Head First Ruby - Helion
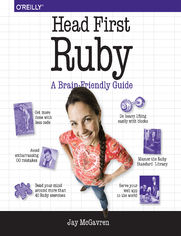
ISBN: 978-14-493-7267-5
stron: 572, Format: ebook
Data wydania: 2015-08-21
Księgarnia: Helion
Cena książki: 143,65 zł (poprzednio: 167,03 zł)
Oszczędzasz: 14% (-23,38 zł)
What will you learn from this book?
What’s all the buzz about this Ruby language? Is it right for you? Well, ask yourself: are you tired of all those extra declarations, keywords, and compilation steps in your other language? Do you want to be a more productive programmer? Then you’ll love Ruby. With this unique hands-on learning experience, you’ll discover how Ruby takes care of all the details for you, so you can simply have fun and get more done with less code.
Why does this book look so different?
Based on the latest research in cognitive science and learning theory, Head First Ruby uses a visually rich format to engage your mind, rather than a text-heavy approach that puts you to sleep. Why waste your time struggling with new concepts? This multi-sensory learning experience is designed for the way your brain really works.
Osoby które kupowały "Head First Ruby", wybierały także:
- Ruby on Rails. Ćwiczenia 18,75 zł, (3,00 zł -84%)
- Programowanie w Ruby. Poziom drugi. Kurs video. Zaawansowane techniki 69,00 zł, (27,60 zł -60%)
- Ruby on Rails (RoR). Kurs video. Programowanie aplikacji internetowych 99,00 zł, (44,55 zł -55%)
- Ruby on Rails. Kurs video. Wdrażanie (deployment) na własne serwery i chmurę 59,00 zł, (26,55 zł -55%)
- Sass i Compass. Praktyczny przewodnik dla projektantów 49,00 zł, (26,95 zł -45%)
Spis treści
Head First Ruby. A Brain-Friendly Guide eBook -- spis treści
- Head First Ruby
- Dedication
- Author of Head First Ruby
- How to Use This Book: Intro
- Who is this book for?
- Who should probably back away from this book?
- We know what youre thinking
- We know what your brain is thinking
- Metacognition: thinking about thinking
- Heres what WE did
- Heres what YOU can do to bend your brain into submission
- Read me
- Acknowledgments
- Safari Books Online
- Who is this book for?
- 1. More with Less: Code the Way You Want
- The Ruby philosophy
- Get Ruby
- Use Ruby
- Use Rubyinteractively
- Using the irb shell
- Your first Ruby expressions
- Math operations and comparisons
- Strings
- Variables
- Everything is an object!
- Calling a method on an object
- Lets build a game
- Input, storage, and output
- Running scripts
- Comments
- puts and print
- Method arguments
- gets
- Parentheses are optional on method calls
- String interpolation
- Whats in that string?
- Inspecting objects with the inspect and p methods
- Escape sequences in strings
- Commonly used escape sequences
- Calling chomp on the string object
- What methods are available on an object?
- Generating a random number
- Converting to strings
- Ruby makes working with strings easy
- Converting strings to numbers
- Common conversions
- Conditionals
- The opposite of if is unless
- Loops
- Lets try running our game!
- Your Ruby Toolbox
- Up Next...
- 2. Methods and Classes: Getting Organized
- Defining methods
- Calling methods youve defined
- Method names
- Parameters
- Optional parameters
- Return values
- Implicit return values
- Returning from a method early
- Some messy methods
- Too many arguments
- Too many if statements
- Designing a class
- Whats the difference between a class and an object?
- Your first class
- Creating new instances (objects)
- Breaking up our giant methods into classes
- The object-oriented approach
- Creating instances of our new animal classes
- Updating our class diagram with instance methods
- Our objects dont know their names or ages!
- Too many arguments (again)
- Local variables live until the method ends
- Instance variables live as long as the instance does
- Encapsulation
- Attribute accessor methods
- Using accessor methods
- Attribute writers and readers
- Symbols
- Attribute writers and readers in action
- Ensuring data is valid with accessors
- Errorsthe emergency stop button
- Using raise in our attribute writer methods
- Our complete Dog class
- Your Ruby Toolbox
- Up Next...
- 3. Inheritance: Relying on Your Parents
- Copy, paste... Such a waste...
- Mikes code for the virtual test-drive classes
- Inheritance to the rescue!
- Defining a superclass (requires nothing special)
- Defining a subclass (is really easy)
- Adding methods to subclasses
- Subclasses keep inherited methods alongside new ones
- Instance variables belong to the object, not the class!
- Overriding methods
- Bringing our animal classes up to date with inheritance
- Designing the animal class hierarchy
- Code for the Animal class and its subclasses
- Overriding a method in the Animal subclasses
- We need to get at the overridden method!
- The super keyword
- A super-powered subclass
- Difficulties displaying Dogs
- The Object class
- Why everything inherits from the Object class
- Overriding the inherited method
- Your Ruby Toolbox
- Up Next...
