RESTful Java with JAX-RS 2.0. Designing and Developing Distributed Web Services. 2nd Edition - Helion
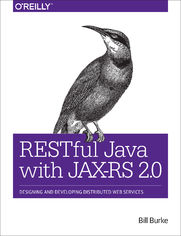
ISBN: 978-14-493-6145-7
stron: 392, Format: ebook
Data wydania: 2013-11-12
Księgarnia: Helion
Cena książki: 109,65 zł (poprzednio: 127,50 zł)
Oszczędzasz: 14% (-17,85 zł)
Learn how to design and develop distributed web services in Java, using RESTful architectural principles and the JAX-RS 2.0 specification in Java EE 7. By focusing on implementation rather than theory, this hands-on reference demonstrates how easy it is to get started with services based on the REST architecture.
With the book’s technical guide, you’ll learn how REST and JAX-RS work and when to use them. The RESTEasy workbook that follows provides step-by-step instructions for installing, configuring, and running several working JAX-RS examples, using the JBoss RESTEasy implementation of JAX-RS 2.0.
- Learn JAX-RS 2.0 features, including a client API, server-side asynchronous HTTP, and filters and interceptors
- Examine the design of a distributed RESTful interface for an e-commerce order entry system
- Use the JAX-RS Response object to return complex responses to your client (ResponseBuilder)
- Increase the performance of your services by leveraging HTTP caching protocols
- Deploy and integrate web services within Java EE7, servlet containers, EJB, Spring, and JPA
- Learn popular mechanisms to perform authentication on the Web, including client-side SSL and OAuth 2.0
Osoby które kupowały "RESTful Java with JAX-RS 2.0. Designing and Developing Distributed Web Services. 2nd Edition", wybierały także:
- JavaFX. Kurs video. Wzorce oraz typy generyczne 79,00 zł, (31,60 zł -60%)
- Platforma Xamarin. Kurs video. Poziom drugi. Zaawansowane techniki tworzenia aplikacji cross-platform 99,00 zł, (39,60 zł -60%)
- Spring Security. Kurs video. Skuteczne metody zabezpieczeń aplikacji 69,00 zł, (27,60 zł -60%)
- JUnit - testy jednostkowe. Kurs video. Automatyzacja procesu testowania w Javie 79,00 zł, (31,60 zł -60%)
- Algorytmy i struktury danych. Kurs video. Java, JavaScript, Python 89,00 zł, (40,05 zł -55%)
Spis treści
RESTful Java with JAX-RS 2.0. Designing and Developing Distributed Web Services. 2nd Edition eBook -- spis treści
- RESTful Java with JAX-RS 2.0
- Foreword
- Preface
- Authors Note
- Who Should Read This Book
- How This Book Is Organized
- Part I, REST and the JAX-RS Standard
- Part II, JAX-RS Workbook
- Conventions Used in This Book
- Using Code Examples
- Safari Books Online
- How to Contact Us
- Acknowledgments
- I. REST and the JAX-RS Standard
- 1. Introduction to REST
- REST and the Rebirth of HTTP
- RESTful Architectural Principles
- Addressability
- The Uniform, Constrained Interface
- Why Is the Uniform Interface Important?
- Representation-Oriented
- Communicate Statelessly
- HATEOAS
- The engine of application state
- Wrapping Up
- 2. Designing RESTful Services
- The Object Model
- Model the URIs
- Defining the Data Format
- Read and Update Format
- Common link element
- The details
- Create Format
- Read and Update Format
- Assigning HTTP Methods
- Browsing All Orders, Customers, or Products
- Obtaining Individual Orders, Customers, or Products
- Creating an Order, Customer, or Product
- Creating with PUT
- Creating with POST
- Updating an Order, Customer, or Product
- Removing an Order, Customer, or Product
- Cancelling an Order
- Overloading the meaning of DELETE
- States versus operations
- Wrapping Up
- 3. Your First JAX-RS Service
- Developing a JAX-RS RESTful Service
- Customer: The Data Class
- CustomerResource: Our JAX-RS Service
- Creating customers
- Retrieving customers
- Updating a customer
- Utility methods
- JAX-RS and Java Interfaces
- Inheritance
- Deploying Our Service
- Writing a Client
- Wrapping Up
- Developing a JAX-RS RESTful Service
- 4. HTTP Method and URI Matching
- Binding HTTP Methods
- HTTP Method Extensions
- @Path
- Binding URIs
- @Path Expressions
- Template parameters
- Regular expressions
- Precedence rules
- Encoding
- Matrix Parameters
- Subresource Locators
- Full Dynamic Dispatching
- Gotchas in Request Matching
- Wrapping Up
- Binding HTTP Methods
- 5. JAX-RS Injection
