Python in a Nutshell. 4th Edition - Helion
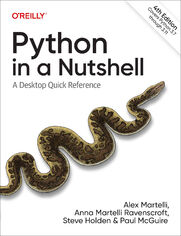
ISBN: 9781098113513
stron: 738, Format: ebook
Data wydania: 2023-01-09
Księgarnia: Helion
Cena książki: 305,15 zł (poprzednio: 359,00 zł)
Oszczędzasz: 15% (-53,85 zł)
Python was recently ranked as today's most popular programming language on the TIOBE index, thanks to its broad applicability to design and prototyping to testing, deployment, and maintenance. With this updated fourth edition, you'll learn how to get the most out of Python, whether you're a professional programmer or someone who needs this language to solve problems in a particular field.
Carefully curated by recognized experts in Python, this new edition focuses on version 3.10, bringing this seminal work on the Python language fully up to date on five version releases, including preview coverage of upcoming 3.11 features.
This handy guide will help you:
- Learn how Python represents data and program as objects
- Understand the value and uses of type annotations
- Examine which language features appeared in which recent versions
- Discover how to use modern Python idiomatically
- Learn ways to structure Python projects appropriately
- Understand how to debug Python code
Osoby które kupowały "Python in a Nutshell. 4th Edition", wybierały także:
- Windows Media Center. Domowe centrum rozrywki 66,67 zł, (8,00 zł -88%)
- Ruby on Rails. Ćwiczenia 18,75 zł, (3,00 zł -84%)
- Efekt piaskownicy. Jak szefować żeby roboty nie zabrały ci roboty 59,50 zł, (11,90 zł -80%)
- Przywództwo w świecie VUCA. Jak być skutecznym liderem w niepewnym środowisku 58,64 zł, (12,90 zł -78%)
- Scrum. O zwinnym zarządzaniu projektami. Wydanie II rozszerzone 58,64 zł, (12,90 zł -78%)
Spis treści
Python in a Nutshell. 4th Edition eBook -- spis treści
- Preface
- How To Use This Book
- Part I, Getting Started with Python
- Part II, Core Python Language and Built-ins
- Part III, Python Library and Extension Modules
- Part IV, Network and Web Programming
- Part V, Extending, Distributing, and Version Upgrade and Migration
- Conventions Used in This Book
- Reference Conventions
- Version Conventions
- Typographic Conventions
- Using Code Examples
- OReilly Online Learning
- How to Contact Us
- Acknowledgments
- How To Use This Book
- 1. Introduction to Python
- The Python Language
- The Python Standard Library and Extension Modules
- Python Implementations
- CPython
- PyPy
- Choosing Between CPython, PyPy, and Other Implementations
- Other Developments, Implementations, and Distributions
- Jython and IronPython
- Numba
- Pyjion
- IPython
- MicroPython
- Anaconda and Miniconda
- pyenv: Simple support for multiple versions
- Transcrypt: Convert your Python to JavaScript
- Licensing and Price Issues
- Python Development and Versions
- Python Resources
- Documentation
- Python documentation for nonprogrammers
- Extension modules and Python sources
- Books
- Community
- Python Software Foundation
- Workgroups
- Python conferences
- User groups and organizations
- Mailing lists
- Social media
- Documentation
- Installation
- Installing Python from Binaries
- Installing Python from Source Code
- Microsoft Windows
- Uncompressing and unpacking the Python source code
- Building the Python source code
- Unix-Like Platforms
- Uncompressing and unpacking the Python source code
- Configuring, building, and testing
- Installing after the build
- Microsoft Windows
- 2. The Python Interpreter
- The python Program
- Environment Variables
- Command-Line Syntax and Options
- The Windows py Launcher
- The PyPy Interpreter
- Interactive Sessions
- Python Development Environments
- IDLE
- Other Python IDEs
- Free Text Editors with Python Support
- Tools for Checking Python Programs
- Running Python Programs
- Running Python in the Browser
- PyScript
- Jupyter
- The python Program
- 3. The Python Language
- Lexical Structure
- Lines and Indentation
- Character Sets
- Tokens
- Identifiers
- Keywords
- Operators
- Delimiters
- Literals
- Statements
- Simple statements
- Compound statements
- Data Types
- Numbers
- Integer numbers
- Floating-point numbers
- Complex numbers
- Underscores in numeric literals
- Sequences
- Iterables
- Strings
- bytes objects
- bytearray objects
- Tuples
- Lists
- Sets
- Dictionaries
- None
- Ellipsis (...)