- 4. Initializing Instances: Off to a Great Start
- Payroll at Chargemore
- An Employee class
- Creating new Employee instances
- A division problem
- Division with Rubys Fixnum class
- Division with Rubys Float class
- Fixing the salary rounding error in Employee
- Formatting numbers for printing
- Format sequences
- Format sequence types
- Format sequence width
- Format sequence width with floating-point numbers
- Using format to fix our pay stubs
- When we forget to set an objects attributes...
- nil stands for nothing
- / is a method
- The initialize method
- Employee safety with initialize
- Arguments to initialize
- Using optional parameters with initialize
- initialize does an end-run around our validation
- initialize and validation
- Call other methods on the same instance with self
- When self is optional
- Implementing hourly employees through inheritance
- Restoring initialize methods
- Inheritance and initialize
- super and initialize
- Same class, same attribute values
- An inefficient factory method
- Class methods
- Our complete source code
- Your Ruby Toolbox
- Up Next...
- 5. Arrays and Blocks: Better Than Loops
- Arrays
- Accessing arrays
- Arrays are objects, too!
- Looping over the items in an array
- The repeating loop
- Eliminating repetition...the WRONG way...
- Chunks of code?
- Blocks
- Blocks are mind-bending stuff. But stick with it!
- Defining a method that takes blocks
- Your first block
- Flow of control between a method and block
- Calling the same method with different blocks
- Calling a block multiple times
- Block parameters
- Using the yield keyword
- Block formats
- The each method
- The each method, step-by-step
- DRYing up our code with each and blocks
- Blocks and variable scope
- Using each with the refund method
- Using each with our last method
- Our complete invoicing methods
- Weve gotten rid of the repetitive loop code!
- Utilities and appliances, blocks and methods
- Your Ruby Toolbox
- Up Next...
- 6. Block Return Values: How Should I Handle This?
- A big collection of words to search through
- Opening the file
- Safely closing the file
- Safely closing the file, with a block
- Dont forget about variable scope!
- Finding array elements we want, with a block
- The verbose way to find array elements, using each
- Introducing a faster method...
- Blocks have a return value
- How the method uses a block return value
- Putting it all together
- A closer look at the block return values
- Eliminating elements we dont want, with a block
- The return values for reject
- Breaking a string into an array of words
- Finding the index of an array element
- Making one array thats based on another, the hard way
- Making one array based on another, using map
- Some additional logic in the map block body
- The finished product
- Your Ruby Toolbox
- Up Next...
- 7. Hashes: Labeling Data
- Counting votes
- An array of arrays...is not ideal
- Hashes
- Hashes are objects
- Hashes return nil by default
- nil (and only nil) is falsy
- Returning something other than nil by default
- Normalizing hash keys
- Hashes and each
- A mess of method arguments
- Using hashes as method parameters
- Hash parameters in our Candidate class
- Leave off the braces!
- Leave out the arrows!
- Making the entire hash optional
- Typos in hash arguments are dangerous
- Keyword arguments
- Using keyword arguments with our Candidate class
- Required keyword arguments
- Your Ruby Toolbox
- Up Next...
- 8. References: Crossed Signals
- Some confusing bugs
- The heap
- References
- When references go wrong
- Aliasing
- Fixing the astronomers program
- Quickly identifying objects with inspect
- Problems with a hash default object
- Were actually modifying the hash default object!
- A more detailed look at hash default objects
- Back to the hash of planets and moons
- Our wish list for hash defaults
- Hash default blocks
- Hash default blocks: Assigning to the hash
- Hash default blocks: Block return value
- Hash default blocks: A shortcut
- The astronomers hash: Our final code
- Using hash default objects safely
- Hash default object rule #1: Dont modify the default object
- Hash default object rule #2: Assign values to the hash
- The rule of thumb for hash defaults
- Your Ruby Toolbox
- Up Next...
- 9. Mixins: Mix It Up
- The media-sharing app
- The media-sharing app...using inheritance
- One of these classes is not (quite) like the others
- Option one: Make Photo a subclass of Clip
- Option two: Copy the methods you want into the Photo class
- Not an option: Multiple inheritance
- Using modules as mixins
- Mixins, behind the scenes
- Creating a mixin for comments
- Using our comments mixin
- A closer look at the revised comments method
- Why you shouldnt add initialize to a mixin
- Mixins and method overriding
- Avoid using initialize methods in modules
- Using the Boolean or operator for assignment
- The conditional assignment operator
- Our complete code
- Your Ruby Toolbox
- Up Next...
- 10. Comparable and Enumerable: Ready-Made Mixes
- Mixins built into Ruby
- A preview of the Comparable mixin
- Choice (of) beef
- Implementing a greater-than method on the Steak class
- Constants
- We have a lot more methods to define...
- The Comparable mixin
- The spaceship operator
- Implementing the spaceship operator on Steak
- Mixing Comparable into Steak
- How the Comparable methods work
- Our next mixin
- The Enumerable module
- A class to mix Enumerable into
- Mixing Enumerable into our class
- Inside the Enumerable module
- Your Ruby Toolbox
- Up Next...