- The Basics
- @PathParam
- More Than One Path Parameter
- Scope of Path Parameters
- PathSegment and Matrix Parameters
- Matching with multiple PathSegments
- Programmatic URI Information
- @MatrixParam
- @QueryParam
- Programmatic Query Parameter Information
- @FormParam
- @HeaderParam
- Raw Headers
- @CookieParam
- @BeanParam
- Common Functionality
- Automatic Java Type Conversion
- Primitive type conversion
- Java object conversion
- ParamConverters
- Collections
- Conversion failures
- @DefaultValue
- @Encoded
- Automatic Java Type Conversion
- Wrapping Up
- 6. JAX-RS Content Handlers
- Built-in Content Marshalling
- javax.ws.rs.core.StreamingOutput
- java.io.InputStream, java.io.Reader
- java.io.File
- byte[]
- String, char[]
- MultivaluedMap<String, String> and Form Input
- javax.xml.transform.Source
- JAXB
- Intro to JAXB
- JAXB JAX-RS Handlers
- Managing your own JAXBContexts with ContextResolvers
- JAXB and JSON
- XML to JSON using BadgerFish
- JSON and JSON Schema
- Custom Marshalling
- MessageBodyWriter
- Adding pretty printing
- Pluggable JAXBContexts using ContextResolvers
- MessageBodyReader
- Life Cycle and Environment
- MessageBodyWriter
- Wrapping Up
- Built-in Content Marshalling
- 7. Server Responses and Exception Handling
- Default Response Codes
- Successful Responses
- Error Responses
- Complex Responses
- Returning Cookies
- The Status Enum
- javax.ws.rs.core.GenericEntity
- Exception Handling
- javax.ws.rs.WebApplicationException
- Exception Mapping
- Exception Hierarchy
- Mapping default exceptions
- Wrapping Up
- Default Response Codes
- 8. JAX-RS Client API
- Client Introduction
- Bootstrapping with ClientBuilder
- Client and WebTarget
- Building and Invoking Requests
- Invocation
- Exception Handling
- Configuration Scopes
- Wrapping Up
- 9. HTTP Content Negotiation
- Conneg Explained
- Preference Ordering
- Language Negotiation
- Encoding Negotiation
- JAX-RS and Conneg
- Method Dispatching
- Leveraging Conneg with JAXB
- Complex Negotiation
- Viewing Accept headers
- Variant processing
- Negotiation by URI Patterns
- Leveraging Content Negotiation
- Creating New Media Types
- Flexible Schemas
- Wrapping Up
- Conneg Explained
- 10. HATEOAS
- HATEOAS and Web Services
- Atom Links
- Advantages of Using HATEOAS with Web Services
- Location transparency
- Decoupling interaction details
- Reduced state transition errors
- W3C standardized relationships
- Link Headers Versus Atom Links
- HATEOAS and JAX-RS
- Building URIs with UriBuilder
- Relative URIs with UriInfo
- Building Links and Link Headers
- Writing Link Headers
- Embedding Links in XML
- Wrapping Up
- HATEOAS and Web Services
- 11. Scaling JAX-RS Applications
- Caching
- HTTP Caching
- Expires Header
- Cache-Control
- Revalidation and Conditional GETs
- Last-Modified
- ETag
- JAX-RS and conditional GETs
- Concurrency
- JAX-RS and Conditional Updates
- Wrapping Up
- Caching
- 12. Filters and Interceptors
- Server-Side Filters
- Server Request Filters
- Server Response Filters
- Reader and Writer Interceptors
- Client-Side Filters
- Deploying Filters and Interceptors
- Ordering Filters and Interceptors
- Per-JAX-RS Method Bindings
- DynamicFeature
- Name Bindings
- DynamicFeature Versus @NameBinding
- Exception Processing
- Wrapping Up
- Server-Side Filters
- 13. Asynchronous JAX-RS
- AsyncInvoker Client API
- Using Futures
- Exception handling
- Using Callbacks
- Futures Versus Callbacks
- Using Futures
- Server Asynchronous Response Processing
- AsyncResponse API
- Exception Handling
- Cancel
- Status Methods
- Timeouts
- Callbacks
- Use Cases for AsyncResponse
- Server-side push
- Publish and subscribe
- Priority scheduling
- Wrapping Up
- AsyncInvoker Client API
- 14. Deployment and Integration
- Deployment
- The Application Class
- Deployment Within a JAX-RS-Aware Container
- Deployment Within a JAX-RS-Unaware Container
- Configuration
- Basic Configuration
- EJB Integration
- Spring Integration
- Wrapping Up
- Deployment
- 15. Securing JAX-RS
- Authentication
- Basic Authentication
- Digest Authentication
- Client Certificate Authentication
- Authorization
- Authentication and Authorization in JAX-RS
- Enforcing Encryption
- Authorization Annotations
