Learning Java. An Introduction to Real-World Programming with Java. 5th Edition - Helion
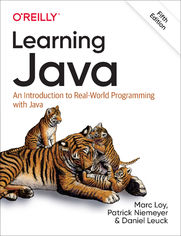
ISBN: 978-14-920-5622-5
stron: 518, Format: ebook
Data wydania: 2020-03-30
Księgarnia: Helion
Cena książki: 269,00 zł
If you’re new to Java—or new to programming—this best-selling book will guide you through the language features and APIs of Java 11. With fun, compelling, and realistic examples, authors Marc Loy, Patrick Niemeyer, and Daniel Leuck introduce you to Java fundamentals—including its class libraries, programming techniques, and idioms—with an eye toward building real applications.
You’ll learn powerful new ways to manage resources and exceptions in your applications—along with core language features included in recent Java versions.
- Develop with Java, using the compiler, interpreter, and other tools
- Explore Java’s built-in thread facilities and concurrency package
- Learn text processing and the powerful regular expressions API
- Write advanced networked or web-based applications and services
Osoby które kupowały "Learning Java. An Introduction to Real-World Programming with Java. 5th Edition", wybierały także:
- Wprowadzenie do Javy. Programowanie i struktury danych. Wydanie XII 193,23 zł, (59,90 zł -69%)
- Spring i Spring Boot. Kurs video. Testowanie aplikacji i bezpiecze 129,00 zł, (51,60 zł -60%)
- Metoda dziel i zwyci 89,00 zł, (35,60 zł -60%)
- JavaFX. Kurs video. Wzorce oraz typy generyczne 79,00 zł, (31,60 zł -60%)
- Platforma Xamarin. Kurs video. Poziom drugi. Zaawansowane techniki tworzenia aplikacji cross-platform 99,00 zł, (39,60 zł -60%)
Spis treści
Learning Java. An Introduction to Real-World Programming with Java. 5th Edition eBook -- spis treści
- Preface
- Who Should Read This Book
- New Developments
- New in This Edition (Java 11, 12, 13, 14)
- Using This Book
- Online Resources
- Conventions Used in This Book
- Using Code Examples
- OReilly Online Learning
- How to Contact Us
- Acknowledgments
- 1. A Modern Language
- Enter Java
- Javas Origins
- Growing Up
- A Virtual Machine
- Java Compared with Other Languages
- Safety of Design
- Simplify, Simplify, Simplify
- Type Safety and Method Binding
- Incremental Development
- Dynamic Memory Management
- Error Handling
- Threads
- Scalability
- Safety of Implementation
- The Verifier
- Class Loaders
- Security Managers
- Application and User-Level Security
- A Java Road Map
- The Past: Java 1.0Java 11
- The Present: Java 14
- Feature overview
- The Future
- Availability
- Enter Java
- 2. A First Application
- Java Tools and Environment
- Installing the JDK
- Installing OpenJDK on Linux
- Installing OpenJDK on macOS
- Installing OpenJDK on Windows
- Configuring IntelliJ IDEA and Creating a Project
- Running the Project
- Grabbing the Learning Java Examples
- HelloJava
- Classes
- The main() Method
- Classes and Objects
- Variables and Class Types
- HelloComponent
- Inheritance
- The JComponent Class
- Relationships and Finger-Pointing
- Package and Imports
- The paintComponent() Method
- HelloJava2: The Sequel
- Instance Variables
- Constructors
- Events
- The repaint() Method
- Interfaces
- Goodbye and Hello Again
- Java Tools and Environment
- 3. Tools of the Trade
- JDK Environment
- The Java VM
- Running Java Applications
- System Properties
- The Classpath
- javap
- Modules
- The Java Compiler
- Trying Java
- JAR Files
- File Compression
- The jar Utility
- JAR manifests
- Making a JAR file runnable
- The pack200 Utility
- Building Up
- 4. The Java Language
- Text Encoding
- Comments
- Javadoc Comments
- Javadoc as metadata
- Annotations
- Javadoc Comments
- Variables and Constants
- Types
- Primitive Types
- Floating-point precision
- Variable declaration and initialization
- Integer literals
- Floating-point literals
- Character literals
- Reference Types
- Inferring Types
- Passing References
- A Word About Strings
- Primitive Types
- Statements and Expressions
- Statements
- if/else conditionals
- switch statements
- do/while loops
- The for loop
- The enhanced for loop
- break/continue
- Unreachable statements
- Expressions
- Operators
- Assignment
- The null value
- Variable access
- Method invocation
- Statements, expressions, and algorithms
- Object creation
- The instanceof operator
- Statements
- Arrays
- Array Types
- Array Creation and Initialization
- Using Arrays
- Anonymous Arrays
- Multidimensional Arrays
- Types and Classes and Arrays, Oh My!
