Head First Kotlin. A Brain-Friendly Guide - Helion
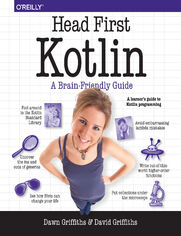
ISBN: 9781491996645
stron: 482, Format: ebook
Data wydania: 2019-02-13
Księgarnia: Helion
Cena książki: 203,15 zł (poprzednio: 236,22 zł)
Oszczędzasz: 14% (-33,07 zł)
What will you learn from this book?
Head First Kotlin is a complete introduction to coding in Kotlin. This hands-on book helps you learn the Kotlin language with a unique method that goes beyond syntax and how-to manuals and teaches you how to think like a great Kotlin developer. You’ll learn everything from language fundamentals to collections, generics, lambdas, and higher-order functions. Along the way, you’ll get to play with both object-oriented and functional programming. If you want to really understand Kotlin, this is the book for you.
Why does this book look so different?
Based on the latest research in cognitive science and learning theory, Head First Kotlin uses a visually rich format to engage your mind rather than a text-heavy approach that puts you to sleep. Why waste your time struggling with new concepts? This multisensory learning experience is designed for the way your brain really works.
Osoby które kupowały "Head First Kotlin. A Brain-Friendly Guide", wybierały także:
- Hello! Flex 4 39,21 zł, (14,90 zł -62%)
- .NET MAUI Projects 98,15 zł, (39,26 zł -60%)
- Swift Design Patterns 98,15 zł, (39,26 zł -60%)
- iOS 15 Application Development for Beginners 78,52 zł, (39,26 zł -50%)
- iOS Developer Solutions Guide 78,52 zł, (39,26 zł -50%)
Spis treści
Head First Kotlin. A Brain-Friendly Guide eBook -- spis treści
- how to use this book: Intro
- Who is this book for?
- Who should probably back away from this book?
- We know what youre thinking
- We know what your brain is thinking
- Metacognition: thinking about thinking
- Heres what WE did:
- Heres what YOU can do to bend your brain into submission
- Read me
- The technical review team
- Acknowledgments
- OReilly
- Who is this book for?
- Table of Contents (the real thing)
- 1. getting started: A Quick Dip
- Welcome to Kotlinville
- Its crisp, concise and readable
- You can use object-oriented AND functional programming
- The compiler keeps you safe
- You can use Kotlin nearly everywhere
- Java Virtual Machines (JVMs)
- Android
- Client-side and server-side JavaScript
- Native apps
- What well do in this chapter
- Install IntelliJ IDEA (Community Edition)
- Lets build a basic application
- 1. Create a new project
- 2. Specify the type of project
- 3. Configure the project
- Youve just created your first Kotlin project
- Add a new Kotlin file to the project
- Anatomy of the main function
- Add the main function to App.kt
- Test drive
- What the Run command does
- What can you say in the main function?
- Loop and loop and loop...
- Simple boolean tests
- A loopy example
- Test drive
- Conditional branching
- Using if to return a value
- Update the main function
- Test drive
- Code Magnets
- Using the Kotlin interactive shell
- You can add multi-line code snippets to the REPL
- Its exercise time
- Code Magnets Solution
- Your Kotlin Toolbox
- Welcome to Kotlinville
- 2. basic types and variables: Being a Variable
- Your code needs variables
- A variable is like a cup
- What happens when you declare a variable
- The value is transformed into an object...
- ...and the compiler infers the variables type from that of the object
- The variable holds a reference to the object
- val vs. var revisited
- Kotlins basic types
- Integers
- Floating points
- Booleans
- Characters and Strings
- How to explicitly declare a variables type
- Declaring the type AND assigning a value
- Use the right value for the variables type
- Assigning a value to another variable
- We need to convert the value
- An object has state and behavior
- How to convert a numeric value to another type
- What happens when you convert a value
- Watch out for overspill
- Store multiple values in an array
- How to create an array
- Create the Phrase-O-Matic application
- Add the code to PhraseOMatic.kt
- The compiler infers the arrays type from its values
- How to explicitly define the arrays type
- var means the variable can point to a different array
- val means the variable points to the same array forever...
