 20. Static Analysis
- Linting
- Writing Your Own Pylint Plug-in
- Breaking Down the Plug-in
- Other Static Analyzers
- Complexity Checkers
- Cyclomatic complexity with mccabe
- Whitespace heuristic
- Security Analysis
- Leaking secrets
- Security flaw checking
- Complexity Checkers
- Closing Thoughts
- Linting
- 21. Testing Strategy
- Defining Your Test Strategy
- What Is a Test?
- The testing pyramid
- What Is a Test?
- Reducing Test Cost
- AAA Testing
- Arrange
- Consistent preconditions versus changing preconditions
- Use test framework features for boilerplate code
- Mocking
- Annihilate
- Dont use shared resources
- Use context managers
- Use fixtures
- Act
- Assert
- Arrange
- AAA Testing
- Closing Thoughts
- Defining Your Test Strategy
- 22. Acceptance Testing
- Behavior-Driven Development
- The Gherkin Language
- Executable Specifications
- Additional behave Features
- Parameterized Steps
- Table-Driven Requirements
- Step Matching
- Customizing the Test Life Cycle
- Using Tags to Selectively Run Tests
- Report Generation
- Closing Thoughts
- Behavior-Driven Development
- 23. Property-Based Testing
- Property-Based Testing with Hypothesis
- The Magic of Hypothesis
- Contrast with Traditional Tests
- Getting the Most Out of Hypothesis
- Hypothesis Strategies
- Generating Algorithms
- Closing Thoughts
- Property-Based Testing with Hypothesis
- 24. Mutation Testing
- What Is Mutation Testing?
- Mutation Testing with mutmut
- Fixing Mutants
- Mutation Testing Reports
- Adopting Mutation Testing
- The Fallacy of Coverage (and Other Metrics)
- Closing Thoughts
- Index