- 5. Objects in Java
- Classes
- Declaring and Instantiating Classes
- Accessing Fields and Methods
- Access modifiers preview
- Static Members
- Methods
- Local Variables
- Shadowing
- The this reference
- Static Methods
- Initializing Local Variables
- Argument Passing and References
- Wrappers for Primitive Types
- Method Overloading
- Object Creation
- Constructors
- Working with Overloaded Constructors
- Object Destruction
- Garbage Collection
- Packages
- Importing Classes
- Importing individual classes
- Importing entire packages
- Skipping imports
- Custom Packages
- Member Visibility and Access
- Compiling with Packages
- Importing Classes
- Advanced Class Design
- Subclassing and Inheritance
- Shadowed variables
- Overriding methods
- Interfaces
- Inner Classes
- Anonymous Inner Classes
- Subclassing and Inheritance
- Organizing Content and Planning for Failure
- Classes
- 6. Error Handling and Logging
- Exceptions
- Exceptions and Error Classes
- Exception Handling
- Bubbling Up
- Stack Traces
- Checked and Unchecked Exceptions
- Throwing Exceptions
- Chaining and rethrowing exceptions
- Narrowed rethrow
- try Creep
- The finally Clause
- try with Resources
- Performance Issues
- Assertions
- Enabling and Disabling Assertions
- Using Assertions
- The Logging API
- Overview
- Loggers
- Handlers
- Filters
- Formatters
- Logging Levels
- A Simple Example
- Logging Setup Properties
- The Logger
- Performance
- Overview
- Real-World Exceptions
- Exceptions
- 7. Collections and Generics
- Collections
- The Collection Interface
- Collection Types
- Set
- List
- Queue
- The Map Interface
- Type Limitations
- Containers: Building a Better Mousetrap
- Can Containers Be Fixed?
- Enter Generics
- Talking About Types
- There Is No Spoon
- Erasure
- Raw Types
- Parameterized Type Relationships
- Why Isnt a List<Date> a List<Object>?
- Casts
- Converting Between Collections and Arrays
- Iterator
- for loop over collections
- A Closer Look: The sort() Method
- Application: Trees on the Field
- Conclusion
- Collections
- 8. Text and Core Utilities
- Strings
- Constructing Strings
- Strings from Things
- Comparing Strings
- Searching
- String Method Summary
- Things from Strings
- Parsing Primitive Numbers
- Tokenizing Text
- StringTokenizer
- Regular Expressions
- Regex Notation
- Write once, run away
- Escaped characters
- Characters and character classes
- Custom character classes
- Position markers
- Iteration (multiplicity)
- Alternation
- Special options
- The java.util.regex API
- Pattern
- The Matcher
- Splitting and tokenizing strings
- Regex Notation
- Math Utilities
- The java.lang.Math Class
- Math in action
- Big/Precise Numbers
- The java.lang.Math Class
- Dates and Times
- Local Dates and Times
- Comparing and Manipulating Dates and Times
- Time Zones
- Parsing and Formatting Dates and Times
- Parsing Errors
- Timestamps
- Other Useful Utilities
- Strings
- 9. Threads
- Introducing Threads
- The Thread Class and the Runnable Interface
- Creating and starting threads
- A natural-born thread
- Controlling Threads
- Deprecated methods
- The sleep() method
- The join() method
- The interrupt() method
- Revisiting animation with threads
- Death of a Thread
- The Thread Class and the Runnable Interface
- Synchronization
- Serializing Access to Methods
- Synchronizing a queue of URLs
- Accessing Class and Instance Variables from Multiple Threads
- Serializing Access to Methods
- Scheduling and Priority
- Thread State
- Time-Slicing
- Priorities
- Yielding
- Thread Performance
- The Cost of Synchronization
- Thread Resource Consumption
- Concurrency Utilities
- Introducing Threads
- 10. Desktop Applications
- Buttons and Sliders and Text Fields, Oh My!