- ...but you can still update the variables in the array
- Code Magnets
- Code Magnets Solution
- Your Kotlin Toolbox
- Your code needs variables
- 3. functions: Getting Out of Main
- Lets build a game: Rock, Paper, Scissors
- How the game will work
- A high-level design of the game
- Heres what were going to do
- Get started: create the project
- Get the game to choose an option
- Create the Rock, Paper, Scissors array
- How you create functions
- You can send things to a function
- You can send more than one thing to a function
- Calling a two-parameter function, and sending it two arguments
- You can pass variables to a function so long as the variable type matches the parameter type
- You can get things back from a function
- Functions with no return value
- Functions with single-expression bodies
- Create the getGameChoice function
- Code Magnets
- Code Magnets Solution
- Add the getGameChoice function to Game.kt
- Behind the scenes: what happens
- The story continues
- The getUserChoice function
- Ask for the users choice
- How for loops work
- Looping through a range of numbers
- Use downTo to reverse the range
- Use step to skip numbers in the range
- Looping through the items in an array
- Ask the user for their choice
- Use the readLine function to read the users input
- We need to validate the users input
- And and Or operators (&& and ||)
- Not equals (!= and !)
- Use parentheses to make your code clear
- Add the getUserChoice function to Game.kt
- Test drive
- We need to print the results
- Add the printResult function to Game.kt
- Test drive
- Your Kotlin Toolbox
- Lets build a game: Rock, Paper, Scissors
- 4. classes and objects: A Bit of Class
- Object types are defined using classes
- You can define your own classes
- How to design your own classes
- Lets define a Dog class
- How to create a Dog object
- How to access properties and functions
- What if the Dog is in a Dog array?
- Create a Songs application
- Test drive
- The miracle of object creation
- How objects are created
- What the Dog constructor looks like
- Behind the scenes: calling the Dog constructor
- Code Magnets
- Code Magnets Solution
- Going deeper into properties
- Behind the scenes of the Dog constructor
- Flexible property initialization
- How to use initializer blocks
- You MUST initialize your properties
- How do you validate property values?
- The solution: custom getters and setters
- How to write a custom getter
- How to write a custom setter
- The full code for the Dogs project
- Test drive
- Your Kotlin Toolbox
- Object types are defined using classes
- 5. subclasses and superclasses: Using Your Inheritance
- Inheritance helps you avoid duplicate code
- An inheritance example
- What were going to do
- Design an animal class inheritance structure
- Use inheritance to avoid duplicate code in subclasses
- What should the subclasses override?
- The animals have different property values...
- ...and different function implementations
- We can group some of the animals
- Add Canine and Feline classes
- Use IS-A to test your class hierarchy
- Use HAS-A to test for other relationships
- The IS-A test works anywhere in the inheritance tree
- Well create some Kotlin animals
- Declare the superclass and its properties and functions as open
- How a subclass inherits from a superclass
- How (and when) to override properties
- Overriding properties lets you do more than assign default values
- How to override functions
- The rules for overriding functions
- An overridden function or property stays open...
- ...until its declared final
- Add the Hippo class to the Animals project
- Code Magnets
- Code Magnets Solution
- Add the Canine and Wolf classes
- Which function is called?
- Inheritance guarantees that all subclasses have the functions and properties defined in the superclass
- Any place where you can use a superclass, you can use one of its subclasses instead
- When you call a function on the variable, its the objects version that responds
- You can use a supertype for a functions parameters and return type
- The updated Animals code
- Test drive
- Your Kotlin Toolbox
- Inheritance helps you avoid duplicate code
- 6. abstract classes and interfaces: Serious Polymorphism
- The Animal class hierarchy revisited
- Some classes shouldnt be instantiated
- Declare a class as abstract to stop it from being instantiated
- Abstract or concrete?
- An abstract class can have abstract properties and functions
- We can mark three properties as abstract
- The Animal class has two abstract functions
- How to implement an abstract class
- You MUST implement all abstract properties and functions
- Lets update the Animals project
- Test drive
- Independent classes can have common behavior
- An interface lets you define common behavior OUTSIDE a superclass hierarchy
- Lets define the Roamable interface
- Interface functions can be abstract or concrete
- How to define interface properties
- Declare that a class implements an interface...
- ...then override its properties and functions
- How to implement multiple interfaces
- How do you know whether to make a class, a subclass, an abstract class, or an interface?
