Functional JavaScript. Introducing Functional Programming with Underscore.js - Helion
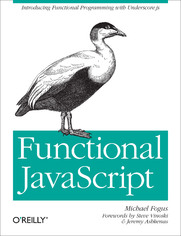
ISBN: 978-14-493-6078-8
stron: 260, Format: ebook
Data wydania: 2013-06-03
Księgarnia: Helion
Cena książki: 72,24 zł (poprzednio: 84,99 zł)
Oszczędzasz: 15% (-12,75 zł)
How can you overcome JavaScript language oddities and unsafe features? With this book, you’ll learn how to create code that’s beautiful, safe, and simple to understand and test by using JavaScript’s functional programming support. Author Michael Fogus shows you how to apply functional-style concepts with Underscore.js, a JavaScript library that facilitates functional programming techniques. Sample code is available on GitHub at https://github.com/funjs/book-source.
Fogus helps you think in a functional way to help you minimize complexity in the programs you build. If you’re a JavaScript programmer hoping to learn functional programming techniques, or a functional programmer looking to learn JavaScript, this book is the ideal introduction.
- Use applicative programming techniques with first-class functions
- Understand how and why you might leverage variable scoping and closures
- Delve into higher-order functions—and learn how they take other functions as arguments for maximum advantage
- Explore ways to compose new functions from existing functions
- Get around JavaScript’s limitations for using recursive functions
- Reduce, hide, or eliminate the footprint of state change in your programs
- Practice flow-based programming with chains and functional pipelines
- Discover how to code without using classes
Osoby które kupowały "Functional JavaScript. Introducing Functional Programming with Underscore.js", wybierały także:
- Windows Media Center. Domowe centrum rozrywki 66,67 zł, (8,00 zł -88%)
- Ruby on Rails. Ćwiczenia 18,75 zł, (3,00 zł -84%)
- DevOps w praktyce. Kurs video. Jenkins, Ansible, Terraform i Docker 190,00 zł, (39,90 zł -79%)
- Przywództwo w świecie VUCA. Jak być skutecznym liderem w niepewnym środowisku 58,64 zł, (12,90 zł -78%)
- Scrum. O zwinnym zarządzaniu projektami. Wydanie II rozszerzone 58,64 zł, (12,90 zł -78%)
Spis treści
Functional JavaScript. Introducing Functional Programming with Underscore.js eBook -- spis treści
- Functional JavaScript
- Dedication
- Foreword by Jeremy Ashkenas
- Foreword by Steve Vinoski
- Preface
- What Is Underscore?
- Getting Underscore
- Using Underscore
- The Source Code for Functional JavaScript
- Running the Code in This Book
- Notational Conventions
- Whom Functional JavaScript Is Written For
- A Roadmap for Functional JavaScript
- Conventions Used in This Book
- Using Code Examples
- Safari Books Online
- How to Contact Us
- Acknowledgments
- What Is Underscore?
