Learning PHP Design Patterns - Helion
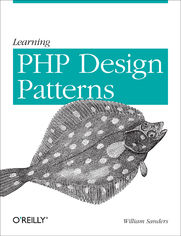
ISBN: 978-14-493-4487-0
stron: 362, Format: ebook
Data wydania: 2013-02-11
Księgarnia: Helion
Cena książki: 126,65 zł (poprzednio: 147,27 zł)
Oszczędzasz: 14% (-20,62 zł)
Build server-side applications more efficiently—and improve your PHP programming skills in the process—by learning how to use design patterns in your code. This book shows you how to apply several object-oriented patterns through simple examples, and demonstrates many of them in full-fledged working applications.
Learn how these reusable patterns help you solve complex problems, organize object-oriented code, and revise a big project by only changing small parts. With Learning PHP Design Patterns, you’ll learn how to adopt a more sophisticated programming style and dramatically reduce development time.
- Learn design pattern concepts, including how to select patterns to handle specific problems
- Get an overview of object-oriented programming concepts such as composition, encapsulation, polymorphism, and inheritance
- Apply creational design patterns to create pages dynamically, using a factory method instead of direct instantiation
- Make changes to existing objects or structure without having to change the original code, using structural design patterns
- Use behavioral patterns to help objects work together to perform tasks
- Interact with MySQL, using behavioral patterns such as Proxy and Chain of Responsibility
- Explore ways to use PHP’s built-in design pattern interfaces
Osoby które kupowały "Learning PHP Design Patterns", wybierały także:
- Tablice informatyczne. PHP7 19,67 zł, (5,90 zł -70%)
- PHP i jQuery. Techniki zaawansowane. Wydanie II 65,31 zł, (20,90 zł -68%)
- PHP. Kurs video. Tworzenie własnego środowiska na podstawie wzorca MVC 119,00 zł, (53,55 zł -55%)
- Rzeczywistość wirtualna (VR) dla każdego - Aframe i HTML 5. VR w HTML 5 na każdym urządzeniu z Internetem! Wydanie II 24,90 zł, (12,45 zł -50%)
- Laravel. Kurs video. Poziom pierwszy. Programowanie aplikacji w PHP 79,00 zł, (39,50 zł -50%)
Spis treści
Learning PHP Design Patterns eBook -- spis treści
- Learning PHP Design Patterns
- Dedication
- Preface
- Audience
- Assumptions This Book Makes
- Contents of This Book
- Conventions Used in This Book
- Using Code Examples
- Safari Books Online
- How to Contact Us
- Acknowledgments
- I. Easing into the Fundamentals of Design Patterns
- 1. PHP and Object-Oriented Programming
- Entering into Intermediate and Advanced Programming
- Why Object-Oriented Programming?
- Making Problem Solving Easier
- Modularization
- Classes and Objects
- Single Responsibility Principle
- Constructor Functions in PHP
- The Client as a Requester Class
- What About Speed?
- The Speed of Development and Change
- The Speed of Teams
- Whats Wrong with Sequential and Procedural Programming?
- Sequential Programming
- Procedural Programming
- Pay Me Now or Pay Me Later
- 2. Basic Concepts in OOP
- Abstraction
- Abstract Classes
- Abstract Properties and Methods
- Interfaces
- Interfaces and Constants
- Type Hinting: Almost Data Typing
- Encapsulation
- Everyday Encapsulation
- Protecting Encapsulation through Visibility
- Private
- Protected
- Public
- Getters and Setters
- Inheritance
- Polymorphism
- One Name with Many Implementations
- Built-In Polymorphism in Design Patterns
- Easy Does It
- Abstraction
- 3. Basic Design Pattern Concepts
- The MVC Loosens and Refocuses Programming
- Basic Principles of Design Patterns
- The First Design Pattern Principle
- Using Interface Data Types in Code Hinting
- Abstract Classes and Their Interfaces
- The Second Design Pattern Principle
- Basic Composition Using a Client
- Delegation: The IS-A and HAS-A Difference
- Design Patterns as a Big Cheat Sheet
- Organization of Design Patterns
- Creational patterns
- Structural patterns
- Behavioral patterns
- Class category
- Object category
- Organization of Design Patterns
- Choosing a Design Pattern
- What Causes Redesign?