- Update the Animals project
- Test drive
- Interfaces let you use polymorphism
- Access uncommon behavior by checking an objects type
- Where to use the is operator
- As the condition for an if
- In conditions using && and ||
- In a while loop
- Use when to compare a variable against a bunch of options
- The is operator usually performs a smart cast
- Use as to perform an explicit cast
- Update the Animals project
- Test drive
- Your Kotlin Toolbox
- 7. data classes: Dealing with Data
- == calls a function named equals
- equals is inherited from a superclass named Any
- The importance of being Any
- The common behavior defined by Any
- We might want equals to check whether two objects are equivalent
- A data class lets you create data objects
- How to create objects from a data class
- Data classes override their inherited behavior
- The equals function compares property values
- Equal objects return the same hashCode value
- toString returns the value of each property
- Copy data objects using the copy function
- Data classes define componentN functions...
- ...that let you destructure data objects
- Create the Recipes project
- Test drive
- Generated functions only use properties defined in the constructor
- Initializing many properties can lead to cumbersome code
- Default parameter values to the rescue!
- How to use a constructors default values
- 1. Passing values in order of declaration
- 2. Using named arguments
- Functions can use default values too
- Overloading a function
- Dos and donts for function overloading:
- Lets update the Recipes project
- The code continued...
- Test drive
- Your Kotlin Toolbox
- 8. nulls and exceptions: Safe and Sound
- How do you remove object references from variables?
- Remove an object reference using null
- Why have nullable types?
- You can use a nullable type everywhere you can use a non-nullable type
- How to create an array of nullable types
- How to access a nullable types functions and properties
- Keep things safe with safe calls
- You can chain safe calls together
- What happens when a safe call chain gets evaluated
- The story continues
- You can use safe calls to assign values...
- ...and assign values to safe calls
- Use let to run code if values are not null
- Using let with array items
- Using let to streamline expressions
- Instead of using an if expression...
- ...you can use the safer Elvis operator
- The !! operator deliberately throws a NullPointerException
- Create the Null Values project
- The code continued...
- Test drive
- An exception is thrown in exceptional circumstances
- You can catch exceptions that are thrown
- Catch exceptions using a try/catch
- Use finally for the things you want to do no matter what
- An exception is an object of type Exception
- You can explicitly throw exceptions
- try and throw are both expressions
- How to use try as an expression
- How to use throw as an expression
- Code Magnets
- Code Magnets Solution
- Your Kotlin Toolbox
- 9. collections: Get Organized
- Arrays can be useful...
- ...but there are things an array cant handle
- You cant change an arrays size
- Arrays are mutable, so they can be updated
- When in doubt, go to the Library
- List, Set and Map
- List - when sequence matters
- Set - when uniqueness matters
- Map - when finding something by key matters
- Fantastic Lists...
- ...and how to use them
- Create a MutableList...
- ..and add values to it
- You can remove a value...
- ...and replace one value with another
- You can change the order and make bulk changes...
- ...or take a copy of the entire MutableList
- Create the Collections project
- Test drive
- Code Magnets
- Code Magnets Solution
- Lists allow duplicate values
- How to create a Set
- How to use a Sets values
- How a Set checks for duplicates
- Hash codes and equality
- Equality using the === operator
- Equality using the == operator
- Rules for overriding hashCode and equals
- How to use a MutableSet
- You can copy a MutableSet
- Update the Collections project
- Test drive
- Time for a Map
- How to create a Map
- How to use a Map
- Create a MutableMap
- Put entries in a MutableMap
- You can remove entries from a MutableMap
- You can copy Maps and MutableMaps
- The full code for the Collections project
- Test drive
- Your Kotlin Toolbox
- 10. generics: Know Your Ins from Your Outs
- Collections use generics
- How a MutableList is defined
- Understanding collection documentation (Or, whats the meaning of E?)
- Using type parameters with MutableList
- Things you can do with a generic class or interface
- Heres what were going to do
- Create the Pet class hierarchy
- Define the Contest class
- Declare that Contest uses a generic type
- You can restrict T to a specific supertype
- Add the scores property
- Create the addScore function
- Create the getWinners function
- Create some Contest objects
- The compiler can infer the generic type
- Create the Generics project
- Test drive
- The Retailer hierarchy
- Define the Retailer interface
- We can create CatRetailer, DogRetailer and FishRetailer objects...
- ...but what about polymorphism?
- Use out to make a generic type covariant
- Collections are defined using covariant types
- Update the Generics project
- Test drive
- We need a Vet class
- Assign a Vet to a Contest
- Create Vet objects
- Pass a Vet to the Contest constructor
- Use in to make a generic type contravariant
- Should a Vet<Cat> ALWAYS accept a Vet<Pet>?
