Java Cookbook. Problems and Solutions for Java Developers. 4th Edition - Helion
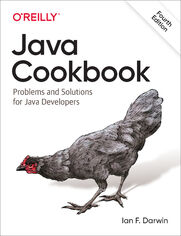
ISBN: 9781492072539
stron: 638, Format: ebook
Data wydania: 2020-03-17
Księgarnia: Helion
Cena książki: 254,15 zł (poprzednio: 299,00 zł)
Oszczędzasz: 15% (-44,85 zł)
Java continues to grow and evolve, and this cookbook continues to evolve in tandem. With this guide, you’ll get up to speed right away with hundreds of hands-on recipes across a broad range of Java topics. You’ll learn useful techniques for everything from string handling and functional programming to network communication.
Each recipe includes self-contained code solutions that you can freely use, along with a discussion of how and why they work. If you’re familiar with Java basics, this cookbook will bolster your knowledge of the language and its many recent changes, including how to apply them in your day-to-day development. This updated edition covers changes through Java 12 and parts of 13 and 14.
Recipes include:
- Blade, Laravel's powerful custom templating tool
- Methods for compiling, running, and debugging
- Packaging Java classes and building applications
- Manipulating, comparing, and rearranging text
- Regular expressions for string and pattern matching
- Handling numbers, dates, and times
- Structuring data with collections, arrays, and other types
- Object-oriented and functional programming techniques
- Input/output, directory, and filesystem operations
- Network programming on both client and server
- Processing JSON for data interchange
- Multithreading and concurrency
- Using Java in big data applications
- Interfacing Java with other languages
Osoby które kupowały "Java Cookbook. Problems and Solutions for Java Developers. 4th Edition", wybierały także:
- Metoda dziel i zwyci 89,00 zł, (26,70 zł -70%)
- Język C. Kurs video. Praktyczne wprowadzenie do programowania 99,00 zł, (29,70 zł -70%)
- Wprowadzenie do Javy. Programowanie i struktury danych. Wydanie XII 193,23 zł, (59,90 zł -69%)
- Spring i Spring Boot. Kurs video. Testowanie aplikacji i bezpiecze 125,42 zł, (45,15 zł -64%)
- Maven. Kurs video. Automatyzacja tworzenia aplikacji w Javie 98,98 zł, (39,59 zł -60%)
Spis treści
Java Cookbook. 4th Edition eBook -- spis treści
- Preface
- Who This Book Is For
- Whats in This Book?
- Java Books
- Conventions Used in This Book
- OReilly Online Learning
- Comments and Questions
- Acknowledgments
- 1. Getting Started: Compiling and Running Java
- 1.0. Introduction
- 1.1. Compiling and Running Java: Standard JDK
- 1.2. Compiling and Running Java: GraalVM for Better Performance
- 1.3. Compiling, Running, and Testing with an IDE
- 1.4. Exploring Java with JShell
- 1.5. Using CLASSPATH Effectively
- 1.6. Downloading and Using the Code Examples
- 1.7. Automating Dependencies, Compilation, Testing, and Deployment with Apache Maven
- 1.8. Automating Dependencies, Compilation, Testing, and Deployment with Gradle
- 1.9. Dealing with Deprecation Warnings
- 1.10. Maintaining Code Correctness with Unit Testing: JUnit
- 1.11. Maintaining Your Code with Continuous Integration
- 1.12. Getting Readable Stack Traces
- 1.13. Finding More Java Source Code
- 1.14. Finding Runnable Java Libraries
- 2. Interacting with the Environment
- 2.0. Introduction
- 2.1. Getting Environment Variables
- 2.2. Getting Information from System Properties
- 2.3. Dealing with Code That Depends on the Java Version or the Operating System
- 2.4. Using Extensions or Other Packaged APIs
- 2.5. Using the Java Modules System
- 3. Strings and Things
- 3.0. Introduction
- 3.1. Taking Strings Apart with Substrings or Tokenizing
- 3.2. Putting Strings Together with StringBuilder
- 3.3. Processing a String One Character at a Time
- 3.4. Aligning, Indenting, and Unindenting Strings
- 3.5. Converting Between Unicode Characters and Strings
- 3.6. Reversing a String by Word or by Character
- 3.7. Expanding and Compressing Tabs
- 3.8. Controlling Case
- 3.9. Entering Nonprintable Characters
- 3.10. Trimming Blanks from the End of a String
- 3.11. Creating a Message with I18N Resources
- 3.12. Using a Particular Locale
- 3.13. Creating a Resource Bundle
- 3.14. Program: A Simple Text Formatter
- 3.15. Program: Soundex Name Comparisons
- 4. Pattern Matching with Regular Expressions
- 4.0. Introduction
- 4.1. Regular Expression Syntax
- 4.2. Using Regexes in Java: Test for a Pattern
- 4.3. Finding the Matching Text
- 4.4. Replacing the Matched Text
- 4.5. Printing All Occurrences of a Pattern
- 4.6. Printing Lines Containing a Pattern
- 4.7. Controlling Case in Regular Expressions
- 4.8. Matching Accented, or Composite, Characters
- 4.9. Matching Newlines in Text
- 4.10. Program: Apache Logfile Parsing
- 4.11. Program: Full Grep
- 5. Numbers
