Java in a Nutshell. A Desktop Quick Reference. 7th Edition - Helion
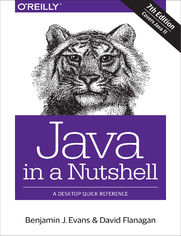
ISBN: 978-14-920-3720-0
stron: 458, Format: ebook
Data wydania: 2018-12-03
Księgarnia: Helion
Cena książki: 209,00 zł
This updated edition of Java in a Nutshell not only helps experienced Java programmers get the most out of Java versions 9 through 11, it’s also a learning path for new developers. Chock full of examples that demonstrate how to take complete advantage of modern Java APIs and development best practices, this thoroughly revised book includes new material on Java Concurrency Utilities.
The book’s first section provides a fast-paced, no-fluff introduction to the Java programming language and the core runtime aspects of the Java platform. The second section is a reference to core concepts and APIs that explains how to perform real programming work in the Java environment.
- Get up to speed on language details, including Java 9-11 changes
- Learn object-oriented programming, using basic Java syntax
- Explore generics, enumerations, annotations, and lambda expressions
- Understand basic techniques used in object-oriented design
- Examine concurrency and memory, and how they’re intertwined
- Work with Java collections and handle common data formats
- Delve into Java’s latest I/O APIs, including asynchronous channels
- Use Nashorn to execute JavaScript on the Java Virtual Machine
- Become familiar with development tools in OpenJDK
Osoby które kupowały "Java in a Nutshell. A Desktop Quick Reference. 7th Edition", wybierały także:
- Maven. Kurs video. Automatyzacja tworzenia aplikacji w Javie 98,98 zł, (39,59 zł -60%)
- Spring i Spring Boot. Kurs video. Testowanie aplikacji i bezpiecze 129,00 zł, (51,60 zł -60%)
- Metoda dziel i zwyci 89,00 zł, (35,60 zł -60%)
- Język C. Kurs video. Praktyczne wprowadzenie do programowania 98,98 zł, (39,59 zł -60%)
- JavaFX. Kurs video. Wzorce oraz typy generyczne 79,00 zł, (31,60 zł -60%)
Spis treści
Java in a Nutshell. A Desktop Quick Reference. 7th Edition eBook -- spis treści
- Foreword
- Preface
- Changes in the Seventh Edition
- Contents of This Book
- Related Books
- Examples Online
- Conventions Used in This Book
- Request for Comments
- OReilly Safari
- Acknowledgments
- I. Introducing Java
- 1. Introduction to the Java Environment
- The Language, the JVM, and the Ecosystem
- What Is the Java Language?
- What Is the JVM?
- What Is the Java Ecosystem?
- A Brief History of Java and the JVM
- The Lifecycle of a Java Program
- Frequently Asked Questions
- What is bytecode?
- Is javac a compiler?
- Why is it called bytecode?
- Is bytecode optimized?
- Is bytecode really machine independent? What about things like endianness?
- Is Java an interpreted language?
- Can other languages run on the JVM?
- Frequently Asked Questions
- Java Security
- Comparing Java to Other Languages
- Java Compared to C
- Java Compared to C++
- Java Compared to Python
- Java Compared to JavaScript
- Answering Some Criticisms of Java
- Overly Verbose
- Slow to Change
- Performance Problems
- Insecure
- Too Corporate
- The Language, the JVM, and the Ecosystem
- 2. Java Syntax from the Ground Up
- Java Programs from the Top Down
- Lexical Structure
- The Unicode Character Set
- Case Sensitivity and Whitespace
- Comments
- Reserved Words
- Identifiers
- Literals
- Punctuation
- Primitive Data Types
- The boolean Type
- The char Type
- String literals
- Integer Types
- Floating-Point Types
- Primitive Type Conversions
- Expressions and Operators
- Operator Summary
- Precedence
- Associativity
- Operator summary table
- Operand number and type
- Return type
- Side effects
- Order of evaluation
- Arithmetic Operators
- String Concatenation Operator
- Increment and Decrement Operators
- Comparison Operators
- Boolean Operators
- Bitwise and Shift Operators
- Assignment Operators
- The Conditional Operator
- The instanceof Operator
- Special Operators
- Operator Summary
- Statements
- Expression Statements
- Compound Statements
- The Empty Statement
- Labeled Statements
- Local Variable Declaration Statements