- A generic type can be locally contravariant
- Update the Generics project
- Test drive
- Your Kotlin Toolbox
- 11. lambdas and higher-order functions: Treating Code Like Data
- Introducing lambdas
- What were going to do
- What lambda code looks like
- You can assign a lambda to a variable
- Execute a lambdas code by invoking it
- What happens when you invoke a lambda
- Lambda expressions have a type
- The compiler can infer lambda parameter types
- You can replace a single parameter with it
- Use the right lambda for the variables type
- Use Unit to say a lambda has no return value
- Create the Lambdas project
- Test drive
- You can pass a lambda to a function
- Add a lambda parameter to a function by specifying its name and type
- Invoke the lambda in the function body
- Call the function by passing it parameter values
- What happens when you call the function
- You can move the lambda OUTSIDE the ()s...
- ...or remove the ()s entirely
- Update the Lambdas project
- Test drive
- A function can return a lambda
- Write a function that receives AND returns lambdas
- Define the parameters and return type
- Define the function body
- How to use the combine function
- What happens when the code runs
- You can make lambda code more readable
- Use typealias to provide a different name for an existing type
- Update the Lambdas project
- Test drive
- Code Magnets
- Code Magnets Solution
- Your Kotlin Toolbox
- Introducing lambdas
- 12. built-in higher-order functions: Power Up Your Code
- Kotlin has a bunch of built-in higher-order functions
- The min and max functions work with basic types
- The minBy and maxBy functions work with ALL types
- A closer look at minBy and maxBys lambda parameter
- What about minBy and maxBys return type?
- The sumBy and sumByDouble functions
- sumBy and sumByDoubles lambda parameter
- Create the Groceries project
- Test drive
- Meet the filter function
- Theres a whole FAMILY of filter functions
- Use map to apply a transform to your collection
- You can chain function calls together
- What happens when the code runs
- The story continues...
- forEach works like a for loop
- forEach has no return value
- Lambdas have access to variables
- Update the Groceries project
- Test drive
- Use groupBy to split your collection into groups
- You can use groupBy in function call chains
- How to use the fold function
- Behind the scenes: the fold function
- Some more examples of fold
- Find the product of a List<Int>
- Concatenate together the name of each item in a List<Grocery>
- Subtract the total price of items from an initial value
- Update the Groceries project
- Test drive
- Your Kotlin Toolbox
- Leaving town...
- Its been great having you here in Kotlinville
- A. coroutines: Running Code in Parallel
- Lets build a drum machine
- 1. Create a new GRADLE project
- 2. Enter an artifact ID
- 3. Specify configuration details
- 4. Specify the project name
- Add the audio files
- Add the code to the project
- Test drive
- Use coroutines to make beats play in parallel
- 1. Add a coroutines dependency
- 2. Launch a coroutine
- Test drive
- A coroutine is like a lightweight thread
- Use runBlocking to run coroutines in the same scope
- Test drive
- Thread.sleep pauses the current THREAD
- The delay function pauses the current COROUTINE
- The full project code
- Test drive
- Lets build a drum machine
- B. testing: Hold Your Code to Account
- Kotlin can use existing testing libraries
- Add the JUnit library
- Create a JUnit test class
- Using KotlinTest
- Use rows to test against sets of data
- Kotlin can use existing testing libraries
- C. leftovers: The Top Ten Things: (We Didnt Cover)
- 1. Packages and imports
- How to add a package
- Package declarations
- The fully qualified name
- Type the fully qualified name...
- ...or import it
- 2. Visibility modifiers
- Visibility modifiers and top level code
- Visibility modifiers and classes/interfaces
- 3. Enum classes
- Enum constructors
- enum properties and functions
- 4. Sealed classes
- Sealed classes to the rescue!
- How to use sealed classes
- 5. Nested and inner classes
- An inner class can access the outer class members
- 6. Object declarations and expressions
- Class objects...
- ...and companion objects
- Object expressions
- 7. Extensions
- 8. Return, break and continue
- Using labels with break and continue
- Using labels with return
- 9. More fun with functions
- vararg
- infix
- inline
- 10. Interoperability
- Interoperability with Java
- Using Kotlin with JavaScript
- Writing native code with Kotlin
- 1. Packages and imports
- Index