- 5.0. Introduction
- 5.1. Checking Whether a String Is a Valid Number
- 5.2. Converting Numbers to Objects and Vice Versa
- 5.3. Taking a Fraction of an Integer Without Using Floating Point
- 5.4. Working with Floating-Point Numbers
- 5.5. Formatting Numbers
- 5.6. Converting Among Binary, Octal, Decimal, and Hexadecimal
- 5.7. Operating on a Series of Integers
- 5.8. Formatting with Correct Plurals
- 5.9. Generating Random Numbers
- 5.10. Multiplying Matrices
- 5.11. Using Complex Numbers
- 5.12. Handling Very Large Numbers
- 5.13. Program: TempConverter
- 5.14. Program: Number Palindromes
- 6. Dates and Times
- 6.0. Introduction
- 6.1. Finding Todays Date
- 6.2. Formatting Dates and Times
- 6.3. Converting Among Dates/Times, YMDHMS, and Epoch Seconds
- 6.4. Parsing Strings into Dates
- 6.5. Difference Between Two Dates
- 6.6. Adding to or Subtracting from a Date
- 6.7. Handling Recurring Events
- 6.8. Computing Dates Involving Time Zones
- 6.9. Interfacing with Legacy Date and Calendar Classes
- 7. Structuring Data with Java
- 7.0. Introduction
- 7.1. Using Arrays for Data Structuring
- 7.2. Resizing an Array
- 7.3. The Collections Framework
- 7.4. Like an Array, but More Dynamic
- 7.5. Using Generic Types in Your Own Class
- 7.6. How Shall I Iterate Thee? Let Me Enumerate the Ways
- 7.7. Eschewing Duplicates with a Set
- 7.8. Structuring Data in a Linked List
- 7.9. Mapping with Hashtable and HashMap
- 7.10. Storing Strings in Properties and Preferences
- 7.11. Sorting a Collection
- 7.12. Avoiding the Urge to Sort
- 7.13. Finding an Object in a Collection
- 7.14. Converting a Collection to an Array
- 7.15. Making Your Data Iterable
- 7.16. Using a Stack of Objects
- 7.17. Multidimensional Structures
- 7.18. Simplifying Data Objects with Lombok or Record
- 7.19. Program: Timing Comparisons
- 8. Object-Oriented Techniques
- 8.0. Introduction
- 8.1. Object Methods: Formatting Objects with toString(), Comparing with Equals
- 8.2. Using Inner Classes
- 8.3. Providing Callbacks via Interfaces
- 8.4. Polymorphism/Abstract Methods
- 8.5. Using Typesafe Enumerations
- 8.6. Avoiding NPEs with Optional
- 8.7. Enforcing the Singleton Pattern
- 8.8. Roll Your Own Exceptions
- 8.9. Using Dependency Injection
- 8.10. Program: Plotter
- 9. Functional Programming Techniques: Functional Interfaces, Streams, and Parallel Collections
- 9.0. Introduction
- 9.1. Using Lambdas/Closures Instead of Inner Classes
- 9.2. Using Lambda Predefined Interfaces Instead of Your Own
- 9.3. Simplifying Processing with Streams
- 9.4. Simplifying Streams with Collectors
- 9.5. Improving Throughput with Parallel Streams and Collections
- 9.6. Using Existing Code as Functional with Method References
- 9.7. Java Mixins: Mixing in Methods
- 10. Input and Output: Reading, Writing, and Directory Tricks
- 10.0. Introduction
- 10.1. About InputStreams/OutputStreams and Readers/Writers
- 10.2. Reading a Text File
- 10.3. Reading from the Standard Input or from the Console/Controlling Terminal
- 10.4. Printing with Formatter and printf
- 10.5. Scanning Input with StreamTokenizer
- 10.6. Scanning Input with the Scanner Class
- 10.7. Scanning Input with Grammatical Structure
- 10.8. Copying a File
- 10.9. Reassigning the Standard Streams
- 10.10. Duplicating a Stream as It Is Written; Reassigning Standard Streams
- 10.11. Reading/Writing a Different Character Set
- 10.12. Those Pesky End-of-Line Characters
- 10.13. Beware Platform-Dependent File Code
- 10.14. Reading/Writing Binary Data
- 10.15. Reading and Writing JAR or ZIP Archives
- 10.16. Finding Files in a Filesystem-Neutral Way with getResource() and getResourceAsStream()
- 10.17. Getting File Information: Files and Path
- 10.18. Creating a New File or Directory
- 10.19. Changing a Files Name or Other Attributes
- 10.20. Deleting a File
- 10.21. Creating a Transient/Temporary File
- 10.22. Listing a Directory
- 10.23. Getting the Directory Roots
- 10.24. Using the FileWatcher Service to Get Notified About File Changes
- 10.25. Program: Save User Data to Disk
- 10.26. Program: FindWalking a File Tree
- 11. Data Science and R
- 11.1. Machine Learning with Java
- 11.2. Using Data In Apache Spark
- 11.3. Using R Interactively
- 11.4. Comparing/Choosing an R Implementation
- 11.5. Using R from Within a Java App: Renjin
- 11.6. Using Java from Within an R Session
- 11.7. Using FastR, the GraalVM Implementation of R
- 11.8. Using R in a Web App
- 12. Network Clients
- 12.0. Introduction
- 12.1. HTTP/REST Web Client
- 12.2. Contacting a Socket Server
- 12.3. Finding and Reporting Network Addresses
- 12.4. Handling Network Errors
- 12.5. Reading and Writing Textual Data
- 12.6. Reading and Writing Binary or Serialized Data
- 12.7. UDP Datagrams
- 12.8. URI, URL, or URN?