- What Varies?
- What Is the Difference Between Design Patterns and Frameworks?
- 4. Using UMLs with Design Patterns
- Why Unified Modeling Language (UML)?
- Class Diagrams
- Participant Symbols
- Relationship Notations
- Acquaintance Relations
- Aggregation Relationship
- Inheritance and Implementation Relations
- Creates Relations
- Multiple Relations
- Object Diagrams
- Interaction Diagrams
- The Role of Diagrams and Notations in Object-Oriented Programming
- Tools for UMLs
- Other UMLs
- 1. PHP and Object-Oriented Programming
- II. Creational Design Patterns
- 5. Factory Method Design Pattern
- What Is the Factory Method Pattern?
- When to Use the Factory Method
- A Minimalist Example
- Factory Work
- The Product
- The Client
- Factory Work
- Accommodating Class Changes
- Adding Graphic Elements
- Coordinating Products
- Changing the Text Product
- Changing the Graphic Product
- Adding New Products and Parameterized Requests
- One Factory and Multiple Products
- The New Factories
- The New Products
- The Client with Parameters
- Helper Classes
- File Diagram
- Product Changes: Leave the Interface Alone!
- 6. Prototype Design Pattern
- What Is the Prototype Design Pattern?
- When to Use the Prototype Pattern
- The Clone Function
- Constructor Does Not Relaunch with Clone
- The Constructor Function Should Do No Real Work
- A Minimalist Prototype Example
- Studying Fruit Flies
- The abstract class interface and concrete implementation
- The Client
- Studying Fruit Flies
- Adding OOP to the Prototype
- The Modern Business Organization
- Encapsulation in the Interface
- The Interface Implementations
- The Organizational Client
- Making Changes, Adding Features
- Dynamic Object Instantiation
- Variables to objects
- The Prototype in PHP Land
- 5. Factory Method Design Pattern
- III. Structural Design Patterns
- 7. The Adapter Pattern
- What Is the Adapter Pattern?
- When to Use the Adapter Pattern
- The Adapter Pattern Using Inheritance
- A Minimal Example of a Class Adapter: The Currency Exchange
- Enter the euro
- Creating a euro adapter
- A Minimal Example of a Class Adapter: The Currency Exchange
- The Adapter Pattern Using Composition
- From Desktop to Mobile
- Just the desktop
- Adapting to mobile
- The Client class as participant
- Just the desktop
- Adapters and Change
- From Desktop to Mobile
- 8. Decorator Design Pattern
- What Is the Decorator Pattern?
- When to Use the Decorator Pattern
- Minimalist Decorator
- The Component Interface
- The Decorator Interface
- Concrete Component
- Concrete Decorators
- Maintenance
- Video
- Database
- The Client
- What About Wrappers?
- Primitives in Wrappers
- Built-in Wrappers in PHP
- Design Pattern Wrappers
- Decorators with Multiple Components
- Multiple Concrete Components
- Concrete Decorators with Multiple States and Values
- The Developer Dating Service
- Component interface
- Concrete components
- Decorator with component methods
- Concrete decorators
- The Client
- HTML User Interface (UI)
- The Client Class Passing HTML Data
- From a Variable Name to an Object Instance
- Adding a Decoration
- 7. The Adapter Pattern
- IV. Behavioral Design Patterns
- 9. The Template Method Pattern
- What Is the Template Method Pattern?
- When to Use the Template Method
- Using the Template Method with Images and Captions: A Minimal Example
- The Abstract Class
- The Concrete Class
- The Client
- The Hollywood Principle
- Using the Template Method with Other Design Patterns
- The Clients Reduced Workload
- The Template Method Participants
- The Factory Method Participants
- The Hook in the Template Method Design Pattern
- Setting Up the Hook
- Implementing the Hook
- The Client and Tripping the Hook
- Setting the Boolean with comparison operators
- The Client class
- The Small and Mighty Template Method
- 10. The State Design Pattern
- What Is the State Pattern?