- Programmatic Security
- Client Security
- Verifying the Server
- OAuth 2.0
- Signing and Encrypting Message Bodies
- Digital Signatures
- DKIM/DOSETA
- JOSE JWS
- Encrypting Representations
- Digital Signatures
- Wrapping Up
- Authentication
- 16. Alternative Java Clients
- java.net.URL
- Caching
- Authentication
- Client Certificate Authentication
- Advantages and Disadvantages
- Apache HttpClient
- Authentication
- Client Certificate authentication
- Advantages and Disadvantages
- Authentication
- RESTEasy Client Proxies
- Advantages and Disadvantages
- Wrapping Up
- java.net.URL
- 1. Introduction to REST
- II. JAX-RS Workbook
- 17. Workbook Introduction
- Installing RESTEasy and the Examples
- Example Requirements and Structure
- Code Directory Structure
- Environment Setup
- 18. Examples for Chapter 3
- Build and Run the Example Program
- Deconstructing pom.xml
- Running the Build
- Examining the Source Code
- Build and Run the Example Program
- 19. Examples for Chapter 4
- Example ex04_1: HTTP Method Extension
- Build and Run the Example Program
- The Server Code
- The Client Code
- Example ex04_2: @Path with Expressions
- Build and Run the Example Program
- The Server Code
- The Client Code
- Example ex04_3: Subresource Locators
- Build and Run the Example Program
- The Server Code
- The Client Code
- Example ex04_1: HTTP Method Extension
- 20. Examples for Chapter 5
- Example ex05_1: Injecting URI Information
- The Server Code
- The Client Code
- Build and Run the Example Program
- Example ex05_2: Forms and Cookies
- The Server Code
- Build and Run the Example Program
- Example ex05_1: Injecting URI Information
- 21. Examples for Chapter 6
- Example ex06_1: Using JAXB
- The Client Code
- Changes to pom.xml
- Build and Run the Example Program
- Example ex06_2: Creating a Content Handler
- The Content Handler Code
- The Resource Class
- The Application Class
- The Client Code
- Build and Run the Example Program
- Example ex06_1: Using JAXB
- 22. Examples for Chapter 7
- Example ex07_1: ExceptionMapper
- The Client Code
- Build and Run the Example Program
- Example ex07_1: ExceptionMapper
- 23. Examples for Chapter 9
- Example ex09_1: Conneg with JAX-RS
- The Client Code
- Build and Run the Example Program
- Example ex09_2: Conneg via URL Patterns
- The Server Code
- Build and Run the Example Program
- Example ex09_1: Conneg with JAX-RS
- 24. Examples for Chapter 10
- Example ex10_1: Atom Links
- The Server Code
- The Client Code
- Build and Run the Example Program
- Example ex10_2: Link Headers
- The Server Code
- OrderResource
- StoreResource
- The Client Code
- Build and Run the Example Program
- The Server Code
- Example ex10_1: Atom Links
- 25. Examples for Chapter 11
- Example ex11_1: Caching and Concurrent Updates
- The Server Code
- The Client Code
- Build and Run the Example Program
- Example ex11_1: Caching and Concurrent Updates
- 26. Examples for Chapter 12
- Example ex12_1 : ContainerResponseFilter and DynamicFeature
- The Server Code
- The Client Code
- Build and Run the Example Program
- Example ex12_2: Implementing a WriterInterceptor
- The Client Code
- Build and Run the Example Program
- Example ex12_1 : ContainerResponseFilter and DynamicFeature
- 27. Examples for Chapter 13
- Example ex13_1: Chat REST Interface
- The Client Code
- The Server Code
- Posting a new message
- Handling poll requests
- Build and Run the Example Program
- Example ex13_1: Chat REST Interface
- 28. Examples for Chapter 14
- Example ex14_1: EJB and JAX-RS
- Project Structure
- The EJBs
- The Remaining Server Code
- The ExceptionMappers
- Changes to Application class
- The Client Code
- Build and Run the Example Program
- Example ex14_2: Spring and JAX-RS
- Build and Run the Example Program
- Example ex14_1: EJB and JAX-RS
- 29. Examples for Chapter 15
- Example ex15_1: Custom Security
- One-Time Password Authentication
- The server code
- Allowed-per-Day Access Policy
- The client code
- Build and Run the Example Program
- One-Time Password Authentication
- Example ex15_1: JSON Web Encryption
- Build and Run the Example Program
- Example ex15_1: Custom Security
- 17. Workbook Introduction
- Index
- Colophon
- Copyright