- 1. Introducing Functional JavaScript
- The Case for JavaScript
- Some Limitations of JavaScript
- Getting Started with Functional Programming
- Why Functional Programming Matters
- Functions as Units of Abstraction
- Encapsulation and Hiding
- Functions as Units of Behavior
- Data as Abstraction
- A Taste of Functional JavaScript
- On Speed
- The Case for Underscore
- Summary
- The Case for JavaScript
- 2. First-Class Functions and Applicative Programming
- Functions as First-Class Things
- JavaScripts Multiple Paradigms
- Imperative programming
- Prototype-based object-oriented programming
- Metaprogramming
- JavaScripts Multiple Paradigms
- Applicative Programming
- Collection-Centric Programming
- Other Examples of Applicative Programming
- reduceRight
- find
- reject
- all
- any
- sortBy, groupBy, and countBy
- Defining a Few Applicative Functions
- Data Thinking
- Table-Like Data
- Summary
- Functions as First-Class Things
- 3. Variable Scope and Closures
- Global Scope
- Lexical Scope
- Dynamic Scope
- JavaScripts Dynamic Scope
- Function Scope
- Closures
- Simulating Closures
- Free variables
- Shadowing
- Using Closures
- Closures as an Abstraction
- Simulating Closures
- Summary
- 4. Higher-Order Functions
- Functions That Take Other Functions
- Thinking About Passing Functions: max, finder, and best
- Tightening it up a bit
- More Thinking About Passing Functions: repeat, repeatedly, and iterateUntil
- Use functions, not values
- I said, Use functions, not values
- Thinking About Passing Functions: max, finder, and best
- Functions That Return Other Functions
- Capturing Arguments to Higher-Order Functions
- Capturing Variables for Great Good
- Take care when mutating values
- A Function to Guard Against Nonexistence: fnull
- Putting It All Together: Object Validators
- Summary
- Functions That Take Other Functions
- 5. Function-Building Functions
- The Essence of Functional Composition
- Mutation Is a Low-Level Operation
- Currying
- To Curry Right, or To Curry Left
- Automatically Currying Parameters
- Building new functions using currying
- Currying three parameters to implement HTML hex color builders
- Currying for Fluent APIs
- The Disadvantages of Currying in JavaScript
- Partial Application
- Partially Applying One and Two Known Arguments
- Partially Applying an Arbitrary Number of Arguments
- Partial Application in Action: Preconditions
- Stitching Functions End-to-End with Compose
- Pre- and Postconditions Using Composition
- Summary
- The Essence of Functional Composition
- 6. Recursion
- Self-Absorbed Functions (Functions That Call Themselves)
- Graph Walking with Recursion
- Depth-First Self-Recursive Search with Memory
- Tail (self-)recursion
- Recursion and Composing Functions: Conjoin and Disjoin
- Codependent Functions (Functions Calling Other Functions That Call Back)
- Deep Cloning with Recursion
- Walking Nested Arrays
- Too Much Recursion!
- Generators
- The Trampoline Principle and Callbacks
- Recursion Is a Low-Level Operation
- Summary
- Self-Absorbed Functions (Functions That Call Themselves)
- 7. Purity, Immutability, and Policies for Change
- Purity
- The Relationship Between Purity and Testing
- Separating the Pure from the Impure
- Property-Testing Impure Functions
- Purity and the Relationship to Referential Transparency
- Purity and the Relationship to Idempotence
- Immutability
- If a Tree Falls in the Woods, Does It Make a Sound?
- Immutability and the Relationship to Recursion
- Defensive Freezing and Cloning
- Observing Immutability at the Function Level
- Observing Immutability in Objects
- Objects Are Often a Low-Level Operation
- Policies for Controlling Change
- Summary
- Purity
- 8. Flow-Based Programming
- Chaining
- A Lazy Chain
- Promises
- Pipelining
- Data Flow versus Control Flow
- Finding a Common Shape
- A Function to Simplify Action Creation
- Summary
- Chaining
- 9. Programming Without Class
- Data Orientation
- Building Toward Functions
- Mixins
- Core Prototype Munging
- Class Hierarchies
- Changing Hierarchies
- Flattening the Hierarchy with Mixins
- New Semantics via Mixin Extension
- New Types via Mixin Mixing
- Methods Are Low-Level Operations
- }).call(Finis);
- Data Orientation
- A. Functional JavaScript in the Wild
- Functional Libraries for JavaScript
- Functional JavaScript
- Underscore-contrib
- RxJS
- Bilby
- allong.es
- Other Functional Libraries
- Functional Programming Languages Targeting JavaScript
- ClojureScript
- CoffeeScript
- Roy
- Elm
- Functional Libraries for JavaScript
- B. Annotated Bibliography
- Papers/Books/Blog Posts/Talks
- Presentations
- Blog Posts
- Journal Articles
- Index
- About the Author
- Colophon
- Copyright