- The if/else Statement
- The else if clause
- The switch Statement
- The while Statement
- The do Statement
- The for Statement
- The foreach Statement
- What foreach cannot do
- The break Statement
- The continue Statement
- The return Statement
- The synchronized Statement
- The throw Statement
- The try/catch/finally Statement
- try
- catch
- finally
- The try-with-resources Statement
- The assert Statement
- Enabling assertions
- Methods
- Defining Methods
- Method Modifiers
- Checked and Unchecked Exceptions
- Working with checked exceptions
- Variable-Length Argument Lists
- Introduction to Classes and Objects
- Defining a Class
- Creating an Object
- Using an Object
- Object Literals
- String literals
- Type literals
- The null reference
- Lambda Expressions
- Arrays
- Array Types
- Array type widening conversions
- C compatibility syntax
- Creating and Initializing Arrays
- Array initializers
- Using Arrays
- Accessing array elements
- Array bounds
- Iterating arrays
- Copying arrays
- Array utilities
- Multidimensional Arrays
- Array Types
- Reference Types
- Reference Versus Primitive Types
- Manipulating Objects and Reference Copies
- Comparing Objects
- Boxing and Unboxing Conversions
- Packages and the Java Namespace
- Package Declaration
- Globally Unique Package Names
- Importing Types
- Naming conflicts and shadowing
- Importing Static Members
- Static member imports and overloaded methods
- Java Source File Structure
- Defining and Running Java Programs
- Summary
- 3. Object-Oriented Programming in Java
- Overview of Classes
- Basic OO Definitions
- Other Reference Types
- Class Definition Syntax
- Fields and Methods
- Field Declaration Syntax
- Class Fields
- Class Methods
- Instance Fields
- Instance Methods
- How the this Reference Works
- Creating and Initializing Objects
- Defining a Constructor
- Defining Multiple Constructors
- Invoking One Constructor from Another
- Field Defaults and Initializers
- Initializer blocks
- Subclasses and Inheritance
- Extending a Class
- Final classes
- Superclasses, Object, and the Class Hierarchy
- Subclass Constructors
- Constructor Chaining and the Default Constructor
- The default constructor
- Hiding Superclass Fields
- Overriding Superclass Methods
- Overriding is not hiding
- Virtual method lookup
- Invoking an overridden method
- Extending a Class
- Data Hiding and Encapsulation
- Access Control
- Access to modules
- Access to packages
- Access to classes
- Access to members
- Access control and inheritance
- Member access summary
- Data Accessor Methods
- Access Control
- Abstract Classes and Methods
- Reference Type Conversions
- Modifier Summary
- Overview of Classes
- 4. The Java Type System
- Interfaces
- Defining an Interface
- Extending Interfaces
- Implementing an Interface
- Default Methods
- Backward compatibility
- Implementation of default methods
- Marker Interfaces
- Java Generics
- Introduction to Generics
- Generic Types and Type Parameters
- Diamond Syntax
- Type Erasure
- Bounded Type Parameters
- Introducing Covariance
- Wildcards
- Bounded wildcards
- Generic Methods
- Compile and Runtime Typing
- Using and Designing Generic Types
- Enums and Annotations
- Enums
- Annotations
- Defining Custom Annotations
- Type Annotations
- Lambda Expressions
- Lambda Expression Conversion
- Method References
- Functional Programming
- Lexical Scoping and Local Variables
- Nested Types
- Static Member Types
- Features of static member types
- Nonstatic Member Classes
- Features of member classes
- Syntax for member classes
- Local Classes
- Features of local classes
- Scope of a local class
- Anonymous Classes
- Static Member Types
- Non-Denotable Types and var
- Summary
- Interfaces
- 5. Introduction to Object-Oriented Design in Java
- Java Values
- Important Methods of java.lang.Object
- toString()
- equals()
- hashCode()
- Comparable::compareTo()
- clone()
- Aspects of Object-Oriented Design
- Constants
- Interfaces Versus Abstract Classes
- Can Default Methods Be Used as Traits?
- Instance Methods or Class Methods?