- 11. Documentation: Read the Manual
- Learning how to learn more
- Rubys core classes and modules
- Documentation
- HTML documentation
- Listing available classes and modules
- Looking up instance methods
- Instance methods denoted with # in the docs
- Instance method documentation
- Arguments in call signatures
- Blocks in call signatures
- Read the docs for the superclass and mixins, too!
- Read the docs for the superclass and mixins, too! (continued)
- Pool Puzzle
- Pool Puzzle Solution
- Looking up class methods
- Class method documentation
- Docs for a class that doesnt exist?!
- The Ruby standard library
- Looking up classes and modules in the standard library
- Where Ruby docs come from: rdoc
- What rdoc can deduce about your classes
- Adding your own documentation, with comments
- The initialize instance method appears as the new class method
- Your Ruby Toolbox
- Up Next...
- 12. Exceptions: Handling the Unexpected
- Dont use method return values for error messages
- Using raise to report errors
- Using raise by itself creates new problems
- Exceptions: When somethings gone wrong
- Rescue clauses: A chance to fix the problem
- Rubys search for a rescue clause
- Using a rescue clause with our SmallOven class
- We need a description of the problem from its source
- Exception messages
- Our code so far...
- Different rescue logic for different exceptions
- Exception classes
- Specifying exception class for a rescue clause
- Multiple rescue clauses in one begin/end block
- Updating our oven code with custom exception classes
- Trying again after an error with retry
- Updating our oven code with retry
- Things you want to do no matter what
- The ensure clause
- Ensuring the oven gets turned off
- Your Ruby Toolbox
- Up Next...
- 13. Unit Testing: Code Quality Assurance
- Automated tests find your bugs before someone else does
- A program we should have had automated tests for
- Types of automated tests
- MiniTest: Rubys standard unit-testing library
- Running a test
- Testing a class
- A closer look at the test code
- Red, green, refactor
- Tests for ListWithCommas
- Getting the test to pass
- Another bug to fix
- Test failure messages
- A better way to assert that two values are equal
- Some other assertion methods
- Removing duplicated code from your tests
- The setup method
- The teardown method
- Updating our code to use the setup method
- Your Ruby Toolbox
- Up Next...
- 14. Web Apps: Serving HTML
- Writing web apps in Ruby
- Our task list
- Project directory structure
- Browsers, requests, servers, and responses
- Sinatra takes requests
- Downloading and installing libraries with RubyGems
- Installing the Sinatra gem
- A simple Sinatra app
- Your computer is talking to itself
- Request type
- Resource path
- Sinatra routes
- Multiple routes in the same Sinatra app
- A route for the list of movies
- Making a movie list in HTML
- Accessing the HTML from Sinatra
- A class to hold our movie data
- Setting up a Movie object in the Sinatra app
- ERB embedding tags
- The ERB output embedding tag
- Embedding a movie title in our HTML
- Pool Puzzle
- Pool Puzzle Solution
- The regular embedding tag
- Looping over several movie titles in our HTML
- Letting users add data with HTML forms
- Getting an HTML form for adding a movie
- HTML tables
- Cleaning up our form with an HTML table
- Theres still more to do
- Your Ruby Toolbox
- Up Next...
- 15. Saving and Loading Data: Keep It Around
- Saving and retrieving form data
- Our browser can GET the form...
- ... But it needs to POST the response
- Setting the HTML form to send a POST request
- Setting up a Sinatra route for a POST request
- Converting objects to and from strings with YAML
- Saving objects to a file with YAML::Store
- Saving movies to a file with YAML::Store
- A system for finding Movies in the YAML::Store
- Numeric IDs for Movies
- Finding the next available movie ID
- A class to manage our YAML::Store
- Using our MovieStore class in the Sinatra app
- Testing the MovieStore
- Loading all movies from the MovieStore
- Loading all movies from the MovieStore (continued)
- Loading all movies in the Sinatra app
- Building HTML links to individual movies
- Building HTML links to individual movies (continued)
- Named parameters in Sinatra routes
- Using a named parameter to get a movies ID
- Defining routes in order of priority
- Finding a Movie in the YAML::Store
- An ERB template for an individual movie
- Finishing the Sinatra route for individual movies
- Lets try it all out!
- Our complete app code
- Your Ruby Toolbox
- Up Next...
- A. Leftovers: The top ten topics (we didnt cover)
- #1 Other cool libraries
- Ruby on Rails
- dRuby
- CSV
- #2 Inline if and unless
- #3 Private methods
- #4 Command-line arguments
- #5 Regular expressions
- #6 Singleton methods
- #7 Call any method, even undefined ones
- #7 Call any method, even undefined ones (continued)
- #8 Automating tasks with Rake
- #9 Bundler
- #10 Other books
- #1 Other cool libraries
- B. This isnt goodbye
- C. Oreilly: Ruby
- What will you learn from this book?
- Whats so special about this book?
- Index
- About the Author
- Copyright