Introducing Elixir. Getting Started in Functional Programming - Helion
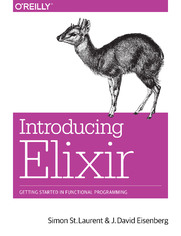
ISBN: 978-14-493-6995-8
stron: 210, Format: ebook
Data wydania: 2014-09-10
Księgarnia: Helion
Cena książki: 59,42 zł (poprzednio: 69,09 zł)
Oszczędzasz: 14% (-9,67 zł)
Elixir is an excellent language if you want to learn about functional programming, and with this hands-on introduction, you’ll discover just how powerful and fun Elixir can be. This language combines the robust functional programming of Erlang with a syntax similar to Ruby, and includes powerful features for metaprogramming.
This book shows you how to write simple Elixir programs by teaching one skill at a time. Once you pick up pattern matching, process-oriented programming, and other concepts, you’ll understand why Elixir makes it easier to build concurrent and resilient programs that scale up and down with ease.
- Get comfortable with IEx, Elixir’s command line interface
- Discover atoms, pattern matching, and guards: the foundations of your program structure
- Delve into the heart of Elixir with recursion, strings, lists, and higher-order functions
- Create processes, send messages among them, and apply pattern matching to incoming messages
- Store and manipulate structured data with Erlang Term Storage and the Mnesia database
- Build resilient applications with Erlang’s Open Telecom Platform
- Define macros with Elixir’s metaprogramming tools
Osoby które kupowały "Introducing Elixir. Getting Started in Functional Programming", wybierały także:
- Windows Media Center. Domowe centrum rozrywki 66,67 zł, (8,00 zł -88%)
- 66,19 zł, (13,90 zł -79%)
- Superinteligencja. Scenariusze, strategie, zagro 66,19 zł, (13,90 zł -79%)
- Przywództwo w świecie VUCA. Jak być skutecznym liderem w niepewnym środowisku 58,64 zł, (12,90 zł -78%)
- Twoja firma w social mediach. Podr 57,92 zł, (13,90 zł -76%)
Spis treści
Introducing Elixir. Getting Started in Functional Programming eBook -- spis treści
- Introducing Elixir
- Preface
- Who This Book Is For
- Who This Book Is Not For
- What This Book Will Do For You
- How This Book Works
- Other Resources
- Elixir Will Change You
- Conventions Used in This Book
- Using Code Examples
- Help This Book Grow
- Please Use It For Good
- Safari Books Online
- How to Contact Us
- Acknowledgments
- 1. Getting Comfortable
- Installation
- Installing Erlang
- Installing Elixir
- Firing It Up
- First Steps
- Moving Through Text and History
- Moving Through Files
- Doing Something
- Calling Functions
- Numbers in Elixir
- Working with Variables in the Shell
- Installation
- 2. Functions and Modules
- Fun with fn
- And the &
- Defining Modules
- From Module to Free-Floating Function
- Splitting Code Across Modules
- Combining Functions with the Pipe Operator
- Importing Functions
- Default Values for Arguments
- Documenting Code
- Documenting Functions
- Documenting Modules
- 3. Atoms, Tuples, and Pattern Matching
- Atoms
- Pattern Matching with Atoms
- Atomic Booleans
- Guards
- Underscoring That You Dont Care
- Adding Structure: Tuples
- Pattern Matching with Tuples
- Processing Tuples
- 4. Logic and Recursion
- Logic Inside of Functions
- Evaluating Cases
- Adjusting to Conditions
- If, or else
- Variable Assignment in case and if Constructs
- The Gentlest Side Effect: IO.puts
- Simple Recursion
- Counting Down
- Counting Up
- Recursing with Return Values
- Logic Inside of Functions
- 5. Communicating with Humans
- Strings
- Multiline Strings
- Unicode
- Character Lists
- String Sigils
- Asking Users for Information
- Gathering Characters
- Reading Lines of Text
- 6. Lists
- List Basics
- Splitting Lists into Heads and Tails
- Processing List Content
- Creating Lists with Heads and Tails
- Mixing Lists and Tuples
- Building a List of Lists
- 7. Name-Value Pairs
- Keyword Lists
- Lists of Tuples with Multiple Keys
- Hash Dictionaries
- From Lists to Maps
- Creating Maps
- Updating Maps
- Reading Maps
- From Maps to Structs
- Setting Up Structs
- Creating and Reading Structs
- Pattern Matching Against Structs
- Using Structs in Functions
- Adding Behavior to Structs
- Adding to Existing Protocols
- 8. Higher-Order Functions and List Comprehensions
- Simple Higher-Order Functions
- Creating New Lists with Higher-Order Functions
- Reporting on a List
- Running List Values Through a Function
- Filtering List Values
- Beyond List Comprehensions
- Testing Lists
- Splitting Lists
- Folding Lists
- 9. Playing with Processes
- The Shell Is a Process
- Spawning Processes from Modules
- Lightweight Processes
- Registering a Process
- When Processes Break
- Processes Talking Amongst Themselves
- Watching Your Processes
- Watching Messages Among Processes
- Breaking Things and Linking Processes
- 10. Exceptions, Errors, and Debugging
- Flavors of Errors
- Rescuing Code from Runtime Errors as They Happen
- Logging Progress and Failure
- Tracing Messages
- Watching Function Calls
- Writing Unit Tests
- 11. Storing Structured Data
- Records: Structured Data Before structs
- Setting Up Records
- Creating and Reading Records
- Using Records in Functions
- Storing Data in Erlang Term Storage
- Creating and Populating a Table
- Simple Queries
- Overwriting Values
- ETS Tables and Processes
- Next Steps
- Storing Records in Mnesia
- Starting up Mnesia
- Creating Tables
- Reading Data
- Records: Structured Data Before structs
- 12. Getting Started with OTP
- Creating Services with gen_server
- A Simple Supervisor
- Packaging an Application with Mix
- 13. Using Macros to Extend Elixir
- Functions versus Macros
- A Simple Macro
- Creating New Logic
- Creating Functions Programatically
- When (Not) to Use Macros
- Sharing the Gospel of Elixir
- A. An Elixir Parts Catalog
- Shell Commands
- Reserved Words
- Operators
- Guard Components
- Common Functions
- Datatypes for Documentation and Analysis
- B. Generating Documentation with ExDoc
- Using ExDoc with mix
- Index
- Colophon
- Copyright