- A word about System.out.println()
- Composition Versus Inheritance
- Field Inheritance and Accessors
- Singleton
- Object-Oriented Design with Lambdas
- Lambdas Versus Nested Classes
- Lambdas Versus Method References
- Exceptions and Exception Handling
- Safe Java Programming
- 6. Javas Approach to Memory and Concurrency
- Basic Concepts of Java Memory Management
- Memory Leaks in Java
- Introducing Mark-and-Sweep
- The Basic Mark-and-Sweep Algorithm
- How the JVM Optimizes Garbage Collection
- Evacuation
- The HotSpot Heap
- Other Collectors
- ParallelOld
- Concurrent Mark-and-Sweep
- Finalization
- Finalization Details
- Javas Support for Concurrency
- Thread Lifecycle
- Visibility and Mutability
- Concurrent safety
- Exclusion and Protecting State
- volatile
- Useful Methods of Thread
- getId()
- getPriority() and setPriority()
- setName() and getName()
- getState()
- isAlive()
- start()
- interrupt()
- join()
- setDaemon()
- setUncaughtExceptionHandler()
- Deprecated Methods of Thread
- stop()
- suspend(), resume(), and countStackFrames()
- destroy()
- Working with Threads
- Summary
- Basic Concepts of Java Memory Management
- II. Working with the Java Platform
- 7. Programming and Documentation Conventions
- Naming and Capitalization Conventions
- Practical Naming
- Java Documentation Comments
- Structure of a Doc Comment
- Doc-Comment Tags
- Inline Doc-Comment Tags
- Cross-References in Doc Comments
- Doc Comments for Packages
- Doclets
- Conventions for Portable Programs
- 8. Working with Java Collections
- Introduction to Collections API
- The Collection Interface
- The Set Interface
- The List Interface
- Foreach loops and iteration
- Random access to Lists
- The Map Interface
- The Queue and BlockingQueue Interfaces
- Adding Elements to Queues
- Removing Elements from Queues
- Querying
- Utility Methods
- Special-case collections
- Arrays and Helper Methods
- Java Streams and Lambda Expressions
- Functional Approaches
- Filter
- Map
- forEach
- Reduce
- The Streams API
- Lazy evaluation
- Streams utility default methods
- Functional Approaches
- Summary
- Introduction to Collections API
- 9. Handling Common Data Formats
- Text
- Special Syntax for Strings
- String literals
- toString()
- String concatenation
- String Immutability
- Hash codes and effective immutability
- Regular Expressions
- Special Syntax for Strings
- Numbers and Math
- How Java Represents Integer Types
- Java and Floating-Point Numbers
- BigDecimal
- Javas Standard Library of Mathematical Functions
- Java 8 Date and Time
- Introducing the Java 8 Date and Time API
- The parts of a timestamp
- Example
- Queries
- Adjusters
- Legacy Date and Time
- Introducing the Java 8 Date and Time API
- Summary
- Text
- 10. File Handling and I/O
- Classic Java I/O
- Files
- Streams
- Readers and Writers
- try-with-resources Revisited
- Problems with Classic I/O
- Modern Java I/O
- Files
- Path
- NIO Channels and Buffers
- ByteBuffer
- Mapped Byte Buffers
- Async I/O
- Future-Based Style
- Callback-Based Style
- Watch Services and Directory Searching
- Networking
- HTTP
- TCP
- IP
- Classic Java I/O
- 11. Classloading, Reflection, and Method Handles
- Class Files, Class Objects, and Metadata
- Examples of Class Objects
- Class Objects and Metadata
- Phases of Classloading
- Loading
- Verification
- Preparation and Resolution
- Initialization
- Secure Programming and Classloading
- Applied Classloading
- Classloader Hierarchy
- Bootstrap classloader
- Platform classloader
- Application classloader
- Custom classloader
- Classloader Hierarchy
- Reflection
- When to Use Reflection
- How to Use Reflection
- Method objects
- Problems with Reflection
- Dynamic Proxies
- Method Handles
- MethodType
- Method Lookup
- Invoking Method Handles
- Class Files, Class Objects, and Metadata
- 12. Java Platform Modules
- Why Modules?
- Modularizing the JDK
- Writing Your Own Modules
- Basic Modules Syntax
- Building a Simple Modular Application
- The Module Path
- Automatic Modules
- Open Modules
- Services
- Multi-Release JARs
- Converting to a Multi-Release JAR
- Migrating to Modules
- Custom Runtime Images
- Issues with Modules
- Unsafe and Related Problems
- Lack of Versioning
- Slow Adoption Rates
- Summary
- Why Modules?
- 13. Platform Tools
- Command-Line Tools
- Introduction to JShell
- Summary
- A. Additional Tools
- Introduction to Nashorn
- Non-Java Languages on the JVM
- Motivation
- Executing JavaScript with Nashorn
- Running from the Command Line
- Using the Nashorn Shell
- Nashorn and javax.script
- Introducing javax.script with Nashorn
- The javax.script API
- Introducing javax.script with Nashorn
- Advanced Nashorn
- Calling Java from Nashorn
- JavaClass and JavaPackage
- JavaScript functions and Java lambda expressions
- Nashorns JavaScript Language Extensions
- Foreach loops
- Single expression functions
- Multiple catch clauses
- Under the Hood
- Calling Java from Nashorn
- The Future of Nashorn and GraalVM
- VisualVM
- Introduction to Nashorn
- Index