- Callables
- Boolean Values
- Numbers
- Variables and Other References
- Variables
- Object attributes and items
- Accessing nonexistent references
- Assignment Statements
- Plain assignment
- Augmented assignment
- del Statements
- Variables
- Expressions and Operators
- Comparison Chaining
- Short-Circuiting Operators
- The conditional operator
- Assignment Expressions
- := in an if/elif statement
- := in a while statement
- := in a list comprehension filter
- Numeric Operations
- Numeric Conversions
- Arithmetic Operations
- Division
- Exponentiation
- Comparisons
- Bitwise Operations on Integers
- Sequence Operations
- Sequences in General
- Sequence conversions
- Concatenation and repetition
- Membership testing
- Indexing a sequence
- Slicing a sequence
- Strings
- Tuples
- Lists
- Modifying a list
- In-place operations on a list
- List methods
- Sorting a list
- Sequences in General
- Set Operations
- Set Membership
- Set Methods
- Dictionary Operations
- Dictionary Membership
- Indexing a Dictionary
- Dictionary Methods
- Control Flow Statements
- The if Statement
- The match Statement
- Building patterns
- Literal patterns
- The wildcard pattern
- Capture patterns
- Value patterns
- OR patterns
- Group patterns
- Sequence patterns
- as patterns
- Mapping patterns
- Class patterns
- Guards
- Configuring classes for positional matching
- The while Statement
- The for Statement
- Iterators
- Iterables versus iterators
- range
- List comprehensions
- Set comprehensions
- Dictionary comprehensions
- The break Statement
- The continue Statement
- The else Clause on Loop Statements
- The pass Statement
- The try and raise Statements
- The with Statement
- Functions
- Defining Functions: The def Statement
- Parameters
- Positional parameters
- Named parameters
- Positional-only marker
- Positional argument collector
- Named argument collector
- Parameter sequence
- Mutable default parameter values
- Argument collector parameters
- Attributes of Function Objects
- Docstrings
- Other attributes of function objects
- Function Annotations
- The return Statement
- Calling Functions
- Positional and named arguments
- Keyword-only parameters
- Matching arguments to parameters
- The semantics of argument passing
- Namespaces
- The global statement
- Nested functions and nested scopes
- lambda Expressions
- Generators
- yield from
- Generators as near-coroutines
- Generator expressions
- Recursion
- Lexical Structure
- 4. Object-Oriented Python
- Classes and Instances
- Python Classes
- The class Statement
- The Class Body
- Attributes of class objects
- Function definitions in a class body
- Class-private variables
- Class documentation strings
- Descriptors
- Overriding and nonoverriding descriptors
- Instances
- __init__
- Attributes of instance objects
- The factory function idiom
- __new__
- Attribute Reference Basics
- Getting an attribute from a class
- Getting an attribute from an instance
- Setting an attribute
- Bound and Unbound Methods
- Inheritance
- Method resolution order
- Overriding attributes
- Delegating to superclass methods
- Cooperative superclass method calling
- Dynamic class definition using the type built-in function
- Deleting class attributes
- The Built-in object Type
- Class-Level Methods
- Static methods
- Class methods
- Properties
- Why properties are important
- Properties and inheritance
- __slots__
- __getattribute__
- Per Instance Methods
- Inheritance from Built-in Types
- Special Methods
- General-Purpose Special Methods
- Special Methods for Containers
- Sequences
- Mappings
- Sets
- Container slicing
- Container methods
- Abstract Base Classes
- The abc module
- ABCs in the collections module
- ABCs in the numbers module
- Special Methods for Numeric Objects
- Decorators
- Metaclasses
- Alternatives to Custom Metaclasses for Simple Class Customization
- How Python Determines a Classs Metaclass
- How a Metaclass Creates a Class
- Defining and using your own metaclasses
- A substantial custom metaclass example
- Data Classes
- Enumerated Types (Enums)
- Classes and Instances
- 5. Type Annotations
- History
- Type-Checking Utilities
- mypy
- Other Type Checkers
- Type Annotation Syntax
- The typing Module
- Types
- Type Expression Parameters
- Abstract Base Classes