- Component Hierarchies
- Model View Controller Architecture
- Labels and Buttons
- Buttons
- Text Components
- Text fields
- Text areas
- Text scrolling
- Other Components
- JSlider
- JList
- Containers and Layouts
- Frames and Windows
- JPanel
- Layout Managers
- BorderLayout
- GridLayout
- GridBagLayout
- Events
- Mouse Events
- Mouse adapters
- Action Events
- Change Events
- Other Events
- Mouse Events
- Modals and Pop Ups
- Message Dialogs
- Confirmation Dialogs
- Input Dialogs
- Threading Considerations
- SwingUtilities and Component Updates
- Timers
- Animation with Timer
- Other Timer uses
- Next Steps
- Menus
- Preferences
- Custom Components and Java2D
- JavaFX
- User Interface and User Experience
- Buttons and Sliders and Text Fields, Oh My!
- 11. Networking and I/O
- Streams
- Basic I/O
- Character Streams
- Stream Wrappers
- Data streams
- Buffered streams
- PrintWriter and PrintStream
- The java.io.File Class
- File constructors
- Path localization
- File operations
- File Streams
- RandomAccessFile
- The NIO File API
- FileSystem and Path
- Path to classic file and back
- NIO File Operations
- FileSystem and Path
- The NIO Package
- Asynchronous I/O
- Performance
- Mapped and Locked Files
- Channels
- Buffers
- Buffer operations
- Buffer types
- Byte order
- Allocating buffers
- Character Encoders and Decoders
- CharsetEncoder and CharsetDecoder
- FileChannel
- Concurrent access
- File locking
- Network Programming
- Sockets
- Clients and Servers
- Clients
- Servers
- Sockets and security
- The DateAtHost Client
- A Distributed Game
- Setting up the UI
- The game server
- The game client
- The game protocol
- Clients and Servers
- More to Explore
- Streams
- 12. Programming for the Web
- Uniform Resource Locators
- The URL Class
- Stream Data
- Getting the Content as an Object
- Managing Connections
- Handlers in Practice
- Useful Handler Frameworks
- Talking to Web Applications
- Using the GET Method
- Using the POST Method
- The HttpURLConnection
- SSL and Secure Web Communications
- Java Web Applications
- The Servlet Life Cycle
- Servlets
- The HelloClient Servlet
- ServletExceptions
- Content type
- The Servlet Response
- Servlet Parameters
- GET, POST, and extra path
- GET or POST: Which one to use?
- The ShowParameters Servlet
- User Session Management
- The ShowSession Servlet
- Servlet Containers
- Configuration with web.xml and Annotations
- URL Pattern Mappings
- Deploying HelloClient
- Reloading web apps
- The World Wide Web Is, Well, Wide
- 13. Expanding Java
- Java Releases
- JCP and JSRs
- Lambda Expressions
- Retrofitting Your Code
- Feature research
- Basic lambda expressions
- Method references
- Eventful lambdas
- Replacing Runnable
- Retrofitting Your Code
- Expanding Java Beyond the Core
- Final Wrap-Up and Next Steps
- Java Releases
- A. Code Examples and IntelliJ IDEA
- Grabbing the Main Code Examples
- Installing IntelliJ IDEA
- Installing on Linux
- Installing on a macOS
- Installing on Windows
- Importing the Examples
- Running the Examples
- Grabbing the Web Code Examples
- Working with Servlets
- Glossary
- Index