- 12.9. Program: TFTP UDP Client
- 12.10. Program: Sockets-Based Chat Client
- 12.11. Program: Simple HTTP Link Checker
- 13. Server-Side Java
- 13.0. Introduction
- 13.1. Opening a Server Socket for Business
- 13.2. Finding Network Interfaces
- 13.3. Returning a Response (String or Binary)
- 13.4. Returning Object Information Across a Network Connection
- 13.5. Handling Multiple Clients
- 13.6. Serving the HTTP Protocol
- 13.7. Securing a Web Server with SSL and JSSE
- 13.8. Creating a REST Service with JAX-RS
- 13.9. Network Logging
- 13.10. Setting Up SLF4J
- 13.11. Network Logging with Log4j
- 13.12. Network Logging with java.util.logging
- 14. Processing JSON Data
- 14.0. Introduction
- 14.1. Generating JSON Directly
- 14.2. Parsing and Writing JSON with Jackson
- 14.3. Parsing and Writing JSON with org.json
- 14.4. Parsing and Writing JSON with JSON-B
- 14.5. Finding JSON Elements with JSON Pointer
- 15. Packages and Packaging
- 15.0. Introduction
- 15.1. Creating a Package
- 15.2. Documenting Classes with Javadoc
- 15.3. Beyond Javadoc: Annotations/Metadata
- 15.4. Preparing a Class as a JavaBean
- 15.5. Archiving with JAR
- 15.6. Running a Program from a JAR
- 15.7. Packaging Web Tier Components into a WAR File
- 15.8. Creating a Smaller Distribution with jlink
- 15.9. Using JPMS to Create a Module
- 16. Threaded Java
- 16.0. Introduction
- 16.1. Running Code in a Different Thread
- 16.2. Displaying a Moving Image with Animation
- 16.3. Stopping a Thread
- 16.4. Rendezvous and Timeouts
- 16.5. Synchronizing Threads with the synchronized Keyword
- 16.6. Simplifying Synchronization with Locks
- 16.7. Simplifying Producer/Consumer with the Queue Interface
- 16.8. Optimizing Parallel Processing with Fork/Join
- 16.9. Scheduling Tasks: Future Times, Background Saving in an Editor
- 17. Reflection, or A Class Named Class
- 17.0. Introduction
- 17.1. Getting a Class Descriptor
- 17.2. Finding and Using Methods and Fields
- 17.3. Accessing Private Methods and Fields via Reflection
- 17.4. Loading and Instantiating a Class Dynamically
- 17.5. Constructing a Class from Scratch with a ClassLoader
- 17.6. Constructing a Class from Scratch with JavaCompiler
- 17.7. Performance Timing
- 17.8. Printing Class Information
- 17.9. Listing Classes in a Package
- 17.10. Using and Defining Annotations
- 17.11. Finding Plug-In-Like Classes via Annotations
- 17.12. Program: CrossRef
- 18. Using Java with Other Languages
- 18.0. Introduction
- 18.1. Running an External Program from Java
- 18.2. Running a Program and Capturing Its Output
- 18.3. Calling Other Languages via javax.script
- 18.4. Mixing Languages with GraalVM
- 18.5. Marrying Java and Perl
- 18.6. Calling Other Languages via Native Code
- 18.7. Calling Java from Native Code
- Afterword
- A. Java Then and Now
- Introduction: Always in Motion the Java Is
- What Was New in Java 8
- What Was New in Java 9
- What Was New in Java 10 (March 2018)
- What Was New in Java 11 (September 2018)
- What Was New in Java 12 (March 2019)
- What Is New in Java 13 (September 2019)
- Looking Ahead
- Index