- Protocols
- Utilities and Decorators
- Defining Custom Types
- Generics and TypeVars
- Restricting TypeVar to specific types
- NamedTuple
- TypedDict
- TypeAlias
- NewType
- Using Type Annotations at Runtime
- How to Add Type Annotations to Your Code
- Adding Type Annotations to New Code
- Adding Type Annotations to Existing Code (Gradual Typing)
- Using .pyi Stub Files
- Summary
- 6. Exceptions
- The try Statement
- try/except
- try/finally
- try/except/finally
- The raise Statement
- The with Statement and Context Managers
- Generators and Exceptions
- Exception Propagation
- Exception Objects
- The Hierarchy of Standard Exceptions
- Standard Exception Classes
- OSError subclasses
- Exceptions wrapping other exceptions or tracebacks
- Custom Exception Classes
- Custom Exceptions and Multiple Inheritance
- Other Exceptions Used in the Standard Library
- ExceptionGroup and except*
- Error-Checking Strategies
- LBYL Versus EAFP
- Handling Errors in Large Programs
- Logging Errors
- The logging module
- Configuring logging
- The assert Statement
- The try Statement
- 7. Modules and Packages
- Module Objects
- The import Statement
- The module body
- Attributes of module objects
- Python built-ins
- Module documentation strings
- Module-private variables
- The from Statement
- from...import *
- from versus import
- Handling import failures
- The import Statement
- Module Loading
- Built-in Modules
- Searching the Filesystem for a Module
- The Main Program
- Reloading Modules
- Circular Imports
- Custom Importers
- Rebinding __import__
- Import hooks
- Packages
- Special Attributes of Package Objects
- Absolute Versus Relative Imports
- Distribution Utilities (distutils) and setuptools
- Python Environments
- Enter the Virtual Environment
- What Is a Virtual Environment?
- Creating and Deleting Virtual Environments
- Working with Virtual Environments
- Managing Dependency Requirements
- Other Environment Management Solutions
- Best Practices with Virtualenvs
- Module Objects
- 8. Core Built-ins and Standard Library Modules
- Built-in Types
- Built-in Functions
- The sys Module
- The copy Module
- The collections Module
- ChainMap
- Counter
- OrderedDict
- defaultdict
- deque
- The functools Module
- The heapq Module
- The DecorateSortUndecorate Idiom
- The argparse Module
- The itertools Module
- 9. Strings and Things
- Methods of String Objects
- The string Module
- String Formatting
- Formatted String Literals (F-Strings)
- Debug printing with f-strings
- Formatting Using format Calls
- Value Conversion
- Value Formatting: The Format Specifier
- Fill and alignment
- Sign indication
- Zero normalization (z)
- Radix indicator (#)
- Leading zero indicator (0)
- Field width
- Grouping option
- Precision specification
- Format type
- Nested Format Specifications
- Formatting of User-Coded Classes
- Legacy String Formatting with %
- Format Specifier Syntax
- Formatted String Literals (F-Strings)
- Text Wrapping and Filling
- The pprint Module
- The reprlib Module
- Unicode
- The codecs Module
- The unicodedata Module
- 10. Regular Expressions
- Regular Expressions and the re Module
- REs and bytes Versus str
- Pattern String Syntax
- Common Regular Expression Idioms
- Sets of Characters
- Alternatives
- Groups
- Optional Flags
- Match Versus Search
- Anchoring at String Start and End
- Regular Expression Objects
- Match Objects
- Functions of the re Module
- REs and the := Operator
- The Third-Party regex Module
- Regular Expressions and the re Module
- 11. File and Text Operations
- The io Module
- Creating a File Object with open
- mode
- Binary and text modes
- Buffering
- Sequential and nonsequential (random) access
- Attributes and Methods of File Objects
- Iteration on File Objects
- File-Like Objects and Polymorphism
- Creating a File Object with open
- The tempfile Module
- Auxiliary Modules for File I/O
- The fileinput Module
- The struct Module
- In-Memory Files: io.StringIO and io.BytesIO
- Archived and Compressed Files
- The tarfile Module
- The TarFile class
- The TarInfo class
- The zipfile Module
- The ZipFile class
- The ZipInfo class
- The tarfile Module
- The os Module
- Filesystem Operations
- Path-string attributes of the os module
- Permissions
- File and directory functions of the os module