- When to Use the State Pattern?
- The State Machine
- Light On, Light Off: The Minimal State Design Pattern
- Context Is King
- State instances in the Context class
- Calling the state methods: Context trigger methods
- Setting the current state
- The state getters
- The Context class summary
- The States
- OnState
- OffState
- The Client Request through the Context
- Context Is King
- Adding States
- Changing the Interface
- Changing the States
- OffState
- OnState
- BrighterState
- BrightestState
- Updating the Context Class
- An Updated Client
- The Navigator: More Choices and Cells
- Setting Up a Matrix Statechart
- Setting Up the Interface
- The Context
- The States
- Cell1State
- Cell2State
- Cell3State
- Cell4State
- Cell5State
- Cell6State
- Cell7State
- Cell8State
- Cell9State
- The Client Picks a Path
- The State Pattern and PHP
- 9. The Template Method Pattern
- V. MySQL and PHP Design Patterns
- 11. A Universal Class for Connections and a Proxy Pattern for Security
- A Simple Interface and Class for MySQL
- The Pregnant Interface
- Universal MySQL Connection Class and Static Variables
- Easy Client
- The Protection Proxy for Login
- Setting Up Login Registration
- Implementing the Login Proxy
- The login form and the Client
- The Proxy at work
- The real subject
- The Proxy and Real-World Security
- A Simple Interface and Class for MySQL
- 12. The Flexibility of the Strategy Design Pattern
- Encapsulating Algorithms
- Differentiating the Strategy from the State Design Pattern
- No Conditional Statements, Please
- A Family of Algorithms
- A Minimalist Strategy Pattern
- The Client and the Trigger Scripts
- The Context Class and Strategy Interface
- The Concrete Strategies
- DataEntry
- DisplayData
- SearchData
- UpdateData
- DeleteRecord
- Connection interface and class
- Expanded Strategy Pattern with Data Security and Parameterized Algorithms
- A Data Security Helper Class
- Adding a Parameter to an Algorithm Method
- The Survey Table
- Data Entry Modules
- The Client Calls for Help
- The Minor but Major Change in Context Class
- The Concrete Strategies
- DataEntry
- DisplayAll
- SearchData
- UpdateData
- DeleteRecord
- The Flexible Strategy Pattern
- Encapsulating Algorithms
- 13. The Chain of Responsibility Design Pattern
- Passing the Buck
- The Chain of Responsibility in a MySQL Help Desk
- Building and Loading the Response Table
- InsertData.php
- UpdateData.php
- The Help Desk Chain of Responsibility
- HTML Data Entry, Client and Request Participants
- Handler interface and concrete handlers
- Building and Loading the Response Table
- Automated Chain of Responsibility and Factory Method
- The Chain of Responsibility and Date-Driven Requests
- Factory Method Finishes Job
- The Creator and HungerFactory
- The product and individual countries
- Helpers, resources, and style
- Ease of Update
- 14. Building a Multidevice CMS with the Observer Pattern
- Built-In Observer Interfaces
- When to Use the Observer Pattern
- Using SPL with the Observer Pattern
- SplSubject
- SplObserver
- SplObjectStorage
- The SPL Concrete Subject
- The SPL Concrete Observer
- The SPL Client
- Free Range PHP and the Observer Pattern
- The Abstract Subject Class and ConcreteSubject Implementation
- Observer and Multiple Concrete Observers
- ConcreteObserverDT (Desktop implementation)
- ConcreteObserverTablet (Tablet implementation)
- ConcreteObserverPhone (Smartphone implementation)
- The Client
- Making a Simple CMS
- CMS Utilities
- CMS table
- CMS data entry and update
- The Multiple Device Observer
- Two HTML UI documents
- The sniffer client
- The Subject classes
- Multiple concrete observers
- The mobile phone observer
- Tablet observer
- Desktop view
- CMS Utilities
- Thinking OOP
- 11. A Universal Class for Connections and a Proxy Pattern for Security
- Index
- About the Author
- Colophon
- Copyright