- File descriptor operations
- The os.path Module
- OSError Exceptions
- Filesystem Operations
- The errno Module
- The pathlib Module
- The stat Module
- The filecmp Module
- The fnmatch Module
- The glob Module
- The shutil Module
- Text Input and Output
- Standard Output and Standard Error
- The print Function
- Standard Input
- The getpass Module
- Richer-Text I/O
- The readline Module
- Console I/O
- curses
- The msvcrt module
- Internationalization
- The locale Module
- The gettext Module
- Using gettext for localization
- Essential gettext functions
- More Internationalization Resources
- The io Module
- 12. Persistence and Databases
- Serialization
- The csv Module
- csv functions and classes
- A csv example
- The json Module
- json functions
- A json example
- The pickle Module
- pickle functions and classes
- A pickling example
- Pickling instances
- Pickling customization with the copyreg module
- The shelve Module
- A shelving example
- The csv Module
- DBM Modules
- The dbm Package
- Examples of DBM-Like File Use
- The Python Database API (DBAPI)
- Exception Classes
- Thread Safety
- Parameter Style
- Factory Functions
- Type Description Attributes
- The connect Function
- Connection Objects
- Cursor Objects
- DBAPI-Compliant Modules
- SQLite
- The sqlite3.Connection class
- The sqlite3.Row class
- A sqlite3 example
- Serialization
- 13. Time Operations
- The time Module
- The datetime Module
- The date Class
- The time Class
- The datetime Class
- The timedelta Class
- The tzinfo Abstract Class
- The timezone Class
- The zoneinfo Module
- The dateutil Module
- The sched Module
- The calendar Module
- 14. Customizing Execution
- Per-Site Customization
- Termination Functions
- Dynamic Execution and exec
- Avoiding exec
- Expressions
- compile and Code Objects
- Never exec or eval Untrusted Code
- Internal Types
- Type Objects
- The Code Object Type
- The Frame Type
- Garbage Collection
- The gc Module
- Instrumenting garbage collection
- The weakref Module
- The gc Module
- 15. Concurrency: Threads and Processes
- Threads in Python
- The threading Module
- Thread Objects
- Thread Synchronization Objects
- Timeout parameters
- Lock and RLock objects
- Condition objects
- Event objects
- Semaphore and BoundedSemaphore objects
- Timer objects
- Barrier objects
- Thread Local Storage
- The queue Module
- The multiprocessing Module
- Differences Between multiprocessing and threading
- Structural differences
- The Process class
- Differences in queues
- Sharing State: Classes Value, Array, and Manager
- The Value class
- The Array class
- The Manager class
- Process Pools
- The Pool class
- The AsyncResult class
- The ThreadPool class
- Differences Between multiprocessing and threading
- The concurrent.futures Module
- Threaded Program Architecture
- Process Environment
- Running Other Programs
- Using the Subprocess Module
- What to run, and how
- Subprocess files
- Other, advanced arguments
- Attributes of subprocess.Popen instances
- Methods of subprocess.Popen instances
- Running Other Programs with the os Module
- Using the Subprocess Module
- The mmap Module
- Methods of mmap Objects
- Using mmap Objects for IPC
- 16. Numeric Processing
- Floating-Point Values
- The math and cmath Modules
- The statistics Module
- The operator Module
- Random and Pseudorandom Numbers
- The random Module
- Crypto-Quality Random Numbers: The secrets Module
- The fractions Module
- The decimal Module
- Array Processing
- The array Module
- Extensions for Numeric Array Computation
- NumPy
- Creating a NumPy array
- Shape, indexing, and slicing
- Matrix operations in NumPy
- SciPy
- Additional numeric packages
- 17. Testing, Debugging, and Optimizing
- Testing
- Unit Testing and System Testing
- The doctest Module
- The unittest Module
- The TestCase class
- Unit tests dealing with large amounts of data
- Testing with nose2
- Testing with pytest
- Debugging
- Before You Debug
- The inspect Module
- Introspecting callables
- An example of using inspect
- The traceback Module
- The pdb Module
- Other Debugging Modules
- ipdb
- pudb
- The warnings Module
- Classes
- Objects
- Filters
- Functions
- Optimization
- Developing a Fast-Enough Python Application
- Benchmarking
- Large-Scale Optimization
- List operations
- String operations
- Dictionary operations
- Set operations
- Summary of big-O times for operations on Python built-in types
- Profiling
- The profile module
- Calibration
- The pstats module
- Small-Scale Optimization
- The timeit module
- Memoizing
- Precomputing a lookup table
- Building up a string from pieces
- Searching and sorting
- Avoid exec and from ... import *
- Short-circuiting of Boolean expressions
- Short-circuiting of iterators
- Optimizing loops
- Using multiprocessing for heavy CPU work
- Optimizing I/O
- Testing
- 18. Networking Basics
- The Berkeley Socket Interface
- Socket Addresses
- Client/Server Computing
- Connectionless client and server structures
- Connection-oriented client and server structures
- The socket Module
- Socket Objects
- A Connectionless Socket Client
- A Connectionless Socket Server
- A Connection-Oriented Socket Client
- A Connection-Oriented Socket Server
- Transport Layer Security
- SSLContext
- The Berkeley Socket Interface
- 19. Client-Side Network Protocol Modules
- Email Protocols
- The poplib Module
- The smtplib Module
- HTTP and URL Clients
- URL Access
- The urllib Package
- The urllib.parse module
- The urllib.request module
- The Third-Party requests Package
- Sending requests
- requests optional named parameters
- The files argument (and other ways to specify the requests body)
- How to interpret requests examples
- The Response class
- Other Network Protocols
- Email Protocols
- 20. Serving HTTP
- http.server
- WSGI
- WSGI Servers
- ASGI
- Python Web Frameworks
- Full-Stack Versus Lightweight Frameworks
- A Few Popular Full-Stack Frameworks
- Considerations When Using Lightweight Frameworks
- A Few Popular Lightweight Frameworks
- Flask
- Flask request objects
- Flask response objects
- FastAPI
- Flask
- 21. Email, MIME, and Other Network Encodings
- MIME and Email Format Handling
- Functions in the email Package
- The email.message Module
- The email.Generator Module
- Creating Messages
- The email.encoders Module
- The email.utils Module
- Example Uses of the email Package
- Encoding Binary Data as ASCII Text
- The base64 Module
- The quopri Module
- The uu Module
- MIME and Email Format Handling
- 22. Structured Text: HTML
- The html.entities Module
- The BeautifulSoup Third-Party Package
- The BeautifulSoup Class
- Which parser BeautifulSoup uses
- BeautifulSoup, Unicode, and encoding
- The Navigable Classes of bs4
- Indexing instances of Tag
- Getting an actual string
- Attribute references on instances of BeautifulSoup and Tag
- contents, children, and descendants
- parent and parents
- next_sibling, previous_sibling, next_siblings, and previous_siblings
- next_element, previous_element, next_elements, and previous_elements
- bs4 find Methods (aka Search Methods)
- Arguments of search methods
- Filters
- name
- string
- attrs
- Other named arguments
- Arguments of search methods
- bs4 CSS Selectors
- An HTML Parsing Example with BeautifulSoup
- The BeautifulSoup Class
- Generating HTML
- Editing and Creating HTML with bs4
- Building and adding new nodes
- Replacing and removing nodes
- Building HTML with bs4
- Templating
- The jinja2 Package
- The jinja2.Environment class
- The jinja2.Template class
- Building HTML with jinja2
- Editing and Creating HTML with bs4
- 23. Structured Text: XML
- ElementTree
- The Element Class
- The ElementTree Class
- Functions in the ElementTree Module
- Parsing XML with ElementTree.parse
- Selecting Elements from an ElementTree
- Editing an ElementTree
- Building an ElementTree from Scratch
- Parsing XML Iteratively
- ElementTree
- 24. Packaging Programs and Extensions
- What We Dont Cover in This Chapter
- A Brief History of Python Packaging
- Online Material
- 25. Extending and Embedding Classic Python
- Online Material
- 26. v3.7 to v3.n Migration
- Significant Changes in Python Through 3.11
- Planning a Python Version Upgrade
- Choosing a Target Version
- Scoping the Work
- Applying the Code Changes
- Upgrade Automation Using pyupgrade
- Multiversion Testing
- Use a Controlled Deployment Process
- How Often Should You Upgrade?
- Summary
- A. New Features and Changes in Python 3.7 Through 3.11
- Python 3.7
- Python 3.8
- Python 3.9
- Python 3.10
- Python 3.11
- Index